![]() |
|
SFXEmitter Class Reference
[Sound]
An invisible 3D object that emits sound. More...
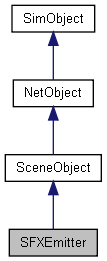
Public Member Functions | |
SFXSource | getSource () |
Get the sound source object from the emitter. | |
void | play () |
Manually start playback of the emitter's sound. | |
void | stop () |
Manually stop playback of the emitter's sound. | |
Public Attributes | |
3D Sound | |
int | coneInsideAngle |
Angle of inner volume cone of 3D sound in degrees. | |
int | coneOutsideAngle |
Angle of outer volume cone of 3D sound in degrees. | |
float | coneOutsideVolume |
Volume scale factor of outside of outer volume 3D sound cone. | |
bool | is3D |
Whether to play fileName as a positional (3D) sound or not. | |
float | maxDistance |
Distance at which to stop volume attenuation of the 3D sound. | |
float | referenceDistance |
Distance at which to start volume attenuation of the 3D sound. | |
Point3F | scatterDistance |
Bounds on random offset to apply to initial 3D sound position. | |
Sound | |
float | fadeInTime |
Number of seconds to gradually fade in volume from zero when playback starts. | |
float | fadeOutTime |
Number of seconds to gradually fade out volume down to zero when playback is stopped or paused. | |
bool | isLooping |
Whether to play fileName in an infinite loop. | |
bool | isStreaming |
Whether to use streamed playback for fileName. | |
float | pitch |
Pitch shift to apply to the sound. Default is 1 = play at normal speed. | |
bool | playOnAdd |
Whether playback of the emitter's sound should start as soon as the emitter object is added to the level. | |
SFXSource | sourceGroup |
The SFXSource to which to assign the sound of this emitter as a child. | |
bool | useTrackDescriptionOnly |
If this is true, all fields except for playOnAdd and track are ignored on the emitter object. | |
float | volume |
Volume level to apply to the sound. | |
Media | |
filename | fileName |
The sound file to play. | |
SFXTrack | track |
The track which the emitter should play. | |
Static Public Attributes | |
static bool | isRenderable |
Disables rendering of all instances of this type. | |
static bool | isSelectable |
Disables selection of all instances of this type. | |
static ColorI | renderColorInnerCone |
The color with which to render dots in the inner sound cone (Editor only). | |
static ColorI | renderColorOuterCone |
The color with which to render dots in the outer sound cone (Editor only). | |
static ColorI | renderColorOutsideVolume |
The color with which to render dots outside of the outer sound cone (Editor only). | |
static ColorI | renderColorPlayingInRange |
The color with which to render a sound emitter's marker cube in the editor when the emitter's sound is playing and in range of the listener. | |
static ColorI | renderColorPlayingOutOfRange |
The color with which to render a sound emitter's marker cube in the editor when the emitter's sound is playing but out of the range of the listener. | |
static ColorI | renderColorRangeSphere |
The color of the range sphere with which to render sound emitters in the editor. | |
static ColorI | renderColorStoppedInRange |
The color with which to render a sound emitter's marker cube in the editor when the emitter's sound is not playing but the emitter is in range of the listener. | |
static ColorI | renderColorStoppedOutOfRange |
The color with which to render a sound emitter's marker cube in the editor when the emitter's sound is not playing and the emitter is out of range of the listener. | |
static bool | renderEmitters |
Whether to render enhanced range feedback in the editor on all emitters regardless of selection state. | |
static float | renderPointDistance |
The distance between individual points in the sound emitter rendering in the editor as the points move from the emitter's center away to maxDistance. | |
static float | renderRadialIncrements |
The stepping (in degrees) for the radial sweep along the axis of the XY plane sweep for sound emitter rendering in the editor. | |
static float | renderSweepIncrements |
The stepping (in degrees) for the radial sweep on the XY plane for sound emitter rendering in the editor. |
Detailed Description
An invisible 3D object that emits sound.
Sound emitters are used to place sounds in the level. They are full 3D objects with their own position and orientation and when assigned 3D sounds, the transform and velocity of the sound emitter object will be applied to the 3D sound.
Sound emitters can be set up of in either of two ways:
-
By assigning an existing SFXTrack to the emitter's track property.
In this case the general sound setup (3D, streaming, looping, etc.) will be taken from track. However, the emitter's own properties will still override their corresponding properties in the track's SFXDescription.
-
By directly assigning a sound file to the emitter's fileName property.
In this case, the sound file will be set up for playback according to the properties defined on the emitter.
Using playOnAdd emitters can be configured to start playing immediately when they are added to the system (e.g. when the level objects are loaded from the mission file).
- Note:
- A sound emitter need not necessarily emit a 3D sound. Instead, sound emitters may also be used to play non-positional sounds. For placing background audio to a level, however, it is usually easier to use LevelInfo::soundAmbience.
Sound Emitters and Networking
It is important to be aware of the fact that sounds will only play client-side whereas SFXEmitter objects are server-side entities. This means that a server-side object has no connection to the actual sound playing on the client. It is thus not possible for the server-object to perform queries about playback status and other source-related properties as these may in fact differ from client to client.
Member Function Documentation
SFXSource SFXEmitter::getSource | ( | ) |
Get the sound source object from the emitter.
- Returns:
- The sound source used by the emitter or null.
- Note:
- This method will return null when called on the server-side SFXEmitter object. Only client-side ghosts actually hold on to SFXSources.
void SFXEmitter::play | ( | ) |
Manually start playback of the emitter's sound.
If this is called on the server-side object, the play command will be related to all client-side ghosts.
void SFXEmitter::stop | ( | ) |
Manually stop playback of the emitter's sound.
If this is called on the server-side object, the stop command will be related to all client-side ghosts.
Member Data Documentation
Angle of inner volume cone of 3D sound in degrees.
- Note:
- This field is ignored if useTrackDescriptionOnly is true.
- See also:
- SFXDescription::coneInsideAngle
Angle of outer volume cone of 3D sound in degrees.
- Note:
- This field is ignored if useTrackDescriptionOnly is true.
- See also:
- SFXDescription::coneOutsideAngle
Volume scale factor of outside of outer volume 3D sound cone.
- Note:
- This field is ignored if useTrackDescriptionOnly is true.
- See also:
- SFXDescription::coneOutsideVolume
float SFXEmitter::fadeInTime |
Number of seconds to gradually fade in volume from zero when playback starts.
- Note:
- This field is ignored if useTrackDescriptionOnly is true.
- See also:
- SFXDescription::fadeInTime
float SFXEmitter::fadeOutTime |
Number of seconds to gradually fade out volume down to zero when playback is stopped or paused.
- Note:
- This field is ignored if useTrackDescriptionOnly is true.
- See also:
- SFXDescription::fadeOutTime
filename SFXEmitter::fileName |
bool SFXEmitter::is3D |
Whether to play fileName as a positional (3D) sound or not.
If a track is assigned, the value of this field is ignored.
- See also:
- SFXDescription::is3D
Whether to play fileName in an infinite loop.
If a track is assigned, the value of this field is ignored.
- See also:
- SFXDescription::isLooping
Whether to use streamed playback for fileName.
If a track is assigned, the value of this field is ignored.
- See also:
- SFXDescription::isStreaming
float SFXEmitter::maxDistance |
Distance at which to stop volume attenuation of the 3D sound.
- Note:
- This field is ignored if useTrackDescriptionOnly is true.
- See also:
- SFXDescription::maxDistance
float SFXEmitter::pitch |
Pitch shift to apply to the sound. Default is 1 = play at normal speed.
- Note:
- This field is ignored if useTrackDescriptionOnly is true.
- See also:
- SFXDescription::pitch
Whether playback of the emitter's sound should start as soon as the emitter object is added to the level.
If this is true, the emitter will immediately start to play when the level is loaded.
Distance at which to start volume attenuation of the 3D sound.
- Note:
- This field is ignored if useTrackDescriptionOnly is true.
- See also:
- SFXDescription::referenceDistance
Point3F SFXEmitter::scatterDistance |
Bounds on random offset to apply to initial 3D sound position.
- Note:
- This field is ignored if useTrackDescriptionOnly is true.
- See also:
- SFXDescription::scatterDistance
The SFXSource to which to assign the sound of this emitter as a child.
- Note:
- This field is ignored if useTrackDescriptionOnly is true.
- See also:
- SFXDescription::sourceGroup
The track which the emitter should play.
- Note:
- If assigned, this field will take precedence over a fileName that may also be assigned to the emitter.
float SFXEmitter::volume |
Volume level to apply to the sound.
- Note:
- This field is ignored if useTrackDescriptionOnly is true.
- See also:
- SFXDescription::volume