![]() |
|
SimXMLDocument Class Reference
[File I/O]
File I/O object used for creating, reading, and writing XML documents. More...
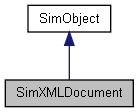
Public Member Functions | |
void | addComment (string comment) |
Add the given comment as a child of the document. | |
void | addData (string text) |
Add the given text as a child of current Element. | |
void | addHeader () |
Add a XML header to a document. | |
void | addNewElement (string name) |
Create a new element with the given name as child of current Element's parent and push it onto the Element stack making it the current one. | |
void | addText (string text) |
Add the given text as a child of current Element. | |
string | attribute (string attributeName) |
Get a string attribute from the current Element on the stack. | |
bool | attributeExists (string attributeName) |
Tests if the requested attribute exists. | |
float | attributeF32 (string attributeName) |
Get float attribute from the current Element on the stack. | |
int | attributeS32 (string attributeName) |
Get int attribute from the current Element on the stack. | |
void | clear () |
Set this document to its default state. | |
void | clearError () |
Clear the last error description. | |
string | elementValue () |
Get the Element's value if it exists. | |
string | firstAttribute () |
Obtain the name of the current Element's first attribute. | |
string | getData () |
Gets the text from the current Element. | |
string | getErrorDesc () |
Get last error description. | |
string | getText () |
Gets the text from the current Element. | |
string | lastAttribute () |
Obtain the name of the current Element's last attribute. | |
bool | loadFile (string fileName) |
Load in given filename and prepare it for use. | |
string | nextAttribute () |
Get the name of the next attribute for the current Element after a call to firstAttribute(). | |
bool | nextSiblingElement (string name) |
Put the next sibling Element with the given name on the stack, making it the current one. | |
void | parse (string xmlString) |
Create a document from a XML string. | |
void | popElement () |
Pop the last Element off the stack. | |
string | prevAttribute () |
Get the name of the previous attribute for the current Element after a call to lastAttribute(). | |
bool | pushChildElement (int index) |
Push the child Element at the given index onto the stack, making it the current one. | |
bool | pushFirstChildElement (string name) |
Push the first child Element with the given name onto the stack, making it the current Element. | |
void | pushNewElement (string name) |
Create a new element with the given name as child of current Element and push it onto the Element stack making it the current one. | |
string | readComment (int index) |
Gives the comment at the specified index, if any. | |
void | removeText () |
Remove any text on the current Element. | |
void | reset () |
Set this document to its default state. | |
bool | saveFile (string fileName) |
Save document to the given file name. | |
void | setAttribute (string attributeName, string value) |
Set the attribute of the current Element on the stack to the given value. | |
void | setObjectAttributes (string objectID) |
Add the given SimObject's fields as attributes of the current Element on the stack. |
Detailed Description
File I/O object used for creating, reading, and writing XML documents.
A SimXMLDocument is a container of various XML nodes. The Document level may contain a header (sometimes called a declaration), comments and child Elements. Elements may contain attributes, data (or text) and child Elements.
You build new Elements using addNewElement(). This makes the new Element the current one you're working with. You then use setAttribute() to add attributes to the Element. You use addData() or addText() to write to the text area of an Element.
- Example:
// Thanks to Rex Hiebert for this example // Given the following XML <?xml version="1.0" encoding="utf-8" standalone="yes" ?> <DataTables> <table tableName="2DShapes"> <rec id="1">Triangle</rec> <rec id="2">Square</rec> <rec id="3">Circle</rec> </table> <table tableName="3DShapes"> <rec id="1">Pyramid</rec> <rec id="2">Cube</rec> <rec id="3">Sphere</rec> </table> </DataTables> // Using SimXMLDocument by itself function readXmlExample(%filename) { %xml = new SimXMLDocument() {}; %xml.loadFile(%filename); %xml.pushChildElement("DataTables"); %xml.pushFirstChildElement("table"); while(true) { echo("TABLE:" SPC %xml.attribute("tableName")); %xml.pushFirstChildElement("rec"); while (true) { %id = %xml.attribute("id"); %desc = %xml.getData(); echo(" Shape" SPC %id SPC %desc); if (!%xml.nextSiblingElement("rec")) break; } %xml.popElement(); if (!%xml.nextSiblingElement("table")) break; } } // Thanks to Scott Peal for this example // Using FileObject in conjunction with SimXMLDocument // This example uses an XML file with a format of: // <Models> // <Model category="" name="" path="" /> // </Models> function getModelsInCatagory() { %file = "./Catalog.xml"; %fo = new FileObject(); %text = ""; if(%fo.openForRead(%file)) { while(!%fo.isEOF()) { %text = %text @ %fo.readLine(); if (!%fo.isEOF()) %text = %text @ "\n"; } } else { echo("Unable to locate the file: " @ %file); } %fo.delete(); %xml = new SimXMLDocument() {}; %xml.parse(%text); // "Get" inside of the root element, "Models". %xml.pushChildElement(0); // "Get" into the first child element if (%xml.pushFirstChildElement("Model")) { while (true) { // // Here, i read the element's attributes. // You might want to save these values in an array or call the %xml.getElementValue() // if you have a different XML structure. %catagory = %xml.attribute("catagory"); %name = %xml.attribute("name"); %path = %xml.attribute("path"); // now, read the next "Model" if (!%xml.nextSiblingElement("Model")) break; } } }
- Note:
- SimXMLDocument is a wrapper around TinyXml, a standard XML library. If you're familiar with its concepts, you'll find they also apply here.
- See also:
- FileObject
Member Function Documentation
void SimXMLDocument::addComment | ( | string | comment | ) |
Add the given comment as a child of the document.
- Parameters:
-
comment String containing the comment.
- Example:
// Create a new XML document with a header, a comment and single element. %x = new SimXMLDocument(); %x.addHeader(); %x.addComment("This is a test comment"); %x.addNewElement("NewElement"); %x.saveFile("test.xml"); // Produces the following file: // <?xml version="1.0" encoding="utf-8" standalone="yes" ?> // <!--This is a test comment--> // <NewElement />
- See also:
- readComment()
void SimXMLDocument::addData | ( | string | text | ) |
Add the given text as a child of current Element.
Use getData() to retrieve any text from the current Element.
addData() and addText() may be used interchangeably. As there is no difference between data and text, you may also use removeText() to clear any data from the current Element.
- Parameters:
-
text String containing the text.
- Example:
// Create a new XML document with a header and single element // with some added data. %x = new SimXMLDocument(); %x.addHeader(); %x.addNewElement("NewElement"); %x.addData("Some text"); %x.saveFile("test.xml"); // Produces the following file: // <?xml version="1.0" encoding="utf-8" standalone="yes" ?> // <NewElement>Some text</NewElement>
- See also:
- getData()
- addText()
- getText()
- removeText()
void SimXMLDocument::addHeader | ( | ) |
Add a XML header to a document.
Sometimes called a declaration, you typically add a standard header to the document before adding any elements. SimXMLDocument always produces the following header:
<?xml version="1.0" encoding="utf-8" standalone="yes" ?>
- Example:
// Create a new XML document with just a header and single element. %x = new SimXMLDocument(); %x.addHeader(); %x.addNewElement("NewElement"); %x.saveFile("test.xml"); // Produces the following file: // <?xml version="1.0" encoding="utf-8" standalone="yes" ?> // <NewElement />
void SimXMLDocument::addNewElement | ( | string | name | ) |
Create a new element with the given name as child of current Element's parent and push it onto the Element stack making it the current one.
- Note:
- This differs from pushNewElement() in that it adds the new Element to the current Element's parent (or document if there is no parent Element). This makes the new Element a sibling of the current one.
- Parameters:
-
name XML tag for the new Element.
- See also:
- pushNewElement()
void SimXMLDocument::addText | ( | string | text | ) |
Add the given text as a child of current Element.
Use getText() to retrieve any text from the current Element and removeText() to clear any text.
addText() and addData() may be used interchangeably.
- Parameters:
-
text String containing the text.
- Example:
// Create a new XML document with a header and single element // with some added text. %x = new SimXMLDocument(); %x.addHeader(); %x.addNewElement("NewElement"); %x.addText("Some text"); %x.saveFile("test.xml"); // Produces the following file: // <?xml version="1.0" encoding="utf-8" standalone="yes" ?> // <NewElement>Some text</NewElement>
- See also:
- getText()
- removeText()
- addData()
- getData()
string SimXMLDocument::attribute | ( | string | attributeName | ) |
Get a string attribute from the current Element on the stack.
- Parameters:
-
attributeName Name of attribute to retrieve.
- Returns:
- The attribute string if found. Otherwise returns an empty string.
bool SimXMLDocument::attributeExists | ( | string | attributeName | ) |
Tests if the requested attribute exists.
- Parameters:
-
attributeName Name of attribute being queried for.
- Returns:
- True if the attribute exists.
float SimXMLDocument::attributeF32 | ( | string | attributeName | ) |
Get float attribute from the current Element on the stack.
- Parameters:
-
attributeName Name of attribute to retrieve.
- Returns:
- The value of the given attribute in the form of a float.
int SimXMLDocument::attributeS32 | ( | string | attributeName | ) |
Get int attribute from the current Element on the stack.
- Parameters:
-
attributeName Name of attribute to retrieve.
- Returns:
- The value of the given attribute in the form of an integer.
void SimXMLDocument::clear | ( | ) |
void SimXMLDocument::clearError | ( | ) |
Clear the last error description.
string SimXMLDocument::elementValue | ( | ) |
Get the Element's value if it exists.
Usually returns the text from the Element.
- Returns:
- The value from the Element, or an empty string if none is found.
string SimXMLDocument::firstAttribute | ( | ) |
Obtain the name of the current Element's first attribute.
- Returns:
- String containing the first attribute's name, or an empty string if none is found.
string SimXMLDocument::getData | ( | ) |
Gets the text from the current Element.
Use addData() to add text to the current Element.
getData() and getText() may be used interchangeably. As there is no difference between data and text, you may also use removeText() to clear any data from the current Element.
- Returns:
- String containing the text in the current Element.
- Example:
// Using the following test.xml file as an example: // <?xml version="1.0" encoding="utf-8" standalone="yes" ?> // <NewElement>Some data</NewElement> // Load in the file %x = new SimXMLDocument(); %x.loadFile("test.xml"); // Make the first Element the current one %x.pushFirstChildElement("NewElement"); // Store the current Element's data ('Some data' in this example) // into 'result' %result = %x.getData(); echo( %result );
- See also:
- addData()
- addText()
- getText()
- removeText()
string SimXMLDocument::getErrorDesc | ( | ) |
Get last error description.
- Returns:
- A string of the last error message.
string SimXMLDocument::getText | ( | ) |
Gets the text from the current Element.
Use addText() to add text to the current Element and removeText() to clear any text.
getText() and getData() may be used interchangeably.
- Returns:
- String containing the text in the current Element.
- Example:
// Using the following test.xml file as an example: // <?xml version="1.0" encoding="utf-8" standalone="yes" ?> // <NewElement>Some text</NewElement> // Load in the file %x = new SimXMLDocument(); %x.loadFile("test.xml"); // Make the first Element the current one %x.pushFirstChildElement("NewElement"); // Store the current Element's text ('Some text' in this example) // into 'result' %result = %x.getText(); echo( %result );
- See also:
- addText()
- removeText()
- addData()
- getData()
string SimXMLDocument::lastAttribute | ( | ) |
Obtain the name of the current Element's last attribute.
- Returns:
- String containing the last attribute's name, or an empty string if none is found.
bool SimXMLDocument::loadFile | ( | string | fileName | ) |
Load in given filename and prepare it for use.
- Note:
- Clears the current document's contents.
- Parameters:
-
fileName Name and path of XML document
- Returns:
- True if the file was loaded successfully.
string SimXMLDocument::nextAttribute | ( | ) |
Get the name of the next attribute for the current Element after a call to firstAttribute().
- Returns:
- String containing the next attribute's name, or an empty string if none is found.
bool SimXMLDocument::nextSiblingElement | ( | string | name | ) |
Put the next sibling Element with the given name on the stack, making it the current one.
- Parameters:
-
name String containing name of the next sibling.
- Returns:
- True if the Element was found and made the current one.
void SimXMLDocument::parse | ( | string | xmlString | ) |
Create a document from a XML string.
- Note:
- Clears the current document's contents.
- Parameters:
-
xmlString Valid XML to parse and store as a document.
void SimXMLDocument::popElement | ( | ) |
Pop the last Element off the stack.
string SimXMLDocument::prevAttribute | ( | ) |
Get the name of the previous attribute for the current Element after a call to lastAttribute().
- Returns:
- String containing the previous attribute's name, or an empty string if none is found.
bool SimXMLDocument::pushChildElement | ( | int | index | ) |
Push the child Element at the given index onto the stack, making it the current one.
- Parameters:
-
index Numerical index of Element being pushed.
- Returns:
- True if the Element was found and made the current one.
bool SimXMLDocument::pushFirstChildElement | ( | string | name | ) |
Push the first child Element with the given name onto the stack, making it the current Element.
- Parameters:
-
name String containing name of the child Element.
- Returns:
- True if the Element was found and made the current one.
- Example:
// Using the following test.xml file as an example: // <?xml version="1.0" encoding="utf-8" standalone="yes" ?> // <NewElement>Some text</NewElement> // Load in the file %x = new SimXMLDocument(); %x.loadFile("test.xml"); // Make the first Element the current one %x.pushFirstChildElement("NewElement"); // Store the current Element's text ('Some text' in this example) // into 'result' %result = %x.getText(); echo( %result );
void SimXMLDocument::pushNewElement | ( | string | name | ) |
Create a new element with the given name as child of current Element and push it onto the Element stack making it the current one.
- Note:
- This differs from addNewElement() in that it adds the new Element as a child of the current Element (or a child of the document if no Element exists).
- Parameters:
-
name XML tag for the new Element.
- See also:
- addNewElement()
string SimXMLDocument::readComment | ( | int | index | ) |
Gives the comment at the specified index, if any.
Unlike addComment() that only works at the document level, readComment() may read comments from the document or any child Element. The current Element (or document if no Elements have been pushed to the stack) is the parent for any comments, and the provided index is the number of comments in to read back.
- Parameters:
-
index Comment index number to query from the current Element stack
- Returns:
- String containing the comment, or an empty string if no comment is found.
- See also:
- addComment()
void SimXMLDocument::removeText | ( | ) |
void SimXMLDocument::reset | ( | ) |
bool SimXMLDocument::saveFile | ( | string | fileName | ) |
Save document to the given file name.
- Parameters:
-
fileName Path and name of XML file to save to.
- Returns:
- True if the file was successfully saved.
void SimXMLDocument::setAttribute | ( | string | attributeName, | |
string | value | |||
) |
Set the attribute of the current Element on the stack to the given value.
- Parameters:
-
attributeName Name of attribute being changed value New value to assign to the attribute
void SimXMLDocument::setObjectAttributes | ( | string | objectID | ) |
Add the given SimObject's fields as attributes of the current Element on the stack.
- Parameters:
-
objectID ID of SimObject being copied.