![]() |
|
VehicleData Class Reference
[Vehicles]
Base properties shared by all Vehicles (FlyingVehicle, HoverVehicle, WheeledVehicle). More...
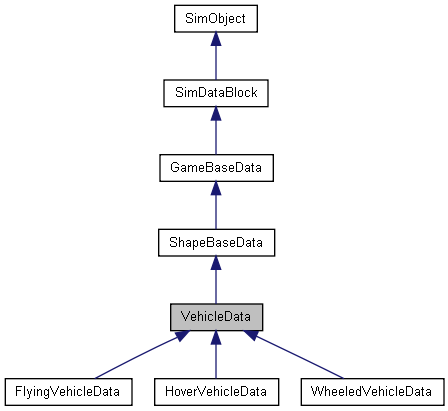
Public Member Functions | |
Callbacks | |
void | onEnterLiquid (Vehicle obj, float coverage, string type) |
Called when the vehicle enters liquid. | |
void | onLeaveLiquid (Vehicle obj, string type) |
Called when the vehicle leaves liquid. | |
Public Attributes | |
float | bodyFriction |
Collision friction coefficient. | |
float | bodyRestitution |
Collision 'bounciness'. | |
float | cameraDecay |
How quickly the camera moves back towards the vehicle when stopped. | |
float | cameraLag |
How much the camera lags behind the vehicle depending on vehicle speed. | |
float | cameraOffset |
Vertical (Z axis) height of the camera above the vehicle. | |
bool | cameraRoll |
If true, the camera will roll with the vehicle. If false, the camera will always have the positive Z axis as up. | |
float | collDamageMultiplier |
Damage to this vehicle after a collision (multiplied by collision velocity). | |
float | collDamageThresholdVel |
Minimum collision velocity to cause damage to this vehicle. | |
float | collisionTol |
Minimum distance between objects for them to be considered as colliding. | |
float | contactTol |
Maximum relative velocity between objects for collisions to be resolved as contacts. | |
ParticleEmitterData | damageEmitter [3] |
Array of particle emitters used to generate damage (dust, smoke etc) effects. | |
Point3F | damageEmitterOffset [2] |
Object space "x y z" offsets used to emit particles for the active damageEmitter. | |
float | damageLevelTolerance [2] |
Damage levels (as a percentage of maxDamage) above which to begin emitting particles from the associated damageEmitter. | |
ParticleEmitterData | dustEmitter |
Dust particle emitter. | |
float | dustHeight |
Height above ground at which to emit particles from the dustEmitter. | |
SFXProfile | exitingWater |
Sound to play when exiting the water. | |
float | exitSplashSoundVelocity |
Minimum velocity when leaving the water for the exitingWater sound to play. | |
SFXProfile | hardImpactSound |
Sound to play on a 'hard' impact. | |
float | hardImpactSpeed |
Minimum collision speed for the hardImpactSound to be played. | |
float | hardSplashSoundVelocity |
Minimum velocity when entering the water for the imapactWaterHard sound to play. | |
SFXProfile | impactWaterEasy |
Sound to play when entering the water with speed >= softSplashSoundVelocity and < mediumSplashSoundVelocity. | |
SFXProfile | impactWaterHard |
Sound to play when entering the water with speed >= hardSplashSoundVelocity. | |
SFXProfile | impactWaterMedium |
Sound to play when entering the water with speed >= mediumSplashSoundVelocity and < hardSplashSoundVelocity. | |
int | integration |
Number of integration steps per tick. | |
float | jetEnergyDrain |
Energy amount to drain for each tick the vehicle is jetting. | |
float | jetForce |
Additional force applied to the vehicle when it is jetting. | |
Point3F | massBox |
Define the box used to estimate the vehicle's moment of inertia. | |
Point3F | massCenter |
Defines the vehicle's center of mass (offset from the origin of the model). | |
float | maxDrag |
Maximum drag coefficient. | |
float | maxSteeringAngle |
Maximum yaw (horizontal) and pitch (vertical) steering angle in radians. | |
float | mediumSplashSoundVelocity |
Minimum velocity when entering the water for the imapactWaterMedium sound to play. | |
float | minDrag |
Minimum drag coefficient. | |
float | minImpactSpeed |
Minimum collision speed for the onImpact callback to be invoked. | |
float | minJetEnergy |
Minimum vehicle energy level to begin jetting. | |
float | minRollSpeed |
Unused. | |
float | numDmgEmitterAreas |
Number of damageEmitterOffset values to use for each damageEmitter. | |
SFXProfile | softImpactSound |
Sound to play on a 'soft' impact. | |
float | softImpactSpeed |
Minimum collision speed for the softImpactSound to be played. | |
float | softSplashSoundVelocity |
Minimum velocity when entering the water for the imapactWaterEasy sound to play. | |
ParticleEmitterData | splashEmitter [2] |
Array of particle emitters used to generate splash effects. | |
float | splashFreqMod |
Number of splash particles to generate based on vehicle speed. | |
float | splashVelEpsilon |
Minimum speed when moving through water to generate splash particles. | |
float | triggerDustHeight |
Maximum height above surface to emit dust particles. | |
SFXProfile | waterWakeSound |
Looping sound to play while moving through the water. |
Detailed Description
Base properties shared by all Vehicles (FlyingVehicle, HoverVehicle, WheeledVehicle).
This datablock defines properties shared by all Vehicle types, but should not be instantiated directly. Instead, set the desired properties in the FlyingVehicleData, HoverVehicleData or WheeledVehicleData datablock.
Damage
The VehicleData class extends the basic energy/damage functionality provided by ShapeBaseData to include damage from collisions, as well as particle emitters activated automatically when damage levels reach user specified thresholds.
The example below shows how to setup a Vehicle to:
- take damage when colliding with another object
- emit gray smoke particles from two locations on the Vehicle when damaged above 50%
- emit black smoke particles from two locations on the Vehicle when damaged above 85%
- emit bubbles when any active damage emitter point is underwater
- Example:
// damage from collisions collDamageMultiplier = 0.05; collDamageThresholdVel = 15; // damage levels damageLevelTolerance[0] = 0.5; damageEmitter[0] = GraySmokeEmitter; // emitter used when damage is >= 50% damageLevelTolerance[1] = 0.85; damageEmitter[1] = BlackSmokeEmitter; // emitter used when damage is >= 85% damageEmitter[2] = DamageBubbleEmitter; // emitter used instead of damageEmitter[0:1] // when offset point is underwater // emit offsets (used for all active damage level emitters) damageEmitterOffset[0] = "0.5 3 1"; damageEmitterOffset[1] = "-0.5 3 1"; numDmgEmitterAreas = 2;
Member Function Documentation
void VehicleData::onEnterLiquid | ( | Vehicle | obj, | |
float | coverage, | |||
string | type | |||
) |
Called when the vehicle enters liquid.
- Parameters:
-
obj the Vehicle object coverage percentage of the vehicle's bounding box covered by the liquid type type of liquid the vehicle has entered
void VehicleData::onLeaveLiquid | ( | Vehicle | obj, | |
string | type | |||
) |
Called when the vehicle leaves liquid.
- Parameters:
-
obj the Vehicle object type type of liquid the vehicle has left
Member Data Documentation
Collision friction coefficient.
How well this object will slide against objects it collides with.
Collision 'bounciness'.
Normally in the range 0 (not bouncy at all) to 1 (100% bounciness).
float VehicleData::cameraDecay |
How quickly the camera moves back towards the vehicle when stopped.
- See also:
- cameraLag.
float VehicleData::cameraLag |
How much the camera lags behind the vehicle depending on vehicle speed.
Increasing this value will make the camera fall further behind the vehicle as it accelerates away.
- See also:
- cameraDecay.
Vertical (Z axis) height of the camera above the vehicle.
If true, the camera will roll with the vehicle. If false, the camera will always have the positive Z axis as up.
Damage to this vehicle after a collision (multiplied by collision velocity).
Currently unused.
Minimum collision velocity to cause damage to this vehicle.
Currently unused.
Minimum distance between objects for them to be considered as colliding.
float VehicleData::contactTol |
Maximum relative velocity between objects for collisions to be resolved as contacts.
Velocities greater than this are handled as collisions.
Array of particle emitters used to generate damage (dust, smoke etc) effects.
Currently, the first two emitters (indices 0 and 1) are used when the damage level exceeds the associated damageLevelTolerance. The 3rd emitter is used when the emitter point is underwater.
- See also:
- damageEmitterOffset
Point3F VehicleData::damageEmitterOffset[2] |
Object space "x y z" offsets used to emit particles for the active damageEmitter.
- Example:
// damage levels damageLevelTolerance[0] = 0.5; damageEmitter[0] = SmokeEmitter; // emit offsets (used for all active damage level emitters) damageEmitterOffset[0] = "0.5 3 1"; damageEmitterOffset[1] = "-0.5 3 1"; numDmgEmitterAreas = 2;
float VehicleData::damageLevelTolerance[2] |
Damage levels (as a percentage of maxDamage) above which to begin emitting particles from the associated damageEmitter.
Levels should be in order of increasing damage.
- See also:
- damageEmitterOffset
Dust particle emitter.
- See also:
- triggerDustHeight
- dustHeight
float VehicleData::dustHeight |
Height above ground at which to emit particles from the dustEmitter.
Sound to play when exiting the water.
Minimum velocity when leaving the water for the exitingWater sound to play.
Sound to play on a 'hard' impact.
This sound is played if the impact speed >= hardImpactSpeed.
- See also:
- hardImpactSpeed
Minimum collision speed for the hardImpactSound to be played.
Minimum velocity when entering the water for the imapactWaterHard sound to play.
- See also:
- impactWaterHard
Sound to play when entering the water with speed >= softSplashSoundVelocity and < mediumSplashSoundVelocity.
Sound to play when entering the water with speed >= hardSplashSoundVelocity.
Sound to play when entering the water with speed >= mediumSplashSoundVelocity and < hardSplashSoundVelocity.
Number of integration steps per tick.
Increase this to improve simulation stability (also increases simulation processing time).
Energy amount to drain for each tick the vehicle is jetting.
Once the vehicle's energy level reaches 0, it will no longer be able to jet.
float VehicleData::jetForce |
Additional force applied to the vehicle when it is jetting.
For WheeledVehicles, the force is applied in the forward direction. For FlyingVehicles, the force is applied in the thrust direction.
Point3F VehicleData::massBox |
Define the box used to estimate the vehicle's moment of inertia.
Currently only used by WheeledVehicle; other vehicle types use a unit sphere to compute inertia.
Point3F VehicleData::massCenter |
Defines the vehicle's center of mass (offset from the origin of the model).
float VehicleData::maxDrag |
Maximum drag coefficient.
Currently unused.
Maximum yaw (horizontal) and pitch (vertical) steering angle in radians.
Minimum velocity when entering the water for the imapactWaterMedium sound to play.
- See also:
- impactWaterMedium
float VehicleData::minDrag |
Minimum drag coefficient.
Currently only used by FlyingVehicle.
Minimum collision speed for the onImpact callback to be invoked.
Minimum vehicle energy level to begin jetting.
Unused.
Number of damageEmitterOffset values to use for each damageEmitter.
- See also:
- damageEmitterOffset
Sound to play on a 'soft' impact.
This sound is played if the impact speed is < hardImpactSpeed and >= softImpactSpeed.
- See also:
- softImpactSpeed
Minimum collision speed for the softImpactSound to be played.
Minimum velocity when entering the water for the imapactWaterEasy sound to play.
- See also:
- impactWaterEasy
Array of particle emitters used to generate splash effects.
Number of splash particles to generate based on vehicle speed.
This value is multiplied by the current speed to determine how many particles to generate each frame.
Minimum speed when moving through water to generate splash particles.
Maximum height above surface to emit dust particles.
If the vehicle is less than triggerDustHeight above a static surface with a material that has 'showDust' set to true, the vehicle will emit particles from the dustEmitter.
Looping sound to play while moving through the water.