![]() |
|
GuiMenuBar Class Reference
[Core Controls]
GUI Control which displays a horizontal bar with individual drop-down menu items. Each menu item may also have submenu items. More...
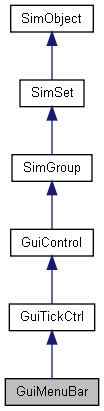
Public Member Functions | |
void | addMenu (string menuText, int menuId) |
Adds a new menu to the menu bar. | |
void | addMenuItem (string targetMenu="", string menuItemText="", int menuItemId=0, string accelerator=NULL, int checkGroup=-1) |
Adds a menu item to the specified menu. The menu argument can be either the text of a menu or its id. | |
void | addSubmenuItem (string menuTarget, string menuItem, string submenuItemText, int submenuItemId, string accelerator, int checkGroup) |
Adds a menu item to the specified menu. The menu argument can be either the text of a menu or its id. | |
void | clearMenuItems (string menuTarget) |
Removes all the menu items from the specified menu. | |
void | clearMenus (int param1, int param2) |
Clears all the menus from the menu bar. | |
void | clearSubmenuItems (string menuTarget, string menuItem) |
Removes all the menu items from the specified submenu. | |
void | removeMenu (string menuTarget) |
Removes the specified menu from the menu bar. | |
void | removeMenuItem (string menuTarget, string menuItemTarget) |
Removes the specified menu item from the menu. | |
void | setCheckmarkBitmapIndex (int bitmapindex) |
Sets the menu bitmap index for the check mark image. | |
void | setMenuBitmapIndex (string menuTarget, int bitmapindex, bool bitmaponly, bool drawborder) |
Sets the bitmap index for the menu and toggles rendering only the bitmap. | |
void | setMenuItemBitmap (string menuTarget, string menuItemTarget, int bitmapIndex) |
Sets the specified menu item bitmap index in the bitmap array. Setting the item's index to -1 will remove any bitmap. | |
void | setMenuItemChecked (string menuTarget, string menuItemTarget, bool checked) |
Sets the menu item bitmap to a check mark, which by default is the first element in the bitmap array (although this may be changed with setCheckmarkBitmapIndex()). Any other menu items in the menu with the same check group become unchecked if they are checked. | |
void | setMenuItemEnable (string menuTarget, string menuItemTarget, bool enabled) |
sets the menu item to enabled or disabled based on the enable parameter. The specified menu and menu item can either be text or ids. | |
void | setMenuItemSubmenuState (string menuTarget, string menuItem, bool isSubmenu) |
Sets the given menu item to be a submenu. | |
void | setMenuItemText (string menuTarget, string menuItemTarget, string newMenuItemText) |
Sets the text of the specified menu item to the new string. | |
void | setMenuItemVisible (string menuTarget, string menuItemTarget, bool isVisible) |
Brief Description. | |
void | setMenuMargins (int horizontalMargin, int verticalMargin, int bitmapToTextSpacing) |
Sets the menu rendering margins: horizontal, vertical, bitmap spacing. | |
void | setMenuText (string menuTarget, string newMenuText) |
Sets the text of the specified menu to the new string. | |
void | setMenuVisible (string menuTarget, bool visible) |
Sets the whether or not to display the specified menu. | |
void | setSubmenuItemChecked (string menuTarget, string menuItemTarget, string submenuItemText, bool checked) |
Sets the menu item bitmap to a check mark, which by default is the first element in the bitmap array (although this may be changed with setCheckmarkBitmapIndex()). Any other menu items in the menu with the same check group become unchecked if they are checked. | |
Callbacks | |
void | onMenuItemSelect (string menuId, string menuText, string menuItemId, string menuItemText) |
Called whenever an item in a menu is selected. | |
void | onMenuSelect (string menuId, string menuText) |
Called whenever a menu is selected. | |
void | onMouseInMenu (bool isInMenu) |
Called whenever the mouse enters, or persists is in the menu. | |
void | onSubmenuSelect (string submenuId, string submenuText) |
Called whenever a submenu is selected. | |
Public Attributes | |
int | padding |
Extra padding to add to the bounds of the control. |
Detailed Description
GUI Control which displays a horizontal bar with individual drop-down menu items. Each menu item may also have submenu items.
- Example:
new GuiMenuBar(newMenuBar) { Padding = "0"; //Properties not specific to this control have been omitted from this example. }; // Add a menu to the menu bar newMenuBar.addMenu(0,"New Menu"); // Add a menu item to the New Menu newMenuBar.addMenuItem(0,"New Menu Item",0,"n",-1); // Add a submenu item to the New Menu Item newMenuBar.addSubmenuItem(0,1,"New Submenu Item",0,"s",-1);
- See also:
- GuiTickCtrl
Member Function Documentation
void GuiMenuBar::addMenu | ( | string | menuText, | |
int | menuId | |||
) |
Adds a new menu to the menu bar.
- Parameters:
-
menuText Text to display for the new menu item. menuId ID for the new menu item.
- Example:
// Define the menu text %menuText = "New Menu"; // Define the menu ID. %menuId = "2"; // Inform the GuiMenuBar control to add the new menu %thisGuiMenuBar.addMenu(%menuText,%menuId);
- See also:
- GuiTickCtrl
void GuiMenuBar::addMenuItem | ( | string | targetMenu = "" , |
|
string | menuItemText = "" , |
|||
int | menuItemId = 0 , |
|||
string | accelerator = NULL , |
|||
int | checkGroup = -1 | |||
) |
Adds a menu item to the specified menu. The menu argument can be either the text of a menu or its id.
- Parameters:
-
menu Menu name or menu Id to add the new item to. menuItemText Text for the new menu item. menuItemId Id for the new menu item. accelerator Accelerator key for the new menu item. checkGroup Check group to include this menu item in.
- Example:
// Define the menu we wish to add the item to %targetMenu = "New Menu"; or %menu = "4"; // Define the text for the new menu item %menuItemText = "Menu Item"; // Define the id for the new menu item %menuItemId = "3"; // Set the accelerator key to toggle this menu item with %accelerator = "n"; // Define the Check Group that this menu item will be in, if we want it to be in a check group. -1 sets it in no check group. %checkGroup = "4"; // Inform the GuiMenuBar control to add the new menu item with the defined fields %thisGuiMenuBar.addMenuItem(%menu,%menuItemText,%menuItemId,%accelerator,%checkGroup);
- See also:
- GuiTickCtrl
void GuiMenuBar::addSubmenuItem | ( | string | menuTarget, | |
string | menuItem, | |||
string | submenuItemText, | |||
int | submenuItemId, | |||
string | accelerator, | |||
int | checkGroup | |||
) |
Adds a menu item to the specified menu. The menu argument can be either the text of a menu or its id.
- Parameters:
-
menuTarget Menu to affect a submenu in menuItem Menu item to affect submenuItemText Text to show for the new submenu submenuItemId Id for the new submenu accelerator Accelerator key for the new submenu checkGroup Which check group the new submenu should be in, or -1 for none.
- Example:
// Define the menuTarget %menuTarget = "New Menu"; or %menuTarget = "3"; // Define the menuItem %menuItem = "New Menu Item"; or %menuItem = "5"; // Define the text for the new submenu %submenuItemText = "New Submenu Item"; // Define the id for the new submenu %submenuItemId = "4"; // Define the accelerator key for the new submenu %accelerator = "n"; // Define the checkgroup for the new submenu %checkgroup = "7"; // Request the GuiMenuBar control to add the new submenu with the defined information %thisGuiMenuBar.addSubmenuItem(%menuTarget,%menuItem,%submenuItemText,%submenuItemId,%accelerator,%checkgroup);
- See also:
- GuiTickCtrl
void GuiMenuBar::clearMenuItems | ( | string | menuTarget | ) |
Removes all the menu items from the specified menu.
- Parameters:
-
menuTarget Menu to remove all items from
- Example:
// Define the menuTarget %menuTarget = "New Menu"; or %menuTarget = "3"; // Inform the GuiMenuBar control to clear all menu items from the defined menu %thisGuiMenuBar.clearMenuItems(%menuTarget);
- See also:
- GuiTickCtrl
void GuiMenuBar::clearMenus | ( | int | param1, | |
int | param2 | |||
) |
Clears all the menus from the menu bar.
- Example:
// Inform the GuiMenuBar control to clear all menus from itself. %thisGuiMenuBar.clearMenus();
- See also:
- GuiTickCtrl
void GuiMenuBar::clearSubmenuItems | ( | string | menuTarget, | |
string | menuItem | |||
) |
Removes all the menu items from the specified submenu.
- Parameters:
-
menuTarget Menu to affect a submenu in menuItem Menu item to affect
- Example:
// Define the menuTarget %menuTarget = "New Menu"; or %menuTarget = "3"; // Define the menuItem %menuItem = "New Menu Item"; or %menuItem = "5"; // Inform the GuiMenuBar to remove all submenu items from the defined menu item %thisGuiMenuBar.clearSubmenuItems(%menuTarget,%menuItem);
- See also:
- GuiControl
void GuiMenuBar::onMenuItemSelect | ( | string | menuId, | |
string | menuText, | |||
string | menuItemId, | |||
string | menuItemText | |||
) |
Called whenever an item in a menu is selected.
- Parameters:
-
menuId Index id of the menu which contains the selected menu item menuText Text of the menu which contains the selected menu item menuItemId Index id of the selected menu item menuItemText Text of the selected menu item
- Example:
// A menu item has been selected, causing the callback to occur. GuiMenuBar::onMenuItemSelect(%this,%menuId,%menuText,%menuItemId,%menuItemText) { // Code to run when the callback occurs }
- See also:
- GuiTickCtrl
void GuiMenuBar::onMenuSelect | ( | string | menuId, | |
string | menuText | |||
) |
Called whenever a menu is selected.
- Parameters:
-
menuId Index id of the clicked menu menuText Text of the clicked menu
- Example:
// A menu has been selected, causing the callback to occur. GuiMenuBar::onMenuSelect(%this,%menuId,%menuText) { // Code to run when the callback occurs }
- See also:
- GuiTickCtrl
void GuiMenuBar::onMouseInMenu | ( | bool | isInMenu | ) |
Called whenever the mouse enters, or persists is in the menu.
- Parameters:
-
isInMenu True if the mouse has entered the menu, otherwise is false.
- Note:
- To receive this callback, call setProcessTicks(true) on the menu bar.
- Example:
// Mouse enters or persists within the menu, causing the callback to occur. GuiMenuBar::onMouseInMenu(%this,%hasLeftMenu) { // Code to run when the callback occurs }
- See also:
- GuiTickCtrl
void GuiMenuBar::onSubmenuSelect | ( | string | submenuId, | |
string | submenuText | |||
) |
Called whenever a submenu is selected.
- Parameters:
-
submenuId Id of the selected submenu submenuText Text of the selected submenu
- Example:
GuiMenuBar::onSubmenuSelect(%this,%submenuId,%submenuText) { // Code to run when the callback occurs }
- See also:
- GuiTickCtrl
void GuiMenuBar::removeMenu | ( | string | menuTarget | ) |
Removes the specified menu from the menu bar.
- Parameters:
-
menuTarget Menu to remove from the menu bar
- Example:
// Define the menuTarget %menuTarget = "New Menu"; or %menuTarget = "3"; // Inform the GuiMenuBar to remove the defined menu from the menu bar %thisGuiMenuBar.removeMenu(%menuTarget);
- See also:
- GuiTickCtrl
void GuiMenuBar::removeMenuItem | ( | string | menuTarget, | |
string | menuItemTarget | |||
) |
Removes the specified menu item from the menu.
- Parameters:
-
menuTarget Menu to affect the menu item in menuItem Menu item to affect
- Example:
// Define the menuTarget %menuTarget = "New Menu"; or %menuTarget = "3"; // Define the menuItem %menuItem = "New Menu Item"; or %menuItem = "5"; // Request the GuiMenuBar control to remove the define menu item %thisGuiMenuBar.removeMenuItem(%menuTarget,%menuItem);
- See also:
- GuiTickCtrl
void GuiMenuBar::setCheckmarkBitmapIndex | ( | int | bitmapindex | ) |
Sets the menu bitmap index for the check mark image.
- Parameters:
-
bitmapIndex Bitmap index for the check mark image.
- Example:
// Define the bitmap index %bitmapIndex = "2"; // Inform the GuiMenuBar control of the proper bitmap index for the check mark image %thisGuiMenuBar.setCheckmarkBitmapIndex(%bitmapIndex);
- See also:
- GuiTickCtrl
void GuiMenuBar::setMenuBitmapIndex | ( | string | menuTarget, | |
int | bitmapindex, | |||
bool | bitmaponly, | |||
bool | drawborder | |||
) |
Sets the bitmap index for the menu and toggles rendering only the bitmap.
- Parameters:
-
menuTarget Menu to affect bitmapindex Bitmap index to set for the menu bitmaponly If true, only the bitmap will be rendered drawborder If true, a border will be drawn around the menu.
- Example:
// Define the menuTarget to affect %menuTarget = "New Menu"; or %menuTarget = "3"; // Set the bitmap index %bitmapIndex = "5"; // Set if we are only to render the bitmap or not %bitmaponly = "true"; // Set if we are rendering a border or not %drawborder = "true"; // Inform the GuiMenuBar of the bitmap and rendering changes %thisGuiMenuBar.setMenuBitmapIndex(%menuTarget,%bitmapIndex,%bitmapOnly,%drawBorder);
- See also:
- GuiTickCtrl
void GuiMenuBar::setMenuItemBitmap | ( | string | menuTarget, | |
string | menuItemTarget, | |||
int | bitmapIndex | |||
) |
Sets the specified menu item bitmap index in the bitmap array. Setting the item's index to -1 will remove any bitmap.
- Parameters:
-
menuTarget Menu to affect the menuItem in menuItem Menu item to affect bitmapIndex Bitmap index to set the menu item to
- Example:
// Define the menuTarget %menuTarget = "New Menu"; or %menuTarget = "3"; // Define the menuItem" %menuItem = "New Menu Item"; or %menuItem = "2"; // Define the bitmapIndex %bitmapIndex = "6"; // Inform the GuiMenuBar control to set the menu item to the defined bitmap %thisGuiMenuBar.setMenuItemBitmap(%menuTarget,%menuItem,%bitmapIndex);
- See also:
- GuiTickCtrl
void GuiMenuBar::setMenuItemChecked | ( | string | menuTarget, | |
string | menuItemTarget, | |||
bool | checked | |||
) |
Sets the menu item bitmap to a check mark, which by default is the first element in the bitmap array (although this may be changed with setCheckmarkBitmapIndex()). Any other menu items in the menu with the same check group become unchecked if they are checked.
- Parameters:
-
menuTarget Menu to work in menuItem Menu item to affect checked Whether we are setting it to checked or not
- Example:
- Returns:
- If not void, return value and description
- See also:
- References
void GuiMenuBar::setMenuItemEnable | ( | string | menuTarget, | |
string | menuItemTarget, | |||
bool | enabled | |||
) |
sets the menu item to enabled or disabled based on the enable parameter. The specified menu and menu item can either be text or ids.
Detailed description
- Parameters:
-
menuTarget Menu to work in menuItemTarget The menu item inside of the menu to enable or disable enabled Boolean enable / disable value.
- Example:
// Define the menu %menu = "New Menu"; or %menu = "4"; // Define the menu item %menuItem = "New Menu Item"; or %menuItem = "2"; // Define the enabled state %enabled = "true"; // Inform the GuiMenuBar control to set the enabled state of the requested menu item %thisGuiMenuBar.setMenuItemEnable(%menu,%menuItme,%enabled);
- See also:
- GuiTickCtrl
void GuiMenuBar::setMenuItemSubmenuState | ( | string | menuTarget, | |
string | menuItem, | |||
bool | isSubmenu | |||
) |
Sets the given menu item to be a submenu.
- Parameters:
-
menuTarget Menu to affect a submenu in menuItem Menu item to affect isSubmenu Whether or not the menuItem will become a subMenu or not
- Example:
// Define the menuTarget %menuTarget = "New Menu"; or %menuTarget = "3"; // Define the menuItem %menuItem = "New Menu Item"; or %menuItem = "5"; // Define whether or not the Menu Item is a sub menu or not %isSubmenu = "true"; // Inform the GuiMenuBar control to set the defined menu item to be a submenu or not. %thisGuiMenuBar.setMenuItemSubmenuState(%menuTarget,%menuItem,%isSubmenu);
- See also:
- GuiTickCtrl
void GuiMenuBar::setMenuItemText | ( | string | menuTarget, | |
string | menuItemTarget, | |||
string | newMenuItemText | |||
) |
Sets the text of the specified menu item to the new string.
- Parameters:
-
menuTarget Menu to affect menuItem Menu item in the menu to change the text at newMenuItemText New menu text
- Example:
// Define the menuTarget %menuTarget = "New Menu"; or %menuTarget = "4"; // Define the menuItem %menuItem = "New Menu Item"; or %menuItem = "2"; // Define the new text for the menu item %newMenuItemText = "Very New Menu Item"; // Inform the GuiMenuBar control to change the defined menu item with the new text %thisGuiMenuBar.setMenuItemText(%menuTarget,%menuItem,%newMenuItemText);
- See also:
- GuiTickCtrl
void GuiMenuBar::setMenuItemVisible | ( | string | menuTarget, | |
string | menuItemTarget, | |||
bool | isVisible | |||
) |
Brief Description.
Detailed description
- Parameters:
-
menuTarget Menu to affect the menu item in menuItem Menu item to affect isVisible Visible state to set the menu item to.
- Example:
// Define the menuTarget %menuTarget = "New Menu"; or %menuTarget = "3"; // Define the menuItem %menuItem = "New Menu Item"; or %menuItem = "2"; // Define the visibility state %isVisible = "true"; // Inform the GuiMenuBarControl of the visibility state of the defined menu item %thisGuiMenuBar.setMenuItemVisible(%menuTarget,%menuItem,%isVisible);
- See also:
- GuiTickCtrl
void GuiMenuBar::setMenuMargins | ( | int | horizontalMargin, | |
int | verticalMargin, | |||
int | bitmapToTextSpacing | |||
) |
Sets the menu rendering margins: horizontal, vertical, bitmap spacing.
Detailed description
- Parameters:
-
horizontalMargin Number of pixels on the left and right side of a menu's text. verticalMargin Number of pixels on the top and bottom of a menu's text. bitmapToTextSpacing Number of pixels between a menu's bitmap and text.
- Example:
// Define the horizontalMargin %horizontalMargin = "5"; // Define the verticalMargin %verticalMargin = "5"; // Define the bitmapToTextSpacing %bitmapToTextSpacing = "12"; // Inform the GuiMenuBar control to set its margins based on the defined values. %thisGuiMenuBar.setMenuMargins(%horizontalMargin,%verticalMargin,%bitmapToTextSpacing);
- See also:
- GuiTickCtrl
void GuiMenuBar::setMenuText | ( | string | menuTarget, | |
string | newMenuText | |||
) |
Sets the text of the specified menu to the new string.
- Parameters:
-
menuTarget Menu to affect newMenuText New menu text
- Example:
// Define the menu to affect%menu = "New Menu"; or %menu = "3"; // Define the text to change the menu to %newMenuText = "Still a New Menu"; // Inform the GuiMenuBar control to change the defined menu to the defined text %thisGuiMenuBar.setMenuText(%menu,%newMenuText);
- See also:
- GuiTickCtrl
void GuiMenuBar::setMenuVisible | ( | string | menuTarget, | |
bool | visible | |||
) |
Sets the whether or not to display the specified menu.
- Parameters:
-
menuTarget Menu item to affect visible Whether the menu item will be visible or not
- Example:
- See also:
- GuiTickCtrl
void GuiMenuBar::setSubmenuItemChecked | ( | string | menuTarget, | |
string | menuItemTarget, | |||
string | submenuItemText, | |||
bool | checked | |||
) |
Sets the menu item bitmap to a check mark, which by default is the first element in the bitmap array (although this may be changed with setCheckmarkBitmapIndex()). Any other menu items in the menu with the same check group become unchecked if they are checked.
- Parameters:
-
menuTarget Menu to affect a submenu in menuItem Menu item to affect submenuItemText Text to show for submenu checked Whether or not this submenu item will be checked.
- Example:
// Define the menuTarget %menuTarget = "New Menu"; or %menuTarget = "3"; // Define the menuItem %menuItem = "New Menu Item"; or %menuItem = "5"; // Define the text for the new submenu %submenuItemText = "Submenu Item"; // Define if this submenu item should be checked or not %checked = "true"; // Inform the GuiMenuBar control to set the checked state of the defined submenu item %thisGuiMenuBar.setSubmenuItemChecked(%menuTarget,%menuItem,%submenuItemText,%checked);
- Returns:
- If not void, return value and description
- See also:
- References
Member Data Documentation
Extra padding to add to the bounds of the control.