![]() |
|
ArrayObject Class Reference
[Scripting]
Data structure for storing indexed sequences of key/value pairs. More...
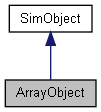
Public Member Functions | |
void | add (string key, string value="") |
Adds a new element to the end of an array (same as push_back()). | |
bool | append (ArrayObject target) |
Appends the target array to the array object. | |
int | count () |
Get the number of elements in the array. | |
int | countKey (string key) |
Get the number of times a particular key is found in the array. | |
int | countValue (string value) |
Get the number of times a particular value is found in the array. | |
bool | crop (ArrayObject target) |
Removes elements with matching keys from array. | |
bool | duplicate (ArrayObject target) |
Alters array into an exact duplicate of the target array. | |
void | echo () |
Echos the array contents to the console. | |
void | empty () |
Emptys all elements from an array. | |
void | erase (int index) |
Removes an element at a specific position from the array. | |
int | getCurrent () |
Gets the current pointer index. | |
int | getIndexFromKey (string key) |
Search the array from the current position for the key. | |
int | getIndexFromValue (string value) |
Search the array from the current position for the element. | |
string | getKey (int index) |
Get the key of the array element at the submitted index. | |
string | getValue (int index) |
Get the value of the array element at the submitted index. | |
void | insert (string key, string value, int index) |
Adds a new element to a specified position in the array. | |
int | moveFirst () |
Moves array pointer to start of array. | |
int | moveLast () |
Moves array pointer to end of array. | |
int | moveNext () |
Moves array pointer to next position. | |
int | movePrev () |
Moves array pointer to prev position. | |
void | pop_back () |
Removes the last element from the array. | |
void | pop_front () |
Removes the first element from the array. | |
void | push_back (string key, string value="") |
Adds a new element to the end of an array. | |
void | push_front (string key, string value="") |
Adds a new element to the front of an array. | |
void | setCurrent (int index) |
Sets the current pointer index. | |
void | setKey (string key, int index) |
Set the key at the given index. | |
void | setValue (string value, int index) |
Set the value at the given index. | |
void | sort (bool ascending=false) |
Alpha sorts the array by value. | |
void | sorta () |
Alpha sorts the array by value in ascending order. | |
void | sortd () |
Alpha sorts the array by value in descending order. | |
void | sortf (string functionName) |
Sorts the array by value in ascending order using the given callback function. | |
void | sortfd (string functionName) |
Sorts the array by value in descending order using the given callback function. | |
void | sortfk (string functionName) |
Sorts the array by key in ascending order using the given callback function. | |
void | sortfkd (string functionName) |
Sorts the array by key in descending order using the given callback function. | |
void | sortk (bool ascending=false) |
Alpha sorts the array by key. | |
void | sortka () |
Alpha sorts the array by key in ascending order. | |
void | sortkd () |
Alpha sorts the array by key in descending order. | |
void | sortn (bool ascending=false) |
Numerically sorts the array by value. | |
void | sortna () |
Numerically sorts the array by value in ascending order. | |
void | sortnd () |
Numerically sorts the array by value in descending order. | |
void | sortnk (bool ascending=false) |
Numerically sorts the array by key. | |
void | sortnka () |
Numerical sorts the array by key in ascending order. | |
void | sortnkd () |
Numerical sorts the array by key in descending order. | |
void | uniqueKey () |
Removes any elements that have duplicated keys (leaving the first instance). | |
void | uniqueValue () |
Removes any elements that have duplicated values (leaving the first instance). | |
Public Attributes | |
bool | caseSensitive |
Makes the keys and values case-sensitive. | |
caseString | key |
Helper field which allows you to add new key['keyname'] = value pairs. |
Detailed Description
Data structure for storing indexed sequences of key/value pairs.
This is a powerful array class providing PHP style arrays in TorqueScript.
The following features are supported:
- array pointers: this allows you to move forwards or backwards through the array as if it was a list, including jumping to the start or end.
- sorting: the array can be sorted in either alphabetic or numeric mode, on the key or the value, and in ascending or descending order
- add/remove elements: elements can be pushed/popped from the start or end of the array, or can be inserted/erased from anywhere in the middle
- removal of duplicates: remove duplicate keys or duplicate values
- searching: search the array and return the index of a particular key or value
- counting: count the number of instaces of a particular value or key in the array, as well as the total number of elements
- advanced features: array append, array crop and array duplicate
Array element keys and values can be strings or numbers
Member Function Documentation
void ArrayObject::add | ( | string | key, | |
string | value = "" | |||
) |
Adds a new element to the end of an array (same as push_back()).
- Parameters:
-
key Key for the new element value Value for the new element
bool ArrayObject::append | ( | ArrayObject | target | ) |
Appends the target array to the array object.
- Parameters:
-
target ArrayObject to append to the end of this array
int ArrayObject::count | ( | ) |
Get the number of elements in the array.
int ArrayObject::countKey | ( | string | key | ) |
Get the number of times a particular key is found in the array.
- Parameters:
-
key Key value to count
int ArrayObject::countValue | ( | string | value | ) |
Get the number of times a particular value is found in the array.
- Parameters:
-
value Array element value to count
bool ArrayObject::crop | ( | ArrayObject | target | ) |
Removes elements with matching keys from array.
- Parameters:
-
target ArrayObject containing keys to remove from this array
bool ArrayObject::duplicate | ( | ArrayObject | target | ) |
Alters array into an exact duplicate of the target array.
- Parameters:
-
target ArrayObject to duplicate
void ArrayObject::echo | ( | ) |
Echos the array contents to the console.
void ArrayObject::empty | ( | ) |
Emptys all elements from an array.
void ArrayObject::erase | ( | int | index | ) |
Removes an element at a specific position from the array.
- Parameters:
-
index 0-based index of the element to remove
int ArrayObject::getCurrent | ( | ) |
Gets the current pointer index.
int ArrayObject::getIndexFromKey | ( | string | key | ) |
Search the array from the current position for the key.
- Parameters:
-
value Array key to search for
- Returns:
- Index of the first element found, or -1 if none
int ArrayObject::getIndexFromValue | ( | string | value | ) |
Search the array from the current position for the element.
- Parameters:
-
value Array value to search for
- Returns:
- Index of the first element found, or -1 if none
string ArrayObject::getKey | ( | int | index | ) |
Get the key of the array element at the submitted index.
- Parameters:
-
index 0-based index of the array element to get
- Returns:
- The key associated with the array element at the specified index, or "" if the index is out of range
string ArrayObject::getValue | ( | int | index | ) |
Get the value of the array element at the submitted index.
- Parameters:
-
index 0-based index of the array element to get
- Returns:
- The value of the array element at the specified index, or "" if the index is out of range
void ArrayObject::insert | ( | string | key, | |
string | value, | |||
int | index | |||
) |
Adds a new element to a specified position in the array.
- index = 0 will insert an element at the start of the array (same as push_front())
- index = array.count() will insert an element at the end of the array (same as push_back())
- Parameters:
-
key Key for the new element value Value for the new element index 0-based index at which to insert the new element
int ArrayObject::moveFirst | ( | ) |
Moves array pointer to start of array.
- Returns:
- Returns the new array pointer
int ArrayObject::moveLast | ( | ) |
Moves array pointer to end of array.
- Returns:
- Returns the new array pointer
int ArrayObject::moveNext | ( | ) |
Moves array pointer to next position.
- Returns:
- Returns the new array pointer, or -1 if already at the end
int ArrayObject::movePrev | ( | ) |
Moves array pointer to prev position.
- Returns:
- Returns the new array pointer, or -1 if already at the start
void ArrayObject::pop_back | ( | ) |
Removes the last element from the array.
void ArrayObject::pop_front | ( | ) |
Removes the first element from the array.
void ArrayObject::push_back | ( | string | key, | |
string | value = "" | |||
) |
Adds a new element to the end of an array.
- Parameters:
-
key Key for the new element value Value for the new element
void ArrayObject::push_front | ( | string | key, | |
string | value = "" | |||
) |
Adds a new element to the front of an array.
void ArrayObject::setCurrent | ( | int | index | ) |
Sets the current pointer index.
- Parameters:
-
index New 0-based pointer index
void ArrayObject::setKey | ( | string | key, | |
int | index | |||
) |
Set the key at the given index.
- Parameters:
-
key New key value index 0-based index of the array element to update
void ArrayObject::setValue | ( | string | value, | |
int | index | |||
) |
Set the value at the given index.
- Parameters:
-
value New array element value index 0-based index of the array element to update
void ArrayObject::sort | ( | bool | ascending = false |
) |
Alpha sorts the array by value.
- Parameters:
-
ascending [optional] True for ascending sort, false for descending sort
void ArrayObject::sorta | ( | ) |
Alpha sorts the array by value in ascending order.
void ArrayObject::sortd | ( | ) |
Alpha sorts the array by value in descending order.
void ArrayObject::sortf | ( | string | functionName | ) |
Sorts the array by value in ascending order using the given callback function.
- Parameters:
-
functionName Name of a function that takes two arguments A and B and returns -1 if A is less, 1 if B is less, and 0 if both are equal.
- Example:
function mySortCallback(%a, %b) { return strcmp( %a.name, %b.name ); } %array.sortf( "mySortCallback" );
void ArrayObject::sortfd | ( | string | functionName | ) |
Sorts the array by value in descending order using the given callback function.
- Parameters:
-
functionName Name of a function that takes two arguments A and B and returns -1 if A is less, 1 if B is less, and 0 if both are equal.
- See also:
- sortf
void ArrayObject::sortfk | ( | string | functionName | ) |
Sorts the array by key in ascending order using the given callback function.
- Parameters:
-
functionName Name of a function that takes two arguments A and B and returns -1 if A is less, 1 if B is less, and 0 if both are equal.
- See also:
- sortf
void ArrayObject::sortfkd | ( | string | functionName | ) |
Sorts the array by key in descending order using the given callback function.
- Parameters:
-
functionName Name of a function that takes two arguments A and B and returns -1 if A is less, 1 if B is less, and 0 if both are equal.
- See also:
- sortf
void ArrayObject::sortk | ( | bool | ascending = false |
) |
Alpha sorts the array by key.
- Parameters:
-
ascending [optional] True for ascending sort, false for descending sort
void ArrayObject::sortka | ( | ) |
Alpha sorts the array by key in ascending order.
void ArrayObject::sortkd | ( | ) |
Alpha sorts the array by key in descending order.
void ArrayObject::sortn | ( | bool | ascending = false |
) |
Numerically sorts the array by value.
- Parameters:
-
ascending [optional] True for ascending sort, false for descending sort
void ArrayObject::sortna | ( | ) |
Numerically sorts the array by value in ascending order.
void ArrayObject::sortnd | ( | ) |
Numerically sorts the array by value in descending order.
void ArrayObject::sortnk | ( | bool | ascending = false |
) |
Numerically sorts the array by key.
- Parameters:
-
ascending [optional] True for ascending sort, false for descending sort
void ArrayObject::sortnka | ( | ) |
Numerical sorts the array by key in ascending order.
void ArrayObject::sortnkd | ( | ) |
Numerical sorts the array by key in descending order.
void ArrayObject::uniqueKey | ( | ) |
Removes any elements that have duplicated keys (leaving the first instance).
void ArrayObject::uniqueValue | ( | ) |
Removes any elements that have duplicated values (leaving the first instance).
Member Data Documentation
Makes the keys and values case-sensitive.
By default, comparison of key and value strings will be case-insensitive.
caseString ArrayObject::key |
Helper field which allows you to add new key['keyname'] = value pairs.