![]() |
|
GuiTextListCtrl Class Reference
[General Controls]
GUI control that displays a list of text. Text items in the list can be individually selected. More...
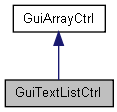
Public Member Functions | |
int | addRow (int id=0, string text="", int index=-1) |
Adds a new row at end of the list with the defined id and text. If index is used, then the new row is inserted at the row location of 'index'. | |
void | clear () |
Clear the list. | |
void | clearSelection () |
Set the selection to nothing. | |
int | findTextIndex (string needle) |
Find needle in the list, and return the row number it was found in. | |
int | getRowId (int index) |
Get the row ID for an index. | |
int | getRowNumById (int id) |
Get the row number for a specified id. | |
string | getRowText (int index) |
Get the text of the row with the specified index. | |
string | getRowTextById (int id) |
Get the text of a row with the specified id. | |
int | getSelectedId () |
Get the ID of the currently selected item. | |
int | getSelectedRow () |
Returns the selected row index (not the row ID). | |
bool | isRowActive (int rowNum) |
Check if the specified row is currently active or not. | |
void | removeRow (int index) |
Remove a row from the table, based on its index. | |
void | removeRowById (int id) |
Remove row with the specified id. | |
int | rowCount () |
Get the number of rows. | |
void | scrollVisible (int rowNum) |
Scroll so the specified row is visible. | |
void | setRowActive (int rowNum, bool active) |
Mark a specified row as active/not. | |
void | setRowById (int id, string text) |
Sets the text at the defined id. | |
void | setSelectedById (int id) |
Finds the specified entry by id, then marks its row as selected. | |
void | setSelectedRow (int rowNum) |
void | sort (int columnId, bool increasing=true) |
Performs a standard (alphabetical) sort on the values in the specified column. | |
void | sortNumerical (int columnID, bool increasing=true) |
Perform a numerical sort on the values in the specified column. | |
Callbacks | |
void | onDeleteKey (string id) |
Called when the delete key has been pressed. | |
void | onSelect (string cellid, string text) |
Called whenever an item in the list is selected. | |
Public Attributes | |
bool | clipColumnText |
If true, text exceeding a column's given width will get clipped. | |
intList | columns |
A vector of column offsets. The number of values determines the number of columns in the table. | |
bool | fitParentWidth |
If true, the width of this control will match the width of its parent. |
Detailed Description
GUI control that displays a list of text. Text items in the list can be individually selected.
- Example:
new GuiTextListCtrl(EndGameGuiList) { columns = "0 256"; fitParentWidth = "1"; clipColumnText = "0"; //Properties not specific to this control have been omitted from this example. };
- See also:
- Reference
Member Function Documentation
int GuiTextListCtrl::addRow | ( | int | id = 0 , |
|
string | text = "" , |
|||
int | index = -1 | |||
) |
Adds a new row at end of the list with the defined id and text. If index is used, then the new row is inserted at the row location of 'index'.
- Parameters:
-
id Id of the new row. text Text to display at the new row. index Index to insert the new row at. If not used, new row will be placed at the end of the list.
- Example:
// Define the id %id = "4"; // Define the text to display %text = "Display Text" // Define the index (optional) %index = "2" // Inform the GuiTextListCtrl control to add the new row with the defined information. %rowIndex = %thisGuiTextListCtrl.addRow(%id,%text,%index);
- Returns:
- Returns the row index of the new row. If 'index' was defined, then this just returns the number of rows in the list.
- See also:
- References
void GuiTextListCtrl::clear | ( | ) |
Clear the list.
- Example:
// Inform the GuiTextListCtrl control to clear its contents %thisGuiTextListCtrl.clear();
- See also:
- GuiControl
void GuiTextListCtrl::clearSelection | ( | ) |
Set the selection to nothing.
- Example:
// Deselect anything that is currently selected %thisGuiTextListCtrl.clearSelection();
- See also:
- GuiControl
int GuiTextListCtrl::findTextIndex | ( | string | needle | ) |
Find needle in the list, and return the row number it was found in.
- Parameters:
-
needle Text to find in the list.
- Example:
// Define the text to find in the list %needle = "Text To Find"; // Request the row number that contains the defined text to find %rowNumber = %thisGuiTextListCtrl.findTextIndex(%needle);
- Returns:
- Row number that the defined text was found in,
- See also:
- GuiControl
int GuiTextListCtrl::getRowId | ( | int | index | ) |
Get the row ID for an index.
- Parameters:
-
index Index to get the RowID at
- Example:
// Define the index %index = "3"; // Request the row ID at the defined index %rowId = %thisGuiTextListCtrl.getRowId(%index);
- Returns:
- RowId at the defined index.
- See also:
- GuiControl
int GuiTextListCtrl::getRowNumById | ( | int | id | ) |
Get the row number for a specified id.
- Parameters:
-
id Id to get the row number at
- Example:
// Define the id %id = "4"; // Request the row number from the GuiTextListCtrl control at the defined id. %rowNumber = %thisGuiTextListCtrl.getRowNumById(%id);
- See also:
- GuiControl
string GuiTextListCtrl::getRowText | ( | int | index | ) |
Get the text of the row with the specified index.
- Parameters:
-
index Row index to acquire the text at.
- Example:
// Define the row index %index = "5"; // Request the text from the row at the defined index %rowText = %thisGuiTextListCtrl.getRowText(%index);
- Returns:
- Text at the defined row index.
- See also:
- GuiControl
string GuiTextListCtrl::getRowTextById | ( | int | id | ) |
Get the text of a row with the specified id.
- Example:
// Define the id %id = "4"; // Inform the GuiTextListCtrl control to return the text at the defined row id %rowText = %thisGuiTextListCtrl.getRowTextById(%id);
- Returns:
- Row text at the requested row id.
- See also:
- GuiControl
int GuiTextListCtrl::getSelectedId | ( | ) |
Get the ID of the currently selected item.
- Example:
// Acquire the ID of the selected item in the list. %id = %thisGuiTextListCtrl.getSelectedId();
- Returns:
- The id of the selected item in the list.
- See also:
- GuiControl
int GuiTextListCtrl::getSelectedRow | ( | ) |
Returns the selected row index (not the row ID).
- Example:
// Acquire the selected row index %rowIndex = %thisGuiTextListCtrl.getSelectedRow();
- Returns:
- Index of the selected row
- See also:
- GuiControl
bool GuiTextListCtrl::isRowActive | ( | int | rowNum | ) |
Check if the specified row is currently active or not.
- Parameters:
-
rowNum Row number to check the active state.
- Example:
// Define the row number %rowNum = "5"; // Request the active state of the defined row number from the GuiTextListCtrl control. %rowActiveState = %thisGuiTextListCtrl.isRowActive(%rowNum);
- Returns:
- Active state of the defined row number.
- See also:
- GuiControl
void GuiTextListCtrl::onDeleteKey | ( | string | id | ) |
Called when the delete key has been pressed.
- Parameters:
-
id Id of the selected item in the list
- Example:
// The delete key was pressed while the GuiTextListCtrl was in focus, causing the callback to occur. GuiTextListCtrl::onDeleteKey(%this,%id) { // Code to run when the delete key is pressed }
- See also:
- GuiControl
void GuiTextListCtrl::onSelect | ( | string | cellid, | |
string | text | |||
) |
Called whenever an item in the list is selected.
- Parameters:
-
cellid The ID of the cell that was selected text The text in the selected cel
- Example:
// A cel in the control was selected, causing the callback to occur GuiTextListCtrl::onSelect(%this,%callid,%text) { // Code to run when a cel item is selected }
- See also:
- GuiControl
void GuiTextListCtrl::removeRow | ( | int | index | ) |
Remove a row from the table, based on its index.
- Parameters:
-
index Row index to remove from the list.
- Example:
// Define the row index %index = "4"; // Inform the GuiTextListCtrl control to remove the row at the defined row index %thisGuiTextListCtrl.removeRow(%index);
- See also:
- GuiControl
void GuiTextListCtrl::removeRowById | ( | int | id | ) |
Remove row with the specified id.
- Parameters:
-
id Id to remove the row entry at
- Example:
// Define the id %id = "4"; // Inform the GuiTextListCtrl control to remove the row at the defined id %thisGuiTextListCtrl.removeRowById(%id);
- See also:
- GuiControl
int GuiTextListCtrl::rowCount | ( | ) |
Get the number of rows.
- Example:
// Get the number of rows in the list %rowCount = %thisGuiTextListCtrl.rowCount();
- Returns:
- Number of rows in the list.
- See also:
- GuiControl
void GuiTextListCtrl::scrollVisible | ( | int | rowNum | ) |
Scroll so the specified row is visible.
- Parameters:
-
rowNum Row number to make visible
- Example:
// Define the row number to make visible %rowNum = "4"; // Inform the GuiTextListCtrl control to scroll the list so the defined rowNum is visible. %thisGuiTextListCtrl.scrollVisible(%rowNum);
- See also:
- GuiControl
void GuiTextListCtrl::setRowActive | ( | int | rowNum, | |
bool | active | |||
) |
Mark a specified row as active/not.
- Parameters:
-
rowNum Row number to change the active state. active Boolean active state to set the row number.
- Example:
// Define the row number %rowNum = "4"; // Define the boolean active state %active = "true"; // Informthe GuiTextListCtrl control to set the defined active state at the defined row number. %thisGuiTextListCtrl.setRowActive(%rowNum,%active);
- See also:
- GuiControl
void GuiTextListCtrl::setRowById | ( | int | id, | |
string | text | |||
) |
Sets the text at the defined id.
- Parameters:
-
id Id to change. text Text to use at the Id.
- Example:
// Define the id %id = "4"; // Define the text %text = "Text To Display"; // Inform the GuiTextListCtrl control to display the defined text at the defined id %thisGuiTextListCtrl.setRowById(%id,%text);
- See also:
- GuiControl
void GuiTextListCtrl::setSelectedById | ( | int | id | ) |
Finds the specified entry by id, then marks its row as selected.
- Parameters:
-
id Entry within the text list to make selected.
- Example:
// Define the id %id = "5"; // Inform the GuiTextListCtrl control to set the defined id entry as selected %thisGuiTextListCtrl.setSelectedById(%id);
- See also:
- GuiControl
void GuiTextListCtrl::setSelectedRow | ( | int | rowNum | ) |
the specified row.
- Parameters:
-
rowNum Row number to set selected.
- Example:
// Define the row number to set selected %rowNum = "4"; %guiTextListCtrl.setSelectedRow(%rowNum);
- See also:
- GuiControl
void GuiTextListCtrl::sort | ( | int | columnId, | |
bool | increasing = true | |||
) |
Performs a standard (alphabetical) sort on the values in the specified column.
- Parameters:
-
columnId Column ID to perform the sort on. increasing If false, sort will be performed in reverse.
- Example:
// Define the columnId %id = "1"; // Define if we are increasing or not %increasing = "false"; // Inform the GuiTextListCtrl to perform the sort operation %thisGuiTextListCtrl.sort(%id,%increasing);
- See also:
- GuiControl
void GuiTextListCtrl::sortNumerical | ( | int | columnID, | |
bool | increasing = true | |||
) |
Perform a numerical sort on the values in the specified column.
Detailed description
- Parameters:
-
columnId Column ID to perform the sort on. increasing If false, sort will be performed in reverse.
- Example:
// Define the columnId %id = "1"; // Define if we are increasing or not %increasing = "false"; // Inform the GuiTextListCtrl to perform the sort operation %thisGuiTextListCtrl.sortNumerical(%id,%increasing);
- See also:
- GuiControl
Member Data Documentation
If true, text exceeding a column's given width will get clipped.
intList GuiTextListCtrl::columns |
A vector of column offsets. The number of values determines the number of columns in the table.
If true, the width of this control will match the width of its parent.