![]() |
|
FileObject Class Reference
[File I/O]
This class is responsible opening, reading, creating, and saving file contents. More...
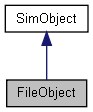
Public Member Functions | |
void | close () |
Close the file. | |
bool | isEOF () |
Determines if the parser for this FileObject has reached the end of the file. | |
bool | openForAppend (string filename) |
Open a specified file for writing, adding data to the end of the file. | |
bool | openForRead (string filename) |
Open a specified file for reading. | |
bool | openForWrite (string filename) |
Open a specified file for writing. | |
string | peekLine () |
Read a line from the file without moving the stream position. | |
string | readLine () |
Read a line from file. | |
void | writeLine (string text) |
Write a line to the file, if it was opened for writing. | |
void | writeObject (SimObject *object) |
Write an object to a text file. | |
void | writeObject (SimObject *object, string prepend) |
Write an object to a text file, with some data added first. |
Detailed Description
This class is responsible opening, reading, creating, and saving file contents.
FileObject acts as the interface with OS level files. You create a new FileObject and pass into it a file's path and name. The FileObject class supports three distinct operations for working with files:
Operation | FileObject Method | Description |
---|---|---|
Read | openForRead() | Open the file for reading |
Write | openForWrite() | Open the file for writing to and replace its contents (if any) |
Append | openForAppend() | Open the file and start writing at its end |
Before you may work with a file you need to use one of the three above methods on the FileObject.
- Example:
// Create a file object for writing %fileWrite = new FileObject(); // Open a file to write to, if it does not exist it will be created %result = %fileWrite.OpenForWrite("./test.txt"); if ( %result ) { // Write a line to the text files %fileWrite.writeLine("READ. READ CODE. CODE"); } // Close the file when finished %fileWrite.close(); // Cleanup the file object %fileWrite.delete(); // Create a file object for reading %fileRead = new FileObject(); // Open a text file, if it exists %result = %fileRead.OpenForRead("./test.txt"); if ( %result ) { // Read in the first line %line = %fileRead.readline(); // Print the line we just read echo(%line); } // Close the file when finished %fileRead.close(); // Cleanup the file object %fileRead.delete();
Member Function Documentation
void FileObject::close | ( | ) |
Close the file.
It is EXTREMELY important that you call this function when you are finished reading or writing to a file. Failing to do so is not only a bad programming practice, but could result in bad data or corrupt files. Remember: Open, Read/Write, Close, Delete...in that order!
- Example:
// Create a file object for reading %fileRead = new FileObject(); // Open a text file, if it exists %fileRead.OpenForRead("./test.txt"); // Peek the first line %line = %fileRead.peekLine(); // Print the line we just peeked echo(%line); // If we peek again... %line = %fileRead.peekLine(); // We will get the same output as the first time // since the stream did not move forward echo(%line); // Close the file when finished %fileWrite.close(); // Cleanup the file object %fileWrite.delete();
bool FileObject::isEOF | ( | ) |
Determines if the parser for this FileObject has reached the end of the file.
- Example:
// Create a file object for reading %fileRead = new FileObject(); // Open a text file, if it exists %fileRead.OpenForRead("./test.txt"); // Keep reading until we reach the end of the file while( !%fileRead.isEOF() ) { %line = %fileRead.readline(); echo(%line); } // Made it to the end echo("Finished reading file");
- Returns:
- True if the parser has reached the end of the file, false otherwise
bool FileObject::openForAppend | ( | string | filename | ) |
Open a specified file for writing, adding data to the end of the file.
There is no limit as to what kind of file you can write. Any format and data is allowable, not just text. Unlike openForWrite(), which will erase an existing file if it is opened, openForAppend() preserves data in an existing file and adds to it.
- Parameters:
-
filename Path, name, and extension of file to append to
- Example:
// Create a file object for writing %fileWrite = new FileObject(); // Open a file to write to, if it does not exist it will be created // If it does exist, whatever we write will be added to the end %result = %fileWrite.OpenForAppend("./test.txt");
- Returns:
- True if file was successfully opened, false otherwise
bool FileObject::openForRead | ( | string | filename | ) |
Open a specified file for reading.
There is no limit as to what kind of file you can read. Any format and data contained within is accessible, not just text
- Parameters:
-
filename Path, name, and extension of file to be read
- Example:
// Create a file object for reading %fileRead = new FileObject(); // Open a text file, if it exists %result = %fileRead.OpenForRead("./test.txt");
- Returns:
- True if file was successfully opened, false otherwise
bool FileObject::openForWrite | ( | string | filename | ) |
Open a specified file for writing.
There is no limit as to what kind of file you can write. Any format and data is allowable, not just text
- Parameters:
-
filename Path, name, and extension of file to write to
- Example:
// Create a file object for writing %fileWrite = new FileObject(); // Open a file to write to, if it does not exist it will be created %result = %fileWrite.OpenForWrite("./test.txt");
- Returns:
- True if file was successfully opened, false otherwise
string FileObject::peekLine | ( | ) |
Read a line from the file without moving the stream position.
Emphasis on *line*, as in you cannot parse individual characters or chunks of data. There is no limitation as to what kind of data you can read. Unlike readLine, the parser does not move forward after reading.
- Parameters:
-
filename Path, name, and extension of file to be read
- Example:
// Create a file object for reading %fileRead = new FileObject(); // Open a text file, if it exists %fileRead.OpenForRead("./test.txt"); // Peek the first line %line = %fileRead.peekLine(); // Print the line we just peeked echo(%line); // If we peek again... %line = %fileRead.peekLine(); // We will get the same output as the first time // since the stream did not move forward echo(%line);
- Returns:
- String containing the line of data that was just peeked
string FileObject::readLine | ( | ) |
Read a line from file.
Emphasis on *line*, as in you cannot parse individual characters or chunks of data. There is no limitation as to what kind of data you can read.
- Example:
// Create a file object for reading %fileRead = new FileObject(); // Open a text file, if it exists %fileRead.OpenForRead("./test.txt"); // Read in the first line %line = %fileRead.readline(); // Print the line we just read echo(%line);
- Returns:
- String containing the line of data that was just read
void FileObject::writeLine | ( | string | text | ) |
Write a line to the file, if it was opened for writing.
There is no limit as to what kind of text you can write. Any format and data is allowable, not just text. Be careful of what you write, as whitespace, current values, and literals will be preserved.
- Parameters:
-
text The data we are writing out to file.
- Example:
// Create a file object for writing %fileWrite = new FileObject(); // Open a file to write to, if it does not exist it will be created %fileWrite.OpenForWrite("./test.txt"); // Write a line to the text files %fileWrite.writeLine("READ. READ CODE. CODE");
- Returns:
- True if file was successfully opened, false otherwise
void FileObject::writeObject | ( | SimObject * | object | ) |
Write an object to a text file.
Unlike a simple writeLine using specified strings, this function writes an entire object to file, preserving its type, name, and properties. This is similar to the save() functionality of the SimObject class, but with a bit more control.
- Parameters:
-
object The SimObject being written to file, properties, name, and all.
- Example:
// Let's assume this SpawnSphere was created and currently // exists in the running level new SpawnSphere(TestSphere) { spawnClass = "Player"; spawnDatablock = "DefaultPlayerData"; autoSpawn = "1"; radius = "5"; sphereWeight = "1"; indoorWeight = "1"; outdoorWeight = "1"; dataBlock = "SpawnSphereMarker"; position = "-42.222 1.4845 4.80334"; rotation = "0 0 -1 108"; scale = "1 1 1"; canSaveDynamicFields = "1"; }; // Create a file object for writing %fileWrite = new FileObject(); // Open a file to write to, if it does not exist it will be created %fileWrite.OpenForWrite("./spawnSphers.txt"); // Write out the TestSphere %fileWrite.writeObject(TestSphere); // Close the text file %fileWrite.close(); // Cleanup %fileWrite.delete();
void FileObject::writeObject | ( | SimObject * | object, | |
string | prepend | |||
) |
Write an object to a text file, with some data added first.
Unlike a simple writeLine using specified strings, this function writes an entire object to file, preserving its type, name, and properties. This is similar to the save() functionality of the SimObject class, but with a bit more control.
- Parameters:
-
object The SimObject being written to file, properties, name, and all. prepend Data or text that is written out before the SimObject.
- Example:
// Let's assume this SpawnSphere was created and currently // exists in the running level new SpawnSphere(TestSphere) { spawnClass = "Player"; spawnDatablock = "DefaultPlayerData"; autoSpawn = "1"; radius = "5"; sphereWeight = "1"; indoorWeight = "1"; outdoorWeight = "1"; dataBlock = "SpawnSphereMarker"; position = "-42.222 1.4845 4.80334"; rotation = "0 0 -1 108"; scale = "1 1 1"; canSaveDynamicFields = "1"; }; // Create a file object for writing %fileWrite = new FileObject(); // Open a file to write to, if it does not exist it will be created %fileWrite.OpenForWrite("./spawnSphers.txt"); // Write out the TestSphere, with a prefix %fileWrite.writeObject(TestSphere, "$mySphere = "); // Close the text file %fileWrite.close(); // Cleanup %fileWrite.delete();