![]() |
|
ShapeBaseData Class Reference
[Game Objects]
Defines properties for a ShapeBase object. More...
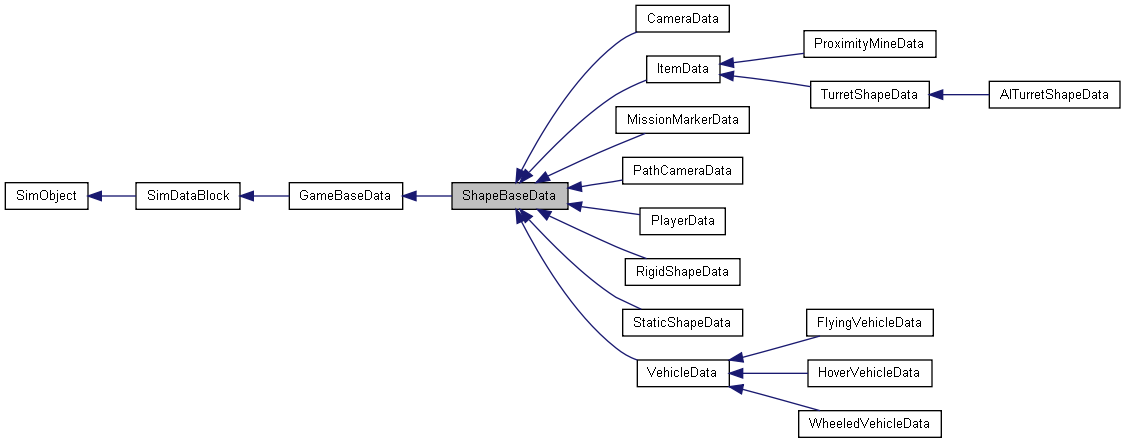
Public Member Functions | |
bool | checkDeployPos (TransformF txfm) |
Check if there is the space at the given transform is free to spawn into. | |
TransformF | getDeployTransform (Point3F pos, Point3F normal) |
Helper method to get a transform from a position and vector (suitable for use with setTransform). | |
Callbacks | |
void | onCollision (ShapeBase obj, SceneObject collObj, VectorF vec, float len) |
Called when we collide with another object. | |
void | onDamage (ShapeBase obj, float delta) |
Called when the object is damaged. | |
void | onDestroyed (ShapeBase obj, string lastState) |
Called when the object damage state changes to Destroyed. | |
void | onDisabled (ShapeBase obj, string lastState) |
Called when the object damage state changes to Disabled. | |
void | onEnabled (ShapeBase obj, string lastState) |
Called when the object damage state changes to Enabled. | |
void | onEndSequence (ShapeBase obj, int slot) |
Called when a thread playing a non-cyclic sequence reaches the end of the sequence. | |
void | onForceUncloak (ShapeBase obj, string reason) |
Called when the object is forced to uncloak. | |
void | onImpact (ShapeBase obj, SceneObject collObj, VectorF vec, float len) |
Called when we collide with another object beyond some impact speed. | |
void | onTrigger (ShapeBase obj, int index, bool state) |
Called when a move trigger input changes state. | |
Public Attributes | |
Camera | |
float | cameraDefaultFov |
The default camera vertical FOV in degrees. | |
float | cameraMaxDist |
The maximum distance from the camera to the object. | |
float | cameraMaxFov |
The maximum camera vertical FOV allowed in degrees. | |
float | cameraMinDist |
The minimum distance from the camera to the object. | |
float | cameraMinFov |
The minimum camera vertical FOV allowed in degrees. | |
bool | firstPersonOnly |
Flag controlling whether the view from this object is first person only. | |
bool | observeThroughObject |
Observe this object through its camera transform and default fov. | |
bool | useEyePoint |
Flag controlling whether the client uses this object's eye point to view from. | |
Misc | |
bool | computeCRC |
If true, verify that the CRC of the client's shape model matches the server's CRC for the shape model when loaded by the client. | |
Reflection | |
string | cubeReflectorDesc |
References a ReflectorDesc datablock that defines performance and quality properties for dynamic reflections. | |
Destruction | |
DebrisData | Debris |
Debris to generate when this shape is blown up. | |
filename | debrisShapeName |
The DTS or DAE model to use for auto-generated breakups. | |
ExplosionData | Explosion |
Explosion to generate when this shape is blown up. | |
bool | renderWhenDestroyed |
Whether to render the shape when it is in the "Destroyed" damage state. | |
ExplosionData | underwaterExplosion |
Explosion to generate when this shape is blown up underwater. | |
Physics | |
float | density |
Shape density. | |
float | drag |
Drag factor. | |
float | mass |
Shape mass. | |
Damage/Energy | |
float | destroyedLevel |
Damage level above which the object is destroyed. | |
float | disabledLevel |
Damage level above which the object is disabled. | |
bool | inheritEnergyFromMount |
Flag controlling whether to manage our own energy level, or to use the energy level of the object we are mounted to. | |
bool | isInvincible |
Invincible flag; when invincible, the object cannot be damaged or repaired. | |
float | maxDamage |
Maximum damage level for this object. | |
float | maxEnergy |
Maximum energy level for this object. | |
float | repairRate |
Rate at which damage is repaired in damage units/tick. | |
Shadows | |
bool | shadowEnable |
Enable shadows for this shape (currently unused, shadows are always enabled). | |
float | shadowMaxVisibleDistance |
Maximum distance at which shadow is visible (currently unused). | |
float | shadowProjectionDistance |
Maximum height above ground to project shadow. If the object is higher than this no shadow will be rendered. | |
int | shadowSize |
Size of the projected shadow texture (must be power of 2). | |
float | shadowSphereAdjust |
Scalar applied to the radius of spot shadows (initial radius is based on the shape bounds but can be adjusted with this field). | |
Render | |
filename | shapeFile |
The DTS or DAE model to use for this object. |
Detailed Description
Defines properties for a ShapeBase object.
- See also:
- ShapeBase
Member Function Documentation
bool ShapeBaseData::checkDeployPos | ( | TransformF | txfm | ) |
Check if there is the space at the given transform is free to spawn into.
The shape's bounding box volume is used to check for collisions at the given world transform. Only interior and static objects are checked for collision.
- Parameters:
-
txfm Deploy transform to check
- Returns:
- True if the space is free, false if there is already something in the way.
- Note:
- This is a server side only check, and is not actually limited to spawning.
TransformF ShapeBaseData::getDeployTransform | ( | Point3F | pos, | |
Point3F | normal | |||
) |
Helper method to get a transform from a position and vector (suitable for use with setTransform).
- Parameters:
-
pos Desired transform position normal Vector of desired direction
- Returns:
- The deploy transform
void ShapeBaseData::onCollision | ( | ShapeBase | obj, | |
SceneObject | collObj, | |||
VectorF | vec, | |||
float | len | |||
) |
Called when we collide with another object.
- Parameters:
-
obj The ShapeBase object collObj The object we collided with vec Collision impact vector len Length of the impact vector
void ShapeBaseData::onDamage | ( | ShapeBase | obj, | |
float | delta | |||
) |
void ShapeBaseData::onDestroyed | ( | ShapeBase | obj, | |
string | lastState | |||
) |
Called when the object damage state changes to Destroyed.
- Parameters:
-
obj The ShapeBase object lastState The previous damage state
void ShapeBaseData::onDisabled | ( | ShapeBase | obj, | |
string | lastState | |||
) |
Called when the object damage state changes to Disabled.
- Parameters:
-
obj The ShapeBase object lastState The previous damage state
void ShapeBaseData::onEnabled | ( | ShapeBase | obj, | |
string | lastState | |||
) |
Called when the object damage state changes to Enabled.
- Parameters:
-
obj The ShapeBase object lastState The previous damage state
void ShapeBaseData::onEndSequence | ( | ShapeBase | obj, | |
int | slot | |||
) |
Called when a thread playing a non-cyclic sequence reaches the end of the sequence.
- Parameters:
-
obj The ShapeBase object slot Thread slot that finished playing
void ShapeBaseData::onForceUncloak | ( | ShapeBase | obj, | |
string | reason | |||
) |
Called when the object is forced to uncloak.
- Parameters:
-
obj The ShapeBase object reason String describing why the object was uncloaked
void ShapeBaseData::onImpact | ( | ShapeBase | obj, | |
SceneObject | collObj, | |||
VectorF | vec, | |||
float | len | |||
) |
Called when we collide with another object beyond some impact speed.
The Player class makes use of this callback when a collision speed is more than PlayerData::minImpactSpeed.
- Parameters:
-
obj The ShapeBase object collObj The object we collided with vec Collision impact vector len Length of the impact vector
void ShapeBaseData::onTrigger | ( | ShapeBase | obj, | |
int | index, | |||
bool | state | |||
) |
Called when a move trigger input changes state.
- Parameters:
-
obj The ShapeBase object index Index of the trigger that changed state New state of the trigger
Member Data Documentation
The default camera vertical FOV in degrees.
The maximum distance from the camera to the object.
Used when computing a custom camera transform for this object.
- See also:
- observeThroughObject
The maximum camera vertical FOV allowed in degrees.
The minimum distance from the camera to the object.
Used when computing a custom camera transform for this object.
- See also:
- observeThroughObject
The minimum camera vertical FOV allowed in degrees.
If true, verify that the CRC of the client's shape model matches the server's CRC for the shape model when loaded by the client.
References a ReflectorDesc datablock that defines performance and quality properties for dynamic reflections.
Debris to generate when this shape is blown up.
filename ShapeBaseData::debrisShapeName |
The DTS or DAE model to use for auto-generated breakups.
- Note:
- may not be functional.
float ShapeBaseData::density |
Shape density.
Used when computing buoyancy when in water.
Damage level above which the object is destroyed.
When the damage level increases above this value, the object damage state is set to "Destroyed".
Damage level above which the object is disabled.
Currently unused.
float ShapeBaseData::drag |
Drag factor.
Reduces velocity of moving objects.
Explosion to generate when this shape is blown up.
Flag controlling whether the view from this object is first person only.
Flag controlling whether to manage our own energy level, or to use the energy level of the object we are mounted to.
Invincible flag; when invincible, the object cannot be damaged or repaired.
float ShapeBaseData::mass |
Shape mass.
Used in simulation of moving objects.
float ShapeBaseData::maxDamage |
Maximum damage level for this object.
float ShapeBaseData::maxEnergy |
Maximum energy level for this object.
Observe this object through its camera transform and default fov.
If true, when this object is the camera it can provide a custom camera transform and FOV (instead of the default eye transform).
Whether to render the shape when it is in the "Destroyed" damage state.
Rate at which damage is repaired in damage units/tick.
This value is subtracted from the damage level until it reaches 0.
Enable shadows for this shape (currently unused, shadows are always enabled).
Maximum distance at which shadow is visible (currently unused).
Maximum height above ground to project shadow. If the object is higher than this no shadow will be rendered.
Size of the projected shadow texture (must be power of 2).
Scalar applied to the radius of spot shadows (initial radius is based on the shape bounds but can be adjusted with this field).
filename ShapeBaseData::shapeFile |
The DTS or DAE model to use for this object.
Explosion to generate when this shape is blown up underwater.
Flag controlling whether the client uses this object's eye point to view from.