![]() |
|
GuiListBoxCtrl Class Reference
[Core Controls]
A list of text items. More...
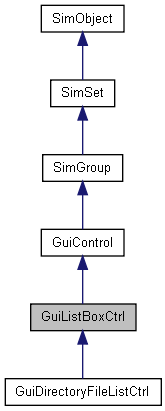
Public Member Functions | |
void | addFilteredItem (string newItem) |
Checks if there is an item with the exact text of what is passed in, and if so the item is removed from the list and adds that item's data to the filtered list. | |
void | clearItemColor (int index) |
Removes any custom coloring from an item at the defined index id in the list. | |
void | clearItems () |
Clears all the items in the listbox. | |
void | clearSelection () |
Sets all currently selected items to unselected. | |
void | deleteItem (int itemIndex) |
Removes the list entry at the requested index id from the control and clears the memory associated with it. | |
void | doMirror () |
Informs the GuiListBoxCtrl object to mirror the contents of the GuiListBoxCtrl stored in the mirrorSet field. | |
int | findItemText (string findText, bool bCaseSensitive=false) |
Returns index of item with matching text or -1 if none found. | |
int | getItemCount () |
Returns the number of items in the list. | |
string | getItemObject (int index) |
Returns the object associated with an item. This only makes sense if you are mirroring a simset. | |
string | getItemText (int index) |
Returns the text of the item at the specified index. | |
int | getLastClickItem () |
Request the item index for the item that was last clicked. | |
int | getSelCount () |
Returns the number of items currently selected. | |
int | getSelectedItem () |
Returns the selected items index or -1 if none selected. If multiple selections exist it returns the first selected item. | |
string | getSelectedItems () |
Returns a space delimited list of the selected items indexes in the list. | |
void | insertItem (string text, int index) |
Inserts an item into the list at the specified index and returns the index assigned or -1 on error. | |
void | removeFilteredItem (string itemName) |
Removes an item of the entered name from the filtered items list. | |
void | setCurSel (int indexId) |
Sets the currently selected item at the specified index. | |
void | setCurSelRange (int indexStart, int indexStop=999999) |
Sets the current selection range from index start to stop. If no stop is specified it sets from start index to the end of the list. | |
void | setItemColor (int index, ColorF color) |
Sets the color of a single list entry at the specified index id. | |
void | setItemText (int index, string newtext) |
Sets the items text at the specified index. | |
void | setItemTooltip (int index, string text) |
Set the tooltip text to display for the given list item. | |
void | setMultipleSelection (bool allowMultSelections) |
Enable or disable multiple selections for this GuiListBoxCtrl object. | |
void | setSelected (int index, bool setSelected=true) |
Sets the item at the index specified to selected or not. | |
Callbacks | |
bool | isObjectMirrored (string indexIdString) |
Checks if a list item at a defined index id is mirrored, and returns the result. | |
void | onClearSelection () |
Called whenever a selected item in the list is cleared. | |
void | onDeleteKey () |
Called whenever the Delete key on the keyboard has been pressed while in this control. | |
void | onDoubleClick () |
Called whenever an item in the list has been double clicked. | |
void | onMouseDragged () |
Called whenever the mouse is dragged across the control. | |
void | onMouseUp (string itemHit, string mouseClickCount) |
Called whenever the mouse has previously been clicked down (onMouseDown) and has now been raised on the control. If an item in the list was hit during the click cycle, then the index id of the clicked object along with how many clicks occured are passed into the callback. | |
void | onSelect (string index, string itemText) |
Called whenever an item in the list is selected. | |
void | onUnselect (string index, string itemText) |
Called whenever a selected item in the list has been unselected. | |
Public Attributes | |
bool | allowMultipleSelections |
If true, will allow the selection of multiple items in the listbox. | |
bool | colorBullet |
If true, colored items will render a colored rectangular bullet next to the item text. | |
bool | fitParentWidth |
If true, the width of the listbox will match the width of its parent control. | |
string | makeNameCallback |
A script snippet to control what is displayed in the list for a SimObject. Within this snippet, $ThisControl is bound to the guiListBoxCtrl and $ThisObject to the contained object in question. | |
string | mirrorSet |
If populated with the name of another GuiListBoxCtrl, then this list box will mirror the contents of the mirrorSet listbox. |
Detailed Description
A list of text items.
A list of text items where each individual entry can have its own text value, text color and associated SimObject.
- Example:
new GuiListBoxCtrl(GuiMusicPlayerMusicList) { allowMultipleSelections = "true"; fitParentWidth = "true"; mirrorSet = "AnotherGuiListBoxCtrl"; makeNameCallback = ""; colorBullet = "1"; //Properties not specific to this control have been omitted from this example. };
- See also:
- GuiControl
Member Function Documentation
void GuiListBoxCtrl::addFilteredItem | ( | string | newItem | ) |
Checks if there is an item with the exact text of what is passed in, and if so the item is removed from the list and adds that item's data to the filtered list.
- Parameters:
-
itemName Name of the item that we wish to add to the filtered item list of the GuiListBoxCtrl.
- Example:
// Define the itemName that we wish to add to the filtered item list. %itemName = "This Item Name"; // Add the item name to the filtered item list. %thisGuiListBoxCtrl.addFilteredItem(%filteredItemName);
- See also:
- GuiControl
void GuiListBoxCtrl::clearItemColor | ( | int | index | ) |
Removes any custom coloring from an item at the defined index id in the list.
- Parameters:
-
index Index id for the item to clear any custom color from.
- Example:
// Define the index id %index = "4"; // Request the GuiListBoxCtrl object to remove any custom coloring from the defined index entry %thisGuiListBoxCtrl.clearItemColor(%index);
- See also:
- GuiControl
void GuiListBoxCtrl::clearItems | ( | ) |
Clears all the items in the listbox.
- Example:
// Inform the GuiListBoxCtrl object to clear all items from its list. %thisGuiListBoxCtrl.clearItems();
- See also:
- GuiControl
void GuiListBoxCtrl::clearSelection | ( | ) |
Sets all currently selected items to unselected.
Detailed description
- Example:
// Inform the GuiListBoxCtrl object to set all of its items to unselected./n%thisGuiListBoxCtrl.clearSelection();
- See also:
- GuiControl
void GuiListBoxCtrl::deleteItem | ( | int | itemIndex | ) |
Removes the list entry at the requested index id from the control and clears the memory associated with it.
- Parameters:
-
itemIndex Index id location to remove the item from.
- Example:
// Define the index id we want to remove from the list %itemIndex = "8"; // Inform the GuiListBoxCtrl object to remove the item at the defined index id. %thisGuiListBoxCtrl.deleteItem(%itemIndex);
- See also:
- References
void GuiListBoxCtrl::doMirror | ( | ) |
Informs the GuiListBoxCtrl object to mirror the contents of the GuiListBoxCtrl stored in the mirrorSet field.
- Example:
\ Inform the object to mirror the object located at %thisGuiListBox.mirrorSet %thisGuiListBox.doMirror();
- See also:
- Core Controls
int GuiListBoxCtrl::findItemText | ( | string | findText, | |
bool | bCaseSensitive = false | |||
) |
Returns index of item with matching text or -1 if none found.
- Parameters:
-
findText Text in the list to find. isCaseSensitive If true, the search will be case sensitive.
- Example:
// Define the text we wish to find in the list. %findText = "Hickory Smoked Gideon"/n/n// Define if this is a case sensitive search or not. %isCaseSensitive = "false"; // Ask the GuiListBoxCtrl object what item id in the list matches the requested text. %matchingId = %thisGuiListBoxCtrl.findItemText(%findText,%isCaseSensitive);
- Returns:
- Index id of item with matching text or -1 if none found.
- See also:
- GuiControl
int GuiListBoxCtrl::getItemCount | ( | ) |
Returns the number of items in the list.
- Example:
// Request the number of items in the list of the GuiListBoxCtrl object. %listItemCount = %thisGuiListBoxCtrl.getItemCount();
- Returns:
- The number of items in the list.
- See also:
- GuiControl
string GuiListBoxCtrl::getItemObject | ( | int | index | ) |
Returns the object associated with an item. This only makes sense if you are mirroring a simset.
- Parameters:
-
index Index id to request the associated item from.
- Example:
// Define the index id %index = "12"; // Request the item from the GuiListBoxCtrl object %object = %thisGuiListBoxCtrl.getItemObject(%index);
- Returns:
- The object associated with the item in the list.
- See also:
- References
string GuiListBoxCtrl::getItemText | ( | int | index | ) |
Returns the text of the item at the specified index.
- Parameters:
-
index Index id to return the item text from.
- Example:
// Define the index id entry to request the text from %index = "12"; // Request the item id text from the GuiListBoxCtrl object. %text = %thisGuiListBoxCtrl.getItemText(%index);
- Returns:
- The text of the requested index id.
- See also:
- GuiControl
int GuiListBoxCtrl::getLastClickItem | ( | ) |
Request the item index for the item that was last clicked.
- Example:
// Request the item index for the last clicked item in the list %lastClickedIndex = %thisGuiListBoxCtrl.getLastClickItem();
- Returns:
- Index id for the last clicked item in the list.
- See also:
- GuiControl
int GuiListBoxCtrl::getSelCount | ( | ) |
Returns the number of items currently selected.
- Example:
// Request the number of currently selected items %selectedItemCount = %thisGuiListBoxCtrl.getSelCount();
- Returns:
- Number of currently selected items.
- See also:
- GuiControl
int GuiListBoxCtrl::getSelectedItem | ( | ) |
Returns the selected items index or -1 if none selected. If multiple selections exist it returns the first selected item.
- Example:
// Request the index id of the currently selected item %selectedItemId = %thisGuiListBoxCtrl.getSelectedItem();
- Returns:
- The selected items index or -1 if none selected.
- See also:
- GuiControl
string GuiListBoxCtrl::getSelectedItems | ( | ) |
Returns a space delimited list of the selected items indexes in the list.
- Example:
// Request a space delimited list of the items in the GuiListBoxCtrl object. %selectionList = %thisGuiListBoxCtrl.getSelectedItems();
- Returns:
- Space delimited list of the selected items indexes in the list
- See also:
- GuiControl
void GuiListBoxCtrl::insertItem | ( | string | text, | |
int | index | |||
) |
Inserts an item into the list at the specified index and returns the index assigned or -1 on error.
- Parameters:
-
text Text item to add. index Index id to insert the list item text at.
- Example:
// Define the text to insert %text = "Secret Agent Gideon"; // Define the index entry to insert the text at %index = "14"; // In form the GuiListBoxCtrl object to insert the text at the defined index. %assignedId = %thisGuiListBoxCtrl.insertItem(%text,%index);
- Returns:
- If successful will return the index id assigned. If unsuccessful, will return -1.
- See also:
- GuiControl
bool GuiListBoxCtrl::isObjectMirrored | ( | string | indexIdString | ) |
Checks if a list item at a defined index id is mirrored, and returns the result.
- Parameters:
-
indexIdString Index id of the list to check.
- Example:
// Engine has requested of the script level to determine if a list entry is mirrored or not. GuiListBoxCtrl::isObjectMirrored(%this, %indexIdString) { // Perform code required to check and see if the list item at the index id is mirrored or not. return %isMirrored; }
- Returns:
- A boolean value on if the list item is mirrored or not.
- See also:
- GuiControl
void GuiListBoxCtrl::onClearSelection | ( | ) |
Called whenever a selected item in the list is cleared.
- Example:
// A selected item is cleared, causing the callback to occur. GuiListBoxCtrl::onClearSelection(%this) { // Code to run whenever a selected item is cleared }
- See also:
- GuiControl
void GuiListBoxCtrl::onDeleteKey | ( | ) |
Called whenever the Delete key on the keyboard has been pressed while in this control.
- Example:
// The delete key on the keyboard has been pressed while this control is in focus, causing the callback to occur. GuiListBoxCtrl::onDeleteKey(%this) { // Code to call whenever the delete key is pressed }
- See also:
- GuiControl
void GuiListBoxCtrl::onDoubleClick | ( | ) |
Called whenever an item in the list has been double clicked.
- Example:
// An item in the list is double clicked, causing the callback to occur. GuiListBoxCtrl::onDoubleClick(%this) { // Code to run whenever an item in the control has been double clicked }
- See also:
- GuiControl
void GuiListBoxCtrl::onMouseDragged | ( | ) |
Called whenever the mouse is dragged across the control.
- Example:
// Mouse is dragged across the control, causing the callback to occur. GuiListBoxCtrl::onMouseDragged(%this) { // Code to run whenever the mouse is dragged across the control }
- See also:
- GuiControl
void GuiListBoxCtrl::onMouseUp | ( | string | itemHit, | |
string | mouseClickCount | |||
) |
Called whenever the mouse has previously been clicked down (onMouseDown) and has now been raised on the control. If an item in the list was hit during the click cycle, then the index id of the clicked object along with how many clicks occured are passed into the callback.
Detailed description
- Parameters:
-
itemHit Index id for the list item that was hit mouseClickCount How many mouse clicks occured on this list item
- Example:
// Mouse was previously clicked down, and now has been released, causing the callback to occur. GuiListBoxCtrl::onMouseUp(%this, %itemHit, %mouseClickCount) { // Code to call whenever the mouse has been clicked and released on the control }
- See also:
- GuiControl
void GuiListBoxCtrl::onSelect | ( | string | index, | |
string | itemText | |||
) |
Called whenever an item in the list is selected.
- Parameters:
-
index Index id for the item in the list that was selected. itemText Text for the list item at the index that was selected.
- Example:
// An item in the list is selected, causing the callback to occur GuiListBoxCtrl::onSelect(%this, %index, %itemText) { // Code to run whenever an item in the list is selected }
- See also:
- GuiControl
void GuiListBoxCtrl::onUnselect | ( | string | index, | |
string | itemText | |||
) |
Called whenever a selected item in the list has been unselected.
- Parameters:
-
index Index id of the item that was unselected itemText Text for the list entry at the index id that was unselected
- Example:
// A selected item is unselected, causing the callback to occur GuiListBoxCtrl::onUnSelect(%this, %indexId, %itemText) { // Code to run whenever a selected list item is unselected }
- See also:
- GuiControl
void GuiListBoxCtrl::removeFilteredItem | ( | string | itemName | ) |
Removes an item of the entered name from the filtered items list.
- Parameters:
-
itemName Name of the item to remove from the filtered list.
- Example:
// Define the itemName that you wish to remove. %itemName = "This Item Name"; // Remove the itemName from the GuiListBoxCtrl %thisGuiListBoxCtrl.removeFilteredItem(%itemName);
- See also:
- GuiControl
void GuiListBoxCtrl::setCurSel | ( | int | indexId | ) |
Sets the currently selected item at the specified index.
- Parameters:
-
indexId Index Id to set selected.
- Example:
// Define the index id that we wish to select. %selectId = "4"; // Inform the GuiListBoxCtrl object to set the requested index as selected. %thisGuiListBoxCtrl.setCurSel(%selectId);
- See also:
- GuiControl
void GuiListBoxCtrl::setCurSelRange | ( | int | indexStart, | |
int | indexStop = 999999 | |||
) |
Sets the current selection range from index start to stop. If no stop is specified it sets from start index to the end of the list.
- Parameters:
-
indexStart Index Id to start selection. indexStop Index Id to end selection.
- Example:
// Set start id %indexStart = "3"; // Set end id %indexEnd = "6"; // Request the GuiListBoxCtrl object to select the defined range. %thisGuiListBoxCtrl.setCurSelRange(%indexStart,%indexEnd);
- See also:
- GuiControl
void GuiListBoxCtrl::setItemColor | ( | int | index, | |
ColorF | color | |||
) |
Sets the color of a single list entry at the specified index id.
- Parameters:
-
index Index id to modify the color of in the list. color Color value to set the list entry to.
- Example:
// Define the index id value %index = "5"; // Define the color value %color = "1.0 0.0 0.0"; // Inform the GuiListBoxCtrl object to change the color of the requested index %thisGuiListBoxCtrl.setItemColor(%index,%color);
- See also:
- GuiControl
void GuiListBoxCtrl::setItemText | ( | int | index, | |
string | newtext | |||
) |
Sets the items text at the specified index.
- Parameters:
-
index Index id to set the item text at. newtext Text to change the list item at index id to.
- Example:
// Define the index id/n%index = "12"; // Define the text to set the list item to %newtext = "Gideon's Fancy Goggles"; // Inform the GuiListBoxCtrl object to change the text at the requested index %thisGuiListBoxCtrl.setItemText(%index,%newText);
- See also:
- GuiControl
void GuiListBoxCtrl::setItemTooltip | ( | int | index, | |
string | text | |||
) |
Set the tooltip text to display for the given list item.
- Parameters:
-
index Index id to change the tooltip text text Text for the tooltip.
- Example:
- See also:
- GuiControl
void GuiListBoxCtrl::setMultipleSelection | ( | bool | allowMultSelections | ) |
Enable or disable multiple selections for this GuiListBoxCtrl object.
- Parameters:
-
allowMultSelections Boolean variable to set the use of multiple selections or not.
- Example:
// Define the multiple selection use state. %allowMultSelections = "true"; // Set the allow multiple selection state on the GuiListBoxCtrl object. %thisGuiListBoxCtrl.setMultipleSelection(%allowMultSelections);
- See also:
- GuiControl
void GuiListBoxCtrl::setSelected | ( | int | index, | |
bool | setSelected = true | |||
) |
Sets the item at the index specified to selected or not.
Detailed description
- Parameters:
-
index Item index to set selected or unselected. setSelected Boolean selection state to set the requested item index.
- Example:
// Define the index %index = "5"; // Define the selection state %selected = "true" // Inform the GuiListBoxCtrl object of the new selection state for the requested index entry. %thisGuiListBoxCtrl.setSelected(%index,%selected);
- See also:
- GuiControl
Member Data Documentation
If true, will allow the selection of multiple items in the listbox.
If true, colored items will render a colored rectangular bullet next to the item text.
If true, the width of the listbox will match the width of its parent control.
A script snippet to control what is displayed in the list for a SimObject. Within this snippet, $ThisControl is bound to the guiListBoxCtrl and $ThisObject to the contained object in question.
string GuiListBoxCtrl::mirrorSet |
If populated with the name of another GuiListBoxCtrl, then this list box will mirror the contents of the mirrorSet listbox.