![]() |
|
PostEffect Class Reference
[Rendering]
A fullscreen shader effect. More...
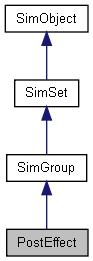
Public Member Functions | |
void | clearShaderMacros () |
Remove all shader macros. | |
void | disable () |
Disables the effect. | |
String | dumpShaderDisassembly () |
Dumps this PostEffect shader's disassembly to a temporary text file. | |
void | enable () |
Enables the effect. | |
float | getAspectRatio () |
bool | isEnabled () |
void | reload () |
Reloads the effect shader and textures. | |
void | removeShaderMacro (string key) |
Remove a shader macro. This will usually be called within the preProcess callback. | |
void | setShaderConst (string name, string value) |
Sets the value of a uniform defined in the shader. This will usually be called within the setShaderConsts callback. Array type constants are not supported. | |
void | setShaderMacro (string key, string value="") |
Adds a macro to the effect's shader or sets an existing one's value. This will usually be called within the onAdd or preProcess callback. | |
void | setTexture (int index, string filePath) |
This is used to set the texture file and load the texture on a running effect. If the texture file is not different from the current file nothing is changed. If the texture cannot be found a null texture is assigned. | |
bool | toggle () |
Toggles the effect between enabled / disabled. | |
Callbacks | |
void | onAdd () |
Called when this object is first created and registered. | |
void | onDisabled () |
Called when this effect becomes disabled. | |
bool | onEnabled () |
Called when this effect becomes enabled. If the user returns false from this callback the effect will not be enabled. | |
void | preProcess () |
Called when an effect is processed but before textures are bound. This allows the user to change texture related paramaters or macros at runtime. | |
void | setShaderConsts () |
Called immediate before processing this effect. This is the user's chance to set the value of shader uniforms (constants). | |
Public Attributes | |
bool | allowReflectPass |
Is this effect processed during reflection render passes. | |
bool | isEnabled |
Is the effect on. | |
bool | oneFrameOnly |
Allows you to turn on a PostEffect for only a single frame. | |
bool | onThisFrame |
Allows you to turn on a PostEffect for only a single frame. | |
string | renderBin |
Name of a renderBin, used if renderTime is PFXBeforeBin or PFXAfterBin. | |
float | renderPriority |
PostEffects are processed in DESCENDING order of renderPriority if more than one has the same renderBin/Time. | |
PFXRenderTime | renderTime |
When to process this effect during the frame. | |
string | shader |
Name of a GFXShaderData for this effect. | |
bool | skip |
Skip processing of this PostEffect and its children even if its parent is enabled. Parent and sibling PostEffects in the chain are still processed. | |
GFXStateBlockData | stateBlock |
Name of a GFXStateBlockData for this effect. | |
string | target |
String identifier of this effect's target texture. | |
PFXTargetClear | targetClear |
Describes when the target texture should be cleared. | |
ColorF | targetClearColor |
Color to which the target texture is cleared before rendering. | |
string | targetDepthStencil |
Optional string identifier for this effect's target depth/stencil texture. | |
GFXFormat | targetFormat |
Format of the target texture, not applicable if writing to the backbuffer. | |
Point2F | targetScale |
If targetSize is zero this is used to set a relative size from the current target. | |
Point2I | targetSize |
If non-zero this is used as the absolute target size. | |
filename | texture [6] |
Input textures to this effect ( samplers ). |
Detailed Description
A fullscreen shader effect.
PFXTextureIdentifiers
Member Function Documentation
void PostEffect::clearShaderMacros | ( | ) |
Remove all shader macros.
void PostEffect::disable | ( | ) |
Disables the effect.
String PostEffect::dumpShaderDisassembly | ( | ) |
Dumps this PostEffect shader's disassembly to a temporary text file.
- Returns:
- Full path to the dumped file or an empty string if failed.
void PostEffect::enable | ( | ) |
Enables the effect.
float PostEffect::getAspectRatio | ( | ) |
- Returns:
- Width over height of the backbuffer.
bool PostEffect::isEnabled | ( | ) |
- Returns:
- True if the effect is enabled.
void PostEffect::onAdd | ( | ) |
Called when this object is first created and registered.
void PostEffect::onDisabled | ( | ) |
Called when this effect becomes disabled.
bool PostEffect::onEnabled | ( | ) |
Called when this effect becomes enabled. If the user returns false from this callback the effect will not be enabled.
- Returns:
- True to allow this effect to be enabled.
void PostEffect::preProcess | ( | ) |
Called when an effect is processed but before textures are bound. This allows the user to change texture related paramaters or macros at runtime.
- Example:
function SSAOPostFx::preProcess( %this ) { if ( $SSAOPostFx::quality !$= %this.quality ) { %this.quality = mClamp( mRound( $SSAOPostFx::quality ), 0, 2 ); %this.setShaderMacro( "QUALITY", %this.quality ); } %this.targetScale = $SSAOPostFx::targetScale; }
- See also:
- setShaderConst
- setShaderMacro
void PostEffect::reload | ( | ) |
Reloads the effect shader and textures.
void PostEffect::removeShaderMacro | ( | string | key | ) |
Remove a shader macro. This will usually be called within the preProcess callback.
- Parameters:
-
key Macro to remove.
void PostEffect::setShaderConst | ( | string | name, | |
string | value | |||
) |
Sets the value of a uniform defined in the shader. This will usually be called within the setShaderConsts callback. Array type constants are not supported.
- Parameters:
-
name Name of the constanst, prefixed with '$'. value Value to set, space seperate values with more than one element.
- Example:
function MyPfx::setShaderConsts( %this ) { // example float4 uniform %this.setShaderConst( "$colorMod", "1.0 0.9 1.0 1.0" ); // example float1 uniform %this.setShaderConst( "$strength", "3.0" ); // example integer uniform %this.setShaderConst( "$loops", "5" );}
void PostEffect::setShaderConsts | ( | ) |
Called immediate before processing this effect. This is the user's chance to set the value of shader uniforms (constants).
- See also:
- setShaderConst
void PostEffect::setShaderMacro | ( | string | key, | |
string | value = "" | |||
) |
Adds a macro to the effect's shader or sets an existing one's value. This will usually be called within the onAdd or preProcess callback.
- Parameters:
-
key lval of the macro. value rval of the macro, or may be empty.
- Example:
function MyPfx::onAdd( %this ) { %this.setShaderMacro( "NUM_SAMPLES", "10" ); %this.setShaderMacro( "HIGH_QUALITY_MODE" ); // In the shader looks like... // #define NUM_SAMPLES 10 // #define HIGH_QUALITY_MODE }
void PostEffect::setTexture | ( | int | index, | |
string | filePath | |||
) |
This is used to set the texture file and load the texture on a running effect. If the texture file is not different from the current file nothing is changed. If the texture cannot be found a null texture is assigned.
- Parameters:
-
index The texture stage index. filePath The file name of the texture to set.
bool PostEffect::toggle | ( | ) |
Toggles the effect between enabled / disabled.
- Returns:
- True if effect is enabled.
Member Data Documentation
Is this effect processed during reflection render passes.
Is the effect on.
Allows you to turn on a PostEffect for only a single frame.
Allows you to turn on a PostEffect for only a single frame.
string PostEffect::renderBin |
Name of a renderBin, used if renderTime is PFXBeforeBin or PFXAfterBin.
PostEffects are processed in DESCENDING order of renderPriority if more than one has the same renderBin/Time.
When to process this effect during the frame.
string PostEffect::shader |
Name of a GFXShaderData for this effect.
bool PostEffect::skip |
Skip processing of this PostEffect and its children even if its parent is enabled. Parent and sibling PostEffects in the chain are still processed.
Name of a GFXStateBlockData for this effect.
string PostEffect::target |
String identifier of this effect's target texture.
- See also:
- PFXTextureIdentifiers
Describes when the target texture should be cleared.
ColorF PostEffect::targetClearColor |
Color to which the target texture is cleared before rendering.
Optional string identifier for this effect's target depth/stencil texture.
- See also:
- PFXTextureIdentifiers
Format of the target texture, not applicable if writing to the backbuffer.
Point2F PostEffect::targetScale |
If targetSize is zero this is used to set a relative size from the current target.
Point2I PostEffect::targetSize |
If non-zero this is used as the absolute target size.
filename PostEffect::texture[6] |
Input textures to this effect ( samplers ).
- See also:
- PFXTextureIdentifiers