![]() |
|
GuiControl Class Reference
[Core Controls]
Base class for all Gui control objects. More...
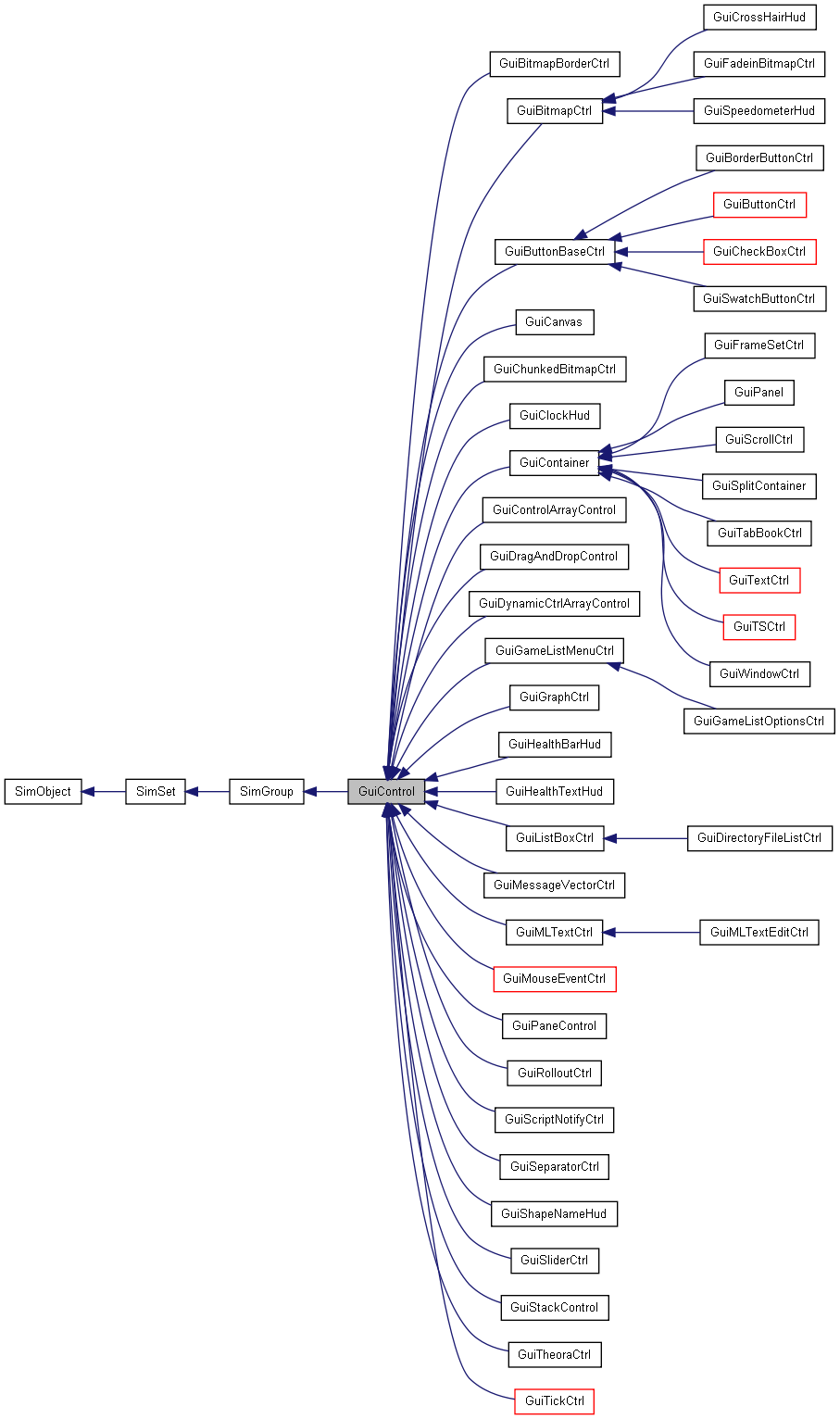
Public Member Functions | |
void | addGuiControl (GuiControl control) |
Add the given control as a child to this control. | |
void | clearFirstResponder (bool ignored=false) |
Clear this control from being the first responder in its hierarchy chain. | |
bool | controlIsChild (GuiControl control) |
Test whether the given control is a direct or indirect child to this control. | |
GuiControl | findHitControl (int x, int y) |
Find the topmost child control located at the given coordinates. | |
string | findHitControls (int x, int y, int width, int height) |
Find all visible child controls that intersect with the given rectangle. | |
float | getAspect () |
Get the aspect ratio of the control's extents. | |
Point2I | getCenter () |
Get the coordinate of the control's center point relative to its parent. | |
Point2I | getExtent () |
Get the width and height of the control. | |
GuiControl | getFirstResponder () |
Get the first responder set on this GuiControl tree. | |
Point2I | getGlobalCenter () |
Get the coordinate of the control's center point in coordinates relative to the root control in its control hierarchy. | |
Point2I | getGlobalPosition () |
Get the position of the control relative to the root of the GuiControl hierarchy it is contained in. | |
Point2I | getMinExtent () |
Get the minimum allowed size of the control. | |
GuiControl | getParent () |
Get the immediate parent control of the control. | |
Point2I | getPosition () |
Get the control's current position relative to its parent. | |
GuiCanvas | getRoot () |
Get the canvas on which the control is placed. | |
bool | isAwake () |
Test whether the control is currently awake. | |
bool | isFirstResponder () |
Test whether the control is the current first responder. | |
bool | isMouseLocked () |
Indicates if the mouse is locked in this control. | |
bool | isVisible () |
Test whether the control is currently set to be visible. | |
void | makeFirstResponder (bool isFirst) |
bool | pointInControl (int x, int y) |
Test whether the given point lies within the rectangle of the control. | |
void | resize (int x, int y, int width, int height) |
Resize and reposition the control using the give coordinates and dimensions. Child controls will resize according to their layout behaviors. | |
void | setActive (bool state=true) |
void | setCenter (int x, int y) |
Set the control's position by its center point. | |
void | setExtent (S32 width, S32 height) |
Resize the control to the given dimensions. | |
void | setExtent (Point2I p) |
Resize the control to the given dimensions. | |
void | setFirstResponder () |
Make this control the current first responder. | |
void | setPosition (int x, int y) |
Position the control in the local space of the parent control. | |
void | setPositionGlobal (int x, int y) |
Set position of the control relative to the root of the GuiControl hierarchy it is contained in. | |
void | setProfile (GuiControlProfile profile) |
Set the control profile for the control to use. | |
void | setValue (string value) |
Set the value associated with the control. | |
void | setVisible (bool state=true) |
Set whether the control is visible or not. | |
Callbacks | |
void | onAction () |
Called when the control's associated action is triggered and no 'command' is defined for the control. | |
void | onActive (bool state) |
Called when the control changes its activeness state, i.e. when going from active to inactive or vice versa. | |
void | onAdd () |
Called when the control object is registered with the system after the control has been created. | |
void | onControlDragEnter (GuiControl control, Point2I dropPoint) |
Called when a drag&drop operation through GuiDragAndDropControl has entered the control. This is only called for topmost visible controls as the GuiDragAndDropControl moves over them. | |
void | onControlDragExit (GuiControl control, Point2I dropPoint) |
Called when a drag&drop operation through GuiDragAndDropControl has exited the control and moved over a different control. This is only called for topmost visible controls as the GuiDragAndDropControl moves off of them. | |
void | onControlDragged (GuiControl control, Point2I dropPoint) |
Called when a drag&drop operation through GuiDragAndDropControl is moving across the control after it has entered it. This is only called for topmost visible controls as the GuiDragAndDropControl moves across them. | |
void | onControlDropped (GuiControl control, Point2I dropPoint) |
Called when a drag&drop operation through GuiDragAndDropControl has completed and is dropping its payload onto the control. This is only called for topmost visible controls as the GuiDragAndDropControl drops its payload on them. | |
void | onDialogPop () |
Called when the control is removed as a dialog from the canvas. | |
void | onDialogPush () |
Called when the control is pushed as a dialog onto the canvas. | |
void | onGainFirstResponder () |
Called when the control gains first responder status on the GuiCanvas. | |
void | onLoseFirstResponder () |
Called when the control loses first responder status on the GuiCanvas. | |
void | onRemove () |
Called when the control object is removed from the system before it is deleted. | |
void | onSleep () |
Called when the control is put to sleep. | |
void | onVisible (bool state) |
Called when the control changes its visibility state, i.e. when going from visible to invisible or vice versa. | |
void | onWake () |
Called when the control is woken up. | |
Public Attributes | |
string | getValue |
bool | isActive |
Control | |
string | accelerator |
Key combination that triggers the control's primary action when the control is on the canvas. | |
bool | active |
Whether the control is enabled for user interaction. | |
string | altCommand |
Command to execute on the secondary action of the control. | |
string | command |
Command to execute on the primary action of the control. | |
deprecated | modal |
GuiControlProfile | profile |
The control profile that determines fill styles, font settings, etc. | |
deprecated | setFirstResponder |
string | variable |
Name of the variable to which the value of this control will be synchronized. | |
bool | visible |
Whether the control is visible or hidden. | |
Layout | |
Point2I | extent |
The width and height of the control. | |
GuiHorizontalSizing | horizSizing |
The horizontal resizing behavior. | |
Point2I | minExtent |
The minimum width and height of the control. The control will not be resized smaller than this. | |
Point2I | position |
The position relative to the parent control. | |
GuiVerticalSizing | vertSizing |
The vertical resizing behavior. | |
ToolTip | |
int | hovertime |
Time for mouse to hover over control until tooltip is shown (in milliseconds). | |
string | tooltip |
String to show in tooltip for this control. | |
GuiControlProfile | tooltipProfile |
Control profile to use when rendering tooltips for this control. | |
Editing | |
bool | isContainer |
If true, the control may contain child controls. | |
Localization | |
string | langTableMod |
Name of string table to use for lookup of internationalized text. |
Detailed Description
Base class for all Gui control objects.
GuiControl is the basis for the Gui system. It represents an individual control that can be placed on the canvas and with which the mouse and keyboard can potentially interact with.
Control Hierarchies
GuiControls are arranged in a hierarchy. All children of a control are placed in their parent's coordinate space, i.e. their coordinates are relative to the upper left corner of their immediate parent. When a control is moved, all its child controls are moved along with it.
Since GuiControl's are SimGroups, hierarchy also implies ownership. This means that if a control is destroyed, all its children are destroyed along with it. It also means that a given control can only be part of a single GuiControl hierarchy. When adding a control to another control, it will automatically be reparented from another control it may have previously been parented to.
Layout System
GuiControls have a two-dimensional position and are rectangular in shape.
Event System
Control Profiles
Common data accessed by GuiControls is stored in so-called "Control Profiles." This includes font, color, and texture information. By pooling this data in shared objects, the appearance of any number of controls can be changed quickly and easily by modifying only the shared profile object.
If not explicitly assigned a profile, a control will by default look for a profile object that matches its class name. This means that the class GuiMyCtrl, for example, will look for a profile called 'GuiMyProfile'. If this profile cannot be found, the control will fall back to GuiDefaultProfile which must be defined in any case for the Gui system to work.
In addition to its primary profile, a control may be assigned a second profile called 'tooltipProfile' that will be used to render tooltip popups for the control.
Triggered Actions
First Responders
At any time, a single control can be what is called the "first responder" on the GuiCanvas is placed on. This control will be the first control to receive keyboard events not bound in the global ActionMap. If the first responder choses to handle a particular keyboard event,
Waking and Sleeping
Visibility and Activeness
By default, a GuiControl is active which means that it
- See also:
- GuiCanvas
- GuiControlProfile
Member Function Documentation
void GuiControl::addGuiControl | ( | GuiControl | control | ) |
Add the given control as a child to this control.
This is synonymous to calling SimGroup::addObject.
- Parameters:
-
control The control to add as a child.
- Note:
- The control will retain its current position and size.
- See also:
- SimGroup::addObject Control Hierarchies
void GuiControl::clearFirstResponder | ( | bool | ignored = false |
) |
Clear this control from being the first responder in its hierarchy chain.
- Parameters:
-
ignored Ignored. Supported for backwards-compatibility.
bool GuiControl::controlIsChild | ( | GuiControl | control | ) |
Test whether the given control is a direct or indirect child to this control.
- Parameters:
-
control The potential child control.
- Returns:
- True if the given control is a direct or indirect child to this control.
GuiControl GuiControl::findHitControl | ( | int | x, | |
int | y | |||
) |
Find the topmost child control located at the given coordinates.
- Note:
- Only children that are both visible and have the 'modal' flag set in their profile will be considered in the search.
- Parameters:
-
x The X coordinate in the control's own coordinate space. y The Y coordinate in the control's own coordinate space.
- Returns:
- The topmost child control at the given coordintes or the control on which the method was called if no matching child could be found.
- See also:
- GuiControlProfile::modal
- findHitControls
string GuiControl::findHitControls | ( | int | x, | |
int | y, | |||
int | width, | |||
int | height | |||
) |
Find all visible child controls that intersect with the given rectangle.
- Note:
- Invisible child controls will not be included in the search.
- Parameters:
-
x The X coordinate of the rectangle's upper left corner in the control's own coordinate space. y The Y coordinate of the rectangle's upper left corner in the control's own coordinate space. width The width of the search rectangle in pixels. height The height of the search rectangle in pixels.
- Returns:
- A space-separated list of the IDs of all visible control objects intersecting the given rectangle.
- Example:
// Lock all controls in the rectangle at x=10 and y=10 and the extent width=100 and height=100. foreach$( %ctrl in %this.findHitControls( 10, 10, 100, 100 ) ) %ctrl.setLocked( true );
- See also:
- findHitControl
float GuiControl::getAspect | ( | ) |
Get the aspect ratio of the control's extents.
- Returns:
- The width of the control divided by its height.
- See also:
- getExtent
Point2I GuiControl::getCenter | ( | ) |
Get the coordinate of the control's center point relative to its parent.
- Returns:
- The coordinate of the control's center point in parent-relative coordinates.
Point2I GuiControl::getExtent | ( | ) |
Get the width and height of the control.
- Returns:
- A point structure containing the width of the control in x and the height in y.
Reimplemented in GuiCanvas.
GuiControl GuiControl::getFirstResponder | ( | ) |
Get the first responder set on this GuiControl tree.
- Returns:
- The first responder set on the control's subtree.
Point2I GuiControl::getGlobalCenter | ( | ) |
Get the coordinate of the control's center point in coordinates relative to the root control in its control hierarchy.
the center coordinate of the control in root-relative coordinates.
Point2I GuiControl::getGlobalPosition | ( | ) |
Get the position of the control relative to the root of the GuiControl hierarchy it is contained in.
- Returns:
- The control's current position in root-relative coordinates.
Point2I GuiControl::getMinExtent | ( | ) |
Get the minimum allowed size of the control.
- Returns:
- The minimum size to which the control can be shrunk.
- See also:
- minExtent
GuiControl GuiControl::getParent | ( | ) |
Get the immediate parent control of the control.
- Returns:
- The immediate parent GuiControl or 0 if the control is not parented to a GuiControl.
Point2I GuiControl::getPosition | ( | ) |
Get the control's current position relative to its parent.
- Returns:
- The coordinate of the control in its parent's coordinate space.
GuiCanvas GuiControl::getRoot | ( | ) |
Get the canvas on which the control is placed.
- Returns:
- The canvas on which the control's hierarchy is currently placed or 0 if the control is not currently placed on a GuiCanvas.
- See also:
- GuiControl_Hierarchy
bool GuiControl::isAwake | ( | ) |
Test whether the control is currently awake.
If a control is awake it means that it is part of the GuiControl hierarchy of a GuiCanvas.
- Returns:
- True if the control is awake.Waking and Sleeping
bool GuiControl::isFirstResponder | ( | ) |
Test whether the control is the current first responder.
- Returns:
- True if the control is the current first responder.
bool GuiControl::isMouseLocked | ( | ) |
Indicates if the mouse is locked in this control.
- Returns:
- True if the mouse is currently locked.
bool GuiControl::isVisible | ( | ) |
Test whether the control is currently set to be visible.
- Returns:
- True if the control is currently set to be visible.
- Note:
- This method does not tell anything about whether the control is actually visible to the user at the moment.
void GuiControl::makeFirstResponder | ( | bool | isFirst | ) |
void GuiControl::onAction | ( | ) |
Called when the control's associated action is triggered and no 'command' is defined for the control.
void GuiControl::onActive | ( | bool | state | ) |
Called when the control changes its activeness state, i.e. when going from active to inactive or vice versa.
- Parameters:
-
stat The new activeness state.
- See also:
- isActive
- setActive Visibility and Activeness
void GuiControl::onAdd | ( | ) |
Called when the control object is registered with the system after the control has been created.
void GuiControl::onControlDragEnter | ( | GuiControl | control, | |
Point2I | dropPoint | |||
) |
Called when a drag&drop operation through GuiDragAndDropControl has entered the control. This is only called for topmost visible controls as the GuiDragAndDropControl moves over them.
- Parameters:
-
control The payload of the drag operation. dropPoint The point at which the payload would be dropped if it were released now. Relative to the canvas.
void GuiControl::onControlDragExit | ( | GuiControl | control, | |
Point2I | dropPoint | |||
) |
Called when a drag&drop operation through GuiDragAndDropControl has exited the control and moved over a different control. This is only called for topmost visible controls as the GuiDragAndDropControl moves off of them.
- Parameters:
-
control The payload of the drag operation. dropPoint The point at which the payload would be dropped if it were released now. Relative to the canvas.
void GuiControl::onControlDragged | ( | GuiControl | control, | |
Point2I | dropPoint | |||
) |
Called when a drag&drop operation through GuiDragAndDropControl is moving across the control after it has entered it. This is only called for topmost visible controls as the GuiDragAndDropControl moves across them.
- Parameters:
-
control The payload of the drag operation. dropPoint The point at which the payload would be dropped if it were released now. Relative to the canvas.
void GuiControl::onControlDropped | ( | GuiControl | control, | |
Point2I | dropPoint | |||
) |
Called when a drag&drop operation through GuiDragAndDropControl has completed and is dropping its payload onto the control. This is only called for topmost visible controls as the GuiDragAndDropControl drops its payload on them.
- Parameters:
-
control The control that is being dropped onto this control. dropPoint The point at which the control is being dropped. Relative to the canvas.
void GuiControl::onDialogPop | ( | ) |
Called when the control is removed as a dialog from the canvas.
- See also:
- GuiCanvas::popDialog
void GuiControl::onDialogPush | ( | ) |
Called when the control is pushed as a dialog onto the canvas.
- See also:
- GuiCanvas::pushDialog
void GuiControl::onGainFirstResponder | ( | ) |
Called when the control gains first responder status on the GuiCanvas.
void GuiControl::onLoseFirstResponder | ( | ) |
Called when the control loses first responder status on the GuiCanvas.
void GuiControl::onRemove | ( | ) |
Called when the control object is removed from the system before it is deleted.
void GuiControl::onSleep | ( | ) |
Called when the control is put to sleep.
void GuiControl::onVisible | ( | bool | state | ) |
Called when the control changes its visibility state, i.e. when going from visible to invisible or vice versa.
- Parameters:
-
state The new visibility state.
- See also:
- isVisible
- setVisible Visibility and Activeness
void GuiControl::onWake | ( | ) |
Called when the control is woken up.
bool GuiControl::pointInControl | ( | int | x, | |
int | y | |||
) |
Test whether the given point lies within the rectangle of the control.
- Parameters:
-
x X coordinate of the point in parent-relative coordinates. y Y coordinate of the point in parent-relative coordinates.
- Returns:
- True if the point is within the control, false if not.
- See also:
- getExtent
- getPosition
void GuiControl::resize | ( | int | x, | |
int | y, | |||
int | width, | |||
int | height | |||
) |
Resize and reposition the control using the give coordinates and dimensions. Child controls will resize according to their layout behaviors.
- Parameters:
-
x The new X coordinate of the control in its parent's coordinate space. y The new Y coordinate of the control in its parent's coordinate space. width The new width to which the control should be resized. height The new height to which the control should be resized.
void GuiControl::setActive | ( | bool | state = true |
) |
void GuiControl::setCenter | ( | int | x, | |
int | y | |||
) |
Set the control's position by its center point.
- Parameters:
-
x The X coordinate of the new center point of the control relative to the control's parent. y The Y coordinate of the new center point of the control relative to the control's parent.
void GuiControl::setExtent | ( | S32 | width, | |
S32 | height | |||
) |
Resize the control to the given dimensions.
Child controls will resize according to their layout settings.
- Parameters:
-
width The new width of the control in pixels. height The new height of the control in pixels.
void GuiControl::setExtent | ( | Point2I | p | ) |
Resize the control to the given dimensions.
Child controls with resize according to their layout settings.
- Parameters:
-
p The new ( width, height ) extents of the control.
void GuiControl::setFirstResponder | ( | ) |
Make this control the current first responder.
- Note:
- Only controls with a profile that has canKeyFocus enabled are able to become first responders.
void GuiControl::setPosition | ( | int | x, | |
int | y | |||
) |
Position the control in the local space of the parent control.
- Parameters:
-
x The new X coordinate of the control relative to its parent's upper left corner. y The new Y coordinate of the control relative to its parent's upper left corner.
void GuiControl::setPositionGlobal | ( | int | x, | |
int | y | |||
) |
Set position of the control relative to the root of the GuiControl hierarchy it is contained in.
- Parameters:
-
x The new X coordinate of the control relative to the root's upper left corner. y The new Y coordinate of the control relative to the root's upper left corner.
void GuiControl::setProfile | ( | GuiControlProfile | profile | ) |
Set the control profile for the control to use.
The profile used by a control determines a great part of its behavior and appearance.
- Parameters:
-
profile The new profile the control should use. Control Profiles
void GuiControl::setValue | ( | string | value | ) |
Set the value associated with the control.
- Parameters:
-
value The new value for the control.
void GuiControl::setVisible | ( | bool | state = true |
) |
Set whether the control is visible or not.
- Parameters:
-
state The new visiblity flag state for the control. Visibility and Activeness
Member Data Documentation
string GuiControl::accelerator |
Key combination that triggers the control's primary action when the control is on the canvas.
bool GuiControl::active |
Whether the control is enabled for user interaction.
string GuiControl::altCommand |
Command to execute on the secondary action of the control.
- Note:
- Within this script snippet, the control on which the altCommand is being executed is bound to the global variable $ThisControl.
string GuiControl::command |
Command to execute on the primary action of the control.
- Note:
- Within this script snippet, the control on which the command is being executed is bound to the global variable $ThisControl.
Point2I GuiControl::extent |
The width and height of the control.
string GuiControl::getValue |
The horizontal resizing behavior.
Time for mouse to hover over control until tooltip is shown (in milliseconds).
bool GuiControl::isActive |
If true, the control may contain child controls.
string GuiControl::langTableMod |
Name of string table to use for lookup of internationalized text.
Point2I GuiControl::minExtent |
The minimum width and height of the control. The control will not be resized smaller than this.
deprecated GuiControl::modal |
Point2I GuiControl::position |
The position relative to the parent control.
The control profile that determines fill styles, font settings, etc.
deprecated GuiControl::setFirstResponder |
string GuiControl::tooltip |
String to show in tooltip for this control.
Control profile to use when rendering tooltips for this control.
string GuiControl::variable |
Name of the variable to which the value of this control will be synchronized.
The vertical resizing behavior.
bool GuiControl::visible |
Whether the control is visible or hidden.