![]() |
|
GuiCanvas Class Reference
[Core Controls]
A canvas on which rendering occurs. More...
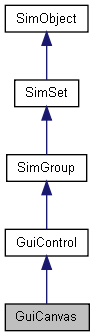
Public Member Functions | |
Point2I | clientToScreen (Point2I coordinate) |
Translate a coordinate from canvas window-space to screen-space. | |
void | cursorOff () |
Turns on the mouse off. | |
void | cursorOn () |
Turns on the mouse cursor. | |
int | getContent () |
Get the GuiControl which is being used as the content. | |
Point2I | getCursorPos () |
Get the current position of the cursor. | |
Point2I | getExtent () |
Returns the dimensions of the canvas. | |
string | getMode (int modeId) |
Gets information on the specified mode of this device. | |
int | getModeCount () |
Gets the number of modes available on this device. | |
int | getMouseControl () |
Gets the gui control under the mouse. | |
string | getVideoMode () |
Gets the current screen mode as a string. | |
Point2I | getWindowPosition () |
Get the current position of the platform window associated with the canvas. | |
void | hideCursor () |
Disable rendering of the cursor. | |
bool | isCursorOn () |
Determines if mouse cursor is enabled. | |
bool | isCursorShown () |
Determines if mouse cursor is rendering. | |
bool | isFullscreen () |
Is this canvas currently fullscreen? | |
bool | isMaximized () |
bool | isMinimized () |
void | maximizeWindow () |
maximize this canvas' window. | |
void | minimizeWindow () |
minimize this canvas' window. | |
void | popDialog (GuiControl ctrl) |
Removes a specific dialog control. | |
void | popDialog () |
Removes a dialog at the front most layer. | |
void | popLayer () |
Removes the top most layer of dialogs. | |
void | popLayer (S32 layer) |
Removes a specified layer of dialogs. | |
void | pushDialog (GuiControl ctrl, int layer=0, bool center=false) |
Adds a dialog control onto the stack of dialogs. | |
void | renderFront (bool enable) |
This turns on/off front-buffer rendering. | |
void | repaint (int elapsedMS=0) |
Force canvas to redraw. If the elapsed time is greater than the time since the last paint then the repaint will be skipped. | |
void | reset () |
Reset the update regions for the canvas. | |
void | restoreWindow () |
restore this canvas' window. | |
Point2I | screenToClient (Point2I coordinate) |
Translate a coordinate from screen-space to canvas window-space. | |
void | setContent (GuiControl ctrl) |
Set the content of the canvas to a specified control. | |
void | setCursor (GuiCursor cursor) |
Sets the cursor for the canvas. | |
bool | setCursorPos (Point2I pos) |
Sets the position of the cursor. | |
bool | setCursorPos (F32 posX, F32 posY) |
Sets the position of the cursor. | |
void | setFocus () |
Claim OS input focus for this canvas' window. | |
void | setVideoMode (int width, int height, bool fullscreen,[int bitDepth],[int refreshRate],[int antialiasLevel]) |
Change the video mode of this canvas. This method has the side effect of setting the $pref::Video::mode to the new values. | |
void | setWindowPosition (Point2I position) |
Set the position of the platform window associated with the canvas. | |
void | setWindowTitle (string newTitle) |
Change the title of the OS window. | |
void | showCursor () |
Enable rendering of the cursor. | |
void | toggleFullscreen () |
toggle canvas from fullscreen to windowed mode or back. | |
Public Attributes | |
Mouse Handling | |
bool | alwaysHandleMouseButtons |
Deal with mouse buttons, even if the cursor is hidden. | |
Canvas Rendering | |
int | numFences |
The number of GFX fences to use. |
Detailed Description
A canvas on which rendering occurs.
What a GUICanvas Can Contain...
Content Control
A content control is the top level GuiControl for a screen. This GuiControl will be the parent control for all other GuiControls on that particular screen.
Dialogs
A dialog is essentially another screen, only it gets overlaid on top of the current content control, and all input goes to the dialog. This is most akin to the "Open File" dialog box found in most operating systems. When you choose to open a file, and the "Open File" dialog pops up, you can no longer send input to the application, and must complete or cancel the open file request. Torque keeps track of layers of dialogs. The dialog with the highest layer is on top and will get all the input, unless the dialog is modeless, which is a profile option.
- See also:
- GuiControlProfile
Dirty Rectangles
The GuiCanvas is based on dirty regions. Every frame the canvas paints only the areas of the canvas that are 'dirty' or need updating. In most cases, this only is the area under the mouse cursor. This is why if you look in guiCanvas.cc the call to glClear is commented out. What you will see is a black screen, except in the dirty regions, where the screen will be painted normally. If you are making an animated GuiControl you need to add your control to the dirty areas of the canvas.
- See also:
- GuiControl
Member Function Documentation
Point2I GuiCanvas::clientToScreen | ( | Point2I | coordinate | ) |
Translate a coordinate from canvas window-space to screen-space.
- Parameters:
-
coordinate The coordinate in window-space.
- Returns:
- The given coordinate translated to screen-space.
void GuiCanvas::cursorOff | ( | ) |
Turns on the mouse off.
- Example:
Canvas.cursorOff();
void GuiCanvas::cursorOn | ( | ) |
Turns on the mouse cursor.
- Example:
Canvas.cursorOn();
int GuiCanvas::getContent | ( | ) |
Get the GuiControl which is being used as the content.
- Example:
Canvas.getContent();
- Returns:
- ID of current content control
Point2I GuiCanvas::getCursorPos | ( | ) |
Get the current position of the cursor.
- Parameters:
-
param Description
- Example:
%cursorPos = Canvas.getCursorPos();
- Returns:
- Screen coordinates of mouse cursor, in format "X Y"
Point2I GuiCanvas::getExtent | ( | ) |
Returns the dimensions of the canvas.
- Example:
%extent = Canvas.getExtent();
- Returns:
- Width and height of canvas. Formatted as numerical values in a single string "# #"
Reimplemented from GuiControl.
string GuiCanvas::getMode | ( | int | modeId | ) |
Gets information on the specified mode of this device.
- Parameters:
-
modeId Index of the mode to get data from.
- Returns:
- A video mode string given an adapter and mode index.
- See also:
- GuiCanvas::getVideoMode()
int GuiCanvas::getModeCount | ( | ) |
Gets the number of modes available on this device.
- Parameters:
-
param Description
- Example:
%modeCount = Canvas.getModeCount()
- Returns:
- The number of video modes supported by the device
int GuiCanvas::getMouseControl | ( | ) |
Gets the gui control under the mouse.
- Example:
%underMouse = Canvas.getMouseControl();
- Returns:
- ID of the gui control, if one was found. NULL otherwise
string GuiCanvas::getVideoMode | ( | ) |
Gets the current screen mode as a string.
The return string will contain 5 values (width, height, fullscreen, bitdepth, refreshRate). You will need to parse out each one for individual use.
- Example:
- Returns:
- String formatted with screen width, screen height, screen mode, bit depth, and refresh rate.
Point2I GuiCanvas::getWindowPosition | ( | ) |
Get the current position of the platform window associated with the canvas.
- Returns:
- The window position of the canvas in screen-space.
void GuiCanvas::hideCursor | ( | ) |
Disable rendering of the cursor.
- Example:
Canvas.hideCursor();
bool GuiCanvas::isCursorOn | ( | ) |
Determines if mouse cursor is enabled.
- Example:
// Is cursor on? if(Canvas.isCursorOn()) echo("Canvas cursor is on");
- Returns:
- Returns true if the cursor is on.
bool GuiCanvas::isCursorShown | ( | ) |
Determines if mouse cursor is rendering.
- Example:
// Is cursor rendering? if(Canvas.isCursorShown()) echo("Canvas cursor is rendering");
- Returns:
- Returns true if the cursor is rendering.
bool GuiCanvas::isFullscreen | ( | ) |
Is this canvas currently fullscreen?
bool GuiCanvas::isMaximized | ( | ) |
bool GuiCanvas::isMinimized | ( | ) |
void GuiCanvas::maximizeWindow | ( | ) |
maximize this canvas' window.
void GuiCanvas::minimizeWindow | ( | ) |
minimize this canvas' window.
void GuiCanvas::popDialog | ( | GuiControl | ctrl | ) |
Removes a specific dialog control.
- Parameters:
-
ctrl Dialog to pop
- Example:
Canvas.popDialog(RecordingsDlg);
void GuiCanvas::popDialog | ( | ) |
Removes a dialog at the front most layer.
- Example:
// Pops whatever is on layer 0 Canvas.popDialog();
void GuiCanvas::popLayer | ( | ) |
Removes the top most layer of dialogs.
- Example:
Canvas.popLayer();
void GuiCanvas::popLayer | ( | S32 | layer | ) |
Removes a specified layer of dialogs.
- Parameters:
-
layer Number of the layer to pop
- Example:
Canvas.popLayer(1);
void GuiCanvas::pushDialog | ( | GuiControl | ctrl, | |
int | layer = 0 , |
|||
bool | center = false | |||
) |
Adds a dialog control onto the stack of dialogs.
- Parameters:
-
ctrl Dialog to add layer Layer to put dialog on (optional) center True to center dialog on canvas (optional)
- Example:
Canvas.pushDialog(RecordingsDlg);
void GuiCanvas::renderFront | ( | bool | enable | ) |
This turns on/off front-buffer rendering.
- Parameters:
-
enable True if all rendering should be done to the front buffer
- Example:
Canvas.renderFront(false);
void GuiCanvas::repaint | ( | int | elapsedMS = 0 |
) |
Force canvas to redraw. If the elapsed time is greater than the time since the last paint then the repaint will be skipped.
- Parameters:
-
elapsedMS The optional elapsed time in milliseconds.
- Example:
Canvas.repaint();
void GuiCanvas::reset | ( | ) |
Reset the update regions for the canvas.
- Example:
Canvas.reset();
void GuiCanvas::restoreWindow | ( | ) |
restore this canvas' window.
Point2I GuiCanvas::screenToClient | ( | Point2I | coordinate | ) |
Translate a coordinate from screen-space to canvas window-space.
- Parameters:
-
coordinate The coordinate in screen-space.
- Returns:
- The given coordinate translated to window-space.
void GuiCanvas::setContent | ( | GuiControl | ctrl | ) |
Set the content of the canvas to a specified control.
- Parameters:
-
ctrl ID or name of GuiControl to set content to
- Example:
Canvas.setContent(PlayGui);
void GuiCanvas::setCursor | ( | GuiCursor | cursor | ) |
Sets the cursor for the canvas.
- Parameters:
-
cursor Name of the GuiCursor to use
- Example:
Canvas.setCursor("DefaultCursor");
bool GuiCanvas::setCursorPos | ( | Point2I | pos | ) |
Sets the position of the cursor.
- Parameters:
-
pos Point, in screenspace for the cursor. Formatted as ("x y")
- Example:
Canvas.setCursorPos("0 0");
bool GuiCanvas::setCursorPos | ( | F32 | posX, | |
F32 | posY | |||
) |
Sets the position of the cursor.
- Parameters:
-
posX X-coordinate, in screenspace for the cursor. posY Y-coordinate, in screenspace for the cursor.
- Example:
Canvas.setCursorPos(0,0);
void GuiCanvas::setFocus | ( | ) |
Claim OS input focus for this canvas' window.
void GuiCanvas::setVideoMode | ( | int | width, | |
int | height, | |||
bool | fullscreen | |||
) |
Change the video mode of this canvas. This method has the side effect of setting the $pref::Video::mode to the new values.
- Parameters:
-
width The screen width to set. height The screen height to set. fullscreen Specify true to run fullscreen or false to run in a window bitDepth [optional] The desired bit-depth. Defaults to the current setting. This parameter is ignored if you are running in a window. refreshRate [optional] The desired refresh rate. Defaults to the current setting. This parameter is ignored if you are running in a window antialiasLevel [optional] The level of anti-aliasing to apply 0 = none
void GuiCanvas::setWindowPosition | ( | Point2I | position | ) |
Set the position of the platform window associated with the canvas.
- Parameters:
-
position The new position of the window in screen-space.
void GuiCanvas::setWindowTitle | ( | string | newTitle | ) |
Change the title of the OS window.
- Parameters:
-
newTitle String containing the new name
- Example:
Canvas.setWindowTitle("Documentation Rocks!");
void GuiCanvas::showCursor | ( | ) |
Enable rendering of the cursor.
- Example:
Canvas.showCursor();
void GuiCanvas::toggleFullscreen | ( | ) |
toggle canvas from fullscreen to windowed mode or back.
- Example:
// If we are in windowed mode, the following will put is in fullscreen Canvas.toggleFullscreen();
Member Data Documentation
Deal with mouse buttons, even if the cursor is hidden.
The number of GFX fences to use.