![]() |
|
GuiMouseEventCtrl Class Reference
[Core Controls]
Used to overlaps a 'hot region' where you want to catch inputs with and have specific events occur based on individual callbacks. More...
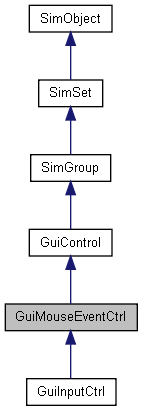
Public Member Functions | |
Callbacks | |
void | onMouseDown (U8 modifier, Point2I mousePoint, U8 mouseClickCount) |
Callback that occurs whenever the mouse is pressed down while in this control. | |
void | onMouseDragged (U8 modifier, Point2I mousePoint, U8 mouseClickCount) |
Callback that occurs whenever the mouse is dragged while in this control. | |
void | onMouseEnter (U8 modifier, Point2I mousePoint, U8 mouseClickCount) |
Callback that occurs whenever the mouse enters this control. | |
void | onMouseLeave (U8 modifier, Point2I mousePoint, U8 mouseClickCount) |
Callback that occurs whenever the mouse leaves this control. | |
void | onMouseMove (U8 modifier, Point2I mousePoint, U8 mouseClickCount) |
Callback that occurs whenever the mouse is moved (without dragging) while in this control. | |
void | onMouseUp (U8 modifier, Point2I mousePoint, U8 mouseClickCount) |
Callback that occurs whenever the mouse is released while in this control. | |
void | onRightMouseDown (U8 modifier, Point2I mousePoint, U8 mouseClickCount) |
Callback that occurs whenever the right mouse button is pressed while in this control. | |
void | onRightMouseDragged (U8 modifier, Point2I mousePoint, U8 mouseClickCount) |
Callback that occurs whenever the mouse is dragged in this control while the right mouse button is pressed. | |
void | onRightMouseUp (U8 modifier, Point2I mousePoint, U8 mouseClickCount) |
Callback that occurs whenever the right mouse button is released while in this control. | |
Public Attributes | |
Input | |
bool | lockMouse |
Whether the control should lock the mouse between up and down button events. |
Detailed Description
Used to overlaps a 'hot region' where you want to catch inputs with and have specific events occur based on individual callbacks.
Mouse event callbacks supported by this control are: onMouseUp, onMouseDown, onMouseMove, onMouseDragged, onMouseEnter, onMouseLeave, onRightMouseDown, onRightMouseUp and onRightMouseDragged.
- Example:
new GuiMouseEventCtrl() { lockMouse = "0"; //Properties not specific to this control have been omitted from this example. };
- See also:
- GuiControl
Member Function Documentation
void GuiMouseEventCtrl::onMouseDown | ( | U8 | modifier, | |
Point2I | mousePoint, | |||
U8 | mouseClickCount | |||
) |
Callback that occurs whenever the mouse is pressed down while in this control.
- Parameters:
-
modifier Key that was pressed during this callback. Values are:
$EventModifier::RSHIFT
$EventModifier::SHIFT
$EventModifier::LCTRL
$EventModifier::RCTRL
$EventModifier::CTRL
$EventModifier::CTRL
$EventModifier::RALT
$EventModifier::ALT
- Parameters:
-
mousePoint X/Y location of the mouse point mouseClickCount How many mouse clicks have occured for this event
- Example:
// Mouse was pressed down in this control, causing the callback GuiMouseEventCtrl::onMouseDown(%this,%modifier,%mousePoint,%mouseClickCount) { // Code to call when a mouse event occurs. }
- See also:
- GuiControl
void GuiMouseEventCtrl::onMouseDragged | ( | U8 | modifier, | |
Point2I | mousePoint, | |||
U8 | mouseClickCount | |||
) |
Callback that occurs whenever the mouse is dragged while in this control.
- Parameters:
-
modifier Key that was pressed during this callback. Values are:
$EventModifier::RSHIFT
$EventModifier::SHIFT
$EventModifier::LCTRL
$EventModifier::RCTRL
$EventModifier::CTRL
$EventModifier::CTRL
$EventModifier::RALT
$EventModifier::ALT
- Parameters:
-
mousePoint X/Y location of the mouse point mouseClickCount How many mouse clicks have occured for this event
- Example:
// Mouse was dragged in this control, causing the callback GuiMouseEventCtrl::onMouseDragged(%this,%modifier,%mousePoint,%mouseClickCount) { // Code to call when a mouse event occurs. }
- See also:
- GuiControl
void GuiMouseEventCtrl::onMouseEnter | ( | U8 | modifier, | |
Point2I | mousePoint, | |||
U8 | mouseClickCount | |||
) |
Callback that occurs whenever the mouse enters this control.
- Parameters:
-
modifier Key that was pressed during this callback. Values are:
$EventModifier::RSHIFT
$EventModifier::SHIFT
$EventModifier::LCTRL
$EventModifier::RCTRL
$EventModifier::CTRL
$EventModifier::CTRL
$EventModifier::RALT
$EventModifier::ALT
- Parameters:
-
mousePoint X/Y location of the mouse point mouseClickCount How many mouse clicks have occured for this event
- Example:
// Mouse entered this control, causing the callback GuiMouseEventCtrl::onMouseEnter(%this,%modifier,%mousePoint,%mouseClickCount) { // Code to call when a mouse event occurs. }
- See also:
- GuiControl
void GuiMouseEventCtrl::onMouseLeave | ( | U8 | modifier, | |
Point2I | mousePoint, | |||
U8 | mouseClickCount | |||
) |
Callback that occurs whenever the mouse leaves this control.
- Parameters:
-
modifier Key that was pressed during this callback. Values are:
$EventModifier::RSHIFT
$EventModifier::SHIFT
$EventModifier::LCTRL
$EventModifier::RCTRL
$EventModifier::CTRL
$EventModifier::CTRL
$EventModifier::RALT
$EventModifier::ALT
- Parameters:
-
mousePoint X/Y location of the mouse point mouseClickCount How many mouse clicks have occured for this event
- Example:
// Mouse left this control, causing the callback GuiMouseEventCtrl::onMouseLeave(%this,%modifier,%mousePoint,%mouseClickCount) { // Code to call when a mouse event occurs. }
- See also:
- GuiControl
void GuiMouseEventCtrl::onMouseMove | ( | U8 | modifier, | |
Point2I | mousePoint, | |||
U8 | mouseClickCount | |||
) |
Callback that occurs whenever the mouse is moved (without dragging) while in this control.
- Parameters:
-
modifier Key that was pressed during this callback. Values are:
$EventModifier::RSHIFT
$EventModifier::SHIFT
$EventModifier::LCTRL
$EventModifier::RCTRL
$EventModifier::CTRL
$EventModifier::CTRL
$EventModifier::RALT
$EventModifier::ALT
- Parameters:
-
mousePoint X/Y location of the mouse point mouseClickCount How many mouse clicks have occured for this event
- Example:
// Mouse was moved in this control, causing the callback GuiMouseEventCtrl::onMouseMove(%this,%modifier,%mousePoint,%mouseClickCount) { // Code to call when a mouse event occurs. }
- See also:
- GuiControl
void GuiMouseEventCtrl::onMouseUp | ( | U8 | modifier, | |
Point2I | mousePoint, | |||
U8 | mouseClickCount | |||
) |
Callback that occurs whenever the mouse is released while in this control.
- Parameters:
-
modifier Key that was pressed during this callback. Values are:
$EventModifier::RSHIFT
$EventModifier::SHIFT
$EventModifier::LCTRL
$EventModifier::RCTRL
$EventModifier::CTRL
$EventModifier::CTRL
$EventModifier::RALT
$EventModifier::ALT
- Parameters:
-
mousePoint X/Y location of the mouse point mouseClickCount How many mouse clicks have occured for this event
- Example:
// Mouse was released in this control, causing the callback GuiMouseEventCtrl::onMouseUp(%this,%modifier,%mousePoint,%mouseClickCount) { // Code to call when a mouse event occurs. }
- See also:
- GuiControl
void GuiMouseEventCtrl::onRightMouseDown | ( | U8 | modifier, | |
Point2I | mousePoint, | |||
U8 | mouseClickCount | |||
) |
Callback that occurs whenever the right mouse button is pressed while in this control.
- Parameters:
-
modifier Key that was pressed during this callback. Values are:
$EventModifier::RSHIFT
$EventModifier::SHIFT
$EventModifier::LCTRL
$EventModifier::RCTRL
$EventModifier::CTRL
$EventModifier::CTRL
$EventModifier::RALT
$EventModifier::ALT
- Parameters:
-
mousePoint X/Y location of the mouse point mouseClickCount How many mouse clicks have occured for this event
- Example:
// Right mouse button was pressed in this control, causing the callback GuiMouseEventCtrl::onRightMouseDown(%this,%modifier,%mousePoint,%mouseClickCount) { // Code to call when a mouse event occurs. }
- See also:
- GuiControl
void GuiMouseEventCtrl::onRightMouseDragged | ( | U8 | modifier, | |
Point2I | mousePoint, | |||
U8 | mouseClickCount | |||
) |
Callback that occurs whenever the mouse is dragged in this control while the right mouse button is pressed.
- Parameters:
-
modifier Key that was pressed during this callback. Values are:
$EventModifier::RSHIFT
$EventModifier::SHIFT
$EventModifier::LCTRL
$EventModifier::RCTRL
$EventModifier::CTRL
$EventModifier::CTRL
$EventModifier::RALT
$EventModifier::ALT
- Parameters:
-
mousePoint X/Y location of the mouse point mouseClickCount How many mouse clicks have occured for this event
- Example:
// Right mouse button was dragged in this control, causing the callback GuiMouseEventCtrl::onRightMouseDragged(%this,%modifier,%mousePoint,%mouseClickCount) { // Code to call when a mouse event occurs. }
- See also:
- GuiControl
void GuiMouseEventCtrl::onRightMouseUp | ( | U8 | modifier, | |
Point2I | mousePoint, | |||
U8 | mouseClickCount | |||
) |
Callback that occurs whenever the right mouse button is released while in this control.
- Parameters:
-
modifier Key that was pressed during this callback. Values are:
$EventModifier::RSHIFT
$EventModifier::SHIFT
$EventModifier::LCTRL
$EventModifier::RCTRL
$EventModifier::CTRL
$EventModifier::CTRL
$EventModifier::RALT
$EventModifier::ALT
- Parameters:
-
mousePoint X/Y location of the mouse point mouseClickCount How many mouse clicks have occured for this event
- Example:
// Right mouse button was released in this control, causing the callback GuiMouseEventCtrl::onRightMouseUp(%this,%modifier,%mousePoint,%mouseClickCount) { // Code to call when a mouse event occurs. }
- See also:
- GuiControl
Member Data Documentation
Whether the control should lock the mouse between up and down button events.