Script::Task Class Reference
#include <task.h>
Inheritance diagram for Script::Task:
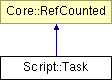
Detailed Description
Represents a task. All tasks without a parentId will be loaded into memory, but the sub tasks will only be loaded for active (unlocked) tasks.(C) 2006 Radon Labs GmbH
Public Types | |
enum | State |
quest states | |
enum | AssertMode |
assert mode for assert method | |
Public Member Functions | |
Task () | |
constructor | |
virtual | ~Task () |
destructor | |
void | Load (const Ptr< Db::Reader > &dbReader) |
initialize quest from database | |
void | Save (const Ptr< Db::Writer > &dbWriter, const Util::String &parentName="") |
save the quest back to the database | |
bool | IsLoaded () const |
return true if quest is loaded | |
void | LoadSubTasks () |
load the quest tasks of this quest from the database | |
bool | HasOpenConditions () const |
return true if task has open conditions | |
const Ptr< Conditions::Condition > & | GetOpenConditions () const |
get the open conditions | |
const Ptr< Conditions::Condition > & | GetCloseConditions () const |
get the close conditions | |
const Ptr< Conditions::Condition > & | GetFailConditions () const |
get fail conditions | |
void | LoadOpenConditions () |
load open conditions | |
void | LoadCloseConditions () |
load close conditions | |
void | LoadFailConditions () |
load fail conditions | |
void | LoadOpenActions () |
load open actions | |
void | LoadCloseActions () |
load close actions | |
void | LoadFailActions () |
load fail actions | |
void | UnloadSubTasks () |
unload the quest tasks of this quest | |
bool | AreSubTasksLoaded () const |
return true if tasks are loaded | |
bool | Assert (AssertMode assertMode) |
asserts open or close conditions or open or close actions | |
bool | EvaluateOpenConditions () |
evaluate the quest task open conditions | |
bool | EvaluateCloseConditions () |
evaluate the quest task closed conditions | |
bool | EvaluateFailConditions () |
evaluate fail conditions | |
void | ExecuteOpenActions () |
execute the open actions | |
void | ExecuteCloseActions () |
execute the close actions | |
void | ExecuteFailActions () |
execute fail actions | |
const Util::Guid & | GetGuid () const |
get the quest's guid | |
const Util::Guid & | GetParentGuid () const |
get parent guid | |
State | GetState () const |
get the current quest state | |
const Util::String & | GetId () const |
get the quest id string | |
const Util::String & | GetTitle () const |
get the title | |
const Util::Array< Ptr< Task > > & | GetSubTasks () const |
get the tasks of the quest | |
int | GetNumSubTasks () const |
get number of subtasks | |
bool | HasSubTasks () const |
has subtasks | |
void | SetOpenText (const Util::String &t) |
set open text | |
const Util::String & | GetOpenText () const |
get open text | |
void | SetCloseText (const Util::String &t) |
set close text | |
const Util::String & | GetCloseText () const |
get close text | |
void | SetFailedText (const Util::String &t) |
set failed text | |
const Util::String & | GetFailedText () const |
get failed text | |
Util::String | GetText () const |
get current state text | |
void | UnlockTask () |
unlock the task | |
void | CloseTask () |
close the task | |
void | FailTask () |
set the task failed | |
void | EvaluateSubTasks () |
evaluate the subtasks | |
bool | HasTaskStatusChanged () const |
checks if task status has changed | |
void | SetTaskStatusUnchanged () |
set task status on unchanged (for updating GUI) | |
const Util::String & | GetTargetEntityName () const |
set task target entity | |
Ptr< Script::Task > | FindSubTaskByGuid (const Util::Guid &guid) |
finds a single task by guid | |
Ptr< Script::Task > | FindSubTaskById (const Util::String &Id) |
finds a single task by Id | |
void | SetParentKey (const Util::String &key) |
set parent key | |
const Util::String & | GetParentKey () const |
get parent key | |
void | SetTaskViewed (bool b) |
set task viewed | |
bool | GetTaskViewed () const |
get task viewed state | |
int | GetRefCount () const |
get the current refcount | |
void | AddRef () |
increment refcount by one | |
void | Release () |
decrement refcount and destroy object if refcount is zero | |
bool | IsInstanceOf (const Rtti &rtti) const |
return true if this object is instance of given class | |
bool | IsInstanceOf (const Util::String &className) const |
return true if this object is instance of given class by string | |
bool | IsInstanceOf (const Util::FourCC &classFourCC) const |
return true if this object is instance of given class by fourcc | |
bool | IsA (const Rtti &rtti) const |
return true if this object is instance of given class, or a derived class | |
bool | IsA (const Util::String &rttiName) const |
return true if this object is instance of given class, or a derived class, by string | |
bool | IsA (const Util::FourCC &rttiFourCC) const |
return true if this object is instance of given class, or a derived class, by fourcc | |
const Util::String & | GetClassName () const |
get the class name | |
Util::FourCC | GetClassFourCC () const |
get the class FourCC code | |
Static Public Member Functions | |
static State | StringToState (const Util::String &s) |
convert string to state | |
static Util::String | StateToString (State s) |
convert state to string | |
static void | DumpRefCountingLeaks () |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!) |
Member Function Documentation
void Script::Task::Load | ( | const Ptr< Db::Reader > & | dbReader | ) |
initialize quest from database
This loads the quest from the database.
void Script::Task::Save | ( | const Ptr< Db::Writer > & | dbWriter, | |
const Util::String & | parentName = "" | |||
) |
save the quest back to the database
Save quest status back to database.
void Script::Task::LoadSubTasks | ( | ) |
load the quest tasks of this quest from the database
Loads all tasks of the quest. This method is usually called automatically as soon as a SetState(Unlocked) is invoked.
void Script::Task::UnloadSubTasks | ( | ) |
unload the quest tasks of this quest
Unloads all tasks of the quest. This method is usually called automatically as soon as a SetState(Closed) is invoked.
bool Script::Task::EvaluateOpenConditions | ( | ) |
evaluate the quest task open conditions
Evaluate open conditions.
bool Script::Task::EvaluateCloseConditions | ( | ) |
evaluate the quest task closed conditions
This evaluates the closed conditions of all open quest tasks. Should only be called when the quest is in the unlocked state. If the ClosedConditions of a task are true, the task will be closed.
bool Script::Task::EvaluateFailConditions | ( | ) |
evaluate fail conditions
This evaluates the closed conditions of all open quest tasks. Should only be called when the quest is in the unlocked state. If the ClosedConditions of a task are true, the task will be closed.
int Script::Task::GetNumSubTasks | ( | ) | const |
get number of subtasks
get the parent guid string
bool Script::Task::HasSubTasks | ( | ) | const |
has subtasks
has subtasks
void Script::Task::CloseTask | ( | ) |
close the task
This is the public method to close the quest. If the quest is not currently in the unlocked state, nothing will happen
void Script::Task::FailTask | ( | ) |
set the task failed
This is the public method to set the task failed. If the task is not currently in the unlocked state, nothing will happen
void Script::Task::EvaluateSubTasks | ( | ) |
evaluate the subtasks
evaluate open condtions of the subtasks, or if they're already open evaluate their subtasks
Ptr< Script::Task > Script::Task::FindSubTaskByGuid | ( | const Util::Guid & | guid | ) |
finds a single task by guid
find a subtask by guid string
Ptr< Script::Task > Script::Task::FindSubTaskById | ( | const Util::String & | id | ) |
finds a single task by Id
find a subtask by Id string
int Core::RefCounted::GetRefCount | ( | ) | const [inline, inherited] |
get the current refcount
Return the current refcount of the object.
void Core::RefCounted::AddRef | ( | ) | [inline, inherited] |
increment refcount by one
Increment the refcount of the object.
void Core::RefCounted::Release | ( | ) | [inline, inherited] |
decrement refcount and destroy object if refcount is zero
Decrement the refcount and destroy object if refcount is zero.
const Util::String & Core::RefCounted::GetClassName | ( | ) | const [inline, inherited] |
get the class name
Get the class name of the object.
Util::FourCC Core::RefCounted::GetClassFourCC | ( | ) | const [inline, inherited] |
get the class FourCC code
Get the class FourCC of the object.
void Core::RefCounted::DumpRefCountingLeaks | ( | ) | [static, inherited] |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!)
This method should be called as the very last before an application exits.