Script::ScriptManager Class Reference
#include <scriptmanager.h>
Inheritance diagram for Script::ScriptManager:
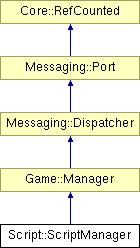
Detailed Description
(C) 2006 Radon Labs GmbH
Public Member Functions | |
ScriptManager () | |
constructor | |
virtual | ~ScriptManager () |
destructor | |
virtual void | OnActivate () |
called when attached to game server | |
virtual void | OnDeactivate () |
called when removed from game server | |
Ptr< Conditions::Condition > | LoadConditionScripts (const Util::Array< Util::String > &scripts, bool combineWithOr=false, const Ptr< Script::InfoLog > &infoLog=0) |
load a list of canscripts and build a condition block | |
Util::Array< Ptr< Actions::Action > > | LoadActionScripts (const Util::Array< Util::String > &scripts, const Ptr< Script::InfoLog > &infoLog=0) |
load a list of actionscripts and build an array of actions | |
Ptr< Actions::ActionList > | LoadStatement (const Util::Guid &blockGuid, const Util::Guid &startId, const Util::String &table="_Scripts_Statements") |
load a statement block | |
Ptr< Conditions::Condition > | LoadCondition (const Util::Guid &blockGuid, const Util::Guid &startId, const Util::String &table="_Scripts_Conditions") |
load condition | |
bool | HasActionScript (const Util::String &script) |
check if action script is available | |
Ptr< Actions::Action > | LoadActionFromScript (const Util::String &scriptName, const Ptr< Script::InfoLog > &infoLog=0) |
load an action from a script (sequence action if needed) | |
Ptr< FSM::StateMachine > | LoadStateMachine (const Util::String &stateMachineName, const Ptr< Game::Entity > &entity) |
load state machine | |
virtual void | ReloadScripts () |
debug helper function to re-initialize scripts | |
bool | IsActive () const |
return true if currently active | |
virtual void | OnBeginFrame () |
called before frame by the game server | |
virtual void | OnFrame () |
called per-frame by the game server | |
virtual void | OnEndFrame () |
called after frame by the game server | |
virtual void | OnLoad () |
called after loading game state | |
virtual void | OnSave () |
called before saving game state | |
virtual void | OnStart () |
called by Game::Server::Start() when the world is started | |
virtual void | OnRenderDebug () |
render a debug visualization | |
virtual void | HandleMessage (const Ptr< Messaging::Message > &msg) |
handle a single message (distribute to ports which accept the message) | |
void | AttachPort (const Ptr< Port > &port) |
attach a message port | |
void | RemovePort (const Ptr< Port > &port) |
remove a message port | |
bool | HasPort (const Ptr< Port > &port) const |
return true if a port exists | |
virtual void | SetupAcceptedMessages () |
override to register accepted messages | |
void | AttachHandler (const Ptr< Handler > &h) |
attach a message handler to the port | |
void | RemoveHandler (const Ptr< Handler > &h) |
remove a message handler from the port | |
void | RemoveAllHandlers () |
remove all message handler from the port | |
SizeT | GetNumHandlers () const |
return number of handlers attached to the port | |
const Ptr< Handler > & | GetHandlerAtIndex (IndexT i) const |
get a message handler by index | |
virtual void | Send (const Ptr< Message > &msg) |
send a message to the port | |
const Util::Array< const Id * > & | GetAcceptedMessages () const |
get the array of accepted messages (sorted) | |
bool | AcceptsMessage (const Id &msgId) const |
return true if port accepts this msg | |
int | GetRefCount () const |
get the current refcount | |
void | AddRef () |
increment refcount by one | |
void | Release () |
decrement refcount and destroy object if refcount is zero | |
bool | IsInstanceOf (const Rtti &rtti) const |
return true if this object is instance of given class | |
bool | IsInstanceOf (const Util::String &className) const |
return true if this object is instance of given class by string | |
bool | IsInstanceOf (const Util::FourCC &classFourCC) const |
return true if this object is instance of given class by fourcc | |
bool | IsA (const Rtti &rtti) const |
return true if this object is instance of given class, or a derived class | |
bool | IsA (const Util::String &rttiName) const |
return true if this object is instance of given class, or a derived class, by string | |
bool | IsA (const Util::FourCC &rttiFourCC) const |
return true if this object is instance of given class, or a derived class, by fourcc | |
const Util::String & | GetClassName () const |
get the class name | |
Util::FourCC | GetClassFourCC () const |
get the class FourCC code | |
Static Public Member Functions | |
static void | DumpRefCountingLeaks () |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!) | |
Protected Member Functions | |
void | LoadCanScript () |
begin a loading sequenz for can scripts, keep dbreader open | |
void | UnloadCanScript () |
end loading sequenz for can scripts, clear dbreader | |
void | LoadOnScript () |
begin a loading sequenz for on scripts, keep dbreader open | |
void | UnloadOnScript () |
end loading sequenz for on scripts, clear dbreader | |
void | LoadStateMachines () |
begin a loading sequenz for can scripts, keep dbreader open | |
void | UnloadStateMachines () |
end loading sequenz for can scripts, clear dbreader | |
void | BuildStateMachineRacks () |
build stateMachineRacks dictionary | |
int | FindGuidIndex (const Util::Dictionary< Util::Guid, int > &statementListIndices, const Util::Guid &guid) |
helper function to find statement with specific guid, return -1 on failure | |
void | LoadTableContent (const Util::Guid &blockGuid, const Util::Guid &startId, const Util::String &table, Util::Array< Statement > &statementList, Util::Dictionary< Util::Guid, int > &statementListIndices) |
load content from a table | |
void | FillActionAndConditionLists (Util::Array< Statement > &statementList, Util::Dictionary< Util::Guid, int > &statementListIndices) |
fill action and condition list | |
void | ConstructContent (Util::Array< Statement > &statementList, Util::Dictionary< Util::Guid, int > &statementListIndices) |
fill action and conditions with content | |
void | LoadStates (Ptr< FSM::StateMachine > stateMachine, IndexT indexRack) |
load state of a state machine | |
void | LoadTransitions (Ptr< FSM::State > state, const Util::String machineName, StateRack indexRack) |
load transitions for a state | |
Ptr< FSM::State > | LoadState (const Util::String &stateMachineName, StateRack rack, IndexT indexRack) |
load a statemachine State | |
Ptr< FSM::Transition > | LoadTransition () |
load a transition | |
void | RegisterMessage (const Id &msgId) |
register a single accepted message |
Member Function Documentation
Ptr< Actions::ActionList > Script::ScriptManager::LoadStatement | ( | const Util::Guid & | blockGuid, | |
const Util::Guid & | startId, | |||
const Util::String & | table = "_Scripts_Statements" | |||
) |
load a statement block
Load statement block from db
Ptr< Conditions::Condition > Script::ScriptManager::LoadCondition | ( | const Util::Guid & | blockGuid, | |
const Util::Guid & | startId, | |||
const Util::String & | table = "_Scripts_Conditions" | |||
) |
load condition
Load condition from db
Ptr< Actions::Action > Script::ScriptManager::LoadActionFromScript | ( | const Util::String & | scriptName, | |
const Ptr< Script::InfoLog > & | infoLog = 0 | |||
) |
load an action from a script (sequence action if needed)
Load an action from a given script name the action is allways a sequence action
void Game::Manager::OnBeginFrame | ( | ) | [virtual, inherited] |
called before frame by the game server
Called before frame, override in subclasses
Reimplemented in BaseGameFeature::EntityManager.
void Game::Manager::OnEndFrame | ( | ) | [virtual, inherited] |
called after frame by the game server
Called after frame, override in subclasses
Reimplemented in BaseGameFeature::EntityManager.
void Messaging::Dispatcher::HandleMessage | ( | const Ptr< Messaging::Message > & | msg | ) | [virtual, inherited] |
handle a single message (distribute to ports which accept the message)
Handle a message. The message will only be distributed to ports which accept the message.
Reimplemented from Messaging::Port.
Reimplemented in Script::DialogManager.
attach a message port
Attach a new message port.
- Parameters:
-
port pointer to a message port object
remove a message port
Remove a message port object.
- Parameters:
-
handler pointer to message port object to be removed
return true if a port exists
Return true if a port is already attached.
attach a message handler to the port
Attach a message handler to the port.
remove a message handler from the port
Remove a message handler from the port.
send a message to the port
Send a message to the port. This will immediately call the HandleMessage() method of all attached handlers. If the message has been handled by at least one of the handlers, the Handled() flag of the message will be set to true.
int Core::RefCounted::GetRefCount | ( | ) | const [inline, inherited] |
get the current refcount
Return the current refcount of the object.
void Core::RefCounted::AddRef | ( | ) | [inline, inherited] |
increment refcount by one
Increment the refcount of the object.
void Core::RefCounted::Release | ( | ) | [inline, inherited] |
decrement refcount and destroy object if refcount is zero
Decrement the refcount and destroy object if refcount is zero.
const Util::String & Core::RefCounted::GetClassName | ( | ) | const [inline, inherited] |
get the class name
Get the class name of the object.
Util::FourCC Core::RefCounted::GetClassFourCC | ( | ) | const [inline, inherited] |
get the class FourCC code
Get the class FourCC of the object.
void Core::RefCounted::DumpRefCountingLeaks | ( | ) | [static, inherited] |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!)
This method should be called as the very last before an application exits.