Models::TransformNode Class Reference
#include <transformnode.h>
Inheritance diagram for Models::TransformNode:
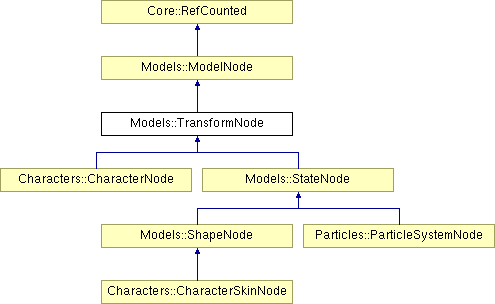
Detailed Description
Defines a transformation in a transform hierarchy.(C) 2007 Radon Labs GmbH
Public Member Functions | |
TransformNode () | |
constructor | |
virtual | ~TransformNode () |
destructor | |
virtual Ptr< ModelNodeInstance > | CreateNodeInstance () const |
create a model node instance | |
virtual bool | ParseDataTag (const Util::FourCC &fourCC, const Ptr< IO::BinaryReader > &reader) |
parse data tag (called by loader code) | |
virtual Resources::Resource::State | GetResourceState () const |
get overall state of contained resources (Initial, Loaded, Pending, Failed, Cancelled) | |
void | SetPosition (const Math::point &p) |
set position | |
const Math::point & | GetPosition () const |
get position | |
void | SetRotation (const Math::quaternion &r) |
set rotate quaternion | |
const Math::quaternion & | GetRotation () const |
get rotate quaternion | |
void | SetScale (const Math::vector &s) |
set scale | |
const Math::vector & | GetScale () const |
get scale | |
void | SetRotatePivot (const Math::point &p) |
set rotate pivot | |
const Math::point & | GetRotatePivot () const |
get rotate pivot | |
void | SetScalePivot (const Math::point &p) |
set scale pivot | |
const Math::point & | GetScalePivot () const |
get scale pivot | |
bool | IsInViewSpace () const |
is transformnode in viewspace | |
void | SetInViewSpace (bool b) |
set transformnode in viewspace | |
float | GetMinDistance () const |
get MinDistance | |
void | SetMinDistance (float val) |
set MinDistance | |
float | GetMaxDistance () const |
get MaxDistance | |
void | SetMaxDistance (float val) |
set MaxDistance | |
bool | LodDistancesUsed () const |
are lod distances used | |
virtual void | OnAttachToModel (const Ptr< Model > &model) |
called when attached to model node | |
bool | GetLockedToViewer () const |
get LockedToViewer | |
void | SetLockedToViewer (bool val) |
set LockedToViewer | |
bool | CheckLodDistance (float distToViewer) const |
helper method to check whether the distance is within lod distances | |
Ptr< ModelNodeInstance > | CreateNodeInstanceHierarchy (const Ptr< ModelInstance > &modelInst) |
recursively create model node instance and child model node instances | |
virtual void | OnRemoveFromModel () |
called when removed from model node | |
virtual void | LoadResources () |
called when resources should be loaded | |
virtual void | UnloadResources () |
called when resources should be unloaded | |
virtual void | OnResourcesLoaded () |
called once when all pending resource have been loaded | |
virtual void | BeginParseDataTags () |
begin parsing data tags | |
virtual void | EndParseDataTags () |
finish parsing data tags | |
virtual void | ApplySharedState (IndexT frameIndex) |
apply state shared by all my ModelNodeInstances | |
bool | IsAttachedToModel () const |
return true if currently attached to a Model | |
const Ptr< Model > & | GetModel () const |
get model this node is attached to | |
void | SetResourceStreamingLevelOfDetail (float factor) |
sets the resourceStreamingLevelOfDetail but only if the given value is bigger than the current one (reseted on frame-start) | |
void | ResetScreenSpaceStats () |
resets all screen space stats e.g. size | |
void | SetBoundingBox (const Math::bbox &b) |
set bounding box | |
const Math::bbox & | GetBoundingBox () const |
get bounding box of model node | |
void | SetName (const Util::StringAtom &n) |
set model node name | |
const Util::StringAtom & | GetName () const |
get model node name | |
void | SetType (ModelNodeType::Code t) |
set ModelNodeType | |
ModelNodeType::Code | GetType () const |
get the ModelNodeType | |
void | SetParent (const Ptr< ModelNode > &p) |
set parent node | |
const Ptr< ModelNode > & | GetParent () const |
get parent node | |
bool | HasParent () const |
return true if node has a parent | |
void | AddChild (const Ptr< ModelNode > &c) |
add a child node | |
const Util::Array< Ptr< ModelNode > > & | GetChildren () const |
get child nodes | |
bool | HasChild (const Util::StringAtom &name) const |
return true if a direct child exists by name | |
const Ptr< ModelNode > & | LookupChild (const Util::StringAtom &name) const |
get pointer to direct child by name | |
void | AddVisibleNodeInstance (IndexT frameIndex, const Ptr< ModelNodeInstance > &nodeInst) |
called by model node instance on NotifyVisible() | |
const Util::Array< Ptr< ModelNodeInstance > > & | GetVisibleModelNodeInstances (ModelNodeType::Code t) const |
get visible model node instances | |
bool | HasStringAttr (const Util::StringAtom &attrId) const |
has string attr | |
const Util::StringAtom & | GetStringAttr (const Util::StringAtom &attrId) const |
get string attr | |
void | SetStringAttr (const Util::StringAtom &attrId, const Util::StringAtom &value) |
add string attribute | |
int | GetRefCount () const |
get the current refcount | |
void | AddRef () |
increment refcount by one | |
void | Release () |
decrement refcount and destroy object if refcount is zero | |
bool | IsInstanceOf (const Rtti &rtti) const |
return true if this object is instance of given class | |
bool | IsInstanceOf (const Util::String &className) const |
return true if this object is instance of given class by string | |
bool | IsInstanceOf (const Util::FourCC &classFourCC) const |
return true if this object is instance of given class by fourcc | |
bool | IsA (const Rtti &rtti) const |
return true if this object is instance of given class, or a derived class | |
bool | IsA (const Util::String &rttiName) const |
return true if this object is instance of given class, or a derived class, by string | |
bool | IsA (const Util::FourCC &rttiFourCC) const |
return true if this object is instance of given class, or a derived class, by fourcc | |
const Util::String & | GetClassName () const |
get the class name | |
Util::FourCC | GetClassFourCC () const |
get the class FourCC code | |
Static Public Member Functions | |
static void | DumpRefCountingLeaks () |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!) | |
Protected Member Functions | |
virtual Ptr< ModelNodeInstance > | RecurseCreateNodeInstanceHierarchy (const Ptr< ModelInstance > &modelInst, const Ptr< ModelNodeInstance > &parentNodeInst) |
recursively create node instance hierarchy | |
Protected Attributes | |
float | resourceStreamingLevelOfDetail |
factor between 0.0 (close) and 1.0 (far away) describing the distance to camera (used for decision of max needed mipMap) |
Member Function Documentation
Ptr< ModelNodeInstance > Models::ModelNode::CreateNodeInstanceHierarchy | ( | const Ptr< ModelInstance > & | modelInst | ) | [inherited] |
recursively create model node instance and child model node instances
Create the node instance hierarchy.
void Models::ModelNode::LoadResources | ( | ) | [virtual, inherited] |
called when resources should be loaded
This method is called when required resources should be loaded.
Reimplemented in Characters::CharacterNode, Models::ShapeNode, Models::StateNode, and Particles::ParticleSystemNode.
void Models::ModelNode::UnloadResources | ( | ) | [virtual, inherited] |
called when resources should be unloaded
This method is called when required resources should be unloaded.
Reimplemented in Characters::CharacterNode, Models::ShapeNode, Models::StateNode, and Particles::ParticleSystemNode.
void Models::ModelNode::OnResourcesLoaded | ( | ) | [virtual, inherited] |
called once when all pending resource have been loaded
This method is called once by Model::OnResourcesLoaded() when all pending resources of a model have been loaded.
Reimplemented in Characters::CharacterNode, and Particles::ParticleSystemNode.
void Models::ModelNode::BeginParseDataTags | ( | ) | [virtual, inherited] |
begin parsing data tags
Begin parsing data tags. This method is called by StreamModelLoader before ParseDataTag() is called for the first time.
void Models::ModelNode::EndParseDataTags | ( | ) | [virtual, inherited] |
finish parsing data tags
End parsing data tags. This method is called by StreamModelLoader after the last ParseDataTag() is called.
void Models::ModelNode::ApplySharedState | ( | IndexT | frameIndex | ) | [virtual, inherited] |
apply state shared by all my ModelNodeInstances
This method is called once before rendering the ModelNode's visible instance nodes through the ModelNodeInstance::ApplyState() and ModelNodeInstance::Render() methods. The method must apply the shader state that is shared across all instances. Since this state is constant across all instance nodes, this only happens once before rendering an instance set.
Reimplemented in Characters::CharacterSkinNode, Models::ShapeNode, and Models::StateNode.
void Models::ModelNode::ResetScreenSpaceStats | ( | ) | [inline, inherited] |
resets all screen space stats e.g. size
Reset resourceStreamingLevelOfDetail to -1.0 as we are able to recognize invisible items this way. (visible items will overwrite this value with a value >= 0.0)
Ptr< ModelNodeInstance > Models::ModelNode::RecurseCreateNodeInstanceHierarchy | ( | const Ptr< ModelInstance > & | modelInst, | |
const Ptr< ModelNodeInstance > & | parentNodeInst | |||
) | [protected, virtual, inherited] |
recursively create node instance hierarchy
Recursively create node instances and attach them to the provided model instance. Returns a pointer to the root node instance.
int Core::RefCounted::GetRefCount | ( | ) | const [inline, inherited] |
get the current refcount
Return the current refcount of the object.
void Core::RefCounted::AddRef | ( | ) | [inline, inherited] |
increment refcount by one
Increment the refcount of the object.
void Core::RefCounted::Release | ( | ) | [inline, inherited] |
decrement refcount and destroy object if refcount is zero
Decrement the refcount and destroy object if refcount is zero.
const Util::String & Core::RefCounted::GetClassName | ( | ) | const [inline, inherited] |
get the class name
Get the class name of the object.
Util::FourCC Core::RefCounted::GetClassFourCC | ( | ) | const [inline, inherited] |
get the class FourCC code
Get the class FourCC of the object.
void Core::RefCounted::DumpRefCountingLeaks | ( | ) | [static, inherited] |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!)
This method should be called as the very last before an application exits.