IO::IoServer Class Reference
#include <ioserver.h>
Inheritance diagram for IO::IoServer:
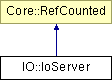
Detailed Description
The central server object of the IO subsystem offers the following services:associate stream classes with URI schemes create the right stream object for a given URI transparant (ZIP) archive support path assign management global filesystem manipulation and query methods
(C) 2006 Radon Labs GmbH
Public Member Functions | |
IoServer () | |
constructor | |
virtual | ~IoServer () |
destructor | |
bool | MountArchive (const URI &uri) |
mount a file archive (without archive file extension!) | |
void | UnmountArchive (const URI &uri) |
unmount a file archive (without archive file extension!) | |
bool | IsArchiveMounted (const URI &uri) const |
return true if a archive is mounted (without archive file extension!) | |
void | SetArchiveFileSystemEnabled (bool b) |
enable/disable transparent archive filesystem layering (default is yes) | |
bool | IsArchiveFileSystemEnabled () const |
return true if transparent archive filesystem is enabled | |
void | MountStandardArchives () |
mount standard archives (e.g. home:export.zip and home:export_.zip) | |
void | UnmountStandardArchives () |
unmount standard archives | |
Ptr< Stream > | CreateStream (const URI &uri) const |
create a stream object for the given uri | |
bool | CreateDirectory (const URI &uri) const |
create all missing directories in the path | |
bool | DeleteDirectory (const URI &path) const |
delete an empty directory | |
bool | DirectoryExists (const URI &path) const |
return true if directory exists | |
bool | CopyFile (const URI &from, const URI &to) const |
copy a file | |
bool | DeleteFile (const URI &path) const |
delete a file | |
bool | FileExists (const URI &path) const |
return true if file exists | |
void | SetReadOnly (const URI &path, bool b) const |
set the readonly status of a file | |
bool | IsReadOnly (const URI &path) const |
return read only status of a file | |
unsigned int | ComputeFileCrc (const URI &path) const |
get the CRC checksum of a file | |
void | SetFileWriteTime (const URI &path, FileTime fileTime) |
set the write-time of a file | |
FileTime | GetFileWriteTime (const URI &path) const |
return the last write-time of a file | |
Util::Array< Util::String > | ListFiles (const URI &dir, const Util::String &pattern, bool asFullPath=false) const |
list all files matching a pattern in a directory | |
Util::Array< Util::String > | ListDirectories (const URI &dir, const Util::String &pattern, bool asFullPath=false) const |
list all subdirectories matching a pattern in a directory | |
int | GetRefCount () const |
get the current refcount | |
void | AddRef () |
increment refcount by one | |
void | Release () |
decrement refcount and destroy object if refcount is zero | |
bool | IsInstanceOf (const Rtti &rtti) const |
return true if this object is instance of given class | |
bool | IsInstanceOf (const Util::String &className) const |
return true if this object is instance of given class by string | |
bool | IsInstanceOf (const Util::FourCC &classFourCC) const |
return true if this object is instance of given class by fourcc | |
bool | IsA (const Rtti &rtti) const |
return true if this object is instance of given class, or a derived class | |
bool | IsA (const Util::String &rttiName) const |
return true if this object is instance of given class, or a derived class, by string | |
bool | IsA (const Util::FourCC &rttiFourCC) const |
return true if this object is instance of given class, or a derived class, by fourcc | |
const Util::String & | GetClassName () const |
get the class name | |
Util::FourCC | GetClassFourCC () const |
get the class FourCC code | |
Static Public Member Functions | |
static void | DumpRefCountingLeaks () |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!) |
Member Function Documentation
void IO::IoServer::SetArchiveFileSystemEnabled | ( | bool | b | ) | [inline] |
enable/disable transparent archive filesystem layering (default is yes)
NOTE: on platforms which provide transparent archive access this method is point less (the archiveFileSystemEnabled flag will be ignored, and IsArchiveFileSystemEnabled() will always return false).
bool IO::IoServer::IsArchiveFileSystemEnabled | ( | ) | const [inline] |
return true if transparent archive filesystem is enabled
NOTE: on platforms which provide transparent archive access through the OS (like on PS3) this method will always return false. This saves some unecessary overhead in the Nebula3 IoServer.
bool IO::IoServer::CreateDirectory | ( | const URI & | uri | ) | const |
create all missing directories in the path
This method creates all missing directories in a path.
copy a file
This copies a file to another file.
unsigned int IO::IoServer::ComputeFileCrc | ( | const URI & | uri | ) | const |
get the CRC checksum of a file
This method computes the CRC checksum for a file.
int Core::RefCounted::GetRefCount | ( | ) | const [inline, inherited] |
get the current refcount
Return the current refcount of the object.
void Core::RefCounted::AddRef | ( | ) | [inline, inherited] |
increment refcount by one
Increment the refcount of the object.
void Core::RefCounted::Release | ( | ) | [inline, inherited] |
decrement refcount and destroy object if refcount is zero
Decrement the refcount and destroy object if refcount is zero.
const Util::String & Core::RefCounted::GetClassName | ( | ) | const [inline, inherited] |
get the class name
Get the class name of the object.
Util::FourCC Core::RefCounted::GetClassFourCC | ( | ) | const [inline, inherited] |
get the class FourCC code
Get the class FourCC of the object.
void Core::RefCounted::DumpRefCountingLeaks | ( | ) | [static, inherited] |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!)
This method should be called as the very last before an application exits.