GraphicsFeature::AnimationControlProperty Class Reference
#include <animationcontrolproperty.h>
Inheritance diagram for GraphicsFeature::AnimationControlProperty:
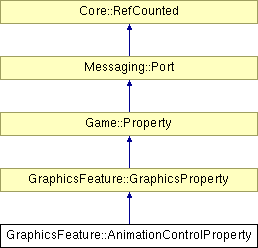
Detailed Description
Graphics property for animated characters.(C) 2008 Radon Labs GmbH
Public Types | |
enum | CallbackType |
callback types | |
Public Member Functions | |
virtual void | SetupDefaultAttributes () |
setup default entity attributes | |
virtual void | SetupAcceptedMessages () |
override to register accepted messages | |
virtual void | HandleMessage (const Ptr< Messaging::Message > &msg) |
handle a single message | |
virtual void | OnActivate () |
called from Entity::ActivateProperties() | |
virtual void | OnDeactivate () |
called from Entity::DeactivateProperties() | |
virtual void | SetupCallbacks () |
setup callbacks for this property, call by entity in OnActivate() | |
virtual const Util::String & | GetGraphicsResource () const |
override to provide a self managed graphics resource (default is Attr::Graphics) | |
virtual void | OnRenderDebug () |
called when game debug visualization is on | |
const Ptr< Entity > & | GetEntity () const |
get entity this property is attached to | |
bool | HasEntity () const |
return true if entity pointer is valid | |
bool | IsActive () const |
return true if property is currently active | |
virtual void | OnLoad () |
called from within Entity::Load() after attributes are loaded | |
virtual void | OnStart () |
called from within Entity::OnStart() after OnLoad when the complete world exist | |
virtual void | OnSave () |
called from within Entity::Save() before attributes are saved back to database | |
virtual void | OnBeginFrame () |
called on begin of frame | |
virtual void | OnMoveBefore () |
called before movement happens | |
virtual void | OnMoveAfter () |
called after movement has happened | |
virtual void | OnRender () |
called before rendering happens | |
virtual void | OnLoseActivity () |
called when game debug visualization is on | |
virtual void | OnGainActivity () |
called when game debug visualization is on | |
void | AttachHandler (const Ptr< Handler > &h) |
attach a message handler to the port | |
void | RemoveHandler (const Ptr< Handler > &h) |
remove a message handler from the port | |
void | RemoveAllHandlers () |
remove all message handler from the port | |
SizeT | GetNumHandlers () const |
return number of handlers attached to the port | |
const Ptr< Handler > & | GetHandlerAtIndex (IndexT i) const |
get a message handler by index | |
virtual void | Send (const Ptr< Message > &msg) |
send a message to the port | |
const Util::Array< const Id * > & | GetAcceptedMessages () const |
get the array of accepted messages (sorted) | |
bool | AcceptsMessage (const Id &msgId) const |
return true if port accepts this msg | |
int | GetRefCount () const |
get the current refcount | |
void | AddRef () |
increment refcount by one | |
void | Release () |
decrement refcount and destroy object if refcount is zero | |
bool | IsInstanceOf (const Rtti &rtti) const |
return true if this object is instance of given class | |
bool | IsInstanceOf (const Util::String &className) const |
return true if this object is instance of given class by string | |
bool | IsInstanceOf (const Util::FourCC &classFourCC) const |
return true if this object is instance of given class by fourcc | |
bool | IsA (const Rtti &rtti) const |
return true if this object is instance of given class, or a derived class | |
bool | IsA (const Util::String &rttiName) const |
return true if this object is instance of given class, or a derived class, by string | |
bool | IsA (const Util::FourCC &rttiFourCC) const |
return true if this object is instance of given class, or a derived class, by fourcc | |
const Util::String & | GetClassName () const |
get the class name | |
Util::FourCC | GetClassFourCC () const |
get the class FourCC code | |
Static Public Member Functions | |
static void | DumpRefCountingLeaks () |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!) | |
Protected Member Functions | |
virtual void | SetupGraphicsEntities () |
setup graphics entities | |
virtual void | UpdateTransform (const Math::matrix44 &m, bool setDirectly=false) |
update the graphics entity's transform | |
void | SetVisible (bool visible) |
void | OnSetOverwriteColor (const Ptr< SetOverwriteColor > &msg) |
on set overwrite color | |
void | OnSetShaderVariable (const Ptr< SetShaderVariable > &msg) |
set shader variable | |
void | SetEntity (const Ptr< Entity > &v) |
Set entity, this is attached to, to `v'. | |
void | ClearEntity () |
Remove entity. | |
void | RegisterMessage (const Id &msgId) |
register a single accepted message |
Member Function Documentation
void GraphicsFeature::GraphicsProperty::OnActivate | ( | ) | [virtual, inherited] |
called from Entity::ActivateProperties()
Attach the property to a game entity. This will create and setup the required graphics entities.
Reimplemented from Game::Property.
Reimplemented in StateObjectFeature::StateGraphicsProperty.
void GraphicsFeature::GraphicsProperty::OnDeactivate | ( | ) | [virtual, inherited] |
called from Entity::DeactivateProperties()
Remove the property from its game entity. This will release the graphics entities owned by the property.
Reimplemented from Game::Property.
Reimplemented in StateObjectFeature::StateGraphicsProperty.
const Util::String & GraphicsFeature::GraphicsProperty::GetGraphicsResource | ( | ) | const [inline, virtual, inherited] |
override to provide a self managed graphics resource (default is Attr::Graphics)
Get the default graphics resource, which is Attr::Graphics. subclasses may override this to provide a self managed resource.
void GraphicsFeature::GraphicsProperty::SetupGraphicsEntities | ( | ) | [protected, virtual, inherited] |
setup graphics entities
Setup the graphics entities. You may override this method in a subclass if different setup is needed.
Reimplemented in GraphicsFeature::ActorGraphicsProperty.
void GraphicsFeature::GraphicsProperty::UpdateTransform | ( | const Math::matrix44 & | m, | |
bool | setDirectly = false | |||
) | [protected, virtual, inherited] |
update the graphics entity's transform
Called to update the graphics entity's transform.
void GraphicsFeature::GraphicsProperty::SetVisible | ( | bool | visible | ) | [protected, inherited] |
Shows or hides all attached graphics entities.
void Game::Property::OnLoad | ( | ) | [virtual, inherited] |
called from within Entity::Load() after attributes are loaded
This method is called from within Game::Entity::Load() after the entity attributes have been loaded from the database. You can override this method in a subclass if further initialization is needed for the property after attributes have been loaded from the database, but please be aware that this method may not be called if the entity is created directly.
Reimplemented in PhysicsFeature::TriggerProperty, and StateObjectFeature::StateProperty.
void Game::Property::OnStart | ( | ) | [virtual, inherited] |
called from within Entity::OnStart() after OnLoad when the complete world exist
This method is called from within Game::Entity::OnStart(). This is the moment when the world is complete and the entity can establish connections to other entitys.
Reimplemented in GraphicsFeature::CameraProperty.
void Game::Property::OnSave | ( | ) | [virtual, inherited] |
called from within Entity::Save() before attributes are saved back to database
This method is called from within Game::Entity::Save() before the entity attributes will be saved to the database. You can override this method in a subclass if actions are needed before a save happens (this is usually the case if entity attributes need to be updated by the property before saving).
Reimplemented in PhysicsFeature::TriggerProperty, and StateObjectFeature::StateProperty.
void Game::Property::OnBeginFrame | ( | ) | [virtual, inherited] |
called on begin of frame
This method is called from Game::Entity::OnBeginFrame() on all properties attached to an entity in the order of attachment. Override this method if your property has to do any work at the beginning of the frame.
Reimplemented in PhysicsFeature::TriggerProperty, and StateObjectFeature::StateProperty.
void Game::Property::OnMoveBefore | ( | ) | [virtual, inherited] |
called before movement happens
This method is called from Game::Entity::OnMoveBefore() on all properties attached to an entity in the order of attachment. Override this method if your property has any work to do before the physics subsystem is triggered.
Reimplemented in PhysicsFeature::ActorPhysicsProperty.
void Game::Property::OnMoveAfter | ( | ) | [virtual, inherited] |
called after movement has happened
This method is called from Game::Entity::OnMoveAfter() on all properties attached to an entity in the order of attachment. Override this method if your property has any work to do after the physics subsystem has been triggered.
Reimplemented in PhysicsFeature::ActorPhysicsProperty, and PhysicsFeature::PhysicsProperty.
void Game::Property::OnRender | ( | ) | [virtual, inherited] |
called before rendering happens
This method is called from Game::Entity::OnRender() on all properties attached to an entity in the order of attachment. Override this method if your property has any work to do before rendering happens.
Reimplemented in GraphicsFeature::CameraProperty, GraphicsFeature::ChaseCameraProperty, and GraphicsFeature::MayaCameraProperty.
void Game::Property::OnLoseActivity | ( | ) | [virtual, inherited] |
called when game debug visualization is on
This method is called from Game::Entity::OnLoseActivity() on all properties attached to an entity in the order of attachment. It indicates that the entity will no longer be trigger, due to leaving the "Activity Bubble", i.e. the area of interest (most probably around the active camera).
Reimplemented in PhysicsFeature::ActorPhysicsProperty.
void Game::Property::OnGainActivity | ( | ) | [virtual, inherited] |
called when game debug visualization is on
This method is called from Game::Entity::OnRenderDebug() on all properties attached to an entity in the order of attachment. It indicates that the entity will be trigger from now on, due to entering the "Activity Bubble", i.e. the area of interest (most probably around the active camera).
attach a message handler to the port
Attach a message handler to the port.
remove a message handler from the port
Remove a message handler from the port.
send a message to the port
Send a message to the port. This will immediately call the HandleMessage() method of all attached handlers. If the message has been handled by at least one of the handlers, the Handled() flag of the message will be set to true.
int Core::RefCounted::GetRefCount | ( | ) | const [inline, inherited] |
get the current refcount
Return the current refcount of the object.
void Core::RefCounted::AddRef | ( | ) | [inline, inherited] |
increment refcount by one
Increment the refcount of the object.
void Core::RefCounted::Release | ( | ) | [inline, inherited] |
decrement refcount and destroy object if refcount is zero
Decrement the refcount and destroy object if refcount is zero.
const Util::String & Core::RefCounted::GetClassName | ( | ) | const [inline, inherited] |
get the class name
Get the class name of the object.
Util::FourCC Core::RefCounted::GetClassFourCC | ( | ) | const [inline, inherited] |
get the class FourCC code
Get the class FourCC of the object.
void Core::RefCounted::DumpRefCountingLeaks | ( | ) | [static, inherited] |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!)
This method should be called as the very last before an application exits.