Direct3D9::D3D9RenderDevice Class Reference
#include <d3d9renderdevice.h>
Inheritance diagram for Direct3D9::D3D9RenderDevice:
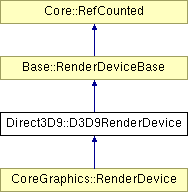
Detailed Description
Implements a RenderDevice on top of Direct3D9.(C) 2007 Radon Labs GmbH
Public Member Functions | |
D3D9RenderDevice () | |
constructor | |
virtual | ~D3D9RenderDevice () |
destructor | |
IDirect3DDevice9 * | GetDirect3DDevice () const |
get pointer to the d3d device | |
bool | Open () |
open the device | |
void | Close () |
close the device | |
bool | BeginFrame () |
begin complete frame | |
void | SetStreamSource (IndexT streamIndex, const Ptr< CoreGraphics::VertexBuffer > &vb, IndexT offsetVertexIndex) |
set the current vertex stream source | |
void | SetVertexLayout (const Ptr< CoreGraphics::VertexLayout > &vl) |
set current vertex layout | |
void | SetIndexBuffer (const Ptr< CoreGraphics::IndexBuffer > &ib) |
set current index buffer | |
void | Draw () |
draw current primitives | |
void | DrawIndexedInstanced (SizeT numInstances) |
draw indexed, instanced primitives (see method header for details) | |
void | EndPass () |
end current pass | |
void | EndFrame () |
end complete frame | |
void | Present () |
present the rendered scene | |
CoreGraphics::ImageFileFormat::Code | SaveScreenshot (CoreGraphics::ImageFileFormat::Code fmt, const Ptr< IO::Stream > &outStream) |
save a screenshot to the provided stream | |
D3DPRESENT_PARAMETERS | GetPresentParams () const |
get the present parameters | |
void | SetOverrideDefaultRenderTarget (const Ptr< CoreGraphics::RenderTarget > &rt) |
set an override for the default render target (call before Open()!) | |
bool | IsOpen () const |
return true if currently open | |
void | AttachEventHandler (const Ptr< CoreGraphics::RenderEventHandler > &h) |
attach a render event handler | |
void | RemoveEventHandler (const Ptr< CoreGraphics::RenderEventHandler > &h) |
remove a render event handler | |
const Ptr< CoreGraphics::RenderTarget > & | GetDefaultRenderTarget () const |
get default render target | |
bool | HasPassRenderTarget () const |
is a pass rendertarget set? | |
const Ptr< CoreGraphics::RenderTarget > & | GetPassRenderTarget () const |
get current set pass render target | |
void | BeginPass (const Ptr< CoreGraphics::RenderTarget > &rt, const Ptr< CoreGraphics::ShaderInstance > &passShader) |
begin rendering a frame pass | |
void | BeginPass (const Ptr< CoreGraphics::MultipleRenderTarget > &mrt, const Ptr< CoreGraphics::ShaderInstance > &passShader) |
begin rendering a frame pass with a multiple rendertarget | |
void | BeginBatch (CoreGraphics::BatchType::Code batchType, const Ptr< CoreGraphics::ShaderInstance > &batchShader) |
begin rendering a batch inside | |
const Ptr< CoreGraphics::VertexBuffer > & | GetStreamVertexBuffer (IndexT streamIndex) const |
get currently set vertex buffer | |
IndexT | GetStreamVertexOffset (IndexT streamIndex) const |
get currently set vertex stream offset | |
const Ptr< CoreGraphics::VertexLayout > & | GetVertexLayout () const |
get current vertex layout | |
const Ptr< CoreGraphics::IndexBuffer > & | GetIndexBuffer () const |
get current index buffer | |
void | SetPrimitiveGroup (const CoreGraphics::PrimitiveGroup &pg) |
set current primitive group | |
const CoreGraphics::PrimitiveGroup & | GetPrimitiveGroup () const |
get current primitive group | |
void | EndBatch () |
end current batch | |
bool | IsInBeginFrame () const |
check if inside BeginFrame | |
bool | GetVisualizeMipMaps () const |
get visualization of mipmaps flag | |
void | SetVisualizeMipMaps (bool val) |
set visualization of mipmaps flag | |
int | GetRefCount () const |
get the current refcount | |
void | AddRef () |
increment refcount by one | |
void | Release () |
decrement refcount and destroy object if refcount is zero | |
bool | IsInstanceOf (const Rtti &rtti) const |
return true if this object is instance of given class | |
bool | IsInstanceOf (const Util::String &className) const |
return true if this object is instance of given class by string | |
bool | IsInstanceOf (const Util::FourCC &classFourCC) const |
return true if this object is instance of given class by fourcc | |
bool | IsA (const Rtti &rtti) const |
return true if this object is instance of given class, or a derived class | |
bool | IsA (const Util::String &rttiName) const |
return true if this object is instance of given class, or a derived class, by string | |
bool | IsA (const Util::FourCC &rttiFourCC) const |
return true if this object is instance of given class, or a derived class, by fourcc | |
const Util::String & | GetClassName () const |
get the class name | |
Util::FourCC | GetClassFourCC () const |
get the class FourCC code | |
Static Public Member Functions | |
static bool | CanCreate () |
test if a compatible render device can be created on this machine | |
static IDirect3D9 * | GetDirect3D () |
get pointer to Direct3D interface, opens Direct3D if not happened yet | |
static void | DumpRefCountingLeaks () |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!) | |
Static Public Attributes | |
static const IndexT | MaxNumVertexStreams = 2 |
max number of vertex streams | |
Protected Member Functions | |
bool | NotifyEventHandlers (const CoreGraphics::RenderEvent &e) |
notify event handlers about an event |
Member Function Documentation
bool Direct3D9::D3D9RenderDevice::CanCreate | ( | ) | [static] |
test if a compatible render device can be created on this machine
Test if the right Direct3D version is installed by trying to open Direct3D.
Reimplemented from Base::RenderDeviceBase.
IDirect3D9 * Direct3D9::D3D9RenderDevice::GetDirect3D | ( | ) | [static] |
get pointer to Direct3D interface, opens Direct3D if not happened yet
Get a pointer to the Direct3D interface. Opens Direct3D if not happened yet.
IDirect3DDevice9 * Direct3D9::D3D9RenderDevice::GetDirect3DDevice | ( | ) | const |
get pointer to the d3d device
Return a pointer to d3d device. Asserts that the device exists.
bool Direct3D9::D3D9RenderDevice::Open | ( | ) |
open the device
Open the render device. When successful, the RenderEvent::DeviceOpen will be sent to all registered event handlers after the Direct3D device has been opened.
Reimplemented from Base::RenderDeviceBase.
void Direct3D9::D3D9RenderDevice::Close | ( | ) |
close the device
Close the render device. The RenderEvent::DeviceClose will be sent to all registered event handlers.
Reimplemented from Base::RenderDeviceBase.
bool Direct3D9::D3D9RenderDevice::BeginFrame | ( | ) |
begin complete frame
Begin a complete frame. Call this once per frame before any rendering happens. If rendering is not possible for some reason (e.g. a lost device) the method will return false. This method may also send the DeviceLost and DeviceRestored RenderEvents to attached event handlers.
Reimplemented from Base::RenderDeviceBase.
void Direct3D9::D3D9RenderDevice::SetStreamSource | ( | IndexT | streamIndex, | |
const Ptr< CoreGraphics::VertexBuffer > & | vb, | |||
IndexT | offsetVertexIndex | |||
) |
set the current vertex stream source
Sets the vertex buffer to use for the next Draw().
Reimplemented from Base::RenderDeviceBase.
void Direct3D9::D3D9RenderDevice::SetVertexLayout | ( | const Ptr< CoreGraphics::VertexLayout > & | vl | ) |
set current vertex layout
Sets the vertex layout for the next Draw()
Reimplemented from Base::RenderDeviceBase.
void Direct3D9::D3D9RenderDevice::SetIndexBuffer | ( | const Ptr< CoreGraphics::IndexBuffer > & | ib | ) |
set current index buffer
Sets the index buffer to use for the next Draw().
Reimplemented from Base::RenderDeviceBase.
void Direct3D9::D3D9RenderDevice::Draw | ( | ) |
draw current primitives
Draw the current primitive group. Requires a vertex buffer, an optional index buffer and a primitive group to be set through the respective methods. To use non-indexed rendering, set the number of indices in the primitive group to 0.
Reimplemented from Base::RenderDeviceBase.
void Direct3D9::D3D9RenderDevice::DrawIndexedInstanced | ( | SizeT | numInstances | ) |
draw indexed, instanced primitives (see method header for details)
Draw N instances of the current primitive group. Requires the following setup:
- vertex stream 0: vertex buffer with instancing data, one vertex per instance
- vertex stream 1: vertex buffer with instance geometry data
- index buffer: index buffer for geometry data
- primitive group: the primitive group which describes one instance
- vertex declaration: describes a combined vertex from stream 0 and stream 1
Reimplemented from Base::RenderDeviceBase.
void Direct3D9::D3D9RenderDevice::EndPass | ( | ) |
end current pass
End the current rendering pass. This will flush all texture stages in order to keep the d3d9 resource reference counts consistent without too much hassle.
Reimplemented from Base::RenderDeviceBase.
void Direct3D9::D3D9RenderDevice::EndFrame | ( | ) |
end complete frame
End a complete frame. Call this once per frame after rendering has happened and before Present(), and only if BeginFrame() returns true.
Reimplemented from Base::RenderDeviceBase.
void Direct3D9::D3D9RenderDevice::Present | ( | ) |
present the rendered scene
NOTE: Present() should be called as late as possible after EndFrame() to improve parallelism between the GPU and the CPU.
Reimplemented from Base::RenderDeviceBase.
ImageFileFormat::Code Direct3D9::D3D9RenderDevice::SaveScreenshot | ( | CoreGraphics::ImageFileFormat::Code | fmt, | |
const Ptr< IO::Stream > & | outStream | |||
) |
save a screenshot to the provided stream
Save the backbuffer to the provided stream.
Reimplemented from Base::RenderDeviceBase.
void Base::RenderDeviceBase::SetOverrideDefaultRenderTarget | ( | const Ptr< CoreGraphics::RenderTarget > & | rt | ) | [inherited] |
void Base::RenderDeviceBase::AttachEventHandler | ( | const Ptr< CoreGraphics::RenderEventHandler > & | h | ) | [inherited] |
attach a render event handler
Attach an event handler to the render device.
void Base::RenderDeviceBase::RemoveEventHandler | ( | const Ptr< CoreGraphics::RenderEventHandler > & | h | ) | [inherited] |
remove a render event handler
Remove an event handler from the display device.
bool Base::RenderDeviceBase::NotifyEventHandlers | ( | const CoreGraphics::RenderEvent & | e | ) | [protected, inherited] |
notify event handlers about an event
Notify all event handlers about an event.
int Core::RefCounted::GetRefCount | ( | ) | const [inline, inherited] |
get the current refcount
Return the current refcount of the object.
void Core::RefCounted::AddRef | ( | ) | [inline, inherited] |
increment refcount by one
Increment the refcount of the object.
void Core::RefCounted::Release | ( | ) | [inline, inherited] |
decrement refcount and destroy object if refcount is zero
Decrement the refcount and destroy object if refcount is zero.
const Util::String & Core::RefCounted::GetClassName | ( | ) | const [inline, inherited] |
get the class name
Get the class name of the object.
Util::FourCC Core::RefCounted::GetClassFourCC | ( | ) | const [inline, inherited] |
get the class FourCC code
Get the class FourCC of the object.
void Core::RefCounted::DumpRefCountingLeaks | ( | ) | [static, inherited] |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!)
This method should be called as the very last before an application exits.