Graphics::ModelEntity Class Reference
#include <modelentity.h>
Inheritance diagram for Graphics::ModelEntity:
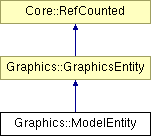
Detailed Description
Client-side proxy of a InternalGraphics::InternalModelEntity.(C) 2008 Radon Labs GmbH
Public Member Functions | |
ModelEntity () | |
constructor | |
virtual | ~ModelEntity () |
destructor | |
void | SetResourceId (const Resources::ResourceId &resId) |
set the model's resource id | |
const Resources::ResourceId & | GetResourceId () const |
get the model's resource id | |
void | SetRootNodePath (const Util::StringAtom &rootNodePath) |
set optional root node path (allows to setup ModelEntity from a child node in a model node hierarchy) | |
const Util::StringAtom & | GetRootNodePath () const |
get optional root node path | |
void | SetRootNodeOffsetMatrix (const Math::matrix44 &offsetMatrix) |
set optional root node offset matrix | |
const Math::matrix44 & | GetRootNodeOffsetMatrix () const |
get optional root node offset matrix | |
void | ConfigureAnimDrivenMotionTracking (bool enabled, const Util::StringAtom &jointName) |
enable anim driven motion tracking | |
void | ConfigureAnimEventTracking (bool enabled, bool onlyDominatingClip) |
enable anim event tracking | |
void | ConfigureCharJointTracking (bool enabled, const Util::Array< Util::StringAtom > &jointNames) |
enable joint tracking | |
bool | IsAnimDrivenMotionTrackingEnabled () const |
return true if anim driven motion tracking is enabled | |
const Util::StringAtom & | GetAnimDrivenMotionJointName () const |
get the anim-driven-motion tracking joint name | |
const Math::vector & | GetAnimDrivenMotionVector () const |
get the current anim-driven-motion tracking vector | |
bool | IsAnimEventTrackingEnabled () const |
return true if anim event tracking is enabled | |
const Util::Array< Animation::AnimEventInfo > & | GetAnimEvents () const |
return the array of anim events of the current frame | |
bool | IsCharJointTrackingEnabled () const |
return true if char joint tracking is enabled | |
bool | IsCharJointDataValid () const |
return true if current char joint data is valid in this frame (may change by visibility) | |
void | AddTrackedCharJoint (const Util::StringAtom &jointName) |
dynamically add a tracked character joint | |
const Shared::CharJointInfo * | GetTrackedCharJointInfo (const Util::StringAtom &jointName) const |
access tracked char joint data, return NULL ptr if joint data not valid or not yet available! | |
void | UpdateModelNodeInstanceShaderVariable (const Util::StringAtom &nodeName, const Util::StringAtom &variableSemantic, const Util::Variant &value) |
update the value of a shader variable on a node instance of the model entity | |
void | UpdateModelNodeInstanceVisibility (const Util::StringAtom &nodeName, bool visible) |
update the visibility of a model node instance node | |
bool | IsValid () const |
return true if entity is valid (is attached to a stage) | |
GraphicsEntityType::Code | GetType () const |
get the entity type | |
void | SetTransform (const Math::matrix44 &m) |
set the entity's world space transform | |
const Math::matrix44 & | GetTransform () const |
get the entity's world space transform | |
void | SetVisible (bool b) |
set the entity's visibility | |
bool | IsVisible () const |
return true if entity is set to visible | |
const Ptr< Stage > & | GetStage () const |
get the stage proxy this entity is attached to | |
Math::ClipStatus::Type | GetClipStatus () const |
get current clip status | |
const Math::bbox & | GetLocalBoundingBox () const |
get the local bounding box | |
const Math::bbox & | GetGlobalBoundingBox () const |
get the global bounding box | |
Timing::Time | GetEntityTime () const |
get current entity time | |
void | SendMsg (const Ptr< GraphicsEntityMessage > &msg) |
send a message to the server-side graphics entity | |
bool | IsObjectRefValid () const |
test if the entity's object ref is valid | |
const Ptr< Threading::ObjectRef > & | GetObjectRef () const |
get the entity handle | |
int | GetRefCount () const |
get the current refcount | |
void | AddRef () |
increment refcount by one | |
void | Release () |
decrement refcount and destroy object if refcount is zero | |
bool | IsInstanceOf (const Rtti &rtti) const |
return true if this object is instance of given class | |
bool | IsInstanceOf (const Util::String &className) const |
return true if this object is instance of given class by string | |
bool | IsInstanceOf (const Util::FourCC &classFourCC) const |
return true if this object is instance of given class by fourcc | |
bool | IsA (const Rtti &rtti) const |
return true if this object is instance of given class, or a derived class | |
bool | IsA (const Util::String &rttiName) const |
return true if this object is instance of given class, or a derived class, by string | |
bool | IsA (const Util::FourCC &rttiFourCC) const |
return true if this object is instance of given class, or a derived class, by fourcc | |
const Util::String & | GetClassName () const |
get the class name | |
Util::FourCC | GetClassFourCC () const |
get the class FourCC code | |
Static Public Member Functions | |
static void | DumpRefCountingLeaks () |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!) | |
Protected Member Functions | |
void | SetType (GraphicsEntityType::Code t) |
set graphics entity type, called from constructor of subclass | |
virtual void | Discard () |
called by stage when entity should discard itself | |
void | SendCreateMsg (const Ptr< CreateGraphicsEntity > &msg) |
send off a specific create message from the subclass | |
virtual void | OnTransformChanged () |
called when transform matrix changed |
Member Function Documentation
const Shared::CharJointInfo * Graphics::ModelEntity::GetTrackedCharJointInfo | ( | const Util::StringAtom & | jointName | ) | const |
access tracked char joint data, return NULL ptr if joint data not valid or not yet available!
NOTE: the method returns 0 if the joint is not valid or has not been found!
void Graphics::GraphicsEntity::SendMsg | ( | const Ptr< GraphicsEntityMessage > & | msg | ) | [inherited] |
send a message to the server-side graphics entity
Send a generic GraphicsEntityMessage to the server-side entity.
void Graphics::GraphicsEntity::Discard | ( | ) | [protected, virtual, inherited] |
called by stage when entity should discard itself
Discard the server-side graphics entity. This method is called from StageProxy::RemoveEntityProxy(). There's special handling if the server-side entity hasn't been created yet, in this case, a pointer to the original create message must be handed over to the render thread.
Reimplemented in Graphics::CameraEntity.
void Graphics::GraphicsEntity::SendCreateMsg | ( | const Ptr< CreateGraphicsEntity > & | msg | ) | [protected, inherited] |
send off a specific create message from the subclass
This method must be called from the Setup() method of a subclass to send off a specific creation message. The message will be stored in the proxy to get the entity handle back later when the server-side graphics entity has been created.
void Graphics::GraphicsEntity::OnTransformChanged | ( | ) | [protected, virtual, inherited] |
called when transform matrix changed
Called by SetTransform(). This gives subclasses a chance to react to changes on the transformation matrix.
Reimplemented in Graphics::CameraEntity.
int Core::RefCounted::GetRefCount | ( | ) | const [inline, inherited] |
get the current refcount
Return the current refcount of the object.
void Core::RefCounted::AddRef | ( | ) | [inline, inherited] |
increment refcount by one
Increment the refcount of the object.
void Core::RefCounted::Release | ( | ) | [inline, inherited] |
decrement refcount and destroy object if refcount is zero
Decrement the refcount and destroy object if refcount is zero.
const Util::String & Core::RefCounted::GetClassName | ( | ) | const [inline, inherited] |
get the class name
Get the class name of the object.
Util::FourCC Core::RefCounted::GetClassFourCC | ( | ) | const [inline, inherited] |
get the class FourCC code
Get the class FourCC of the object.
void Core::RefCounted::DumpRefCountingLeaks | ( | ) | [static, inherited] |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!)
This method should be called as the very last before an application exits.