IO::XmlWriter Class Reference
#include <xmlwriter.h>
Inheritance diagram for IO::XmlWriter:
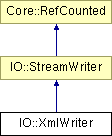
Detailed Description
Write XML-formatted data to a stream.(C) 2006 Radon Labs GmbH
Public Member Functions | |
XmlWriter () | |
constructor | |
virtual | ~XmlWriter () |
destructor | |
virtual bool | Open () |
begin writing the stream | |
virtual void | Close () |
end writing the stream | |
bool | BeginNode (const Util::String &nodeName) |
begin a new node under the current node | |
void | EndNode () |
end current node, set current node to parent | |
void | WriteContent (const Util::String &text) |
write content text | |
void | WriteComment (const Util::String &comment) |
write a comment | |
void | SetString (const Util::String &name, const Util::String &value) |
set string attribute on current node | |
void | SetBool (const Util::String &name, bool value) |
set bool attribute on current node | |
void | SetInt (const Util::String &name, int value) |
set int attribute on current node | |
void | SetFloat (const Util::String &name, float value) |
set float attribute on current node | |
void | SetFloat2 (const Util::String &name, const Math::float2 &value) |
set float2 attribute on current node | |
void | SetFloat4 (const Util::String &name, const Math::float4 &value) |
set float4 attribute on current node | |
void | SetMatrix44 (const Util::String &name, const Math::matrix44 &value) |
set matrix44 attribute on current node | |
template<typename T> | |
void | Set (const Util::String &name, const T &value) |
generic setter, template specializations implemented in nebula3/code/addons/nebula2 | |
void | SetStream (const Ptr< Stream > &s) |
set stream to write to | |
const Ptr< Stream > & | GetStream () const |
get currently set stream | |
bool | HasStream () const |
return true if a stream is set | |
bool | IsOpen () const |
return true if currently open | |
int | GetRefCount () const |
get the current refcount | |
void | AddRef () |
increment refcount by one | |
void | Release () |
decrement refcount and destroy object if refcount is zero | |
bool | IsInstanceOf (const Rtti &rtti) const |
return true if this object is instance of given class | |
bool | IsInstanceOf (const Util::String &className) const |
return true if this object is instance of given class by string | |
bool | IsInstanceOf (const Util::FourCC &classFourCC) const |
return true if this object is instance of given class by fourcc | |
bool | IsA (const Rtti &rtti) const |
return true if this object is instance of given class, or a derived class | |
bool | IsA (const Util::String &rttiName) const |
return true if this object is instance of given class, or a derived class, by string | |
bool | IsA (const Util::FourCC &rttiFourCC) const |
return true if this object is instance of given class, or a derived class, by fourcc | |
const Util::String & | GetClassName () const |
get the class name | |
Util::FourCC | GetClassFourCC () const |
get the class FourCC code | |
Static Public Member Functions | |
static void | DumpRefCountingLeaks () |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!) |
Member Function Documentation
bool IO::XmlWriter::Open | ( | ) | [virtual] |
begin writing the stream
Open the XML stream for writing. This will create a new TiXmlDocument object which will be written to the stream in Close().
Reimplemented from IO::StreamWriter.
void IO::XmlWriter::Close | ( | ) | [virtual] |
bool IO::XmlWriter::BeginNode | ( | const Util::String & | nodeName | ) |
begin a new node under the current node
Begin a new node. The new node will be set as the current node. Nodes may form a hierarchy. Make sure to finalize a node with a corresponding call to EndNode()!
void IO::XmlWriter::EndNode | ( | ) |
end current node, set current node to parent
Finalize current node. This will set the parent of the current node as new current node so that correct hierarchical behaviour is implemented.
void IO::XmlWriter::WriteContent | ( | const Util::String & | text | ) |
write content text
Write inline text at current position.
void IO::XmlWriter::WriteComment | ( | const Util::String & | comment | ) |
write a comment
Write a comment into the XML file.
void IO::XmlWriter::SetString | ( | const Util::String & | name, | |
const Util::String & | value | |||
) |
set string attribute on current node
Set the provided attribute to a string value.
void IO::XmlWriter::SetBool | ( | const Util::String & | name, | |
bool | value | |||
) |
set bool attribute on current node
Set the provided attribute to a bool value.
void IO::XmlWriter::SetInt | ( | const Util::String & | name, | |
int | value | |||
) |
set int attribute on current node
Set the provided attribute to an int value.
void IO::XmlWriter::SetFloat | ( | const Util::String & | name, | |
float | value | |||
) |
set float attribute on current node
Set the provided attribute to a float value.
void IO::XmlWriter::SetFloat4 | ( | const Util::String & | name, | |
const Math::float4 & | value | |||
) |
set float4 attribute on current node
Set the provided attribute to a float4 value.
void IO::XmlWriter::SetMatrix44 | ( | const Util::String & | name, | |
const Math::matrix44 & | value | |||
) |
set matrix44 attribute on current node
Set the provided attribute to a matrix44 value. The stream must be in Write or ReadWrite mode for this.
set stream to write to
Attaches the writer to a stream. This will imcrement the refcount of the stream.
Reimplemented in Messaging::MessageWriter.
get currently set stream
Get pointer to the attached stream. If there is no stream attached, an assertion will be thrown. Use HasStream() to determine if a stream is attached.
bool IO::StreamWriter::HasStream | ( | ) | const [inherited] |
return true if a stream is set
Returns true if a stream is attached to the writer.
int Core::RefCounted::GetRefCount | ( | ) | const [inline, inherited] |
get the current refcount
Return the current refcount of the object.
void Core::RefCounted::AddRef | ( | ) | [inline, inherited] |
increment refcount by one
Increment the refcount of the object.
void Core::RefCounted::Release | ( | ) | [inline, inherited] |
decrement refcount and destroy object if refcount is zero
Decrement the refcount and destroy object if refcount is zero.
const Util::String & Core::RefCounted::GetClassName | ( | ) | const [inline, inherited] |
get the class name
Get the class name of the object.
Util::FourCC Core::RefCounted::GetClassFourCC | ( | ) | const [inline, inherited] |
get the class FourCC code
Get the class FourCC of the object.
void Core::RefCounted::DumpRefCountingLeaks | ( | ) | [static, inherited] |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!)
This method should be called as the very last before an application exits.