Lighting::InternalPointLightEntity Class Reference
#include <internalpointlightentity.h>
Inheritance diagram for Lighting::InternalPointLightEntity:
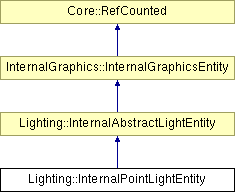
Detailed Description
Implements a local spot light. The spot light's cone is computed from the transformation matrix of the light entity. The spot light cone will point along -z, and the cone's angle will be defined by the length of x and y component at the end of the z component.(C) 2007 Radon Labs GmbH
Public Types | |
enum | LinkType |
visibility link types | |
typedef IndexT | Id |
a unique id for graphics entities | |
Public Member Functions | |
InternalPointLightEntity () | |
constructor | |
virtual Math::ClipStatus::Type | ComputeClipStatus (const Math::bbox &box) |
compute clip status against bounding box | |
virtual void | HandleMessage (const Ptr< Messaging::Message > &msg) |
handle a message | |
LightType::Code | GetLightType () const |
get the light type | |
void | SetColor (const Math::float4 &c) |
set primary light color | |
const Math::float4 & | GetColor () const |
get primary light color | |
void | SetCastShadows (bool b) |
enable/disable shadow casting | |
bool | GetCastShadows () const |
get shadow casting flag | |
void | SetProjMapUvOffsetAndScale (const Math::float4 &v) |
set projection map UV offset and scale (xy->offset, zw->scale) | |
const Math::float4 & | GetProjMapUvOffsetAndScale () const |
get projection map UV offset and scale | |
const Math::matrix44 & | GetInvTransform () const |
get inverse transform (transforms from world to light space) | |
const Math::matrix44 & | GetProjTransform () const |
get light-projection matrix (transforms from light space to light projection space) | |
const Math::matrix44 & | GetInvLightProjTransform () const |
get world-to-light-projection transform (transform from world to light projection space) | |
void | SetShadowBufferUvOffsetAndScale (const Math::float4 &uvOffset) |
set shadow buffer uv rectangle (optionally set by light/shadow servers) | |
const Math::float4 & | GetShadowBufferUvOffsetAndScale () const |
get shadow buffer uv rectangle | |
bool | GetCastShadowsThisFrame () const |
get cast shadows this frame | |
void | SetCastShadowsThisFrame (bool val) |
set CastShadowsThisFrame | |
void | SetShadowTransform (const Math::matrix44 &val) |
set shadow transform | |
const Math::matrix44 & | GetShadowInvTransform () |
get shadow transform | |
const Math::matrix44 & | GetShadowInvLightProjTransform () |
get shadow projection transform | |
const Math::matrix44 & | GetShadowProjTransform () |
get shadow projection transform | |
float | GetShadowIntensity () const |
get ShadowIntensity | |
void | SetShadowIntensity (float val) |
set ShadowIntensity | |
bool | IsActive () const |
return true if entity is currently active (is between OnActivate()/OnDeactivate() | |
bool | IsValid () const |
return true if entity is current valid (ready for rendering) | |
Id | GetId () const |
get the graphics entity's unique id | |
InternalGraphicsEntityType::Code | GetType () const |
get the entity type | |
void | SetTransform (const Math::matrix44 &m) |
set the entity's world space transform | |
const Math::matrix44 & | GetTransform () const |
get the entity's world space transform | |
void | SetVisible (bool b) |
set the entity's visibility | |
bool | IsVisible () const |
return true if entity is set to visible | |
const Ptr< InternalStage > & | GetStage () const |
get the stage this entity is attached to | |
bool | IsAttachedToStage () const |
return true if entity is attached to stage | |
Timing::Time | GetEntityTime () const |
get current entity time | |
const Math::bbox & | GetLocalBoundingBox () |
get the local space bounding box | |
const Math::bbox & | GetGlobalBoundingBox () |
get bounding box in global space | |
void | ClearLinks (LinkType linkType) |
clear all visibility links | |
void | AddLink (LinkType linkType, const Ptr< InternalGraphicsEntity > &entity) |
add visibility link | |
const Util::Array< Ptr< InternalGraphicsEntity > > & | GetLinks (LinkType type) const |
get visibility links by type | |
void | MarkRemove () |
mark the entity for removal from the stage at the next possible time | |
bool | IsMarkedForRemove () const |
return true if this entity has been marked for removal | |
void | AddDeferredMessage (const Ptr< Messaging::Message > &msg) |
add a message for deferred handling once the object becomes valid | |
int | GetRefCount () const |
get the current refcount | |
void | AddRef () |
increment refcount by one | |
void | Release () |
decrement refcount and destroy object if refcount is zero | |
bool | IsInstanceOf (const Rtti &rtti) const |
return true if this object is instance of given class | |
bool | IsInstanceOf (const Util::String &className) const |
return true if this object is instance of given class by string | |
bool | IsInstanceOf (const Util::FourCC &classFourCC) const |
return true if this object is instance of given class by fourcc | |
bool | IsA (const Rtti &rtti) const |
return true if this object is instance of given class, or a derived class | |
bool | IsA (const Util::String &rttiName) const |
return true if this object is instance of given class, or a derived class, by string | |
bool | IsA (const Util::FourCC &rttiFourCC) const |
return true if this object is instance of given class, or a derived class, by fourcc | |
const Util::String & | GetClassName () const |
get the class name | |
Util::FourCC | GetClassFourCC () const |
get the class FourCC code | |
Static Public Member Functions | |
static void | DumpRefCountingLeaks () |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!) | |
Protected Member Functions | |
virtual void | OnTransformChanged () |
called when transform matrix changed | |
virtual void | OnRenderDebug () |
called to render a debug visualization of the entity | |
virtual void | OnResolveVisibility () |
called from internalview | |
void | SetLightType (LightType::Code c) |
set the light type (must be called from sub-classes constructor | |
void | UpdateShadowTransforms () |
update shadow transforms | |
void | SetSharedData (const Ptr< FrameSync::FrameSyncSharedData > &data) |
set pointer to shared data object | |
void | SetType (InternalGraphicsEntityType::Code t) |
set entity type, call in constructor of derived class! | |
void | SetValid (bool b) |
set to valid state (when the entity becomes ready for rendering) | |
void | UpdateClipStatus (Math::ClipStatus::Type c) |
update current clip status | |
void | UpdateTime (Timing::Time time, Timing::Time timeFactor) |
update current time | |
virtual void | OnActivate () |
called when entity is created | |
virtual void | OnDeactivate () |
called before entity is destroyed | |
virtual void | OnAttachToStage (const Ptr< InternalStage > &stage) |
called when attached to Stage | |
virtual void | OnRemoveFromStage () |
called when removed from Stage | |
virtual void | OnSetupSharedData () |
called to setup the client-portion of the shared data object | |
virtual void | OnDiscardSharedData () |
called to discard the client-portion of the shared data object | |
virtual void | OnResetSharedData () |
called per frame to reset the shared data object | |
virtual void | OnShow () |
called when the entity becomes visible | |
virtual void | OnHide () |
called when the entity becomes invisible | |
virtual void | OnCullBefore (Timing::Time time, Timing::Time globalTimeFactor, IndexT frameIndex) |
called before culling on each(!) graphics entity (visible or not!) | |
virtual void | OnNotifyCullingVisible (const Ptr< InternalGraphicsEntity > &observer, IndexT frameIndex) |
called when the entity has been found visible during culling, may be called several times per frame! | |
virtual void | OnRenderBefore (IndexT frameIndex) |
called right before rendering | |
void | SetLocalBoundingBox (const Math::bbox &b) |
set the local space bounding box | |
void | UpdateGlobalBoundingBox () |
update the global bounding box from the transform and local box | |
void | HandleDeferredMessages () |
handle deferred messages (called by subclasses once resources are loaded) |
Member Function Documentation
void Lighting::InternalPointLightEntity::HandleMessage | ( | const Ptr< Messaging::Message > & | msg | ) | [virtual] |
handle a message
Handle a message, override this method accordingly in subclasses!
Reimplemented from Lighting::InternalAbstractLightEntity.
void Lighting::InternalAbstractLightEntity::OnResolveVisibility | ( | ) | [protected, virtual, inherited] |
called from internalview
This method is called whenever the the internalview comes to its Render method. Add light entities to the LightServer or to the ShadowServer.
Reimplemented from InternalGraphics::InternalGraphicsEntity.
void InternalGraphics::InternalGraphicsEntity::AddDeferredMessage | ( | const Ptr< Messaging::Message > & | msg | ) | [inherited] |
add a message for deferred handling once the object becomes valid
Message handlers may decide to defer message handling until the object has become valid.
void InternalGraphics::InternalGraphicsEntity::OnActivate | ( | ) | [protected, virtual, inherited] |
called when entity is created
Activate the entity. This method is called when the entity is created an attached to the graphics server. During OnActivate() the entity should perform any one-time initializations.
Reimplemented in InternalGraphics::InternalModelEntity.
void InternalGraphics::InternalGraphicsEntity::OnDeactivate | ( | ) | [protected, virtual, inherited] |
called before entity is destroyed
Deactivate the entity, this method is called when the entity is removed from the graphics server. Any initialization done in OnActivate() should be undone here.
Reimplemented in InternalGraphics::InternalCameraEntity, and InternalGraphics::InternalModelEntity.
void InternalGraphics::InternalGraphicsEntity::OnAttachToStage | ( | const Ptr< InternalStage > & | s | ) | [protected, virtual, inherited] |
called when attached to Stage
This method is called when the graphics entity is attached to a stage. An entity may only be attached to one stage at any time, but can be attached to different stages during its lifetime. Attachging an entity to a stage may be relatively slow because the entity must be inserted into the cell hierarchy.
void InternalGraphics::InternalGraphicsEntity::OnRemoveFromStage | ( | ) | [protected, virtual, inherited] |
called when removed from Stage
This method is called when the graphics entity is removed from a stage.
void InternalGraphics::InternalGraphicsEntity::OnSetupSharedData | ( | ) | [protected, virtual, inherited] |
called to setup the client-portion of the shared data object
This method is called from OnActivate() to setup the shared data object of the entity. The method must call the ClientSetup() method on the sharedData object with the same template type as the main-thread side entity.
Reimplemented in InternalGraphics::InternalModelEntity.
void InternalGraphics::InternalGraphicsEntity::OnDiscardSharedData | ( | ) | [protected, virtual, inherited] |
called to discard the client-portion of the shared data object
Called from OnDeactivate() to discard the shared data object of the entity from the client side.
Reimplemented in InternalGraphics::InternalModelEntity.
void InternalGraphics::InternalGraphicsEntity::OnResetSharedData | ( | ) | [protected, virtual, inherited] |
called per frame to reset the shared data object
This method is called once per frame before OnCullBefore() to initialize the shared data object with suitable data (which may be overwritten with uptodate-data later in the frame). This is necessary because the SharedData object is double buffered, and thus if an update if missed for one frame invalid data from the previous frame may "leak" into the next frame.
Reimplemented in InternalGraphics::InternalModelEntity.
void InternalGraphics::InternalGraphicsEntity::OnShow | ( | ) | [protected, virtual, inherited] |
called when the entity becomes visible
This method is called from the SetVisible() method when the entity changes from invisible to visible state.
Reimplemented in InternalGraphics::InternalModelEntity.
void InternalGraphics::InternalGraphicsEntity::OnHide | ( | ) | [protected, virtual, inherited] |
called when the entity becomes invisible
This method is called from the SetVisible() method when the entity changes from visible to invisible state.
Reimplemented in InternalGraphics::InternalModelEntity.
void InternalGraphics::InternalGraphicsEntity::OnCullBefore | ( | Timing::Time | time_, | |
Timing::Time | timeFactor_, | |||
IndexT | frameIndex | |||
) | [protected, virtual, inherited] |
called before culling on each(!) graphics entity (visible or not!)
This method is called at the beginning of a frame before culling happens on EVERY entity.
Reimplemented in InternalGraphics::InternalModelEntity.
void InternalGraphics::InternalGraphicsEntity::OnNotifyCullingVisible | ( | const Ptr< InternalGraphicsEntity > & | observer, | |
IndexT | frameIndex | |||
) | [protected, virtual, inherited] |
called when the entity has been found visible during culling, may be called several times per frame!
This method is called during visibility linking when an observed entity is found to be visible by an observer (a camera or a light entity). NOTE that this method will be called several times per frame, so it may be a good idea to use the graphics server's frame counter to protect expensive code from multiple execution.
Reimplemented in InternalGraphics::InternalModelEntity.
void InternalGraphics::InternalGraphicsEntity::OnRenderBefore | ( | IndexT | frameIndex | ) | [protected, virtual, inherited] |
called right before rendering
This method is called on the entity from InternalView::Render() once per frame for every visible entity.
Reimplemented in InternalGraphics::InternalModelEntity.
void InternalGraphics::InternalGraphicsEntity::HandleDeferredMessages | ( | ) | [protected, inherited] |
handle deferred messages (called by subclasses once resources are loaded)
This method is called once when an object with deferred validation (e.g. ModelEntities) become valid (usually after their resources have finished loading). Any deferred messages will be processed here.
int Core::RefCounted::GetRefCount | ( | ) | const [inline, inherited] |
get the current refcount
Return the current refcount of the object.
void Core::RefCounted::AddRef | ( | ) | [inline, inherited] |
increment refcount by one
Increment the refcount of the object.
void Core::RefCounted::Release | ( | ) | [inline, inherited] |
decrement refcount and destroy object if refcount is zero
Decrement the refcount and destroy object if refcount is zero.
const Util::String & Core::RefCounted::GetClassName | ( | ) | const [inline, inherited] |
get the class name
Get the class name of the object.
Util::FourCC Core::RefCounted::GetClassFourCC | ( | ) | const [inline, inherited] |
get the class FourCC code
Get the class FourCC of the object.
void Core::RefCounted::DumpRefCountingLeaks | ( | ) | [static, inherited] |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!)
This method should be called as the very last before an application exits.