BaseGameFeature::FactoryManager Class Reference
#include <factorymanager.h>
Inheritance diagram for BaseGameFeature::FactoryManager:
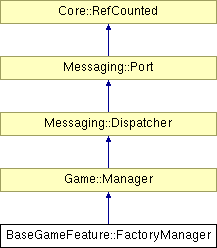
Detailed Description
The FactoryManager is responsible for creating new game entities. FactoryManager must be extended by Mangalore applications if the application needs new game entity classes.The FactoryManager loads the file
data:tables/blueprints.xml
on creation, which contains the construction blueprints for the entity types of your application. This file defines entity types by the properties which are added to them.
(C) 2007 Radon Labs GmbH
Public Member Functions | |
FactoryManager () | |
constructor | |
virtual | ~FactoryManager () |
destructor | |
virtual Ptr< Game::Entity > | CreateEntityByClassName (const Util::String &cppClassName) const |
create a new raw game entity by type name, extend this method in subclasses for new types | |
virtual Ptr< Game::Entity > | CreateEntityByCategory (const Util::String &categoryName, const Ptr< Db::ValueTable > &attrTable, IndexT attrTableRowIndex, bool onlyDistributedProperties=false) const |
create a new entity from its category name | |
virtual Ptr< Game::Entity > | CreateEntityByAttrs (const Util::String &categoryName, const Util::Array< Attr::Attribute > &attrs, bool onlyDistributedProperties=false) const |
create a new entity from scratch and initialize it with the provided attributes | |
virtual Ptr< Game::Entity > | CreateEntityByTemplate (const Util::String &categoryName, const Util::String &templateName, bool onlyDistributedProperties=false) const |
create a new entity from a database template entry | |
virtual Ptr< Game::Entity > | CreateEntityByTemplateAsCategory (const Util::String &categoryName, const Util::String &templateName, const Util::String &targetCategory) const |
create a new entity from a database template entry, and add it into a different category | |
virtual Ptr< Game::Entity > | CreateEntityByEntity (const Ptr< Game::Entity > &sourceEntity) const |
create a new entity cloning an existing one | |
virtual Ptr< Game::Entity > | CreateEntityByEntityAsCategory (const Ptr< Game::Entity > &sourceEntity, const Util::String &targetCategory, bool createMissingAttributes=false) const |
create a new entity in a different category cloning an existing one | |
virtual Ptr< Game::Entity > | CreateEntityByKeyAttr (const Attr::Attribute &key) const |
create new entity from world database using any key attribute | |
virtual Ptr< Game::Entity > | CreateEntityByGuid (const Util::Guid &guid) const |
create new entity from world database using GUID as key attribute | |
virtual Ptr< Game::Property > | CreateProperty (const Util::String &type) const |
create a new property by type name, extend in subclass for new types | |
void | AddProperties (const Ptr< Game::Entity > &entity, const Util::String &categoryName, bool onlyRemoteProperties) const |
add properties to entity according to blue print | |
virtual void | SetupAttributes () |
setup attributes on properties | |
virtual void | OnActivate () |
called when attached to game server | |
virtual void | OnDeactivate () |
called when removed from game server | |
bool | IsActive () const |
return true if currently active | |
virtual void | OnBeginFrame () |
called before frame by the game server | |
virtual void | OnFrame () |
called per-frame by the game server | |
virtual void | OnEndFrame () |
called after frame by the game server | |
virtual void | OnLoad () |
called after loading game state | |
virtual void | OnSave () |
called before saving game state | |
virtual void | OnStart () |
called by Game::Server::Start() when the world is started | |
virtual void | OnRenderDebug () |
render a debug visualization | |
virtual void | HandleMessage (const Ptr< Messaging::Message > &msg) |
handle a single message (distribute to ports which accept the message) | |
void | AttachPort (const Ptr< Port > &port) |
attach a message port | |
void | RemovePort (const Ptr< Port > &port) |
remove a message port | |
bool | HasPort (const Ptr< Port > &port) const |
return true if a port exists | |
virtual void | SetupAcceptedMessages () |
override to register accepted messages | |
void | AttachHandler (const Ptr< Handler > &h) |
attach a message handler to the port | |
void | RemoveHandler (const Ptr< Handler > &h) |
remove a message handler from the port | |
void | RemoveAllHandlers () |
remove all message handler from the port | |
SizeT | GetNumHandlers () const |
return number of handlers attached to the port | |
const Ptr< Handler > & | GetHandlerAtIndex (IndexT i) const |
get a message handler by index | |
virtual void | Send (const Ptr< Message > &msg) |
send a message to the port | |
const Util::Array< const Id * > & | GetAcceptedMessages () const |
get the array of accepted messages (sorted) | |
bool | AcceptsMessage (const Id &msgId) const |
return true if port accepts this msg | |
int | GetRefCount () const |
get the current refcount | |
void | AddRef () |
increment refcount by one | |
void | Release () |
decrement refcount and destroy object if refcount is zero | |
bool | IsInstanceOf (const Rtti &rtti) const |
return true if this object is instance of given class | |
bool | IsInstanceOf (const Util::String &className) const |
return true if this object is instance of given class by string | |
bool | IsInstanceOf (const Util::FourCC &classFourCC) const |
return true if this object is instance of given class by fourcc | |
bool | IsA (const Rtti &rtti) const |
return true if this object is instance of given class, or a derived class | |
bool | IsA (const Util::String &rttiName) const |
return true if this object is instance of given class, or a derived class, by string | |
bool | IsA (const Util::FourCC &rttiFourCC) const |
return true if this object is instance of given class, or a derived class, by fourcc | |
const Util::String & | GetClassName () const |
get the class name | |
Util::FourCC | GetClassFourCC () const |
get the class FourCC code | |
Static Public Member Functions | |
static void | SetBlueprintsFilename (const Util::String &name) |
set a optional blueprints.xml, which is used instead of standard blieprint.xml | |
static void | DumpRefCountingLeaks () |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!) | |
Protected Member Functions | |
bool | ParseBluePrints () |
parse entity blueprints file | |
IndexT | FindBluePrint (const Util::String &entityType) const |
find blueprint index by property type | |
void | RegisterMessage (const Id &msgId) |
register a single accepted message | |
Data Structures | |
struct | PropertyEntry |
an entity blueprint, these are created by ParseBlueprints() More... |
Member Function Documentation
Ptr< Entity > BaseGameFeature::FactoryManager::CreateEntityByClassName | ( | const Util::String & | cppClassName | ) | const [virtual] |
create a new raw game entity by type name, extend this method in subclasses for new types
Create a raw entity by its C++ class name name. This method should be extended by subclasses if a Mangalore application implements new Game::Entity subclasses.
Ptr< Entity > BaseGameFeature::FactoryManager::CreateEntityByCategory | ( | const Util::String & | categoryName, | |
const Ptr< Db::ValueTable > & | attrTable, | |||
IndexT | attrTableRowIndex, | |||
bool | onlyRemoteProperties = false | |||
) | const [virtual] |
create a new entity from its category name
Create an entity from its category name. The category name is looked up in the blueprints.xml file to check what properties must be attached to the entity. All required properties will be attached, and all attributes will be initialised according to the attribute table.
Ptr< Entity > BaseGameFeature::FactoryManager::CreateEntityByAttrs | ( | const Util::String & | categoryName, | |
const Util::Array< Attr::Attribute > & | attrs, | |||
bool | onlyRemoteProperties = false | |||
) | const [virtual] |
create a new entity from scratch and initialize it with the provided attributes
Create a new entity from scratch and initialize it with the provided attributes.
Ptr< Entity > BaseGameFeature::FactoryManager::CreateEntityByTemplate | ( | const Util::String & | categoryName, | |
const Util::String & | templateName, | |||
bool | onlyRemoteProperties = false | |||
) | const [virtual] |
create a new entity from a database template entry
Create an entity from a template in the database. The template is defined by category name and the template name defined by the the Attr::Id attribute. This will create a complete entity with properties and attributes initialized to the values from the template. A new GUID will be assigned to the entity.
Ptr< Entity > BaseGameFeature::FactoryManager::CreateEntityByTemplateAsCategory | ( | const Util::String & | categoryName, | |
const Util::String & | templateName, | |||
const Util::String & | targetCategory | |||
) | const [virtual] |
create a new entity from a database template entry, and add it into a different category
Create a new entity from a template, but put the entity into a different category.
Ptr< Entity > BaseGameFeature::FactoryManager::CreateEntityByEntity | ( | const Ptr< Game::Entity > & | sourceEntity | ) | const [virtual] |
create a new entity cloning an existing one
Create a entity as a clone of a existing one. A new GUID will be assigned.
Ptr< Entity > BaseGameFeature::FactoryManager::CreateEntityByEntityAsCategory | ( | const Ptr< Game::Entity > & | sourceEntity, | |
const Util::String & | targetCategory, | |||
bool | createMissingAttributes = false | |||
) | const [virtual] |
create a new entity in a different category cloning an existing one
Create a entity as a clone of a existing one but in a different category as the original entity. A new GUID will be assigned.
Ptr< Entity > BaseGameFeature::FactoryManager::CreateEntityByKeyAttr | ( | const Attr::Attribute & | key | ) | const [virtual] |
create new entity from world database using any key attribute
This will 'load' a new entity from the world database. This will create a new entity, attach properties as described by blueprints.xml, and update the entity attributes from the database. Changes to attributes can later be written back to the database by calling the Entity::Save() method.
NOTE: this method will not call the Entity::OnLoad() method, which may be required to finally initialize the entity. The OnLoad() method expects that all other entities in the level have already been loaded, so this must be done after loading in a separate pass.
NOTE: use this method only if you know there's only one matching entity in the database (for instance by Guid attribute), otherwise, use the CreateEntitiesByKeyAttr() method, which checks all matches.
Ptr< Entity > BaseGameFeature::FactoryManager::CreateEntityByGuid | ( | const Util::Guid & | guid | ) | const [virtual] |
create new entity from world database using GUID as key attribute
Creates a new entity from the world database using a GUID as key. Simply calls CreateEntityByKeyAttr().
Ptr< Property > BaseGameFeature::FactoryManager::CreateProperty | ( | const Util::String & | type | ) | const [virtual] |
create a new property by type name, extend in subclass for new types
Create a property by its type name. This method should be extended by subclasses if a Mangalore application implements new properties.
void BaseGameFeature::FactoryManager::AddProperties | ( | const Ptr< Game::Entity > & | entity, | |
const Util::String & | categoryName, | |||
bool | onlyRemoteProperties | |||
) | const |
add properties to entity according to blue print
This method checks if a blueprint for the provided entity exists, and adds the properties defined in the blue print to the entity. If no matching blueprint exists, the entity will not be altered.
void BaseGameFeature::FactoryManager::SetupAttributes | ( | ) | [virtual] |
setup attributes on properties
Create the properties of every category and call SetupDefaultAttributes on it.
bool BaseGameFeature::FactoryManager::ParseBluePrints | ( | ) | [protected] |
parse entity blueprints file
This method parses the file data:tables/blueprints.xml into the bluePrints array.
IndexT BaseGameFeature::FactoryManager::FindBluePrint | ( | const Util::String & | entityType | ) | const [protected] |
find blueprint index by property type
This method finds a blueprint index by entity type name. Returns InvalidIndex if blueprint doesn't exist.
void Game::Manager::OnActivate | ( | ) | [virtual, inherited] |
called when attached to game server
This method is called when the manager is attached to the game server. The manager base class will register its message port with the message server.
Reimplemented in BaseGameFeature::TimeManager, BaseGameFeature::CategoryManager, BaseGameFeature::EnvQueryManager, BaseGameFeature::GlobalAttrsManager, Script::DialogManager, and Script::ScriptManager.
void Game::Manager::OnDeactivate | ( | ) | [virtual, inherited] |
called when removed from game server
This method is called when the manager is removed from the game server. It will unregister its message port from the message server at this point.
Reimplemented in BaseGameFeature::TimeManager, BaseGameFeature::CategoryManager, BaseGameFeature::EntityManager, BaseGameFeature::EnvEntityManager, BaseGameFeature::EnvQueryManager, Script::DialogManager, and Script::ScriptManager.
void Game::Manager::OnBeginFrame | ( | ) | [virtual, inherited] |
called before frame by the game server
Called before frame, override in subclasses
Reimplemented in BaseGameFeature::EntityManager.
void Game::Manager::OnEndFrame | ( | ) | [virtual, inherited] |
called after frame by the game server
Called after frame, override in subclasses
Reimplemented in BaseGameFeature::EntityManager.
void Messaging::Dispatcher::HandleMessage | ( | const Ptr< Messaging::Message > & | msg | ) | [virtual, inherited] |
handle a single message (distribute to ports which accept the message)
Handle a message. The message will only be distributed to ports which accept the message.
Reimplemented from Messaging::Port.
Reimplemented in Script::DialogManager.
attach a message port
Attach a new message port.
- Parameters:
-
port pointer to a message port object
remove a message port
Remove a message port object.
- Parameters:
-
handler pointer to message port object to be removed
return true if a port exists
Return true if a port is already attached.
attach a message handler to the port
Attach a message handler to the port.
remove a message handler from the port
Remove a message handler from the port.
send a message to the port
Send a message to the port. This will immediately call the HandleMessage() method of all attached handlers. If the message has been handled by at least one of the handlers, the Handled() flag of the message will be set to true.
int Core::RefCounted::GetRefCount | ( | ) | const [inline, inherited] |
get the current refcount
Return the current refcount of the object.
void Core::RefCounted::AddRef | ( | ) | [inline, inherited] |
increment refcount by one
Increment the refcount of the object.
void Core::RefCounted::Release | ( | ) | [inline, inherited] |
decrement refcount and destroy object if refcount is zero
Decrement the refcount and destroy object if refcount is zero.
const Util::String & Core::RefCounted::GetClassName | ( | ) | const [inline, inherited] |
get the class name
Get the class name of the object.
Util::FourCC Core::RefCounted::GetClassFourCC | ( | ) | const [inline, inherited] |
get the class FourCC code
Get the class FourCC of the object.
void Core::RefCounted::DumpRefCountingLeaks | ( | ) | [static, inherited] |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!)
This method should be called as the very last before an application exits.