IO::ZipFileStream Class Reference
#include <zipfilestream.h>
Inheritance diagram for IO::ZipFileStream:
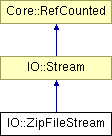
Detailed Description
Wraps a file in a zip archive into a stream. The file int the zip-archive is not cached. Only forward reading is allowed. Only one file must be opened in that archive at a time.The IO::Server allows transparent access to data in zip files through normal "file:" URIs by first checking whether the file is part of a mounted zip archive. Only if this is not the case, the file will be opened as normal.
To force reading from a zip archive, use an URI of the following format:
zip://[samba server]/bla/blob/archive.zip?file=path/in/zipfile&pwd=password
This assumes that the URI scheme "zip" has been associated with the ZipFileStream class using the IO::Server::RegisterUriScheme() method.
The server and local path part of the URI contain the path to the zip archive file. The query part contains the path of the file in the zip archive and an optional password.
(C) 2006 Radon Labs GmbH
Public Types | |
enum | AccessMode |
access modes | |
enum | AccessPattern |
access prefered pattern | |
enum | SeekOrigin |
seek origins | |
typedef int | Position |
typedefs | |
Public Member Functions | |
ZipFileStream () | |
constructor | |
virtual | ~ZipFileStream () |
destructor | |
virtual bool | CanRead () const |
memory streams support reading | |
virtual bool | CanWrite () const |
memory streams support writing | |
virtual bool | CanSeek () const |
memory streams support seeking | |
virtual bool | CanBeMapped () const |
memory streams are mappable | |
virtual Size | GetSize () const |
get the size of the stream in bytes | |
virtual Position | GetPosition () const |
get the current position of the read/write cursor | |
virtual bool | Open () |
open the stream | |
virtual void | Close () |
close the stream | |
virtual Size | Read (void *ptr, Size numBytes) |
directly read from the stream | |
virtual void | Seek (Offset offset, SeekOrigin origin) |
seek in stream, only forward seeks are allowed | |
virtual bool | Eof () const |
return true if end-of-stream reached | |
virtual void * | Map () |
map for direct memory-access | |
virtual void | Unmap () |
unmap a mapped stream | |
void | SetURI (const URI &u) |
set stream location as URI | |
const URI & | GetURI () const |
get stream URI | |
virtual void | SetSize (Size s) |
set a new size for the stream | |
void | SetAccessMode (AccessMode m) |
set the access mode of the stream (default is ReadAccess) | |
AccessMode | GetAccessMode () const |
get the access mode of the stream | |
void | SetAccessPattern (AccessPattern p) |
set the prefered access pattern (default is Sequential) | |
AccessPattern | GetAccessPattern () const |
get the prefered access pattern | |
void | SetMediaType (const MediaType &t) |
set optional media type of stream content | |
const MediaType & | GetMediaType () const |
get optional media type | |
bool | IsOpen () const |
return true if currently open | |
virtual void | Write (const void *ptr, Size numBytes) |
directly write to the stream | |
virtual Size | Read (void *ptr, Size numBytes) |
directly read from the stream | |
virtual void | Seek (Offset offset, SeekOrigin origin) |
seek in stream | |
virtual void | Flush () |
flush unsaved data | |
bool | IsMapped () const |
return true if stream is currently mapped to memory | |
int | GetRefCount () const |
get the current refcount | |
void | AddRef () |
increment refcount by one | |
void | Release () |
decrement refcount and destroy object if refcount is zero | |
bool | IsInstanceOf (const Rtti &rtti) const |
return true if this object is instance of given class | |
bool | IsInstanceOf (const Util::String &className) const |
return true if this object is instance of given class by string | |
bool | IsInstanceOf (const Util::FourCC &classFourCC) const |
return true if this object is instance of given class by fourcc | |
bool | IsA (const Rtti &rtti) const |
return true if this object is instance of given class, or a derived class | |
bool | IsA (const Util::String &rttiName) const |
return true if this object is instance of given class, or a derived class, by string | |
bool | IsA (const Util::FourCC &rttiFourCC) const |
return true if this object is instance of given class, or a derived class, by fourcc | |
const Util::String & | GetClassName () const |
get the class name | |
Util::FourCC | GetClassFourCC () const |
get the class FourCC code | |
Static Public Member Functions | |
static void | DumpRefCountingLeaks () |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!) |
Member Function Documentation
bool IO::ZipFileStream::Open | ( | ) | [virtual] |
open the stream
Open the stream for reading. This will decompress the entire file from the zip archive into memory.
Reimplemented from IO::Stream.
void IO::Stream::SetURI | ( | const URI & | u | ) | [inherited] |
const URI & IO::Stream::GetURI | ( | ) | const [inherited] |
void IO::Stream::SetSize | ( | Size | s | ) | [virtual, inherited] |
set a new size for the stream
This sets a new size for the stream. Not all streams support this method. If the new size if smaller then the existing size, the contents will be clipped.
void IO::Stream::SetAccessMode | ( | AccessMode | m | ) | [inherited] |
set the access mode of the stream (default is ReadAccess)
This method sets the intended access mode of the stream. The actual behaviour depends on the implementation of the derived class. The default is ReadWrite.
Stream::AccessMode IO::Stream::GetAccessMode | ( | ) | const [inherited] |
get the access mode of the stream
Get the access mode of the stream.
void IO::Stream::SetAccessPattern | ( | AccessPattern | p | ) | [inherited] |
set the prefered access pattern (default is Sequential)
Set the prefered access pattern of the stream. This can be Random or Sequential. This is an optional flag to improve performance with some stream implementations. The default is sequential. The pattern cannot be changed while the stream is open.
Stream::AccessPattern IO::Stream::GetAccessPattern | ( | ) | const [inherited] |
get the prefered access pattern
Get the currently set prefered access pattern of the stream.
bool IO::Stream::IsOpen | ( | ) | const [inherited] |
return true if currently open
Return true if the stream is currently open.
void IO::Stream::Write | ( | const void * | ptr, | |
Size | numBytes | |||
) | [virtual, inherited] |
directly write to the stream
Write raw data to the stream. For more convenient writing, attach the stream to an IO::StreamWriter object. This method is only valid if the stream class returns true in CanWrite().
Stream::Size IO::Stream::Read | ( | void * | ptr, | |
Size | numBytes | |||
) | [virtual, inherited] |
directly read from the stream
Read raw data from the stream. For more convenient reading, attach the stream to an IO::StreamReader object. The method returns the number of bytes actually read. This method is only valid if the stream class returns true in CanRead(). Returns the number of bytes actually read from the stream, this may be less then numBytes, or 0 if end-of-stream is reached.
void IO::Stream::Seek | ( | Offset | offset, | |
SeekOrigin | origin | |||
) | [virtual, inherited] |
seek in stream
Move the read/write cursor to a new position, returns the new position in the stream. This method is only supported if the stream class returns true in CanSeek().
void IO::Stream::Flush | ( | ) | [virtual, inherited] |
flush unsaved data
Flush any unsaved data. Note that unsaved data will also be flushed automatically when the stream is closed.
Reimplemented in IO::FileStream.
bool IO::Stream::IsMapped | ( | ) | const [inherited] |
return true if stream is currently mapped to memory
Returns true if the stream is currently mapped.
int Core::RefCounted::GetRefCount | ( | ) | const [inline, inherited] |
get the current refcount
Return the current refcount of the object.
void Core::RefCounted::AddRef | ( | ) | [inline, inherited] |
increment refcount by one
Increment the refcount of the object.
void Core::RefCounted::Release | ( | ) | [inline, inherited] |
decrement refcount and destroy object if refcount is zero
Decrement the refcount and destroy object if refcount is zero.
const Util::String & Core::RefCounted::GetClassName | ( | ) | const [inline, inherited] |
get the class name
Get the class name of the object.
Util::FourCC Core::RefCounted::GetClassFourCC | ( | ) | const [inline, inherited] |
get the class FourCC code
Get the class FourCC of the object.
void Core::RefCounted::DumpRefCountingLeaks | ( | ) | [static, inherited] |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!)
This method should be called as the very last before an application exits.