IO::Console Class Reference
#include <console.h>
Inheritance diagram for IO::Console:
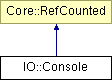
Detailed Description
Nebula3's console, this is the central place for command- line-style communication with the user. By default, all output will just disappear unless console handlers are added. Console handlers are user-derivable objects which do something with the output and may provide text input.(C) 2006 Radon Labs GmbH
Public Member Functions | |
Console () | |
constructor | |
virtual | ~Console () |
destructor | |
void | Open () |
open the console | |
void | Close () |
close the console | |
bool | IsOpen () const |
return true if currently open | |
void | Update () |
called per-frame | |
void | AttachHandler (const Ptr< ConsoleHandler > &handler) |
attach a console handler to the console | |
void | RemoveHandler (const Ptr< ConsoleHandler > &handler) |
remove a console handler from the console | |
Util::Array< Ptr< ConsoleHandler > > | GetHandlers () const |
get array of currently installed handlers | |
bool | HasInput () const |
return true if user input is available | |
Util::String | GetInput () const |
get user input | |
void __cdecl | Print (const char *fmt,...) |
print a formatted line (printf style) | |
void __cdecl | Print (const char *fmt, va_list argList) |
print a formatted line (printf style) | |
void | Print (const Util::String &s) |
print a string object | |
void __cdecl | Error (const char *fmt,...) |
put an error message and cancel execution | |
void __cdecl | Error (const char *fmt, va_list argList) |
put an error message and cancel execution | |
void __cdecl | Warning (const char *fmt,...) |
put a warning message | |
void __cdecl | Warning (const char *fmt, va_list argList) |
put a warning message | |
void __cdecl | Confirm (const char *fmt,...) |
display a confirmation message box | |
void __cdecl | Confirm (const char *fmt, va_list argList) |
display a confirmation message box | |
void __cdecl | DebugOut (const char *fmt,...) |
print a debug-only message | |
void __cdecl | DebugOut (const char *fmt, va_list argList) |
print a debug-only message | |
int | GetRefCount () const |
get the current refcount | |
void | AddRef () |
increment refcount by one | |
void | Release () |
decrement refcount and destroy object if refcount is zero | |
bool | IsInstanceOf (const Rtti &rtti) const |
return true if this object is instance of given class | |
bool | IsInstanceOf (const Util::String &className) const |
return true if this object is instance of given class by string | |
bool | IsInstanceOf (const Util::FourCC &classFourCC) const |
return true if this object is instance of given class by fourcc | |
bool | IsA (const Rtti &rtti) const |
return true if this object is instance of given class, or a derived class | |
bool | IsA (const Util::String &rttiName) const |
return true if this object is instance of given class, or a derived class, by string | |
bool | IsA (const Util::FourCC &rttiFourCC) const |
return true if this object is instance of given class, or a derived class, by fourcc | |
const Util::String & | GetClassName () const |
get the class name | |
Util::FourCC | GetClassFourCC () const |
get the class FourCC code | |
Static Public Member Functions | |
static void | DumpRefCountingLeaks () |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!) |
Member Function Documentation
void IO::Console::Update | ( | ) |
called per-frame
This method may only be called from the main thread!
int Core::RefCounted::GetRefCount | ( | ) | const [inline, inherited] |
get the current refcount
Return the current refcount of the object.
void Core::RefCounted::AddRef | ( | ) | [inline, inherited] |
increment refcount by one
Increment the refcount of the object.
void Core::RefCounted::Release | ( | ) | [inline, inherited] |
decrement refcount and destroy object if refcount is zero
Decrement the refcount and destroy object if refcount is zero.
const Util::String & Core::RefCounted::GetClassName | ( | ) | const [inline, inherited] |
get the class name
Get the class name of the object.
Util::FourCC Core::RefCounted::GetClassFourCC | ( | ) | const [inline, inherited] |
get the class FourCC code
Get the class FourCC of the object.
void Core::RefCounted::DumpRefCountingLeaks | ( | ) | [static, inherited] |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!)
This method should be called as the very last before an application exits.