Base::DisplayDeviceBase Class Reference
#include <displaydevicebase.h>
Inheritance diagram for Base::DisplayDeviceBase:
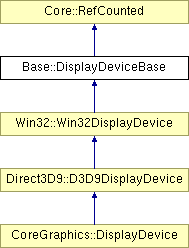
Detailed Description
A DisplayDevice object represents the display where the RenderDevice presents the rendered frame. Use the display device object to get information about available adapters and display modes, and to set the preferred display mode of a Nebula3 application.(C) 2006 Radon Labs GmbH
Public Member Functions | |
DisplayDeviceBase () | |
constructor | |
virtual | ~DisplayDeviceBase () |
destructor | |
bool | AdapterExists (CoreGraphics::Adapter::Code adapter) |
return true if adapter exists | |
Util::Array< CoreGraphics::DisplayMode > | GetAvailableDisplayModes (CoreGraphics::Adapter::Code adapter, CoreGraphics::PixelFormat::Code pixelFormat) |
get available display modes on given adapter | |
bool | SupportsDisplayMode (CoreGraphics::Adapter::Code adapter, const CoreGraphics::DisplayMode &requestedMode) |
return true if a given display mode is supported | |
CoreGraphics::DisplayMode | GetCurrentAdapterDisplayMode (CoreGraphics::Adapter::Code adapter) |
get current adapter display mode (i.e. the desktop display mode) | |
CoreGraphics::AdapterInfo | GetAdapterInfo (CoreGraphics::Adapter::Code adapter) |
get general info about display adapter | |
void | SetAdapter (CoreGraphics::Adapter::Code a) |
set display adapter (make sure adapter exists!) | |
CoreGraphics::Adapter::Code | GetAdapter () const |
get display adapter | |
void | SetDisplayMode (const CoreGraphics::DisplayMode &m) |
set display mode (make sure the display mode is supported!) | |
const CoreGraphics::DisplayMode & | GetDisplayMode () const |
get display mode | |
void | SetAntiAliasQuality (CoreGraphics::AntiAliasQuality::Code aa) |
set antialias quality | |
CoreGraphics::AntiAliasQuality::Code | GetAntiAliasQuality () const |
get antialias quality | |
void | SetFullscreen (bool b) |
set windowed/fullscreen mode | |
bool | IsFullscreen () const |
get windowed/fullscreen mode | |
void | SetDisplayModeSwitchEnabled (bool b) |
enable display mode switch when running fullscreen (default is true); | |
bool | IsDisplayModeSwitchEnabled () const |
is display mode switch enabled for fullscreen? | |
void | SetTripleBufferingEnabled (bool b) |
enable triple buffer for fullscreen (default is double buffering) | |
bool | IsTripleBufferingEnabled () const |
is triple buffer enabled for fullscreen? | |
void | SetAlwaysOnTop (bool b) |
set always-on-top behaviour | |
bool | IsAlwaysOnTop () const |
get always-on-top behaviour | |
void | SetVerticalSyncEnabled (bool b) |
turn vertical sync on/off | |
bool | IsVerticalSyncEnabled () const |
get vertical sync flag | |
void | SetIconName (const Util::String &s) |
set optional window icon resource name | |
const Util::String & | GetIconName () const |
get optional window icon resource name | |
void | SetParentWindow (void *h) |
set optional parent window handle | |
void * | GetParentWindow () const |
get optional parent window handle | |
void | SetWindowTitle (const Util::String &t) |
set window title string (can be changed anytime) | |
const Util::String & | GetWindowTitle () const |
get window title string | |
bool | Open () |
open the display | |
void | Close () |
close the display | |
bool | IsOpen () const |
return true if display is currently open | |
void | ProcessWindowMessages () |
process window system messages, call this method once per frame | |
void | AttachEventHandler (const Ptr< CoreGraphics::DisplayEventHandler > &h) |
attach a display event handler | |
void | RemoveEventHandler (const Ptr< CoreGraphics::DisplayEventHandler > &h) |
remove a display event handler | |
int | GetRefCount () const |
get the current refcount | |
void | AddRef () |
increment refcount by one | |
void | Release () |
decrement refcount and destroy object if refcount is zero | |
bool | IsInstanceOf (const Rtti &rtti) const |
return true if this object is instance of given class | |
bool | IsInstanceOf (const Util::String &className) const |
return true if this object is instance of given class by string | |
bool | IsInstanceOf (const Util::FourCC &classFourCC) const |
return true if this object is instance of given class by fourcc | |
bool | IsA (const Rtti &rtti) const |
return true if this object is instance of given class, or a derived class | |
bool | IsA (const Util::String &rttiName) const |
return true if this object is instance of given class, or a derived class, by string | |
bool | IsA (const Util::FourCC &rttiFourCC) const |
return true if this object is instance of given class, or a derived class, by fourcc | |
const Util::String & | GetClassName () const |
get the class name | |
Util::FourCC | GetClassFourCC () const |
get the class FourCC code | |
Static Public Member Functions | |
static void | DumpRefCountingLeaks () |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!) | |
Protected Member Functions | |
bool | NotifyEventHandlers (const CoreGraphics::DisplayEvent &e) |
notify event handlers about an event |
Member Function Documentation
bool Base::DisplayDeviceBase::AdapterExists | ( | CoreGraphics::Adapter::Code | adapter | ) |
return true if adapter exists
Checks if the given adapter exists.
Reimplemented in Direct3D9::D3D9DisplayDevice.
Util::Array< DisplayMode > Base::DisplayDeviceBase::GetAvailableDisplayModes | ( | CoreGraphics::Adapter::Code | adapter, | |
CoreGraphics::PixelFormat::Code | pixelFormat | |||
) |
get available display modes on given adapter
Returns the display modes on the given adapter in the given pixel format.
Reimplemented in Direct3D9::D3D9DisplayDevice.
bool Base::DisplayDeviceBase::SupportsDisplayMode | ( | CoreGraphics::Adapter::Code | adapter, | |
const CoreGraphics::DisplayMode & | requestedMode | |||
) |
return true if a given display mode is supported
This method checks the available display modes on the given adapter against the requested display modes and returns true if the display mode exists.
Reimplemented in Direct3D9::D3D9DisplayDevice.
DisplayMode Base::DisplayDeviceBase::GetCurrentAdapterDisplayMode | ( | CoreGraphics::Adapter::Code | adapter | ) |
get current adapter display mode (i.e. the desktop display mode)
This method returns the current adapter display mode. It can be used to get the current desktop display mode.
Reimplemented in Direct3D9::D3D9DisplayDevice.
AdapterInfo Base::DisplayDeviceBase::GetAdapterInfo | ( | CoreGraphics::Adapter::Code | adapter | ) |
get general info about display adapter
Returns information about the provided adapter.
Reimplemented in Direct3D9::D3D9DisplayDevice.
void Base::DisplayDeviceBase::SetWindowTitle | ( | const Util::String & | str | ) |
set window title string (can be changed anytime)
Set the window title. An application should be able to change the window title at any time, that's why this is a virtual method, so that a subclass may override it.
bool Base::DisplayDeviceBase::Open | ( | ) |
void Base::DisplayDeviceBase::Close | ( | ) |
void Base::DisplayDeviceBase::ProcessWindowMessages | ( | ) |
process window system messages, call this method once per frame
Process window system messages. Override this method in a subclass.
Reimplemented in Win32::Win32DisplayDevice.
void Base::DisplayDeviceBase::AttachEventHandler | ( | const Ptr< CoreGraphics::DisplayEventHandler > & | h | ) |
attach a display event handler
Attach an event handler to the display device.
void Base::DisplayDeviceBase::RemoveEventHandler | ( | const Ptr< CoreGraphics::DisplayEventHandler > & | h | ) |
remove a display event handler
Remove an event handler from the display device.
bool Base::DisplayDeviceBase::NotifyEventHandlers | ( | const CoreGraphics::DisplayEvent & | e | ) | [protected] |
notify event handlers about an event
Notify all event handlers about an event.
int Core::RefCounted::GetRefCount | ( | ) | const [inline, inherited] |
get the current refcount
Return the current refcount of the object.
void Core::RefCounted::AddRef | ( | ) | [inline, inherited] |
increment refcount by one
Increment the refcount of the object.
void Core::RefCounted::Release | ( | ) | [inline, inherited] |
decrement refcount and destroy object if refcount is zero
Decrement the refcount and destroy object if refcount is zero.
const Util::String & Core::RefCounted::GetClassName | ( | ) | const [inline, inherited] |
get the class name
Get the class name of the object.
Util::FourCC Core::RefCounted::GetClassFourCC | ( | ) | const [inline, inherited] |
get the class FourCC code
Get the class FourCC of the object.
void Core::RefCounted::DumpRefCountingLeaks | ( | ) | [static, inherited] |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!)
This method should be called as the very last before an application exits.