Actions::Action Class Reference
#include <action.h>
Inheritance diagram for Actions::Action:
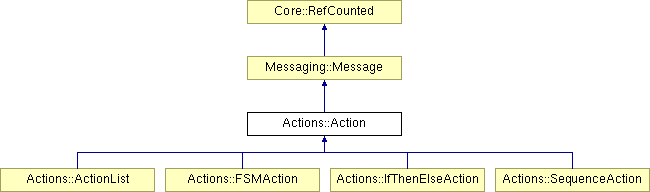
Detailed Description
Actions are blocks that request a state change to the entitys, the world. By default the actions are just msgs that have the receiver set and on execute they will just be send to the target entity. The real action execution must be implemented in the property that handles this action msg.Actions are used by several other DSA subsystems, like the quest and dialog subsystem. Actions could be created from scripting or console.
Actions can be created from a type string by DsaFactoryManager.
(C) 2005 Radon Labs GmbH
Public Member Functions | |
Action () | |
constructor | |
virtual | ~Action () |
destructor | |
virtual void | OnActivate () |
called when state is activated | |
virtual void | OnDeactivate () |
called when state is deactivated | |
virtual void | Notify (const Ptr< Messaging::Message > &msg) |
notify about incoming message | |
virtual bool | Start () |
start the action | |
virtual void | Stop () |
stop the action | |
virtual void | Execute () |
execute the action, by default this sends self as a message to the target entity | |
virtual bool | Trigger () |
virtual void | Assert () |
assert that all required data is present in the world database | |
virtual bool | Assert (const Ptr< Script::InfoLog > &infoLog) |
like Assert() but adds errors to the info log object instead of closing the application | |
virtual void | ParseArgs (const Util::CommandLineArgs &args) |
parse arguments from command line args object | |
virtual void | ShowActionInfo () |
make the action show information what would happen if executed; default: do nothing | |
virtual Timing::Time | GetTimeLeft () |
get time left (default: 0) | |
virtual Util::String | GetDebugTxt () |
get current debug txt | |
virtual void | SetEntity (const Ptr< Game::Entity > &v) |
Set target entity to `v'. | |
const Ptr< Game::Entity > & | GetEntity () const |
Target entity if exists. | |
bool | HasEntity () const |
Does this contain a target entity? | |
virtual void | Init () |
init after creation, parse args and set entity | |
virtual void | Write (const Ptr< Script::ActionReader > &actionReader) |
write to action reader | |
virtual void | Read (const Ptr< Script::ActionReader > &actionReader) |
read from action reader | |
bool | CheckId (const Messaging::Id &id) const |
return true if message is of the given id | |
virtual void | Encode (const Ptr< IO::BinaryWriter > &writer) |
encode message into a stream | |
virtual void | Decode (const Ptr< IO::BinaryReader > &reader) |
decode message from a stream | |
void | SetHandled (bool b) |
set the handled flag | |
bool | Handled () const |
return true if the message has been handled | |
void | SetDeferred (bool b) |
set deferred flag | |
bool | IsDeferred () const |
get deferred flag | |
void | SetDeferredHandled (bool b) |
set the deferred handled flag | |
bool | DeferredHandled () const |
get the deferred handled flag | |
int | GetRefCount () const |
get the current refcount | |
void | AddRef () |
increment refcount by one | |
void | Release () |
decrement refcount and destroy object if refcount is zero | |
bool | IsInstanceOf (const Rtti &rtti) const |
return true if this object is instance of given class | |
bool | IsInstanceOf (const Util::String &className) const |
return true if this object is instance of given class by string | |
bool | IsInstanceOf (const Util::FourCC &classFourCC) const |
return true if this object is instance of given class by fourcc | |
bool | IsA (const Rtti &rtti) const |
return true if this object is instance of given class, or a derived class | |
bool | IsA (const Util::String &rttiName) const |
return true if this object is instance of given class, or a derived class, by string | |
bool | IsA (const Util::FourCC &rttiFourCC) const |
return true if this object is instance of given class, or a derived class, by fourcc | |
const Util::String & | GetClassName () const |
get the class name | |
Util::FourCC | GetClassFourCC () const |
get the class FourCC code | |
Static Public Member Functions | |
static Ptr< Action > | CreateActionFromString (const Util::String &cmd) |
create complete action from command string | |
static Util::Array< Ptr< Action > > | CreateActionsFromString (const Util::String &cmd) |
create several actions from semicolon-separated commands | |
static void | DumpRefCountingLeaks () |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!) |
Member Function Documentation
Ptr< Action > Actions::Action::CreateActionFromString | ( | const Util::String & | cmd | ) | [static] |
create complete action from command string
Static method which creates any action object from a command string of the form:
cmd key0=value0 key1=value1 key2=value2
So you would create an action for instance like this:
Action::CreateFromCmdString("UnlockQuest quest=Testquest");
Util::Array< Ptr< Action > > Actions::Action::CreateActionsFromString | ( | const Util::String & | cmd | ) | [static] |
create several actions from semicolon-separated commands
Static method which creates many action from a string of the form accepted by CreateActionFromString() where several actions are separated by a semicolon.
void Actions::Action::Execute | ( | ) | [virtual] |
execute the action, by default this sends self as a message to the target entity
As default just send self to target.
Reimplemented in Actions::ActionList, Actions::IfThenElseAction, and Actions::SequenceAction.
bool Actions::Action::Trigger | ( | ) | [virtual] |
trigger the action, return if the action is still running, by default this calls execute and returns false
Reimplemented in Actions::FSMAction, Actions::IfThenElseAction, and Actions::SequenceAction.
void Actions::Action::Assert | ( | ) | [virtual] |
assert that all required data is present in the world database
Make sure the data required by the action is valid. This may not change the state of the world.
Reimplemented in Actions::ActionList, and Actions::IfThenElseAction.
bool Actions::Action::Assert | ( | const Ptr< Script::InfoLog > & | infoLog | ) | [virtual] |
like Assert() but adds errors to the info log object instead of closing the application
This method makes sure the data required by the action is valid. This may not change the state of the world. In sub classes errors can be added to the info log object and in case of errors false can be returned instead of closing the application.
Override in subclass! (infoLog in this class is ignored; Assert() will be called; returns always true)
Reimplemented in Actions::IfThenElseAction, and Actions::SequenceAction.
void Actions::Action::ParseArgs | ( | const Util::CommandLineArgs & | args | ) | [virtual] |
parse arguments from command line args object
Initialize the action from a CmdLineArgs object. This is necessary for the automatic scripting support.
void Actions::Action::ShowActionInfo | ( | ) | [virtual] |
make the action show information what would happen if executed; default: do nothing
override in special action
int Core::RefCounted::GetRefCount | ( | ) | const [inline, inherited] |
get the current refcount
Return the current refcount of the object.
void Core::RefCounted::AddRef | ( | ) | [inline, inherited] |
increment refcount by one
Increment the refcount of the object.
void Core::RefCounted::Release | ( | ) | [inline, inherited] |
decrement refcount and destroy object if refcount is zero
Decrement the refcount and destroy object if refcount is zero.
const Util::String & Core::RefCounted::GetClassName | ( | ) | const [inline, inherited] |
get the class name
Get the class name of the object.
Util::FourCC Core::RefCounted::GetClassFourCC | ( | ) | const [inline, inherited] |
get the class FourCC code
Get the class FourCC of the object.
void Core::RefCounted::DumpRefCountingLeaks | ( | ) | [static, inherited] |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!)
This method should be called as the very last before an application exits.