Models::ModelNode Class Reference
#include <modelnode.h>
Inheritance diagram for Models::ModelNode:
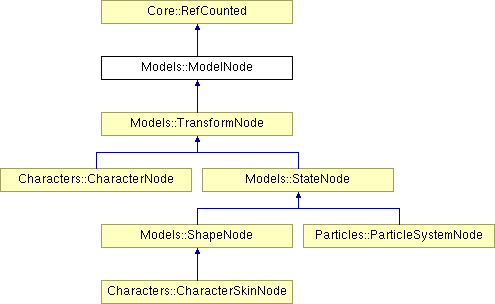
Detailed Description
Represents a transformation hierarchy element inside a Model. Subclasses of ModelNodes represent transformations and geometry of a 3D model arranged in 3d hierarchy (but not in a logical hierarchy of C++ object, instead model nodes live in a flat array to prevent recursive iteration).A ModelNode is roughly comparable to a nSceneNode in Nebula2.
(C) 2007 Radon Labs GmbH
Public Member Functions | |
ModelNode () | |
constructor | |
virtual | ~ModelNode () |
destructor | |
virtual Ptr< ModelNodeInstance > | CreateNodeInstance () const |
create a model node instance | |
Ptr< ModelNodeInstance > | CreateNodeInstanceHierarchy (const Ptr< ModelInstance > &modelInst) |
recursively create model node instance and child model node instances | |
virtual void | OnAttachToModel (const Ptr< Model > &model) |
called when attached to model node | |
virtual void | OnRemoveFromModel () |
called when removed from model node | |
virtual void | LoadResources () |
called when resources should be loaded | |
virtual void | UnloadResources () |
called when resources should be unloaded | |
virtual void | OnResourcesLoaded () |
called once when all pending resource have been loaded | |
virtual void | BeginParseDataTags () |
begin parsing data tags | |
virtual bool | ParseDataTag (const Util::FourCC &fourCC, const Ptr< IO::BinaryReader > &reader) |
parse data tag (called by loader code) | |
virtual void | EndParseDataTags () |
finish parsing data tags | |
virtual void | ApplySharedState (IndexT frameIndex) |
apply state shared by all my ModelNodeInstances | |
virtual Resources::Resource::State | GetResourceState () const |
get overall state of contained resources (Initial, Loaded, Pending, Failed, Cancelled) | |
bool | IsAttachedToModel () const |
return true if currently attached to a Model | |
const Ptr< Model > & | GetModel () const |
get model this node is attached to | |
void | SetResourceStreamingLevelOfDetail (float factor) |
sets the resourceStreamingLevelOfDetail but only if the given value is bigger than the current one (reseted on frame-start) | |
void | ResetScreenSpaceStats () |
resets all screen space stats e.g. size | |
void | SetBoundingBox (const Math::bbox &b) |
set bounding box | |
const Math::bbox & | GetBoundingBox () const |
get bounding box of model node | |
void | SetName (const Util::StringAtom &n) |
set model node name | |
const Util::StringAtom & | GetName () const |
get model node name | |
void | SetType (ModelNodeType::Code t) |
set ModelNodeType | |
ModelNodeType::Code | GetType () const |
get the ModelNodeType | |
void | SetParent (const Ptr< ModelNode > &p) |
set parent node | |
const Ptr< ModelNode > & | GetParent () const |
get parent node | |
bool | HasParent () const |
return true if node has a parent | |
void | AddChild (const Ptr< ModelNode > &c) |
add a child node | |
const Util::Array< Ptr< ModelNode > > & | GetChildren () const |
get child nodes | |
bool | HasChild (const Util::StringAtom &name) const |
return true if a direct child exists by name | |
const Ptr< ModelNode > & | LookupChild (const Util::StringAtom &name) const |
get pointer to direct child by name | |
void | AddVisibleNodeInstance (IndexT frameIndex, const Ptr< ModelNodeInstance > &nodeInst) |
called by model node instance on NotifyVisible() | |
const Util::Array< Ptr< ModelNodeInstance > > & | GetVisibleModelNodeInstances (ModelNodeType::Code t) const |
get visible model node instances | |
bool | HasStringAttr (const Util::StringAtom &attrId) const |
has string attr | |
const Util::StringAtom & | GetStringAttr (const Util::StringAtom &attrId) const |
get string attr | |
void | SetStringAttr (const Util::StringAtom &attrId, const Util::StringAtom &value) |
add string attribute | |
int | GetRefCount () const |
get the current refcount | |
void | AddRef () |
increment refcount by one | |
void | Release () |
decrement refcount and destroy object if refcount is zero | |
bool | IsInstanceOf (const Rtti &rtti) const |
return true if this object is instance of given class | |
bool | IsInstanceOf (const Util::String &className) const |
return true if this object is instance of given class by string | |
bool | IsInstanceOf (const Util::FourCC &classFourCC) const |
return true if this object is instance of given class by fourcc | |
bool | IsA (const Rtti &rtti) const |
return true if this object is instance of given class, or a derived class | |
bool | IsA (const Util::String &rttiName) const |
return true if this object is instance of given class, or a derived class, by string | |
bool | IsA (const Util::FourCC &rttiFourCC) const |
return true if this object is instance of given class, or a derived class, by fourcc | |
const Util::String & | GetClassName () const |
get the class name | |
Util::FourCC | GetClassFourCC () const |
get the class FourCC code | |
Static Public Member Functions | |
static void | DumpRefCountingLeaks () |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!) | |
Protected Member Functions | |
virtual Ptr< ModelNodeInstance > | RecurseCreateNodeInstanceHierarchy (const Ptr< ModelInstance > &modelInst, const Ptr< ModelNodeInstance > &parentNodeInst) |
recursively create node instance hierarchy | |
Protected Attributes | |
float | resourceStreamingLevelOfDetail |
factor between 0.0 (close) and 1.0 (far away) describing the distance to camera (used for decision of max needed mipMap) |
Member Function Documentation
Ptr< ModelNodeInstance > Models::ModelNode::CreateNodeInstanceHierarchy | ( | const Ptr< ModelInstance > & | modelInst | ) |
recursively create model node instance and child model node instances
Create the node instance hierarchy.
void Models::ModelNode::LoadResources | ( | ) | [virtual] |
called when resources should be loaded
This method is called when required resources should be loaded.
Reimplemented in Characters::CharacterNode, Models::ShapeNode, Models::StateNode, and Particles::ParticleSystemNode.
void Models::ModelNode::UnloadResources | ( | ) | [virtual] |
called when resources should be unloaded
This method is called when required resources should be unloaded.
Reimplemented in Characters::CharacterNode, Models::ShapeNode, Models::StateNode, and Particles::ParticleSystemNode.
void Models::ModelNode::OnResourcesLoaded | ( | ) | [virtual] |
called once when all pending resource have been loaded
This method is called once by Model::OnResourcesLoaded() when all pending resources of a model have been loaded.
Reimplemented in Characters::CharacterNode, and Particles::ParticleSystemNode.
void Models::ModelNode::BeginParseDataTags | ( | ) | [virtual] |
begin parsing data tags
Begin parsing data tags. This method is called by StreamModelLoader before ParseDataTag() is called for the first time.
bool Models::ModelNode::ParseDataTag | ( | const Util::FourCC & | fourCC, | |
const Ptr< IO::BinaryReader > & | reader | |||
) | [virtual] |
parse data tag (called by loader code)
Parse a single data tag. If a subclass doesn't accept the data tag, the parent class method must be called!
Reimplemented in Characters::CharacterNode, Characters::CharacterSkinNode, Models::ShapeNode, Models::StateNode, Models::TransformNode, and Particles::ParticleSystemNode.
void Models::ModelNode::EndParseDataTags | ( | ) | [virtual] |
finish parsing data tags
End parsing data tags. This method is called by StreamModelLoader after the last ParseDataTag() is called.
void Models::ModelNode::ApplySharedState | ( | IndexT | frameIndex | ) | [virtual] |
apply state shared by all my ModelNodeInstances
This method is called once before rendering the ModelNode's visible instance nodes through the ModelNodeInstance::ApplyState() and ModelNodeInstance::Render() methods. The method must apply the shader state that is shared across all instances. Since this state is constant across all instance nodes, this only happens once before rendering an instance set.
Reimplemented in Characters::CharacterSkinNode, Models::ShapeNode, and Models::StateNode.
Resource::State Models::ModelNode::GetResourceState | ( | ) | const [virtual] |
get overall state of contained resources (Initial, Loaded, Pending, Failed, Cancelled)
Returns the overall resource state (Initial, Loaded, Pending, Failed, Cancelled). Higher states override lower state (if some resources are already Loaded, and some are Pending, then Pending will be returned, if some resources failed to load, then Failed will be returned, etc...). Subclasses which load resource must override this method, and modify the return value of the parent class as needed).
Reimplemented in Characters::CharacterNode, Models::ShapeNode, Models::StateNode, Models::TransformNode, and Particles::ParticleSystemNode.
void Models::ModelNode::ResetScreenSpaceStats | ( | ) | [inline] |
resets all screen space stats e.g. size
Reset resourceStreamingLevelOfDetail to -1.0 as we are able to recognize invisible items this way. (visible items will overwrite this value with a value >= 0.0)
Ptr< ModelNodeInstance > Models::ModelNode::RecurseCreateNodeInstanceHierarchy | ( | const Ptr< ModelInstance > & | modelInst, | |
const Ptr< ModelNodeInstance > & | parentNodeInst | |||
) | [protected, virtual] |
recursively create node instance hierarchy
Recursively create node instances and attach them to the provided model instance. Returns a pointer to the root node instance.
int Core::RefCounted::GetRefCount | ( | ) | const [inline, inherited] |
get the current refcount
Return the current refcount of the object.
void Core::RefCounted::AddRef | ( | ) | [inline, inherited] |
increment refcount by one
Increment the refcount of the object.
void Core::RefCounted::Release | ( | ) | [inline, inherited] |
decrement refcount and destroy object if refcount is zero
Decrement the refcount and destroy object if refcount is zero.
const Util::String & Core::RefCounted::GetClassName | ( | ) | const [inline, inherited] |
get the class name
Get the class name of the object.
Util::FourCC Core::RefCounted::GetClassFourCC | ( | ) | const [inline, inherited] |
get the class FourCC code
Get the class FourCC of the object.
void Core::RefCounted::DumpRefCountingLeaks | ( | ) | [static, inherited] |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!)
This method should be called as the very last before an application exits.