App::GameApplication Class Reference
#include <gameapplication.h>
Inheritance diagram for App::GameApplication:
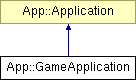
Detailed Description
Nebula3's default game application. It creates and triggers the GameServer. For game features it creates the core and graphicsfeature which is used in every gamestate (such as level gamestates or only gui gamestates).(C) 2007 Radon Labs GmbH
Public Member Functions | |
GameApplication () | |
constructor | |
virtual | ~GameApplication () |
destructor | |
virtual bool | Open () |
open the application | |
virtual void | Close () |
close the application | |
virtual void | Run () |
run the application | |
void | AddStateHandler (const Ptr< StateHandler > &state) |
add an application state handler | |
const Ptr< StateHandler > & | FindStateHandlerByName (const Util::String &stateName) const |
find a state handler by name | |
const Ptr< StateHandler > & | GetCurrentStateHandler () const |
return pointer to current state handler | |
const Util::String & | GetCurrentState () const |
return state handler of current state | |
int | GetNumStates () const |
get number of application states | |
const Ptr< StateHandler > & | GetStateHandlerAt (int index) const |
get state handler at index | |
void | RequestState (const Util::String &stateName) |
request a new state which will be applied at the end of the frame | |
void | SetCompanyName (const Util::String &n) |
set company name | |
const Util::String & | GetCompanyName () const |
get company name | |
void | SetAppTitle (const Util::String &n) |
set application name | |
const Util::String & | GetAppTitle () const |
get application name | |
void | SetAppID (const Util::String &n) |
set application id | |
const Util::String & | GetAppID () const |
get application id | |
void | SetAppVersion (const Util::String &n) |
set application version | |
const Util::String & | GetAppVersion () const |
get application version | |
void | SetCmdLineArgs (const Util::CommandLineArgs &a) |
set command line args | |
const Util::CommandLineArgs & | GetCmdLineArgs () const |
get command line args | |
virtual void | Exit () |
exit the application, call right before leaving main() | |
bool | IsOpen () const |
return true if app is open | |
int | GetReturnCode () const |
get the return code | |
Protected Member Functions | |
virtual void | SetupStateHandlers () |
setup application state handlers | |
virtual void | CleanupStateHandlers () |
cleanup application state handlers | |
virtual void | SetupGameFeatures () |
setup game features | |
virtual void | CleanupGameFeatures () |
cleanup game features | |
virtual void | DoStateTransition () |
perform a state transition | |
void | SetState (const Util::String &s) |
set an application state | |
virtual void | SetupAppFromCmdLineArgs () |
setup app from cmd lines | |
void | SetReturnCode (int c) |
set return code |
Member Function Documentation
void App::GameApplication::Run | ( | ) | [virtual] |
run the application
Run the application. This method will return when the application wishes to exist.
Reimplemented from App::Application.
void App::GameApplication::AddStateHandler | ( | const Ptr< StateHandler > & | handler | ) |
add an application state handler
Register a state handler object with the application.
- Parameters:
-
state pointer to a state handler object
const Ptr< StateHandler > & App::GameApplication::FindStateHandlerByName | ( | const Util::String & | stateName | ) | const |
find a state handler by name
Find a state handler by name.
- Parameters:
-
name of state to return the state handler for
- Returns:
- pointer to state handler object associated with the state (can be 0)
const Ptr< StateHandler > & App::GameApplication::GetCurrentStateHandler | ( | ) | const |
return pointer to current state handler
Get the current state handler.
const Util::String & App::GameApplication::GetCurrentState | ( | ) | const [inline] |
return state handler of current state
Returns the currently active application state. Can be 0 if no valid state is set.
void App::GameApplication::RequestState | ( | const Util::String & | stateName | ) |
request a new state which will be applied at the end of the frame
Request a new state. This is a public method to switch states (SetState() is private because it invokes some internal voodoo). The requested state will be activated at the end of the frame.
void App::GameApplication::SetupStateHandlers | ( | ) | [protected, virtual] |
setup application state handlers
Setup the application state handlers. This method is called by App::Open() after the Mangalore subsystems have been initialized. Override this method to create and attach your application state handlers with the application object.
void App::GameApplication::CleanupStateHandlers | ( | ) | [protected, virtual] |
cleanup application state handlers
Cleanup the application state handlers. This will call the OnRemoveFromApplication() method on all attached state handlers and release them. Usually you don't need to override this method in your app.
void App::GameApplication::SetupGameFeatures | ( | ) | [protected, virtual] |
setup game features
Setup new game features which should be used by this application. Overwrite for all features which have to be used.
void App::GameApplication::CleanupGameFeatures | ( | ) | [protected, virtual] |
cleanup game features
Cleanup all added game features
void App::GameApplication::DoStateTransition | ( | ) | [protected, virtual] |
perform a state transition
Do a state transition. This method is called by SetState() when the new state is different from the previous state.
void App::GameApplication::SetState | ( | const Util::String & | s | ) | [protected] |
set an application state
Set a new application state. This method will call DoStateTransition().