Models::ModelInstance Class Reference
#include <modelinstance.h>
Inheritance diagram for Models::ModelInstance:
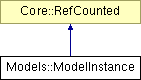
Detailed Description
A ModelInstance contains the per-instance data for rendering a Model. Usually there is one ModelInstance created per game object.A ModelInstance is roughly comparable to a Nebula2 nRenderContext.
(C) 2007 Radon Labs GmbH
Public Member Functions | |
ModelInstance () | |
constructor | |
virtual | ~ModelInstance () |
destructor | |
bool | IsValid () const |
return true if currently valid | |
const Ptr< Model > & | GetModel () const |
get the Model this instance was created from | |
const Ptr< InternalGraphics::InternalModelEntity > & | GetModelEntity () const |
get the ModelEntity which owns this instance | |
void | SetTransform (const Math::matrix44 &m) |
set world space transform of the instance | |
const Math::matrix44 & | GetTransform () const |
get world space transform | |
Ptr< ModelNodeInstance > | LookupNodeInstance (const Util::StringAtom &path) const |
lookup a node instance, return invalid ptr if not exists, this method is SLOW | |
const Util::Array< Ptr< ModelNodeInstance > > & | GetNodeInstances () const |
get all node instances | |
const Ptr< ModelNodeInstance > & | GetRootNodeInstance () const |
get pointer to top-level node instance | |
void | AttachNodeInstance (const Ptr< ModelNodeInstance > &nodeInst) |
attach a node instance which has already been setup | |
void | RemoveNodeInstance (const Ptr< ModelNodeInstance > &nodeInst) |
remove a node instance, does not discard the node instance! | |
void | OnNotifyCullingVisible (IndexT frameIndex, Timing::Time time) |
called from ModelEntity::OnNotifyCullingVisible | |
void | OnRenderBefore (IndexT frameIndex, Timing::Time time) |
called from ModelEntity::OnRenderBefore | |
void | OnVisibilityResolve (IndexT resolveIndex, const Math::matrix44 &cameraTransform) |
called during visibility resolve | |
void | UpdateRenderStats (const Math::matrix44 &cameraTransform) |
calls ModelInstance::OnVisibilityResolve(...) + updating screen space stats | |
void | RenderDebug () |
render node specific debug shape | |
int | GetRefCount () const |
get the current refcount | |
void | AddRef () |
increment refcount by one | |
void | Release () |
decrement refcount and destroy object if refcount is zero | |
bool | IsInstanceOf (const Rtti &rtti) const |
return true if this object is instance of given class | |
bool | IsInstanceOf (const Util::String &className) const |
return true if this object is instance of given class by string | |
bool | IsInstanceOf (const Util::FourCC &classFourCC) const |
return true if this object is instance of given class by fourcc | |
bool | IsA (const Rtti &rtti) const |
return true if this object is instance of given class, or a derived class | |
bool | IsA (const Util::String &rttiName) const |
return true if this object is instance of given class, or a derived class, by string | |
bool | IsA (const Util::FourCC &rttiFourCC) const |
return true if this object is instance of given class, or a derived class, by fourcc | |
const Util::String & | GetClassName () const |
get the class name | |
Util::FourCC | GetClassFourCC () const |
get the class FourCC code | |
Static Public Member Functions | |
static void | DumpRefCountingLeaks () |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!) | |
Protected Member Functions | |
void | Setup (const Ptr< Model > &model, const Ptr< ModelNode > &rootModelNode) |
setup the ModelInstance from a root model node | |
void | Discard () |
cleanup and unlink from model | |
void | SetModelEntity (const Ptr< InternalGraphics::InternalModelEntity > &mdlEntity) |
set pointer to ModelEntity which owns this instance | |
void | OnShow (Timing::Time time) |
called when the entity becomes visible with current time | |
void | OnHide (Timing::Time time) |
called when the entity becomes invisible |
Member Function Documentation
Ptr< ModelNodeInstance > Models::ModelInstance::LookupNodeInstance | ( | const Util::StringAtom & | path | ) | const |
lookup a node instance, return invalid ptr if not exists, this method is SLOW
Careful, this method is SLOW!
const Ptr< ModelNodeInstance > & Models::ModelInstance::GetRootNodeInstance | ( | ) | const |
get pointer to top-level node instance
Get a pointer to the root node instance. This is always the first node instance.
void Models::ModelInstance::AttachNodeInstance | ( | const Ptr< ModelNodeInstance > & | nodeInst | ) |
attach a node instance which has already been setup
Attach a node instance which has already been setup.
void Models::ModelInstance::RemoveNodeInstance | ( | const Ptr< ModelNodeInstance > & | nodeInst | ) |
remove a node instance, does not discard the node instance!
Remove a node instance, do not discard it!
void Models::ModelInstance::OnNotifyCullingVisible | ( | IndexT | frameIndex, | |
Timing::Time | time | |||
) |
called from ModelEntity::OnNotifyCullingVisible
This method is called once per frame on ModelInstances which have been detected as visible during the culling phase (so, relatively early).
void Models::ModelInstance::OnRenderBefore | ( | IndexT | frameIndex, | |
Timing::Time | time | |||
) |
called from ModelEntity::OnRenderBefore
This method is called once per frame on visible ModelInstances right before rendering.
void Models::ModelInstance::OnVisibilityResolve | ( | IndexT | resolveIndex, | |
const Math::matrix44 & | cameraTransform | |||
) |
called during visibility resolve
This method is called by the Graphics subsystem when we are currently visible. Once all visible model instances are notified, the Graphics subsystem can get a render-order-optimized list of all visible model-node-instances through the ModelServer.
void Models::ModelInstance::UpdateRenderStats | ( | const Math::matrix44 & | cameraTransform | ) |
calls ModelInstance::OnVisibilityResolve(...) + updating screen space stats
This updates the render stats of the model. This may only be called by the players camera as other camera transforms (e.g. for light and shadow) shall not influence the LOD of a model.
void Models::ModelInstance::RenderDebug | ( | ) |
render node specific debug shape
This method is called from the RenderDebug of Graphics::ModelEntity.
int Core::RefCounted::GetRefCount | ( | ) | const [inline, inherited] |
get the current refcount
Return the current refcount of the object.
void Core::RefCounted::AddRef | ( | ) | [inline, inherited] |
increment refcount by one
Increment the refcount of the object.
void Core::RefCounted::Release | ( | ) | [inline, inherited] |
decrement refcount and destroy object if refcount is zero
Decrement the refcount and destroy object if refcount is zero.
const Util::String & Core::RefCounted::GetClassName | ( | ) | const [inline, inherited] |
get the class name
Get the class name of the object.
Util::FourCC Core::RefCounted::GetClassFourCC | ( | ) | const [inline, inherited] |
get the class FourCC code
Get the class FourCC of the object.
void Core::RefCounted::DumpRefCountingLeaks | ( | ) | [static, inherited] |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!)
This method should be called as the very last before an application exits.