Base::GamePadBase Class Reference
#include <gamepadbase.h>
Inheritance diagram for Base::GamePadBase:
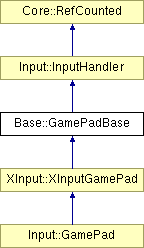
Detailed Description
An input handler which represents one of at most 4 game pads.(C) 2007 Radon Labs GmbH
Public Types | |
enum | Button |
gamepad buttons | |
enum | Axis |
gamepad axis | |
Public Member Functions | |
GamePadBase () | |
constructor | |
virtual | ~GamePadBase () |
destructor | |
bool | IsConnected () const |
return true if this game pad is currently connected | |
void | SetPlayerIndex (IndexT i) |
set player index -> TODO make threadsafe | |
IndexT | GetPlayerIndex () const |
get the player index of this game pad | |
bool | ButtonPressed (Button btn) const |
return true if a button is currently pressed | |
bool | ButtonDown (Button btn) const |
return true if button was down at least once in current frame | |
bool | ButtonUp (Button btn) const |
return true if button was up at least once in current frame | |
float | GetAxisValue (Axis axis) const |
get current axis value | |
void | SetLowFrequencyVibrator (float f) |
set low-frequency vibration effect (0.0f .. 1.0f) | |
float | GetLowFrequencyVibrator () const |
get low-frequency vibration | |
void | SetHighFrequencyVibrator (float f) |
set high-frequency vibration effect (0.0f .. 1.0f) | |
float | GetHighFrequencyVibrator () const |
get high-frequency vibration | |
Util::Array< Input::InputEvent > | GetStateAsInputEvents () const |
get current state as an array of input events (override in subclass!) | |
bool | IsAttached () const |
return true if the input handler is currently attached | |
virtual void | BeginCapture () |
capture input to this event handler | |
virtual void | EndCapture () |
end input capturing to this event handler | |
bool | IsCapturing () const |
return true if this input handler captures input | |
int | GetRefCount () const |
get the current refcount | |
void | AddRef () |
increment refcount by one | |
void | Release () |
decrement refcount and destroy object if refcount is zero | |
bool | IsInstanceOf (const Rtti &rtti) const |
return true if this object is instance of given class | |
bool | IsInstanceOf (const Util::String &className) const |
return true if this object is instance of given class by string | |
bool | IsInstanceOf (const Util::FourCC &classFourCC) const |
return true if this object is instance of given class by fourcc | |
bool | IsA (const Rtti &rtti) const |
return true if this object is instance of given class, or a derived class | |
bool | IsA (const Util::String &rttiName) const |
return true if this object is instance of given class, or a derived class, by string | |
bool | IsA (const Util::FourCC &rttiFourCC) const |
return true if this object is instance of given class, or a derived class, by fourcc | |
const Util::String & | GetClassName () const |
get the class name | |
Util::FourCC | GetClassFourCC () const |
get the class FourCC code | |
Static Public Member Functions | |
static Util::String | ButtonAsString (Button btn) |
convert button code to string | |
static Util::String | AxisAsString (Axis a) |
convert axis to string | |
static SizeT | GetMaxNumPlayers () |
get maximum number of players | |
static void | DumpRefCountingLeaks () |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!) | |
Protected Member Functions | |
virtual void | OnAttach () |
called when the handler is attached to the input server | |
virtual void | OnReset () |
reset the input handler | |
virtual void | OnRemove () |
called when the handler is removed from the input server | |
virtual void | OnBeginFrame () |
called on InputServer::BeginFrame() | |
virtual void | OnEndFrame () |
called on InputServer::EndFrame(); | |
virtual void | OnObtainCapture () |
called when input handler obtains capture | |
virtual void | OnReleaseCapture () |
called when input handler looses capture | |
virtual bool | OnEvent (const InputEvent &inputEvent) |
called when an input event should be processed |
Member Function Documentation
Array< InputEvent > Base::GamePadBase::GetStateAsInputEvents | ( | ) | const |
get current state as an array of input events (override in subclass!)
This method should return the current state of the game pad as input events. It is up to a specific subclass to implement this method.
void Input::InputHandler::BeginCapture | ( | ) | [virtual, inherited] |
capture input to this event handler
Begin capturing input to this input handler. This method must be overriden in a subclass, the derived method must call ObtainMouseCapture(), ObtainKeyboardCapture(), or both, depending on what type input events you want to capture. An input handler which captures input gets all input events of the given type exclusively.
Reimplemented in Base::KeyboardBase, and Base::MouseBase.
void Input::InputHandler::EndCapture | ( | ) | [virtual, inherited] |
end input capturing to this event handler
End capturing input to this input handler. Override this method in a subclass and release the captures obtained in BeginCapture().
Reimplemented in Base::KeyboardBase, and Base::MouseBase.
int Core::RefCounted::GetRefCount | ( | ) | const [inline, inherited] |
get the current refcount
Return the current refcount of the object.
void Core::RefCounted::AddRef | ( | ) | [inline, inherited] |
increment refcount by one
Increment the refcount of the object.
void Core::RefCounted::Release | ( | ) | [inline, inherited] |
decrement refcount and destroy object if refcount is zero
Decrement the refcount and destroy object if refcount is zero.
const Util::String & Core::RefCounted::GetClassName | ( | ) | const [inline, inherited] |
get the class name
Get the class name of the object.
Util::FourCC Core::RefCounted::GetClassFourCC | ( | ) | const [inline, inherited] |
get the class FourCC code
Get the class FourCC of the object.
void Core::RefCounted::DumpRefCountingLeaks | ( | ) | [static, inherited] |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!)
This method should be called as the very last before an application exits.