Graphics::PointLightEntity Class Reference
#include <pointlightentity.h>
Inheritance diagram for Graphics::PointLightEntity:
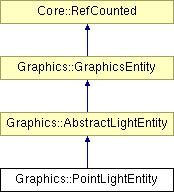
Detailed Description
Client-side proxy of a InternalGraphics::InternalPointLightEntity.(C) 2008 Radon Labs GmbH
Public Member Functions | |
PointLightEntity () | |
constructor | |
void | SetTransformFromPosDirAndRange (const Math::point &pos, const Math::vector &dir, float range) |
set transform from position and range | |
Lighting::LightType::Code | GetLightType () const |
get the light type (defined by subclass) | |
void | SetColor (const Math::float4 &c) |
set primary light color | |
const Math::float4 & | GetColor () const |
get primary light color | |
void | SetCastShadows (bool b) |
enable/disable shadow casting | |
bool | GetCastShadows () const |
get shadow casting flag | |
void | SetProjMapUvOffsetAndScale (const Math::float4 &v) |
set projection map UV offset and scale (xy->offset, zw->scale) | |
const Math::float4 & | GetProjMapUvOffsetAndScale () const |
get projection map UV offset and scale | |
bool | IsValid () const |
return true if entity is valid (is attached to a stage) | |
GraphicsEntityType::Code | GetType () const |
get the entity type | |
void | SetTransform (const Math::matrix44 &m) |
set the entity's world space transform | |
const Math::matrix44 & | GetTransform () const |
get the entity's world space transform | |
void | SetVisible (bool b) |
set the entity's visibility | |
bool | IsVisible () const |
return true if entity is set to visible | |
const Ptr< Stage > & | GetStage () const |
get the stage proxy this entity is attached to | |
Math::ClipStatus::Type | GetClipStatus () const |
get current clip status | |
const Math::bbox & | GetLocalBoundingBox () const |
get the local bounding box | |
const Math::bbox & | GetGlobalBoundingBox () const |
get the global bounding box | |
Timing::Time | GetEntityTime () const |
get current entity time | |
void | SendMsg (const Ptr< GraphicsEntityMessage > &msg) |
send a message to the server-side graphics entity | |
bool | IsObjectRefValid () const |
test if the entity's object ref is valid | |
const Ptr< Threading::ObjectRef > & | GetObjectRef () const |
get the entity handle | |
int | GetRefCount () const |
get the current refcount | |
void | AddRef () |
increment refcount by one | |
void | Release () |
decrement refcount and destroy object if refcount is zero | |
bool | IsInstanceOf (const Rtti &rtti) const |
return true if this object is instance of given class | |
bool | IsInstanceOf (const Util::String &className) const |
return true if this object is instance of given class by string | |
bool | IsInstanceOf (const Util::FourCC &classFourCC) const |
return true if this object is instance of given class by fourcc | |
bool | IsA (const Rtti &rtti) const |
return true if this object is instance of given class, or a derived class | |
bool | IsA (const Util::String &rttiName) const |
return true if this object is instance of given class, or a derived class, by string | |
bool | IsA (const Util::FourCC &rttiFourCC) const |
return true if this object is instance of given class, or a derived class, by fourcc | |
const Util::String & | GetClassName () const |
get the class name | |
Util::FourCC | GetClassFourCC () const |
get the class FourCC code | |
Static Public Member Functions | |
static void | DumpRefCountingLeaks () |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!) | |
Protected Member Functions | |
void | SetLightType (Lighting::LightType::Code c) |
set the light type (must be called from sub-classes constructor | |
void | SetType (GraphicsEntityType::Code t) |
set graphics entity type, called from constructor of subclass | |
virtual void | Discard () |
called by stage when entity should discard itself | |
virtual void | OnSetupSharedData () |
called to setup the FrameSyncSharedData object of the entity | |
virtual void | OnDiscardSharedData () |
called to discard the FrameSyncSharedData object of the entity | |
void | SendCreateMsg (const Ptr< CreateGraphicsEntity > &msg) |
send off a specific create message from the subclass | |
virtual void | OnTransformChanged () |
called when transform matrix changed |
Member Function Documentation
void Graphics::GraphicsEntity::SendMsg | ( | const Ptr< GraphicsEntityMessage > & | msg | ) | [inherited] |
send a message to the server-side graphics entity
Send a generic GraphicsEntityMessage to the server-side entity.
void Graphics::GraphicsEntity::Discard | ( | ) | [protected, virtual, inherited] |
called by stage when entity should discard itself
Discard the server-side graphics entity. This method is called from StageProxy::RemoveEntityProxy(). There's special handling if the server-side entity hasn't been created yet, in this case, a pointer to the original create message must be handed over to the render thread.
Reimplemented in Graphics::CameraEntity.
void Graphics::GraphicsEntity::OnSetupSharedData | ( | ) | [protected, virtual, inherited] |
called to setup the FrameSyncSharedData object of the entity
Setup the shared data object. If subclasses wish to add more members to the shared data object, they must subclass from InternalGraphics::InternalGraphicsEntitySharedData, setup a FrameSyncSharedData object with this data type, and NOT CALL the parent class method! So the lowest subclass defines the type of the shared data!
void Graphics::GraphicsEntity::OnDiscardSharedData | ( | ) | [protected, virtual, inherited] |
called to discard the FrameSyncSharedData object of the entity
Discard the shared data object. See OnSetupSharedData() for details!
void Graphics::GraphicsEntity::SendCreateMsg | ( | const Ptr< CreateGraphicsEntity > & | msg | ) | [protected, inherited] |
send off a specific create message from the subclass
This method must be called from the Setup() method of a subclass to send off a specific creation message. The message will be stored in the proxy to get the entity handle back later when the server-side graphics entity has been created.
void Graphics::GraphicsEntity::OnTransformChanged | ( | ) | [protected, virtual, inherited] |
called when transform matrix changed
Called by SetTransform(). This gives subclasses a chance to react to changes on the transformation matrix.
Reimplemented in Graphics::CameraEntity.
int Core::RefCounted::GetRefCount | ( | ) | const [inline, inherited] |
get the current refcount
Return the current refcount of the object.
void Core::RefCounted::AddRef | ( | ) | [inline, inherited] |
increment refcount by one
Increment the refcount of the object.
void Core::RefCounted::Release | ( | ) | [inline, inherited] |
decrement refcount and destroy object if refcount is zero
Decrement the refcount and destroy object if refcount is zero.
const Util::String & Core::RefCounted::GetClassName | ( | ) | const [inline, inherited] |
get the class name
Get the class name of the object.
Util::FourCC Core::RefCounted::GetClassFourCC | ( | ) | const [inline, inherited] |
get the class FourCC code
Get the class FourCC of the object.
void Core::RefCounted::DumpRefCountingLeaks | ( | ) | [static, inherited] |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!)
This method should be called as the very last before an application exits.