BaseGameFeature::TimeManager Class Reference
#include <timemanager.h>
Inheritance diagram for BaseGameFeature::TimeManager:
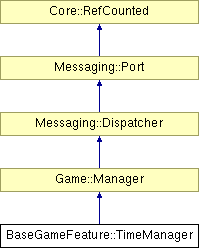
Detailed Description
Singleton object which manages the current game time. These are the standard time source objects provided by Application layer:SystemTimeSource - timing for low level Application layer subsystems GameTimeSource - timing for the game logic CameraTimeSource - extra time source for camera handling GuiTimeSource - time source for user interface stuff
The TimeManager offers a TimeEffect for animating the timefactor of all time sources AND of the graphicsthread to allow "Matrix"-like time effects.
(C) 2009 Radon Labs GmbH
Public Member Functions | |
TimeManager () | |
constructor | |
virtual | ~TimeManager () |
destructor | |
virtual void | OnActivate () |
called when attached to game server | |
virtual void | OnDeactivate () |
called when removed from game server | |
virtual void | OnLoad () |
called after loading game state | |
virtual void | OnSave () |
called before saving game state | |
virtual void | OnFrame () |
on frame | |
void | ResetAll () |
reset all time sources | |
void | PauseAll () |
pause all time sources | |
void | ContinueAll () |
continue all time sources | |
void | SetTimeFactor (float timeFactor, bool resetTimeEffects=true) |
set the time factor | |
float | GetTimeFactor () const |
get the time factor | |
virtual void | OnRenderDebug () |
render a debug visualization | |
void | AttachTimeSource (const Ptr< TimeSource > &timeSource) |
attach a time source | |
void | RemoveTimeSource (const Ptr< TimeSource > &timeSource) |
remove a time source | |
int | GetNumTimeSources () const |
get number of time source | |
const Ptr< TimeSource > & | GetTimeSourceByIndex (int index) const |
get pointer to time source by index | |
Ptr< TimeSource > | GetTimeSourceByClassName (const Util::String &n) const |
get pointer to time source by class name | |
void | StartTimeEffect (float timeFactor, Timing::Tick duration, Timing::Tick fadeIn, Timing::Tick fadeOut) |
start a time effect to animate time factor ("Matrix/MaxPayne" time effect) | |
void | StopTimeEffect (bool immediate=false) |
stop current time effect | |
bool | IsTimeEffectActive () const |
is time effect active | |
bool | IsActive () const |
return true if currently active | |
virtual void | OnBeginFrame () |
called before frame by the game server | |
virtual void | OnEndFrame () |
called after frame by the game server | |
virtual void | OnStart () |
called by Game::Server::Start() when the world is started | |
virtual void | HandleMessage (const Ptr< Messaging::Message > &msg) |
handle a single message (distribute to ports which accept the message) | |
void | AttachPort (const Ptr< Port > &port) |
attach a message port | |
void | RemovePort (const Ptr< Port > &port) |
remove a message port | |
bool | HasPort (const Ptr< Port > &port) const |
return true if a port exists | |
virtual void | SetupAcceptedMessages () |
override to register accepted messages | |
void | AttachHandler (const Ptr< Handler > &h) |
attach a message handler to the port | |
void | RemoveHandler (const Ptr< Handler > &h) |
remove a message handler from the port | |
void | RemoveAllHandlers () |
remove all message handler from the port | |
SizeT | GetNumHandlers () const |
return number of handlers attached to the port | |
const Ptr< Handler > & | GetHandlerAtIndex (IndexT i) const |
get a message handler by index | |
virtual void | Send (const Ptr< Message > &msg) |
send a message to the port | |
const Util::Array< const Id * > & | GetAcceptedMessages () const |
get the array of accepted messages (sorted) | |
bool | AcceptsMessage (const Id &msgId) const |
return true if port accepts this msg | |
int | GetRefCount () const |
get the current refcount | |
void | AddRef () |
increment refcount by one | |
void | Release () |
decrement refcount and destroy object if refcount is zero | |
bool | IsInstanceOf (const Rtti &rtti) const |
return true if this object is instance of given class | |
bool | IsInstanceOf (const Util::String &className) const |
return true if this object is instance of given class by string | |
bool | IsInstanceOf (const Util::FourCC &classFourCC) const |
return true if this object is instance of given class by fourcc | |
bool | IsA (const Rtti &rtti) const |
return true if this object is instance of given class, or a derived class | |
bool | IsA (const Util::String &rttiName) const |
return true if this object is instance of given class, or a derived class, by string | |
bool | IsA (const Util::FourCC &rttiFourCC) const |
return true if this object is instance of given class, or a derived class, by fourcc | |
const Util::String & | GetClassName () const |
get the class name | |
Util::FourCC | GetClassFourCC () const |
get the class FourCC code | |
Static Public Member Functions | |
static void | DumpRefCountingLeaks () |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!) | |
Protected Member Functions | |
void | ApplyGlobalAudioPitch () const |
apply the audio variable | |
void | Update () |
update the time manager | |
void | ApplyTimeEffect () |
apply time effect | |
void | RegisterMessage (const Id &msgId) |
register a single accepted message |
Member Function Documentation
void BaseGameFeature::TimeManager::OnActivate | ( | ) | [virtual] |
called when attached to game server
Activate the time manager. This will create all the standard time sources for Mangalore.
Reimplemented from Game::Manager.
void BaseGameFeature::TimeManager::OnDeactivate | ( | ) | [virtual] |
void BaseGameFeature::TimeManager::OnLoad | ( | ) | [virtual] |
called after loading game state
Checks whether the TimeSources table exists in the database, if yes invokes OnLoad() on all time sources...
Reimplemented from Game::Manager.
void BaseGameFeature::TimeManager::OnSave | ( | ) | [virtual] |
called before saving game state
Ask all time sources to save their status to the database.
Reimplemented from Game::Manager.
void BaseGameFeature::TimeManager::OnFrame | ( | ) | [virtual] |
void BaseGameFeature::TimeManager::ResetAll | ( | ) |
reset all time sources
Reset all time sources. This is usually called at the beginning of an application state.
void BaseGameFeature::TimeManager::PauseAll | ( | ) |
pause all time sources
Pause all time sources. NOTE: there's an independent pause counter inside each time source, a pause just increments the counter, a continue decrements it, when the pause counter is != 0 it means, pause is activated.
void BaseGameFeature::TimeManager::ContinueAll | ( | ) |
continue all time sources
Unpause all time sources.
void BaseGameFeature::TimeManager::OnRenderDebug | ( | ) | [virtual] |
void BaseGameFeature::TimeManager::AttachTimeSource | ( | const Ptr< TimeSource > & | timeSource | ) |
attach a time source
Attach a time source to the time manager. This will invoke OnActivate() on the time source.
void BaseGameFeature::TimeManager::RemoveTimeSource | ( | const Ptr< TimeSource > & | timeSource | ) |
remove a time source
Remove a time source from the time manager. This will invoke OnDeactivate() on the time source.
int BaseGameFeature::TimeManager::GetNumTimeSources | ( | ) | const |
get number of time source
Returns number of time sources attached to the time manager.
const Ptr< TimeSource > & BaseGameFeature::TimeManager::GetTimeSourceByIndex | ( | int | index | ) | const |
get pointer to time source by index
Gets pointer to time source object by index.
Ptr< TimeSource > BaseGameFeature::TimeManager::GetTimeSourceByClassName | ( | const Util::String & | n | ) | const |
get pointer to time source by class name
Get pointer to time source object by class name, returns 0 if not found.
void BaseGameFeature::TimeManager::Update | ( | ) | [protected] |
update the time manager
Update all time sources. This method is called very early in the frame by the current application state handler. This will get the current frame time and call UpdateTime() on all attached time sources.
FIXME: properly handle time exceptions!!!
void Game::Manager::OnBeginFrame | ( | ) | [virtual, inherited] |
called before frame by the game server
Called before frame, override in subclasses
Reimplemented in BaseGameFeature::EntityManager.
void Game::Manager::OnEndFrame | ( | ) | [virtual, inherited] |
called after frame by the game server
Called after frame, override in subclasses
Reimplemented in BaseGameFeature::EntityManager.
void Messaging::Dispatcher::HandleMessage | ( | const Ptr< Messaging::Message > & | msg | ) | [virtual, inherited] |
handle a single message (distribute to ports which accept the message)
Handle a message. The message will only be distributed to ports which accept the message.
Reimplemented from Messaging::Port.
Reimplemented in Script::DialogManager.
attach a message port
Attach a new message port.
- Parameters:
-
port pointer to a message port object
remove a message port
Remove a message port object.
- Parameters:
-
handler pointer to message port object to be removed
return true if a port exists
Return true if a port is already attached.
attach a message handler to the port
Attach a message handler to the port.
remove a message handler from the port
Remove a message handler from the port.
send a message to the port
Send a message to the port. This will immediately call the HandleMessage() method of all attached handlers. If the message has been handled by at least one of the handlers, the Handled() flag of the message will be set to true.
int Core::RefCounted::GetRefCount | ( | ) | const [inline, inherited] |
get the current refcount
Return the current refcount of the object.
void Core::RefCounted::AddRef | ( | ) | [inline, inherited] |
increment refcount by one
Increment the refcount of the object.
void Core::RefCounted::Release | ( | ) | [inline, inherited] |
decrement refcount and destroy object if refcount is zero
Decrement the refcount and destroy object if refcount is zero.
const Util::String & Core::RefCounted::GetClassName | ( | ) | const [inline, inherited] |
get the class name
Get the class name of the object.
Util::FourCC Core::RefCounted::GetClassFourCC | ( | ) | const [inline, inherited] |
get the class FourCC code
Get the class FourCC of the object.
void Core::RefCounted::DumpRefCountingLeaks | ( | ) | [static, inherited] |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!)
This method should be called as the very last before an application exits.