Particles::ParticleSystemNodeInstance Class Reference
#include <particlesystemnodeinstance.h>
Inheritance diagram for Particles::ParticleSystemNodeInstance:
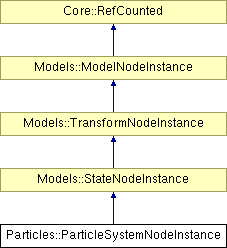
Detailed Description
Wraps a ParticleSystemInstance into a ModelNodeInstance.(C) 2008 Radon Labs GmbH
Public Member Functions | |
ParticleSystemNodeInstance () | |
constructor | |
virtual | ~ParticleSystemNodeInstance () |
destructor | |
virtual void | OnRenderBefore (IndexT frameIndex, Timing::Time time) |
called from ModelEntity::OnRenderBefore | |
virtual void | OnVisibilityResolve (IndexT resolveIndex, float distanceToViewer) |
called during visibility resolve | |
virtual void | ApplyState () |
apply per-instance state prior to rendering | |
virtual void | Render () |
perform rendering | |
const Ptr< ParticleSystemInstance > & | GetParticleSystemInstance () const |
get the node's particle system instance | |
Ptr< CoreGraphics::ShaderVariableInstance > | CreateShaderVariableInstance (const CoreGraphics::ShaderVariable::Semantic &semantic) |
instanciate a shader variable by semantic | |
bool | HasShaderVariableInstance (const CoreGraphics::ShaderVariable::Semantic &semantic) const |
return true if a shader variable has been instantiated | |
const Ptr< CoreGraphics::ShaderVariableInstance > & | GetShaderVariableInstance (const CoreGraphics::ShaderVariable::Semantic &semantic) const |
get a shader variable instance | |
void | SetPosition (const Math::point &p) |
set position | |
const Math::point & | GetPosition () const |
get position | |
void | SetRotate (const Math::quaternion &r) |
set rotate quaternion | |
const Math::quaternion & | GetRotate () const |
get rotate quaternion | |
void | SetScale (const Math::vector &s) |
set scale | |
const Math::vector & | GetScale () const |
get scale | |
void | SetRotatePivot (const Math::point &p) |
set rotate pivot | |
const Math::point & | GetRotatePivot () const |
get rotate pivot | |
void | SetScalePivot (const Math::point &p) |
set scale pivot | |
const Math::point & | GetScalePivot () const |
get scale pivot | |
void | SetOffsetMatrix (const Math::matrix44 &m) |
set optional offset matrix | |
const Math::matrix44 & | GetOffsetMatrix () const |
get optional offset matrix | |
bool | IsInViewSpace () const |
is transformnode in viewspace | |
void | SetInViewSpace (bool b) |
set transformnode in viewspace | |
bool | GetLockedToViewer () const |
get LockedToViewer | |
void | SetLockedToViewer (bool val) |
set LockedToViewer | |
const Math::matrix44 & | GetLocalTransform () |
get resulting local transform matrix in local parent space | |
const Math::matrix44 & | GetModelTransform () const |
get model space transform (valid after Update()) | |
virtual void | DiscardHierarchy () |
discard the model node instance and all of its children | |
bool | IsValid () const |
return true if the model node instance is valid | |
virtual void | OnNotifyCullingVisible (IndexT frameIndex, Timing::Time time) |
called from ModelEntity::OnNotifyCullingVisible | |
const Util::StringAtom & | GetName () const |
get model node name | |
bool | HasParent () const |
return true if node has a parent | |
const Ptr< ModelNodeInstance > & | GetParent () const |
get parent node | |
const Util::Array< Ptr< ModelNodeInstance > > & | GetChildren () const |
get child nodes | |
bool | HasChild (const Util::StringAtom &name) const |
return true if a direct child exists by name | |
const Ptr< ModelNodeInstance > & | LookupChild (const Util::StringAtom &name) const |
get pointer to direct child by name | |
Ptr< ModelNodeInstance > | LookupPath (const Util::String &path) |
get modelnodeinstance by hierarchy path | |
const Ptr< ModelInstance > & | GetModelInstance () const |
get the ModelInstance we are attached to | |
const Ptr< ModelNode > & | GetModelNode () const |
get the ModelNode we're associated with | |
void | SetVisible (bool b, Timing::Time time, bool recursive=true) |
set the node instance's visibility | |
bool | IsVisible () const |
return true if node instance is set to visible | |
IndexT | GetModelNodeInstanceIndex () const |
get model node instance index for current frame | |
int | GetRefCount () const |
get the current refcount | |
void | AddRef () |
increment refcount by one | |
void | Release () |
decrement refcount and destroy object if refcount is zero | |
bool | IsInstanceOf (const Rtti &rtti) const |
return true if this object is instance of given class | |
bool | IsInstanceOf (const Util::String &className) const |
return true if this object is instance of given class by string | |
bool | IsInstanceOf (const Util::FourCC &classFourCC) const |
return true if this object is instance of given class by fourcc | |
bool | IsA (const Rtti &rtti) const |
return true if this object is instance of given class, or a derived class | |
bool | IsA (const Util::String &rttiName) const |
return true if this object is instance of given class, or a derived class, by string | |
bool | IsA (const Util::FourCC &rttiFourCC) const |
return true if this object is instance of given class, or a derived class, by fourcc | |
const Util::String & | GetClassName () const |
get the class name | |
Util::FourCC | GetClassFourCC () const |
get the class FourCC code | |
Static Public Member Functions | |
static void | DumpRefCountingLeaks () |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!) | |
Protected Member Functions | |
virtual void | Setup (const Ptr< Models::ModelInstance > &inst, const Ptr< Models::ModelNode > &node, const Ptr< Models::ModelNodeInstance > &parentNodeInst) |
called when attached to ModelInstance | |
virtual void | Discard () |
called when removed from ModelInstance | |
virtual void | RenderDebug () |
render node specific debug shape | |
virtual void | OnShow (Timing::Time time) |
called when the node becomes visible with current time | |
virtual void | OnHide (Timing::Time time) |
called when the node becomes invisible | |
void | SetParent (const Ptr< ModelNodeInstance > &p) |
set parent node | |
void | AddChild (const Ptr< ModelNodeInstance > &c) |
add a child node |
Member Function Documentation
void Models::ModelNodeInstance::DiscardHierarchy | ( | ) | [virtual, inherited] |
discard the model node instance and all of its children
Discards this model node instance and all of its children recursively.
bool Models::ModelNodeInstance::IsVisible | ( | ) | const [inherited] |
return true if node instance is set to visible
FIXME: The recursion in this is method makes it slow, especially in deep hierarchies. Also it can't be inlined.
int Core::RefCounted::GetRefCount | ( | ) | const [inline, inherited] |
get the current refcount
Return the current refcount of the object.
void Core::RefCounted::AddRef | ( | ) | [inline, inherited] |
increment refcount by one
Increment the refcount of the object.
void Core::RefCounted::Release | ( | ) | [inline, inherited] |
decrement refcount and destroy object if refcount is zero
Decrement the refcount and destroy object if refcount is zero.
const Util::String & Core::RefCounted::GetClassName | ( | ) | const [inline, inherited] |
get the class name
Get the class name of the object.
Util::FourCC Core::RefCounted::GetClassFourCC | ( | ) | const [inline, inherited] |
get the class FourCC code
Get the class FourCC of the object.
void Core::RefCounted::DumpRefCountingLeaks | ( | ) | [static, inherited] |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!)
This method should be called as the very last before an application exits.