Base::RenderTargetBase Class Reference
#include <rendertargetbase.h>
Inheritance diagram for Base::RenderTargetBase:
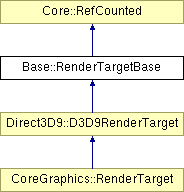
Detailed Description
Base class for render targets. A render targets wraps up to 4 color buffers and an optional depth/stencil buffer into a C++ object. The special default render target represents the backbuffer and default depth/stencil surface.(C) 2007 Radon Labs GmbH
Public Types | |
enum | ClearFlag |
clear flags | |
Public Member Functions | |
RenderTargetBase () | |
constructor | |
virtual | ~RenderTargetBase () |
destructor | |
bool | IsValid () const |
return true if valid (has been setup) | |
void | SetDefaultRenderTarget (bool b) |
set to true if default render target (only called by RenderDevice) | |
bool | IsDefaultRenderTarget () const |
get default render target flag | |
void | SetWidth (SizeT w) |
set render target width | |
SizeT | GetWidth () const |
get width of render target in pixels | |
void | SetHeight (SizeT h) |
set render target height | |
SizeT | GetHeight () const |
get height of render target in pixels | |
void | SetAntiAliasQuality (CoreGraphics::AntiAliasQuality::Code c) |
set antialias quality | |
CoreGraphics::AntiAliasQuality::Code | GetAntiAliasQuality () const |
get anti-alias-quality | |
void | SetColorBufferFormat (CoreGraphics::PixelFormat::Code colorFormat) |
add a color buffer | |
CoreGraphics::PixelFormat::Code | GetColorBufferFormat () const |
get color buffer format at index | |
void | AddDepthStencilBuffer () |
internally create a depth/stencil buffer | |
void | AddSharedDepthStencilBuffer (const Ptr< CoreGraphics::RenderTarget > &rt) |
use external depth-stencil buffer | |
bool | HasDepthStencilBuffer () const |
return true if the render target has a depth/stencil buffer | |
void | SetMipMapsEnabled (bool b) |
enable mipmap generation for this render target | |
bool | AreMipMapsEnabled () const |
get mipmap generation flag | |
void | SetResolveTextureResourceId (const Resources::ResourceId &resId) |
set resolve texture resource id | |
const Resources::ResourceId & | GetResolveTextureResourceId () const |
get resolve texture resource id | |
void | SetResolveDepthTextureResourceId (const Resources::ResourceId &resId) |
set optional resolve depth texture resource id | |
const Resources::ResourceId & | GetResolveDepthTextureResourceId () const |
get optional resolve depth texture resource id | |
void | SetResolveTextureWidth (SizeT w) |
set resolve texture width | |
SizeT | GetResolveTextureWidth () const |
get resolve texture width | |
void | SetResolveTextureHeight (SizeT h) |
set resolve texture height | |
SizeT | GetResolveTextureHeight () const |
get resolve texture height | |
void | SetResolveTargetCpuAccess (bool b) |
set cpu access flag | |
bool | GetResolveTargetCpuAccess () const |
get cpu access flag | |
void | SetMRTIndex (IndexT i) |
set optional MRT (Multiple Render Target) index, default is 0 | |
IndexT | GetMRTIndex () const |
get multiple-render-target index | |
void | SetClearFlags (uint clearFlags) |
set clear flags | |
uint | GetClearFlags () const |
get clear flags | |
void | SetClearColor (const Math::float4 &c) |
set clear color | |
const Math::float4 & | GetClearColor () const |
get clear color | |
void | SetClearDepth (float d) |
set clear depth | |
float | GetClearDepth () const |
get clear depth | |
void | SetClearStencil (uchar s) |
set clear stencil value | |
uchar | GetClearStencil () const |
get clear stencil value | |
void | SetResolveRect (const Math::rectangle< int > &r) |
set the current resolve rectangle (in pixels) | |
const Math::rectangle< int > & | GetResolveRect () const |
get resolve rectangle | |
void | Setup () |
setup the render target object | |
void | Discard () |
discard the render target object | |
void | BeginPass () |
begin rendering to the render target | |
void | BeginBatch (CoreGraphics::BatchType::Code batchType) |
begin a batch | |
void | EndBatch () |
end current batch | |
void | EndPass () |
end current render pass | |
void | GenerateMipLevels () |
generate mipmap levels | |
bool | HasResolveTexture () const |
return true if resolve texture is valid | |
const Ptr< CoreGraphics::Texture > & | GetResolveTexture () const |
get the resolve texture as Nebula texture object | |
bool | HasCPUResolveTexture () const |
return true if cpu access resolve texture is valid | |
const Ptr< CoreGraphics::Texture > & | GetCPUResolveTexture () const |
get the resolve texture as Nebula texture object | |
bool | HasDepthResolveTexture () const |
return true if resolve texture is valid | |
const Ptr< CoreGraphics::Texture > & | GetDepthResolveTexture () const |
get the resolve texture as Nebula texture object | |
virtual void | ResolveDepthBuffer () |
resolve depth buffer | |
SizeT | GetMemorySize () const |
get byte size in memory, implemented in platform specific classes | |
void | SetMemoryOffset (SizeT size) |
set optional memory offset, not used by all platforms | |
int | GetRefCount () const |
get the current refcount | |
void | AddRef () |
increment refcount by one | |
void | Release () |
decrement refcount and destroy object if refcount is zero | |
bool | IsInstanceOf (const Rtti &rtti) const |
return true if this object is instance of given class | |
bool | IsInstanceOf (const Util::String &className) const |
return true if this object is instance of given class by string | |
bool | IsInstanceOf (const Util::FourCC &classFourCC) const |
return true if this object is instance of given class by fourcc | |
bool | IsA (const Rtti &rtti) const |
return true if this object is instance of given class, or a derived class | |
bool | IsA (const Util::String &rttiName) const |
return true if this object is instance of given class, or a derived class, by string | |
bool | IsA (const Util::FourCC &rttiFourCC) const |
return true if this object is instance of given class, or a derived class, by fourcc | |
const Util::String & | GetClassName () const |
get the class name | |
Util::FourCC | GetClassFourCC () const |
get the class FourCC code | |
Static Public Member Functions | |
static void | DumpRefCountingLeaks () |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!) |
Member Function Documentation
int Core::RefCounted::GetRefCount | ( | ) | const [inline, inherited] |
get the current refcount
Return the current refcount of the object.
void Core::RefCounted::AddRef | ( | ) | [inline, inherited] |
increment refcount by one
Increment the refcount of the object.
void Core::RefCounted::Release | ( | ) | [inline, inherited] |
decrement refcount and destroy object if refcount is zero
Decrement the refcount and destroy object if refcount is zero.
const Util::String & Core::RefCounted::GetClassName | ( | ) | const [inline, inherited] |
get the class name
Get the class name of the object.
Util::FourCC Core::RefCounted::GetClassFourCC | ( | ) | const [inline, inherited] |
get the class FourCC code
Get the class FourCC of the object.
void Core::RefCounted::DumpRefCountingLeaks | ( | ) | [static, inherited] |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!)
This method should be called as the very last before an application exits.