Script::InfoLog Class Reference
#include <infolog.h>
Inheritance diagram for Script::InfoLog:
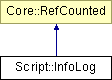
Detailed Description
To an info log object you can add several strings which can contain different information like debug information or error messages.You can also specify a log level, a short description and a source (e.g. information on the creator of the info log object).
Additionally you can open and close sections for a better seperation of general and detailed information. Within a section you can open new sections, too but there is no check if the amount of openings fits to the amount of closings. For further details see Description of BeginSection() and EndSection().
Per default description, source and the list of information strings are empty. The log level will be 'Non'.
An info log object is based on an array of strings.
(C) 2006 Radon Labs GmbH
Public Types | |
enum | LogLevel { Non = 0, Debug = (1<<0), Warning = (1<<1), Error = (1<<2), Graphic = (1<<3) } |
Public Member Functions | |
InfoLog () | |
constructor | |
virtual | ~InfoLog () |
destructor | |
void | SetDescription (Util::String description) |
sets the short description | |
const Util::String & | GetDescription () const |
gets the short description | |
void | SetSource (Util::String source) |
sets the source of this info log (e.g. creator) | |
const Util::String & | GetSource () const |
gets the source of this info log (e.g. creator) | |
void | SetLogLevel (LogLevel logLvl) |
sets the log level | |
LogLevel | GetLogLevel () const |
gets the log level | |
void | AddInfo (const Util::String &infoString) |
adds an information string to this info log | |
void | AddInfo (const Util::Array< Util::String > &infoArray) |
adds an array of information strings to this info log | |
const Util::Array< Util::String > & | GetInfo () const |
gets the list of information strings of this info log | |
void | BeginSection (const Util::String &beginInfo) |
starts new section | |
void | EndSection (const Util::String &endInfo) |
ends current section | |
bool | HasInfo () const |
returns true if information has been added | |
bool | HasInfoInCurrentSection () const |
returns true if information has been added to current section | |
Util::String | ToString () const |
returns a simple string representation of this info log | |
int | GetRefCount () const |
get the current refcount | |
void | AddRef () |
increment refcount by one | |
void | Release () |
decrement refcount and destroy object if refcount is zero | |
bool | IsInstanceOf (const Rtti &rtti) const |
return true if this object is instance of given class | |
bool | IsInstanceOf (const Util::String &className) const |
return true if this object is instance of given class by string | |
bool | IsInstanceOf (const Util::FourCC &classFourCC) const |
return true if this object is instance of given class by fourcc | |
bool | IsA (const Rtti &rtti) const |
return true if this object is instance of given class, or a derived class | |
bool | IsA (const Util::String &rttiName) const |
return true if this object is instance of given class, or a derived class, by string | |
bool | IsA (const Util::FourCC &rttiFourCC) const |
return true if this object is instance of given class, or a derived class, by fourcc | |
const Util::String & | GetClassName () const |
get the class name | |
Util::FourCC | GetClassFourCC () const |
get the class FourCC code | |
Static Public Member Functions | |
static Util::String | LogLevelToString (LogLevel logLvl) |
converts a log level to a string | |
static LogLevel | StringToLogLevel (const Util::String &logLevelString) |
converts a string to a log level | |
static void | DumpRefCountingLeaks () |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!) | |
Static Public Attributes | |
static const Util::String | BEGINLABEL |
label which is used to mark begin section tag | |
static const Util::String | ENDLABEL |
label which is used to mark end section tag |
Member Enumeration Documentation
A log level which specifies type or/and origin of the info log object. It's possible to combine different log levels by using bit operators.
Constructor & Destructor Documentation
Script::InfoLog::InfoLog | ( | ) |
constructor
constructor
Script::InfoLog::~InfoLog | ( | ) | [virtual] |
destructor
destructor
Member Function Documentation
void Script::InfoLog::SetDescription | ( | Util::String | description | ) |
sets the short description
Sets the description.
const Util::String & Script::InfoLog::GetDescription | ( | ) | const |
gets the short description
Gets the description.
void Script::InfoLog::SetSource | ( | Util::String | source | ) |
sets the source of this info log (e.g. creator)
Sets the source of this info log (e.g. creator).
const Util::String & Script::InfoLog::GetSource | ( | ) | const |
gets the source of this info log (e.g. creator)
Gets the source of this info log (e.g. creator).
void Script::InfoLog::SetLogLevel | ( | LogLevel | logLvl | ) |
sets the log level
Sets the log level. There will be no check if the log level is supported.
InfoLog::LogLevel Script::InfoLog::GetLogLevel | ( | ) | const |
gets the log level
Gets the log level.
void Script::InfoLog::AddInfo | ( | const Util::String & | infoString | ) |
adds an information string to this info log
Adds an information string to this info log.
void Script::InfoLog::AddInfo | ( | const Util::Array< Util::String > & | infoArray | ) |
adds an array of information strings to this info log
Adds an array of information strings to this info log.
const Util::Array< Util::String > & Script::InfoLog::GetInfo | ( | ) | const |
gets the list of information strings of this info log
Gets a list of information strings.
void Script::InfoLog::BeginSection | ( | const Util::String & | beginInfo | ) |
starts new section
Start new info log section.
The begin tags for open sections will be added to the list of information strings when the first information in this section has been added.
The begin section tag will have following format: "<InfoLog::BEGINLABEL><beginInfo>".
See EndSection(). See InfoLog::BEGINLABEL.
void Script::InfoLog::EndSection | ( | const Util::String & | endInfo | ) |
ends current section
End current info log section.
If there is a begin section tag without information the begin and the end section tags won't be added to the info log object. If there is a begin section tag with following information the end section tag will be added to the info log object.
The end section tag will always end the last open section tag, therefor to every begin section tag an end section tag should be placed. This method does not check if the amount of begin tags fits to the amount of end tags!
The end section tag will have following format: "<InfoLog::ENDLABEL><endInfo>"
See BeginSection(). See InfoLog::ENDLABEL.
bool Script::InfoLog::HasInfo | ( | ) | const |
returns true if information has been added
Returns true if information has been added.
bool Script::InfoLog::HasInfoInCurrentSection | ( | ) | const |
returns true if information has been added to current section
Returns true if information has been added to current section.
Util::String Script::InfoLog::ToString | ( | ) | const |
returns a simple string representation of this info log
Returns an simple string representation of the information strings. The begin and end labels will be removed and instead the inner sections will be indented.
Util::String Script::InfoLog::LogLevelToString | ( | InfoLog::LogLevel | logLvl | ) | [static] |
converts a log level to a string
Converts a log level to a string.
If there are set more than one bit of the log level the names of the log levels will be seperated by " | ". The string will be empty if no set bit of the log level correspond to a supported log level.
InfoLog::LogLevel Script::InfoLog::StringToLogLevel | ( | const Util::String & | logLevelString | ) | [static] |
converts a string to a log level
Converts a string to a log level.
This method uses a simple pattern matching. If the name of a log level is in the log level string the corresponding bit will be set.
It's not case sensitiv. Not supported log level names will be ignored. If there are no supported log levels the method returns log level "Non".
int Core::RefCounted::GetRefCount | ( | ) | const [inline, inherited] |
get the current refcount
Return the current refcount of the object.
void Core::RefCounted::AddRef | ( | ) | [inline, inherited] |
increment refcount by one
Increment the refcount of the object.
void Core::RefCounted::Release | ( | ) | [inline, inherited] |
decrement refcount and destroy object if refcount is zero
Decrement the refcount and destroy object if refcount is zero.
const Util::String & Core::RefCounted::GetClassName | ( | ) | const [inline, inherited] |
get the class name
Get the class name of the object.
Util::FourCC Core::RefCounted::GetClassFourCC | ( | ) | const [inline, inherited] |
get the class FourCC code
Get the class FourCC of the object.
void Core::RefCounted::DumpRefCountingLeaks | ( | ) | [static, inherited] |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!)
This method should be called as the very last before an application exits.