Script::DialogManager Class Reference
#include <dialogmanager.h>
Inheritance diagram for Script::DialogManager:
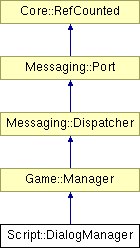
Detailed Description
Base funktions: TalkTo: load a dialog from DB, for a Speaker, and start it. CancelCurrentDialog: Cancel a currently running DialogManage Locked an Unlocked States of dialog, and allowes status request Manage if a Dialog Take was spoken, an save this data.
Over this Manager the flow of the current active dialog is controlled
A complete overview of the dialog system can be found here: http://gambar/wiki/index.php/DSA_Story_Subsystem
(C) 2006 Radon Labs GmbH
Public Member Functions | |
DialogManager () | |
constructor | |
virtual | ~DialogManager () |
destructor | |
virtual void | OnActivate () |
called when attached from game server | |
virtual void | OnDeactivate () |
called when removed from game server | |
virtual void | OnLoad () |
called after loading game state | |
virtual void | OnSave () |
called before saving game state | |
virtual void | SetupAcceptedMessages () |
override to register accepted messages | |
virtual void | HandleMessage (const Ptr< Messaging::Message > &msg) |
handle a single message | |
virtual Ptr< Script::Dialog > | CreateDialog () |
create a Dialog | |
virtual void | StartDialog (const Util::String &speaker) |
start a dialog for a given speaker | |
bool | HasDialogs (const Util::String &speaker, bool asConversation=false) const |
return true if the entity has dialogs assigned | |
void | TalkTo (const Util::String &speaker) |
initiate a dialog with an NPC | |
bool | IsDialogActive () const |
return true if a dialog is currently in progress | |
bool | IsDialogLoaded () const |
return true if a dialog is loaded | |
virtual void | CancelCurrentDialog () |
cancel the currently active dialog | |
bool | DialogExistsById (const Util::String &dialogId) const |
return true if a dialog exists by dialog id | |
bool | DialogTakeExistsById (const Util::String &dialogId, const Util::String &takeId) const |
return true if a dialogtake exists by take id | |
virtual const Util::String & | GetSpeaker () const |
returns the Speaker name | |
const Util::String & | GetDialogId () const |
returns the dialog id | |
const Util::Array< Util::String > & | GetDialogText () const |
get the Take-Text of the current dialog node | |
const Util::Array< Util::String > & | GetResponses () const |
get an array of texts filled with the response-texts of current dialog node | |
const Util::Array< Util::String > & | GetShortResponseTexts () const |
get short response text array | |
const Util::String & | GetTakeSoundID () const |
get the CueId of the current take | |
const Util::Array< Util::String > & | GetResponseSoundIDs () const |
get a array with the CueIDs of the current responses | |
const Util::String & | GetTakeSpeaker () const |
get the Speaker of the current take | |
const Util::Array< Util::String > & | GetResponseSpeakers () const |
get a array with the Speaker's of the current responses | |
const Util::Array< bool > & | GetResponsePassedStates () const |
gets a array with the passed state of the current responses | |
bool | ChooseResponse (int responseIndex) |
this function says the manager witch response is choosed by the user | |
void | SetDialogTakeState (const Util::String &dialogId, const Util::String &takeId, bool isPassed) |
set the state of a dialog take | |
bool | GetDialogTakeState (const Util::String &dialogId, const Util::String &takeId) |
get the state of a dialog take | |
void | SetDialogLockState (const Util::String &dialogId, bool locked) |
sets the locked flag of a dialog | |
bool | GetDialogLockState (const Util::String &dialogId) |
gets the locked flag of a dialog | |
void | SetLocalizeDialog (bool localize) |
set localize flag | |
Ptr< Script::Dialog > | GetDialog (const Util::String &speakerOrGroupName, bool loadGroupDialog, bool asConversation=false) |
get a dialog by speaker or group name | |
Ptr< Script::Dialog > | GetDialog (const Util::String &dialogId) |
get a dialog by id | |
bool | IsActive () const |
return true if currently active | |
virtual void | OnBeginFrame () |
called before frame by the game server | |
virtual void | OnFrame () |
called per-frame by the game server | |
virtual void | OnEndFrame () |
called after frame by the game server | |
virtual void | OnStart () |
called by Game::Server::Start() when the world is started | |
virtual void | OnRenderDebug () |
render a debug visualization | |
void | AttachPort (const Ptr< Port > &port) |
attach a message port | |
void | RemovePort (const Ptr< Port > &port) |
remove a message port | |
bool | HasPort (const Ptr< Port > &port) const |
return true if a port exists | |
void | AttachHandler (const Ptr< Handler > &h) |
attach a message handler to the port | |
void | RemoveHandler (const Ptr< Handler > &h) |
remove a message handler from the port | |
void | RemoveAllHandlers () |
remove all message handler from the port | |
SizeT | GetNumHandlers () const |
return number of handlers attached to the port | |
const Ptr< Handler > & | GetHandlerAtIndex (IndexT i) const |
get a message handler by index | |
virtual void | Send (const Ptr< Message > &msg) |
send a message to the port | |
const Util::Array< const Id * > & | GetAcceptedMessages () const |
get the array of accepted messages (sorted) | |
bool | AcceptsMessage (const Id &msgId) const |
return true if port accepts this msg | |
int | GetRefCount () const |
get the current refcount | |
void | AddRef () |
increment refcount by one | |
void | Release () |
decrement refcount and destroy object if refcount is zero | |
bool | IsInstanceOf (const Rtti &rtti) const |
return true if this object is instance of given class | |
bool | IsInstanceOf (const Util::String &className) const |
return true if this object is instance of given class by string | |
bool | IsInstanceOf (const Util::FourCC &classFourCC) const |
return true if this object is instance of given class by fourcc | |
bool | IsA (const Rtti &rtti) const |
return true if this object is instance of given class, or a derived class | |
bool | IsA (const Util::String &rttiName) const |
return true if this object is instance of given class, or a derived class, by string | |
bool | IsA (const Util::FourCC &rttiFourCC) const |
return true if this object is instance of given class, or a derived class, by fourcc | |
const Util::String & | GetClassName () const |
get the class name | |
Util::FourCC | GetClassFourCC () const |
get the class FourCC code | |
Static Public Member Functions | |
static void | DumpRefCountingLeaks () |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!) | |
Protected Member Functions | |
void | LoadDialogTakeStates () |
load the table DialogTakeState | |
void | SaveDialogTakeStates () |
save the table DialogTakeState | |
void | LoadDialogs () |
load the Dialog table with locked state | |
void | SaveDialogs () |
save the Dialog table with locked state | |
void | ValidateDialog (const Util::Guid &guid) |
make sure dialog by GUID is loaded | |
void | PreloadDialogTables () |
try preload tables through ScriptTemplateManager | |
virtual void | ExecuteTakeActions (const Ptr< DialogTake > &take) |
executes the actions of a given take | |
virtual void | PlayEmotes (const Ptr< DialogTake > &take) |
play the emotes of a given take.. override this | |
virtual void | PlaySound (const Ptr< DialogTake > &take, bool isResponse=false) |
play sound... override this | |
bool | LoadAssociatedDialogs (const Util::String &speaker, bool loadForGroup=false) |
load dialogs associated with an Speaker or group | |
void | UnloadDialog () |
unload currently loaded dialogs | |
virtual bool | ActivateDialog () |
activate the Dialog, dialog must beloaded before | |
void | AssertDialogTakeExists (const Util::String &dialogTakeKey, const Util::String &dialogId, const Util::String &takeId, const Util::String &callerName) |
assert that a dialog and take id exists | |
void | RegisterMessage (const Id &msgId) |
register a single accepted message |
Member Function Documentation
void Script::DialogManager::OnDeactivate | ( | ) | [virtual] |
called when removed from game server
This method shuts down the dialog manager when it is removed from the game server.
Reimplemented from Game::Manager.
void Script::DialogManager::StartDialog | ( | const Util::String & | speaker | ) | [virtual] |
start a dialog for a given speaker
Start a dialog for a given speaker
bool Script::DialogManager::HasDialogs | ( | const Util::String & | speakerName, | |
bool | asConversation = false | |||
) | const |
return true if the entity has dialogs assigned
This method checks if dialogs are assigned to an NPC at all.
void Script::DialogManager::TalkTo | ( | const Util::String & | speaker | ) |
initiate a dialog with an NPC
This method starts a dialog with an Speakername. If a previous dialog is in progress it will be canceled and unloaded.
bool Script::DialogManager::IsDialogActive | ( | ) | const |
return true if a dialog is currently in progress
This method returns true if a dialog is currently in progress.
bool Script::DialogManager::IsDialogLoaded | ( | ) | const |
return true if a dialog is loaded
This method returns true if a dialog is loaded
void Script::DialogManager::CancelCurrentDialog | ( | ) | [virtual] |
cancel the currently active dialog
This method cancels and unloads the currently active dialog
bool Script::DialogManager::DialogTakeExistsById | ( | const Util::String & | dialogId, | |
const Util::String & | takeId | |||
) | const |
return true if a dialogtake exists by take id
Return true if a dialogtake exists by take id.
const Util::String & Script::DialogManager::GetSpeaker | ( | ) | const [virtual] |
returns the Speaker name
Returns the speaker name of the currently active dialog.
const Util::String & Script::DialogManager::GetDialogId | ( | ) | const |
returns the dialog id
Returns the id of the currently active dialog
const Util::Array< Util::String > & Script::DialogManager::GetDialogText | ( | ) | const |
get the Take-Text of the current dialog node
Get the take texts of the current dialog node.
const Util::Array< Util::String > & Script::DialogManager::GetResponses | ( | ) | const |
get an array of texts filled with the response-texts of current dialog node
Get the response texts of the current dialog node.
bool Script::DialogManager::ChooseResponse | ( | int | responseIndex | ) |
this function says the manager witch response is choosed by the user
Tells the dialog manager which response has been choosen by the user.
void Script::DialogManager::SetDialogTakeState | ( | const Util::String & | dialogId, | |
const Util::String & | takeId, | |||
bool | isPassed | |||
) |
set the state of a dialog take
Set a take state to passed (when the take has been displayed).
bool Script::DialogManager::GetDialogTakeState | ( | const Util::String & | dialogId, | |
const Util::String & | takeId | |||
) |
get the state of a dialog take
Return the passed flag of a dialog take.
void Script::DialogManager::SetDialogLockState | ( | const Util::String & | dialogId, | |
bool | locked | |||
) |
sets the locked flag of a dialog
Set the locked flag of a dialog.
bool Script::DialogManager::GetDialogLockState | ( | const Util::String & | dialogId | ) |
gets the locked flag of a dialog
Set the locked flag of a dialog.
void Script::DialogManager::LoadDialogTakeStates | ( | ) | [protected] |
load the table DialogTakeState
Load dialog take state table from database.
void Script::DialogManager::SaveDialogTakeStates | ( | ) | [protected] |
save the table DialogTakeState
Save passed state flags of takes back into database.
void Script::DialogManager::LoadDialogs | ( | ) | [protected] |
load the Dialog table with locked state
Load the dialog table from the database.
void Script::DialogManager::SaveDialogs | ( | ) | [protected] |
save the Dialog table with locked state
Save dialog status back to database, only the locked flag can change, so only write this back into the database.
void Script::DialogManager::ValidateDialog | ( | const Util::Guid & | guid | ) | [protected] |
make sure dialog by GUID is loaded
Make sure a dialog is loaded by GUID.
void Script::DialogManager::PreloadDialogTables | ( | ) | [protected] |
try preload tables through ScriptTemplateManager
Preload tables from db via ScriptTemplateManager
void Script::DialogManager::ExecuteTakeActions | ( | const Ptr< DialogTake > & | take | ) | [protected, virtual] |
executes the actions of a given take
Executes the actions of a given take
void Script::DialogManager::PlayEmotes | ( | const Ptr< DialogTake > & | take | ) | [protected, virtual] |
play the emotes of a given take.. override this
play emotes for current speaker
void Script::DialogManager::PlaySound | ( | const Ptr< DialogTake > & | take, | |
bool | isResponse = false | |||
) | [protected, virtual] |
play sound... override this
play sound... override this
bool Script::DialogManager::LoadAssociatedDialogs | ( | const Util::String & | speakerOrGroupName, | |
bool | loadGroupDialog = false | |||
) | [protected] |
load dialogs associated with an Speaker or group
This private method loads the dialogtree associated with a given speaker or group from the database into the dialog manager. Returns true if at least one dialog has been activated. if loadGroupDialog is false, speaker will be loaded
- Parameters:
-
speakerOrGroup name of a speaker or a group loadGroupDialog if true dialog for given group name will be loaded
- Returns:
- true, a dialog was found in db
void Script::DialogManager::UnloadDialog | ( | ) | [protected] |
unload currently loaded dialogs
Unload the currently loaded dialog.
bool Script::DialogManager::ActivateDialog | ( | ) | [protected, virtual] |
activate the Dialog, dialog must beloaded before
Activate a currently loaded dialog.
void Script::DialogManager::AssertDialogTakeExists | ( | const Util::String & | dialogTakeKey, | |
const Util::String & | dialogId, | |||
const Util::String & | takeId, | |||
const Util::String & | callerName | |||
) | [protected] |
assert that a dialog and take id exists
Make sure that a dialog/take pair exists.
void Game::Manager::OnBeginFrame | ( | ) | [virtual, inherited] |
called before frame by the game server
Called before frame, override in subclasses
Reimplemented in BaseGameFeature::EntityManager.
void Game::Manager::OnEndFrame | ( | ) | [virtual, inherited] |
called after frame by the game server
Called after frame, override in subclasses
Reimplemented in BaseGameFeature::EntityManager.
attach a message port
Attach a new message port.
- Parameters:
-
port pointer to a message port object
remove a message port
Remove a message port object.
- Parameters:
-
handler pointer to message port object to be removed
return true if a port exists
Return true if a port is already attached.
attach a message handler to the port
Attach a message handler to the port.
remove a message handler from the port
Remove a message handler from the port.
send a message to the port
Send a message to the port. This will immediately call the HandleMessage() method of all attached handlers. If the message has been handled by at least one of the handlers, the Handled() flag of the message will be set to true.
int Core::RefCounted::GetRefCount | ( | ) | const [inline, inherited] |
get the current refcount
Return the current refcount of the object.
void Core::RefCounted::AddRef | ( | ) | [inline, inherited] |
increment refcount by one
Increment the refcount of the object.
void Core::RefCounted::Release | ( | ) | [inline, inherited] |
decrement refcount and destroy object if refcount is zero
Decrement the refcount and destroy object if refcount is zero.
const Util::String & Core::RefCounted::GetClassName | ( | ) | const [inline, inherited] |
get the class name
Get the class name of the object.
Util::FourCC Core::RefCounted::GetClassFourCC | ( | ) | const [inline, inherited] |
get the class FourCC code
Get the class FourCC of the object.
void Core::RefCounted::DumpRefCountingLeaks | ( | ) | [static, inherited] |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!)
This method should be called as the very last before an application exits.