Messaging::BlockingHandlerThread Class Reference
#include <blockinghandlerthread.h>
Inheritance diagram for Messaging::BlockingHandlerThread:
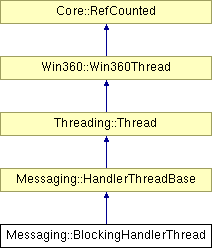
Detailed Description
Message handler thread class which blocks until messages arrive (or optionally, a time-out occurs).(C) 2009 Radon Labs GmbH
Public Types | |
enum | Priority |
thread priorities | |
Public Member Functions | |
BlockingHandlerThread () | |
constructor | |
void | SetWaitTimeout (int milliSec) |
set optional wait timeout (0 if infinite) | |
int | GetWaitTimeout () const |
get wait timeout | |
virtual void | AddMessage (const Ptr< Message > &msg) |
add a message to be handled (override in subclass!) | |
virtual void | CancelMessage (const Ptr< Message > &msg) |
cancel a pending message (override in subclass!) | |
virtual void | EmitWakeupSignal () |
called if thread needs a wakeup call before stopping | |
virtual void | DoWork () |
this method runs in the thread context | |
void | AttachHandler (const Ptr< Handler > &h) |
attach a message handler | |
void | RemoveHandler (const Ptr< Handler > &h) |
dynamically remove a message handler | |
void | ClearHandlers () |
clear all attached message handlers | |
void | WaitForHandlersOpened () |
wait until handlers have been opened | |
virtual void | WaitForMessage (const Ptr< Message > &msg) |
wait for message to be handled (optionally override in subclass!) | |
void | SetPriority (Priority p) |
set the thread priority | |
Priority | GetPriority () const |
get the thread priority | |
void | SetCoreId (System::Cpu::CoreId coreId) |
set cpu core on which the thread should be running | |
System::Cpu::CoreId | GetCoreId () const |
get the cpu core on which the thread should be running | |
void | SetStackSize (SizeT s) |
set stack size in bytes (default is 4 KByte) | |
SizeT | GetStackSize () const |
get stack size | |
void | SetName (const Util::String &n) |
set thread name | |
const Util::String & | GetName () const |
get thread name | |
void | Start () |
start executing the thread code, returns when thread has actually started | |
void | Stop () |
request threading code to stop, returns when thread has actually finished | |
bool | IsRunning () const |
return true if thread has been started | |
int | GetRefCount () const |
get the current refcount | |
void | AddRef () |
increment refcount by one | |
void | Release () |
decrement refcount and destroy object if refcount is zero | |
bool | IsInstanceOf (const Rtti &rtti) const |
return true if this object is instance of given class | |
bool | IsInstanceOf (const Util::String &className) const |
return true if this object is instance of given class by string | |
bool | IsInstanceOf (const Util::FourCC &classFourCC) const |
return true if this object is instance of given class by fourcc | |
bool | IsA (const Rtti &rtti) const |
return true if this object is instance of given class, or a derived class | |
bool | IsA (const Util::String &rttiName) const |
return true if this object is instance of given class, or a derived class, by string | |
bool | IsA (const Util::FourCC &rttiFourCC) const |
return true if this object is instance of given class, or a derived class, by fourcc | |
const Util::String & | GetClassName () const |
get the class name | |
Util::FourCC | GetClassFourCC () const |
get the class FourCC code | |
Static Public Member Functions | |
static void | YieldThread () |
yield the thread (gives up current time slice) | |
static void | SetMyThreadName (const char *n) |
set thread name from within thread context | |
static const char * | GetMyThreadName () |
obtain name of thread from within thread context | |
static Threading::ThreadId | GetMyThreadId () |
get the thread ID of this thread | |
static void | DumpRefCountingLeaks () |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!) | |
Protected Member Functions | |
void | ThreadOpenHandlers () |
open message handlers | |
void | ThreadCloseHandlers () |
close message handlers | |
void | ThreadUpdateHandlers () |
open dynamically added handlers, and call DoWork() on all attached handlers | |
bool | ThreadUpdateDeferredMessages () |
update deferred messages, return true if at least one message has been handled | |
void | ThreadDiscardDeferredMessages () |
clear leftover deferred messages | |
bool | ThreadHandleMessages (const Util::Array< Ptr< Message > > &msgArray) |
handle messages in array, return true if at least one message has been handled | |
bool | ThreadHandleSingleMessage (const Ptr< Message > &msg) |
handle a single message without deferred support, return if message has been handled | |
void | ThreadSignalMessageHandled () |
signal message handled event (only call if at least one message has been handled) | |
bool | ThreadStopRequested () const |
check if stop is requested, call from DoWork() to see if the thread proc should quit |
Member Function Documentation
add a message to be handled (override in subclass!)
This adds a new message to the thread's message queue.
Reimplemented from Messaging::HandlerThreadBase.
cancel a pending message (override in subclass!)
This removes a message from the thread's message queue, regardless of its state.
Reimplemented from Messaging::HandlerThreadBase.
void Messaging::BlockingHandlerThread::EmitWakeupSignal | ( | ) | [virtual] |
called if thread needs a wakeup call before stopping
This method is called by Thread::Stop() after setting the stopRequest event and before waiting for the thread to stop.
Reimplemented from Win360::Win360Thread.
void Messaging::BlockingHandlerThread::DoWork | ( | ) | [virtual] |
this method runs in the thread context
The message processing loop.
Reimplemented from Win360::Win360Thread.
attach a message handler
Attach a message handler to the port. This method may be called from any thread.
dynamically remove a message handler
Remove a message handler. This method may be called form any thread.
void Messaging::HandlerThreadBase::ClearHandlers | ( | ) | [inherited] |
clear all attached message handlers
This clears all attached message handlers.
void Messaging::HandlerThreadBase::WaitForHandlersOpened | ( | ) | [inherited] |
wait until handlers have been opened
Wait on the handlers-opened event (will be signalled by the ThreadOpenHandlers method.
void Messaging::HandlerThreadBase::WaitForMessage | ( | const Ptr< Message > & | msg | ) | [virtual, inherited] |
wait for message to be handled (optionally override in subclass!)
This waits until the message given as argument has been handled. In order for this message to work, the ThreadSignalMessageHandled() must be called from within the handler thread context. Note that subclasses may override this method if they need to.
Reimplemented in FrameSync::FrameSyncHandlerThread.
void Messaging::HandlerThreadBase::ThreadOpenHandlers | ( | ) | [protected, inherited] |
open message handlers
Open attached message handlers. This method must be called at the start of the handler thread.
void Messaging::HandlerThreadBase::ThreadCloseHandlers | ( | ) | [protected, inherited] |
close message handlers
Close attached message handlers. This method must be called right before the handler thread shuts down.
void Messaging::HandlerThreadBase::ThreadUpdateHandlers | ( | ) | [protected, inherited] |
bool Messaging::HandlerThreadBase::ThreadUpdateDeferredMessages | ( | ) | [protected, inherited] |
update deferred messages, return true if at least one message has been handled
This checks every message in the deferred message array whether it has been handled yet, if yes, the message's actual handled flag will be set, and the message will be removed from the deferred handled array. If at least one message has been handled, the method will return true, if no message has been handled, the method returns false. If message have been handled, don't forget to call ThreadSignalMessageHandled() later!
void Messaging::HandlerThreadBase::ThreadDiscardDeferredMessages | ( | ) | [protected, inherited] |
clear leftover deferred messages
This clears any leftover deferred messages. Call right before shutdown of the handler thread.
bool Messaging::HandlerThreadBase::ThreadHandleMessages | ( | const Util::Array< Ptr< Message > > & | msgArray | ) | [protected, inherited] |
handle messages in array, return true if at least one message has been handled
Handle all message in the provided message array. Supports batched and deferred messages. Calls ThreadHandleSingleMessage(). If at least one message has been handled, the method returns true.
bool Messaging::HandlerThreadBase::ThreadHandleSingleMessage | ( | const Ptr< Message > & | msg | ) | [protected, inherited] |
handle a single message without deferred support, return if message has been handled
Handle a single message, called by ThreadHandleMessages(). Return true if message has been handled. This method MUST be called from ThreadHandleMessages(), since this method will not explicitely take the handlers array critical section.
void Messaging::HandlerThreadBase::ThreadSignalMessageHandled | ( | ) | [protected, inherited] |
signal message handled event (only call if at least one message has been handled)
Signal the message-handled flag. Call this method once per handler-loop if either ThreadUpdateDeferredMessages or ThreadHandleMessages returns true!
void Win360::Win360Thread::SetName | ( | const Util::String & | n | ) | [inline, inherited] |
set thread name
Set the thread's name. To obtain the current thread's name from anywhere in the thread's execution context, call the static method Thread::GetMyThreadName().
const Util::String & Win360::Win360Thread::GetName | ( | ) | const [inline, inherited] |
get thread name
Get the thread's name. This is the vanilla method which returns the name member. To obtain the current thread's name from anywhere in the thread's execution context, call the static method Thread::GetMyThreadName().
void Win360::Win360Thread::Start | ( | ) | [inherited] |
void Win360::Win360Thread::Stop | ( | ) | [inherited] |
request threading code to stop, returns when thread has actually finished
This stops the thread by signalling the stopRequestEvent and waits for the thread to actually quit. If the thread code runs in a loop it should use the IsStopRequested() method to see if the thread object wants it to shutdown. If so DoWork() should simply return.
Reimplemented in Jobs::TPWorkerThread.
bool Win360::Win360Thread::IsRunning | ( | ) | const [inherited] |
return true if thread has been started
Returns true if the thread is currently running.
void Win360::Win360Thread::YieldThread | ( | ) | [static, inherited] |
yield the thread (gives up current time slice)
The yield function is empty on Win32 and Xbox360.
void Win360::Win360Thread::SetMyThreadName | ( | const char * | n | ) | [static, inherited] |
set thread name from within thread context
Static method which sets the name of this thread. This is called from within ThreadProc. The string pointed to must remain valid until the thread is terminated!
const char * Win360::Win360Thread::GetMyThreadName | ( | ) | [static, inherited] |
obtain name of thread from within thread context
Static method to obtain the current thread name from anywhere in the thread's code.
Threading::ThreadId Win360::Win360Thread::GetMyThreadId | ( | ) | [static, inherited] |
get the thread ID of this thread
Static method which returns the ThreadId of this thread.
bool Win360::Win360Thread::ThreadStopRequested | ( | ) | const [inline, protected, inherited] |
check if stop is requested, call from DoWork() to see if the thread proc should quit
If the derived DoWork() method is running in a loop it must regularly check if the process wants the thread to terminate by calling ThreadStopRequested() and simply return if the result is true. This will cause the thread to shut down.
int Core::RefCounted::GetRefCount | ( | ) | const [inline, inherited] |
get the current refcount
Return the current refcount of the object.
void Core::RefCounted::AddRef | ( | ) | [inline, inherited] |
increment refcount by one
Increment the refcount of the object.
void Core::RefCounted::Release | ( | ) | [inline, inherited] |
decrement refcount and destroy object if refcount is zero
Decrement the refcount and destroy object if refcount is zero.
const Util::String & Core::RefCounted::GetClassName | ( | ) | const [inline, inherited] |
get the class name
Get the class name of the object.
Util::FourCC Core::RefCounted::GetClassFourCC | ( | ) | const [inline, inherited] |
get the class FourCC code
Get the class FourCC of the object.
void Core::RefCounted::DumpRefCountingLeaks | ( | ) | [static, inherited] |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!)
This method should be called as the very last before an application exits.