Animation::PlayClipJob Class Reference
#include <playclipjob.h>
Inheritance diagram for Animation::PlayClipJob:
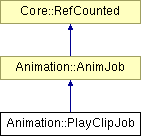
Detailed Description
An AnimJob which simply plays an animation clip.(C) 2008 Radon Labs GmbH
Public Member Functions | |
PlayClipJob () | |
constructor | |
virtual | ~PlayClipJob () |
destructor | |
void | SetClipName (const Util::StringAtom &clipName) |
set the anim clip name to play | |
const Util::StringAtom & | GetClipName () const |
get the anim clip name | |
void | SetLoopCount (float loopCount) |
set the loop count (overrides duration) | |
float | GetLoopCount () const |
get the loop count | |
void | SetName (const Util::StringAtom &id) |
set human readable name (only used for debugging) | |
const Util::StringAtom & | GetName () const |
get human readable name (only used for debugging) | |
bool | IsAttachedToSequencer () const |
return true if the job is currently attached to a sequencer | |
bool | IsActive (Timing::Tick time) const |
return true if the job has currently playing (EvalTime within start/end time) | |
bool | IsPending (Timing::Tick time) const |
return true if the job has been queued for playback but has not started yet | |
bool | IsStoppingOrExpired (Timing::Tick time) const |
return true if anim job is stopping or expired | |
bool | IsExpired (Timing::Tick time) const |
return true when the job has expired | |
void | SetTrackIndex (IndexT trackIndex) |
set track index, defines blend priority and relationship to other jobs on same track | |
IndexT | GetTrackIndex () const |
get track index | |
void | SetEnqueueMode (AnimJobEnqueueMode::Code enqueueMode) |
set the enqueue behaviour of the new job (default is intercept) | |
AnimJobEnqueueMode::Code | GetEnqueueMode () const |
get the enqueue behaviour of the new job | |
void | SetExclusiveTag (IndexT id) |
exclusive tag (for AnimJobEnqueueMode::IgnoreIfSameExclTagActive) | |
IndexT | GetExclusiveTag () const |
exclusive flag set? | |
void | SetStartTime (Timing::Tick time) |
set the start time of the anim job (relativ to base time) | |
Timing::Tick | GetStartTime () const |
get the start time of the anim job (relative to base time) | |
void | SetDuration (Timing::Tick time) |
set the duration of the anim job (0 == infinite) | |
Timing::Tick | GetDuration () const |
get the duration of the anim job | |
bool | IsInfinite () const |
return true if the anim job is infinite | |
void | SetFadeInTime (Timing::Tick fadeInTime) |
set the fade-in time of the anim job | |
Timing::Tick | GetFadeInTime () const |
get the fade-in time of the anim job | |
void | SetFadeOutTime (Timing::Tick fadeOutTime) |
set the fade-out time of the anim job | |
Timing::Tick | GetFadeOutTime () const |
get the fade-out time of the anim job | |
void | SetTimeFactor (float timeFactor) |
set time factor | |
float | GetTimeFactor () const |
get time factor | |
void | SetTimeOffset (Timing::Tick timeOffset) |
set sample time offset (if sampling should not start at the beginning) | |
Timing::Tick | GetTimeOffset () const |
get sample time offset | |
void | SetBlendWeight (float w) |
set blend weight of the anim job (default is 1.0) | |
float | GetBlendWeight () const |
get blend weight of the anim job | |
Timing::Tick | GetAbsoluteStartTime () const |
get the absolute start time (BaseTime + StartTime) | |
Timing::Tick | GetAbsoluteEndTime () const |
get the absolute end time (BaseTime + StartTime + Duration) | |
Timing::Tick | GetAbsoluteStopTime () const |
get the absolute, computed end time ((BaseTime + StartTime + Duration) - FadeOutTime) | |
int | GetRefCount () const |
get the current refcount | |
void | AddRef () |
increment refcount by one | |
void | Release () |
decrement refcount and destroy object if refcount is zero | |
bool | IsInstanceOf (const Rtti &rtti) const |
return true if this object is instance of given class | |
bool | IsInstanceOf (const Util::String &className) const |
return true if this object is instance of given class by string | |
bool | IsInstanceOf (const Util::FourCC &classFourCC) const |
return true if this object is instance of given class by fourcc | |
bool | IsA (const Rtti &rtti) const |
return true if this object is instance of given class, or a derived class | |
bool | IsA (const Util::String &rttiName) const |
return true if this object is instance of given class, or a derived class, by string | |
bool | IsA (const Util::FourCC &rttiFourCC) const |
return true if this object is instance of given class, or a derived class, by fourcc | |
const Util::String & | GetClassName () const |
get the class name | |
Util::FourCC | GetClassFourCC () const |
get the class FourCC code | |
Static Public Member Functions | |
static void | DumpRefCountingLeaks () |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!) | |
Protected Member Functions | |
void | SetBaseTime (Timing::Tick time) |
set the base time of the anim job (set by sequencer when job is attached) | |
Timing::Tick | GetBaseTime () const |
get the base time of the anim job | |
virtual void | OnRemoveFromSequencer () |
called when removed from sequencer | |
float | ComputeBlendWeight (Timing::Tick relEvalTime) const |
compute current blend weight, this should take fade-in into account | |
void | FixFadeTimes () |
fix fade-in/fade-out times if the sum is bigger then the play duration | |
virtual void | UpdateTimes (Timing::Tick time) |
compute sample time for next evaluation, always done, also if character isn't visible and no evaluation takes place | |
virtual void | Stop (Timing::Tick time) |
stop the anim job at the given time |
Member Function Documentation
bool Animation::AnimJob::IsActive | ( | Timing::Tick | time | ) | const [inherited] |
return true if the job has currently playing (EvalTime within start/end time)
This method will return true if the current eval time is between the start time and end time of the anim job.
bool Animation::AnimJob::IsPending | ( | Timing::Tick | time | ) | const [inherited] |
return true if the job has been queued for playback but has not started yet
This method will return true as long as the current eval time is before the start time (the job hasn't started yet).
bool Animation::AnimJob::IsStoppingOrExpired | ( | Timing::Tick | time | ) | const [inherited] |
return true if anim job is stopping or expired
Return true if the anim job is currently in or after the fade-out phase.
bool Animation::AnimJob::IsExpired | ( | Timing::Tick | time | ) | const [inherited] |
return true when the job has expired
This method will return true if the current eval time is greater then the end time of the job.
Timing::Tick Animation::AnimJob::GetAbsoluteStartTime | ( | ) | const [inherited] |
get the absolute start time (BaseTime + StartTime)
Returns the absolute start time (BaseTime + StartTime).
Timing::Tick Animation::AnimJob::GetAbsoluteEndTime | ( | ) | const [inherited] |
get the absolute end time (BaseTime + StartTime + Duration)
Return the absolute end time (BaseTime + StartTime + Duration). Method fails hard if AnimJob is infinite.
Timing::Tick Animation::AnimJob::GetAbsoluteStopTime | ( | ) | const [inherited] |
get the absolute, computed end time ((BaseTime + StartTime + Duration) - FadeOutTime)
Returns the absolute end time before the fadeout-phase starts ((BaseTime + StartTime + Duration) - FadeOut)
float Animation::AnimJob::ComputeBlendWeight | ( | Timing::Tick | relEvalTime | ) | const [protected, inherited] |
compute current blend weight, this should take fade-in into account
This is a helper method for subclasses and returns the current blend weight for the current relative evaluation time, taking the fade-in and fade-out phases into account.
void Animation::AnimJob::FixFadeTimes | ( | ) | [protected, inherited] |
fix fade-in/fade-out times if the sum is bigger then the play duration
This method checks if the fade-in plus the fade-out times would be bigger then the play-duration, if yes it will fix the fade times in order to prevent "blend-popping".
void Animation::AnimJob::UpdateTimes | ( | Timing::Tick | time | ) | [protected, virtual, inherited] |
compute sample time for next evaluation, always done, also if character isn't visible and no evaluation takes place
Updates evaluation times. Must be done every frame, even if character is not visible and so animjob is not evaluated. FIXME: INVISIBLE OBJECTS SHOULD NEVER UPDATE THEIR ANIMATION OR ANIM EVENTS!
void Animation::AnimJob::Stop | ( | Timing::Tick | time | ) | [protected, virtual, inherited] |
stop the anim job at the given time
Stop the anim job at the given time. This will just update the duration member.
int Core::RefCounted::GetRefCount | ( | ) | const [inline, inherited] |
get the current refcount
Return the current refcount of the object.
void Core::RefCounted::AddRef | ( | ) | [inline, inherited] |
increment refcount by one
Increment the refcount of the object.
void Core::RefCounted::Release | ( | ) | [inline, inherited] |
decrement refcount and destroy object if refcount is zero
Decrement the refcount and destroy object if refcount is zero.
const Util::String & Core::RefCounted::GetClassName | ( | ) | const [inline, inherited] |
get the class name
Get the class name of the object.
Util::FourCC Core::RefCounted::GetClassFourCC | ( | ) | const [inline, inherited] |
get the class FourCC code
Get the class FourCC of the object.
void Core::RefCounted::DumpRefCountingLeaks | ( | ) | [static, inherited] |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!)
This method should be called as the very last before an application exits.