Base::JobBase Class Reference
#include <jobbase.h>
Inheritance diagram for Base::JobBase:
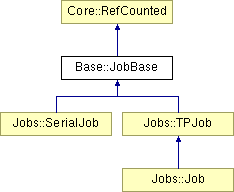
Detailed Description
Job objects are asynchronous data crunchers.A Job is setup from the following components:
- an input data buffer
- an output data buffer
- an uniform data buffer
- the job function
The job function will perform parallel processing of input data into the output buffer. The input data will be split into independent slices and slices will be processed in parallel. The uniform data is identical for every slice and can be used to pass additional arguments to the job function.
(C) 2009 Radon Labs GmbH
Public Member Functions | |
JobBase () | |
constructor | |
virtual | ~JobBase () |
destructor | |
void * | AllocPrivateBuffer (Memory::HeapType heapType, SizeT size) |
allocate a single memory buffer associated with the job, may only be called once, and before Setup()! | |
void * | GetPrivateBuffer () const |
get pointer to job's optional private buffer | |
SizeT | GetPrivateBufferSize () const |
get size of job's optional private buffer | |
void | Setup (const Jobs::JobUniformDesc &uniformDesc, const Jobs::JobDataDesc &inputDesc, const Jobs::JobDataDesc &outputDesc, const Jobs::JobFuncDesc &funcDesc) |
setup the job | |
void | Discard () |
discard the job | |
bool | IsValid () const |
return true if job has been setup | |
void | PatchInputDesc (const Jobs::JobDataDesc &inputDesc) |
patch input pointers after job has been setup | |
void | PatchOutputDesc (const Jobs::JobDataDesc &outputDesc) |
patch output pointers after job has been setup | |
void | PatchUniformDesc (const Jobs::JobUniformDesc &uniformDesc) |
patch uniform pointer after job has been setup | |
const Jobs::JobUniformDesc & | GetUniformDesc () const |
get uniform data descriptor | |
const Jobs::JobDataDesc & | GetInputDesc () const |
get input data descriptor | |
const Jobs::JobDataDesc & | GetOutputDesc () const |
get output data descriptor | |
const Jobs::JobFuncDesc & | GetFuncDesc () const |
get function descriptor | |
int | GetRefCount () const |
get the current refcount | |
void | AddRef () |
increment refcount by one | |
void | Release () |
decrement refcount and destroy object if refcount is zero | |
bool | IsInstanceOf (const Rtti &rtti) const |
return true if this object is instance of given class | |
bool | IsInstanceOf (const Util::String &className) const |
return true if this object is instance of given class by string | |
bool | IsInstanceOf (const Util::FourCC &classFourCC) const |
return true if this object is instance of given class by fourcc | |
bool | IsA (const Rtti &rtti) const |
return true if this object is instance of given class, or a derived class | |
bool | IsA (const Util::String &rttiName) const |
return true if this object is instance of given class, or a derived class, by string | |
bool | IsA (const Util::FourCC &rttiFourCC) const |
return true if this object is instance of given class, or a derived class, by fourcc | |
const Util::String & | GetClassName () const |
get the class name | |
Util::FourCC | GetClassFourCC () const |
get the class FourCC code | |
Static Public Member Functions | |
static void | DumpRefCountingLeaks () |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!) | |
Static Public Attributes | |
static const SizeT | MaxSliceSize = JobMaxSliceSize |
max size of a data slice is 16 kByte - 1 byte |
Member Function Documentation
void * Base::JobBase::AllocPrivateBuffer | ( | Memory::HeapType | heapType, | |
SizeT | size | |||
) |
allocate a single memory buffer associated with the job, may only be called once, and before Setup()!
This method can be used to allocate a single memory buffer, owned by the job object. The method must be called before Setup(), and will remain valid until the destructor of the job object is call (so it will survive a Discard()!).
int Core::RefCounted::GetRefCount | ( | ) | const [inline, inherited] |
get the current refcount
Return the current refcount of the object.
void Core::RefCounted::AddRef | ( | ) | [inline, inherited] |
increment refcount by one
Increment the refcount of the object.
void Core::RefCounted::Release | ( | ) | [inline, inherited] |
decrement refcount and destroy object if refcount is zero
Decrement the refcount and destroy object if refcount is zero.
const Util::String & Core::RefCounted::GetClassName | ( | ) | const [inline, inherited] |
get the class name
Get the class name of the object.
Util::FourCC Core::RefCounted::GetClassFourCC | ( | ) | const [inline, inherited] |
get the class FourCC code
Get the class FourCC of the object.
void Core::RefCounted::DumpRefCountingLeaks | ( | ) | [static, inherited] |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!)
This method should be called as the very last before an application exits.