Resources::ResourceManager Class Reference
#include <resourcemanager.h>
Inheritance diagram for Resources::ResourceManager:
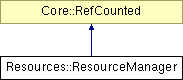
Detailed Description
The ResourceManager adds a management layer between resource using clients and actual resource objects. The main purpose of the manager is resource streaming for large seamless worlds. Resource users request a ManagedResource object from the ResourceManager. A ManagedResource is a wrapper around an actual Resource object, which may change based on the resource management strategy implemented by the manager. The main job of a resource manager is to provide all the resource required for rendering while making the best use of limited resource memory. It will also care about background loading of resources, and provide placeholder resources if a resource hasn't finished loading.While ManagedResources are managed by their appropriate Mappers unmanaged resources are externally created and a reference is stored by the ResourceManager. Primarily these are RenderTargets or other Resources which are 'never' thrown away.
The actual resource management strategies for different resource types are customizable by attaching ResourceMapper objects to the ResourceManager. A ResourceMapper analyzes the usage statistics of existing ManagedResource objects and implements a specific resource management pattern using the following basic operations:
- Load(pri, lod): asynchronously load a resource from external storage into memory given a priority and a level-of-detail.
- Discard: completely unload a resource, freeing up limited resource memory.
- Upgrade(lod): upgrade a loaded resource to a higher level-of-detail
- Degrade(lod): degrade a loaded resource to a lower level-of-detail
If ResourceMapper is a subclass of StreamingResourceMapper a certain ResourceScheduler can be attached on the fly to change management strategy any time.
(C) 2007 Radon Labs GmbH
Public Member Functions | |
ResourceManager () | |
constructor | |
virtual | ~ResourceManager () |
destructor | |
void | Open () |
open the resource manager | |
void | Close () |
close the resource manager | |
bool | IsOpen () const |
return true if resource manager is open | |
void | AttachMapper (const Ptr< ResourceMapper > &mapper) |
register a resource mapper (resource type is defined by mapper) | |
void | RemoveMapper (const Core::Rtti &resourceType) |
unregister a resource mapper by resource type | |
void | RemoveAllMappers () |
unregister all mappers | |
bool | HasMapper (const Core::Rtti &resourceType) const |
return true if a mapper has been registered for the given resource type | |
const Ptr< ResourceMapper > & | GetMapperByResourceType (const Core::Rtti &resourceType) const |
get the resource mapper registered with a resource type | |
Ptr< ManagedResource > | CreateManagedResource (const Core::Rtti &resType, const ResourceId &id, const Ptr< ResourceLoader > &optResourceLoader=0) |
create a ManagedResource object (bumps usecount on existing resource) | |
void | RequestResourceForLoading (const Ptr< ManagedResource > &managedResource) |
reloads an unloaded resource into cache | |
void | DiscardManagedResource (const Ptr< ManagedResource > &managedResource) |
unregister a ManagedResource object | |
bool | HasManagedResource (const ResourceId &id) const |
return true if a managed resource exists | |
const Ptr< ManagedResource > & | LookupManagedResource (const ResourceId &id) const |
lookup a managed resource (does not change usecount of resource) | |
void | AutoManageManagedResource (const ResourceId &id, bool autoManage) |
set if given resource whether should be autoManaged or not | |
void | Prepare (bool waiting) |
prepare stats gathering, call per frame | |
void | Update (IndexT frameIdx) |
perform actual resource management, call per frame | |
bool | CheckPendingResources () |
test if any resources are pending, returns true if not resources are pending | |
bool | WaitForPendingResources (Timing::Time timeOut) |
wait until pending resources are loaded, or time-out is reached (returns false if time-out) | |
Ptr< Resource > | CreateUnmanagedResource (const ResourceId &resId, const Core::Rtti &resClass, const Ptr< ResourceLoader > &loader=0, const Ptr< ResourceSaver > &saver=0) |
void | RegisterUnmanagedResource (const Ptr< Resource > &res) |
register an existing resource object as shared resource | |
void | UnregisterUnmanagedResource (const Ptr< Resource > &res) |
unregister a shared resource (necessary for managing the use count) | |
void | UnregisterUnmanagedResource (const ResourceId &id) |
unregister a shared resource by resource name | |
void | HoldResources () |
increments use count of all resources | |
void | ReleaseResources () |
decrements use count of all resources | |
bool | HasResource (const ResourceId &id) const |
return true if a shared resource exists | |
const Ptr< Resource > & | LookupResource (const ResourceId &id) const |
lookup a shared resource | |
Util::Array< Ptr< Resource > > | GetResourcesByType (const Core::Rtti &type) const |
get shared resources by type (slow) | |
int | GetRefCount () const |
get the current refcount | |
void | AddRef () |
increment refcount by one | |
void | Release () |
decrement refcount and destroy object if refcount is zero | |
bool | IsInstanceOf (const Rtti &rtti) const |
return true if this object is instance of given class | |
bool | IsInstanceOf (const Util::String &className) const |
return true if this object is instance of given class by string | |
bool | IsInstanceOf (const Util::FourCC &classFourCC) const |
return true if this object is instance of given class by fourcc | |
bool | IsA (const Rtti &rtti) const |
return true if this object is instance of given class, or a derived class | |
bool | IsA (const Util::String &rttiName) const |
return true if this object is instance of given class, or a derived class, by string | |
bool | IsA (const Util::FourCC &rttiFourCC) const |
return true if this object is instance of given class, or a derived class, by fourcc | |
const Util::String & | GetClassName () const |
get the class name | |
Util::FourCC | GetClassFourCC () const |
get the class FourCC code | |
Static Public Member Functions | |
static void | DumpRefCountingLeaks () |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!) |
Member Function Documentation
Ptr< ManagedResource > Resources::ResourceManager::CreateManagedResource | ( | const Core::Rtti & | resType, | |
const ResourceId & | resId, | |||
const Ptr< ResourceLoader > & | optResourceLoader = 0 | |||
) |
create a ManagedResource object (bumps usecount on existing resource)
Create a shared ResourceManager object. If a managed resource with the same resource name already exists, its client count will be incremented and it will be returned. Otherwise the right ResourceMapper will be asked to create a new managed resource.
void Resources::ResourceManager::DiscardManagedResource | ( | const Ptr< ManagedResource > & | managedResource | ) |
unregister a ManagedResource object
Discard a shared ManagedResource object. This will decrement the client count. If the client count reaches zero, the ManagedResource object will be released as well.
void Resources::ResourceManager::Prepare | ( | bool | waiting | ) |
prepare stats gathering, call per frame
This method must be called per-frame before rendering begins. This will call the OnPrepare() method on all attached resource mappers, which will at least reset the render statistics in the managed resource.
void Resources::ResourceManager::Update | ( | IndexT | frameIdx | ) |
perform actual resource management, call per frame
This method must be called by the application after render statistics have been gathered and before the actual rendering. The ResourceManager will call the OnUpdate() method on all attached resource mappers. This is the place where the actual resource management will happen.
bool Resources::ResourceManager::CheckPendingResources | ( | ) |
test if any resources are pending, returns true if not resources are pending
This method tests if there are pending resource which haven't been loaded yet. Returns true if there are no pending resources (everything has loaded), false if pending resources exist.
bool Resources::ResourceManager::WaitForPendingResources | ( | Timing::Time | timeOut | ) |
wait until pending resources are loaded, or time-out is reached (returns false if time-out)
This method blocks until all pending resources are loaded, or until a time-out occurs. If a time-out occurs the method will return false, otherwise true.
Ptr< Resource > Resources::ResourceManager::CreateUnmanagedResource | ( | const ResourceId & | resId, | |
const Core::Rtti & | resClass, | |||
const Ptr< ResourceLoader > & | loader = 0 , |
|||
const Ptr< ResourceSaver > & | saver = 0 | |||
) |
Create a unmanaged resource object. If the resource already exists, its use count will be increased and the resource will be returned. If the resource doesn't exist yet, a new resource object will be created and registered as shared resource. Please note that you must call UnregisterUnmanagedResource() when the resource is no longer needed in order to manage the use count properly.
register an existing resource object as shared resource
Register an existing resource object as unmanaged resource. If the resource already has been registered, an assertion will be thrown. This will increment the use count of the resource by one.
unregister a shared resource (necessary for managing the use count)
Unregister an unmanaged resource. This will decrement the use count of the resource. If the use count has reached zero, the resource will be discarded (unloaded and removed from the unmanaged resource pool).
void Resources::ResourceManager::UnregisterUnmanagedResource | ( | const ResourceId & | id | ) |
unregister a shared resource by resource name
Unregister an unmanaged resource by resource id.
bool Resources::ResourceManager::HasResource | ( | const ResourceId & | resId | ) | const |
return true if a shared resource exists
Look up resource in registered unmanaged and managed resources.
const Ptr< Resource > & Resources::ResourceManager::LookupResource | ( | const ResourceId & | resId | ) | const |
lookup a shared resource
Look up resource in registered unmanaged and managed resources.
Array< Ptr< Resource > > Resources::ResourceManager::GetResourcesByType | ( | const Core::Rtti & | type | ) | const |
get shared resources by type (slow)
Returns an array of unmanaged resources by type. This is a slow method.
int Core::RefCounted::GetRefCount | ( | ) | const [inline, inherited] |
get the current refcount
Return the current refcount of the object.
void Core::RefCounted::AddRef | ( | ) | [inline, inherited] |
increment refcount by one
Increment the refcount of the object.
void Core::RefCounted::Release | ( | ) | [inline, inherited] |
decrement refcount and destroy object if refcount is zero
Decrement the refcount and destroy object if refcount is zero.
const Util::String & Core::RefCounted::GetClassName | ( | ) | const [inline, inherited] |
get the class name
Get the class name of the object.
Util::FourCC Core::RefCounted::GetClassFourCC | ( | ) | const [inline, inherited] |
get the class FourCC code
Get the class FourCC of the object.
void Core::RefCounted::DumpRefCountingLeaks | ( | ) | [static, inherited] |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!)
This method should be called as the very last before an application exits.