InternalGraphics::InternalStage Class Reference
#include <internalstage.h>
Inheritance diagram for InternalGraphics::InternalStage:
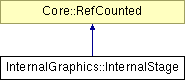
Detailed Description
A graphics stage groups graphics entities (models, cameras and lights) together for rendering. The main job of a Stage is to speed up visibility queries between the attached graphics entities. Different visibility query strategies are implemented by Stage subclasses. Nebula3 comes with a set of generic Stage subclasses for different purposes, but applications are free to derive their own subclasses which implement visibility query mechanisms tailored to the application.Visibility queries exist in the following variations:
- Camera->Light: this finds all light entities visible from a given camera
- Camera->Model: this finds all model entities visible from a given camera
- Light->Model: this finds all model entities which are lit by a given light source
Those visibility queries establish so-called visibility links between graphics entities which are then used by the lower level rendering subsystems to speed up rendering.
To render the content of a stage, at least one View object is needed. A View object binds renders a stage through a camera entity into a render target. Any number of View objects can exist in parallel, and may be bound to any Stage. Furthermore, dependencies between View objects may be defined (so that a View object will first ask the View objects it depends on to render themselves).
(C) 2007 Radon Labs GmbH
Public Member Functions | |
InternalStage () | |
constructor | |
virtual | ~InternalStage () |
destructor | |
bool | IsAttachedToServer () const |
return true if currently attached to graphics server | |
const Util::StringAtom & | GetName () const |
get human readable name of the stage | |
virtual void | AttachEntity (const Ptr< InternalGraphicsEntity > &graphicsEntity) |
attach an entity to the stage | |
virtual void | RemoveEntity (const Ptr< InternalGraphicsEntity > &entity) |
remove an entity from the stage | |
virtual void | NotifyOfEntityTransformChange (const Ptr< InternalGraphicsEntity > &entity) |
notify of an transform change | |
const Util::Array< Ptr< InternalGraphicsEntity > > & | GetEntities () const |
get an array of all entities attached to the stage | |
const Util::Array< Ptr< InternalGraphicsEntity > > & | GetEntitiesByType (InternalGraphicsEntityType::Code type) const |
get entities by type | |
void | RemoveAllEntities () |
remove all entities | |
virtual void | OnCullBefore (Timing::Time curTime, Timing::Time globalTimeFactor, IndexT frameIndex) |
call OnCullBefore in entities in the stage | |
virtual void | UpdateCameraLinks (const Ptr< InternalCameraEntity > &cameraEntity) |
update camera links for a given camera | |
virtual void | UpdateLightLinks () |
update light links for all visible lights (after updating camera links!) | |
void | AttachVisibilitySystems (const Util::Array< Ptr< Visibility::VisibilitySystemBase > > &systems) |
attach visibility systems to checker | |
Visibility::VisibilityChecker & | GetVisibilityChecker () |
get visibility checker | |
void | OnRenderDebug () |
on render debug | |
int | GetRefCount () const |
get the current refcount | |
void | AddRef () |
increment refcount by one | |
void | Release () |
decrement refcount and destroy object if refcount is zero | |
bool | IsInstanceOf (const Rtti &rtti) const |
return true if this object is instance of given class | |
bool | IsInstanceOf (const Util::String &className) const |
return true if this object is instance of given class by string | |
bool | IsInstanceOf (const Util::FourCC &classFourCC) const |
return true if this object is instance of given class by fourcc | |
bool | IsA (const Rtti &rtti) const |
return true if this object is instance of given class, or a derived class | |
bool | IsA (const Util::String &rttiName) const |
return true if this object is instance of given class, or a derived class, by string | |
bool | IsA (const Util::FourCC &rttiFourCC) const |
return true if this object is instance of given class, or a derived class, by fourcc | |
const Util::String & | GetClassName () const |
get the class name | |
Util::FourCC | GetClassFourCC () const |
get the class FourCC code | |
Static Public Member Functions | |
static void | DumpRefCountingLeaks () |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!) | |
Protected Member Functions | |
void | SetName (const Util::StringAtom &name) |
set a human readable name on the stage | |
virtual void | OnAttachToServer () |
called when the stage is attached to graphics server | |
virtual void | OnRemoveFromServer () |
called when the stage is detached from graphics server |
Member Function Documentation
void InternalGraphics::InternalStage::AttachEntity | ( | const Ptr< InternalGraphicsEntity > & | entity | ) | [virtual] |
attach an entity to the stage
Add an entity to the stage. The method OnAttachToStage() will be invoked on the entity, and the entity will be inserted into the cell hierarchy of the stage (which in turn call OnAttachToCell() on the entity).
void InternalGraphics::InternalStage::RemoveEntity | ( | const Ptr< InternalGraphicsEntity > & | entity | ) | [virtual] |
remove an entity from the stage
Remove an entity from the stage. This will remove the entity from the cell hierarchy of the stage (which invoked OnRemoveFromCell() on the entity), and then the method OnRemoveFromStage() will be called on the entity.
void InternalGraphics::InternalStage::OnCullBefore | ( | Timing::Time | curTime, | |
Timing::Time | globalTimeFactor, | |||
IndexT | frameIndex | |||
) | [virtual] |
call OnCullBefore in entities in the stage
Call the OnCullBefore() method on ALL methods in the stage (no matter whether they are visible or not).
void InternalGraphics::InternalStage::UpdateCameraLinks | ( | const Ptr< InternalCameraEntity > & | cameraEntity | ) | [virtual] |
update camera links for a given camera
Update visibility links for a given camera. This will create bidirectional visibility links between the camera and all other entities (most importantly light and model entities) which are visible through this camera. This method must be called once for each active camera after UpdateEntities() and before UpdateVisibleLightLinks().
void InternalGraphics::InternalStage::UpdateLightLinks | ( | ) | [virtual] |
update light links for all visible lights (after updating camera links!)
For each visible light entity, this method will create light links between the light entities, and model entities influenced by this light. This method must be called after UpdateCameraLinks() (this makes sure that no invisible lights and models will be checked).
int Core::RefCounted::GetRefCount | ( | ) | const [inline, inherited] |
get the current refcount
Return the current refcount of the object.
void Core::RefCounted::AddRef | ( | ) | [inline, inherited] |
increment refcount by one
Increment the refcount of the object.
void Core::RefCounted::Release | ( | ) | [inline, inherited] |
decrement refcount and destroy object if refcount is zero
Decrement the refcount and destroy object if refcount is zero.
const Util::String & Core::RefCounted::GetClassName | ( | ) | const [inline, inherited] |
get the class name
Get the class name of the object.
Util::FourCC Core::RefCounted::GetClassFourCC | ( | ) | const [inline, inherited] |
get the class FourCC code
Get the class FourCC of the object.
void Core::RefCounted::DumpRefCountingLeaks | ( | ) | [static, inherited] |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!)
This method should be called as the very last before an application exits.