Input::InputServer Class Reference
#include <inputserver.h>
Inheritance diagram for Input::InputServer:
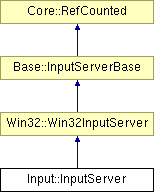
Detailed Description
The InputServer is the central object of the Input subsystem. It mainly manages a prioritized list of input handlers which process incoming input events.(C) 2007 Radon Labs GmbH
Public Member Functions | |
InputServer () | |
constructor | |
virtual | ~InputServer () |
destructor | |
void | Open () |
open the input server | |
void | Close () |
close the input server | |
void | OnFrame () |
call after processing window events (reads DInput raw mouse events) | |
void | SetMaxNumLocalPlayers (SizeT maxNumLocalPlayers) |
set the max number of local players for this application (default is 4) | |
SizeT | GetMaxNumLocalPlayers () const |
get the max number of local players | |
bool | IsOpen () const |
return true if open | |
void | SetQuitRequested (bool b) |
set the quit requested flag | |
bool | IsQuitRequested () const |
return true if some subsystem has requested to quit the app (e.g. Alt-F4) | |
void | Reset () |
reset input state | |
const Ptr< Input::Keyboard > & | GetDefaultKeyboard () const |
get the default keyboard input handler | |
const Ptr< Input::Mouse > & | GetDefaultMouse () const |
get the default mouse input handler | |
Ptr< Input::GamePad > | GetDefaultGamePad (IndexT playerIndex) const |
get default gamepad handler (playerIndex is valid up to MaxNumLocalPlayers) | |
void | AttachInputHandler (Input::InputPriority::Code pri, const Ptr< Input::InputHandler > &inputHandler) |
attach an input handler | |
void | RemoveInputHandler (const Ptr< Input::InputHandler > &inputHandler) |
remove an input handler | |
virtual void | BeginFrame () |
call before processing window events | |
void | EndFrame () |
call at end of frame | |
void | PutEvent (const Input::InputEvent &ie) |
put an input event into the handler chain | |
void | ClearMouseCapture () |
clear the current mouse capture (if exists) | |
void | ClearKeyboardCapture () |
clear the current keyboard capture (if exists) | |
void | ClearCapture () |
clear both mouse and keyboard captures | |
const Ptr< Input::InputHandler > & | GetMouseCaptureHandler () const |
return the current mouse capture input handler (return invalid ptr if no capture set) | |
const Ptr< Input::InputHandler > & | GetKeyboardCaptureHandler () const |
return the current keyboard capture input handler (return invalid ptr if no capture set) | |
void | ObtainMouseCapture (const Ptr< Input::InputHandler > &inputHandler) |
only call from InputHandler: capture mouse input to the given input handler | |
void | ReleaseMouseCapture (const Ptr< Input::InputHandler > &inputHandler) |
only call from InputHandler: release mouse capture | |
void | ObtainKeyboardCapture (const Ptr< Input::InputHandler > &inputHandler) |
only call from InputHandler: capture keyboard input to the given input handler | |
void | ReleaseKeyboardCapture (const Ptr< Input::InputHandler > &inputHandler) |
only call from InputHandler: release keyboard capture | |
int | GetRefCount () const |
get the current refcount | |
void | AddRef () |
increment refcount by one | |
void | Release () |
decrement refcount and destroy object if refcount is zero | |
bool | IsInstanceOf (const Rtti &rtti) const |
return true if this object is instance of given class | |
bool | IsInstanceOf (const Util::String &className) const |
return true if this object is instance of given class by string | |
bool | IsInstanceOf (const Util::FourCC &classFourCC) const |
return true if this object is instance of given class by fourcc | |
bool | IsA (const Rtti &rtti) const |
return true if this object is instance of given class, or a derived class | |
bool | IsA (const Util::String &rttiName) const |
return true if this object is instance of given class, or a derived class, by string | |
bool | IsA (const Util::FourCC &rttiFourCC) const |
return true if this object is instance of given class, or a derived class, by fourcc | |
const Util::String & | GetClassName () const |
get the class name | |
Util::FourCC | GetClassFourCC () const |
get the class FourCC code | |
Static Public Member Functions | |
static void | DumpRefCountingLeaks () |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!) | |
Protected Member Functions | |
bool | OpenDInputMouse () |
setup the DirectInput mouse device for tracking mouse movement | |
void | CloseDInputMouse () |
shutdown the DirectInput mouse device | |
void | ReadDInputMouse () |
get mouse readings | |
const Math::float2 & | GetMouseMovement () const |
get the current mouse movement |
Member Function Documentation
bool Win32::Win32InputServer::OpenDInputMouse | ( | ) | [protected, inherited] |
setup the DirectInput mouse device for tracking mouse movement
This intitialies a DirectInput mouse device in order to track raw mouse movement (WM mouse events stop at the screen borders).
void Win32::Win32InputServer::CloseDInputMouse | ( | ) | [protected, inherited] |
shutdown the DirectInput mouse device
Close the DirectInput mouse and DirectInput.
void Win32::Win32InputServer::ReadDInputMouse | ( | ) | [protected, inherited] |
get mouse readings
Read data from the DirectInput mouse (relative mouse movement since the last frame).
void Base::InputServerBase::SetMaxNumLocalPlayers | ( | SizeT | num | ) | [inherited] |
set the max number of local players for this application (default is 4)
Setup the maximum number of local players for this application. The default number is 1. This defines the number of game pad objects created and queried.
void Base::InputServerBase::EndFrame | ( | ) | [inherited] |
call at end of frame
Call this somewhere towards the end of frame, when it is guaraneteed that noone needs input anymore.
void Base::InputServerBase::PutEvent | ( | const Input::InputEvent & | ie | ) | [inherited] |
put an input event into the handler chain
NOTE: MouseMove and RawMouseMove events will be distributed to all input handlers regardless of mouse capture state!
void Base::InputServerBase::ClearMouseCapture | ( | ) | [inherited] |
clear the current mouse capture (if exists)
This clears the currently set mouse capture (if exists).
void Base::InputServerBase::ClearKeyboardCapture | ( | ) | [inherited] |
clear the current keyboard capture (if exists)
This clears the currently set keyboard capture (if exists).
void Base::InputServerBase::ClearCapture | ( | ) | [inherited] |
clear both mouse and keyboard captures
This clears the mouse and keyboards captures, if set.
void Base::InputServerBase::ObtainMouseCapture | ( | const Ptr< Input::InputHandler > & | inputHandler | ) | [inherited] |
only call from InputHandler: capture mouse input to the given input handler
Obtain the mouse capture. All mouse input will go exclusively to the capture input handler until ReleaseMouseCapture() is called.
void Base::InputServerBase::ReleaseMouseCapture | ( | const Ptr< Input::InputHandler > & | inputHandler | ) | [inherited] |
only call from InputHandler: release mouse capture
Release the mouse capture. The provided pointer must match the current capture input handler.
void Base::InputServerBase::ObtainKeyboardCapture | ( | const Ptr< Input::InputHandler > & | inputHandler | ) | [inherited] |
only call from InputHandler: capture keyboard input to the given input handler
Obtain the keyboard capture. All keyboard input will go exclusively to the capture input handler until ReleaseKeyboardCapture() is called.
void Base::InputServerBase::ReleaseKeyboardCapture | ( | const Ptr< Input::InputHandler > & | inputHandler | ) | [inherited] |
only call from InputHandler: release keyboard capture
Release the mouse capture. The provided pointer must match the current capture input handler.
int Core::RefCounted::GetRefCount | ( | ) | const [inline, inherited] |
get the current refcount
Return the current refcount of the object.
void Core::RefCounted::AddRef | ( | ) | [inline, inherited] |
increment refcount by one
Increment the refcount of the object.
void Core::RefCounted::Release | ( | ) | [inline, inherited] |
decrement refcount and destroy object if refcount is zero
Decrement the refcount and destroy object if refcount is zero.
const Util::String & Core::RefCounted::GetClassName | ( | ) | const [inline, inherited] |
get the class name
Get the class name of the object.
Util::FourCC Core::RefCounted::GetClassFourCC | ( | ) | const [inline, inherited] |
get the class FourCC code
Get the class FourCC of the object.
void Core::RefCounted::DumpRefCountingLeaks | ( | ) | [static, inherited] |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!)
This method should be called as the very last before an application exits.