OSX::OSXThread Class Reference
#include <osxthread.h>
Inheritance diagram for OSX::OSXThread:
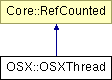
Detailed Description
OSX implementation of Threading::Thread. Uses the pthread API.(C) 2010 Radon Labs GmbH
Public Types | |
enum | Priority |
thread priorities | |
Public Member Functions | |
OSXThread () | |
constructor | |
virtual | ~OSXThread () |
destructor | |
void | SetPriority (Priority p) |
set the thread priority | |
Priority | GetPriority () const |
get the thread priority | |
void | SetCoreId (System::Cpu::CoreId coreId) |
set cpu core on which the thread should be running | |
System::Cpu::CoreId | GetCoreId () const |
get the cpu core on which the thread should be running | |
void | SetStackSize (SizeT s) |
set stack size in bytes (default is 4 KByte) | |
SizeT | GetStackSize () const |
get stack size | |
void | SetName (const Util::String &n) |
set thread name | |
const Util::String & | GetName () const |
get thread name | |
void | Start () |
start executing the thread code, returns when thread has actually started | |
void | Stop () |
request threading code to stop, returns when thread has actually finished | |
bool | IsRunning () const |
return true if thread has been started | |
int | GetRefCount () const |
get the current refcount | |
void | AddRef () |
increment refcount by one | |
void | Release () |
decrement refcount and destroy object if refcount is zero | |
bool | IsInstanceOf (const Rtti &rtti) const |
return true if this object is instance of given class | |
bool | IsInstanceOf (const Util::String &className) const |
return true if this object is instance of given class by string | |
bool | IsInstanceOf (const Util::FourCC &classFourCC) const |
return true if this object is instance of given class by fourcc | |
bool | IsA (const Rtti &rtti) const |
return true if this object is instance of given class, or a derived class | |
bool | IsA (const Util::String &rttiName) const |
return true if this object is instance of given class, or a derived class, by string | |
bool | IsA (const Util::FourCC &rttiFourCC) const |
return true if this object is instance of given class, or a derived class, by fourcc | |
const Util::String & | GetClassName () const |
get the class name | |
Util::FourCC | GetClassFourCC () const |
get the class FourCC code | |
Static Public Member Functions | |
static void | YieldThread () |
yield the thread (gives up current time slice) | |
static Threading::ThreadId | GetMyThreadId () |
get the thread ID of this thread | |
static void | DumpRefCountingLeaks () |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!) | |
Protected Member Functions | |
virtual void | EmitWakeupSignal () |
override this method if your thread loop needs a wakeup call before stopping | |
virtual void | DoWork () |
this method runs in the thread context | |
bool | ThreadStopRequested () const |
check if stop is requested, call from DoWork() to see if the thread proc should quit |
Member Function Documentation
void OSX::OSXThread::SetName | ( | const Util::String & | n | ) | [inline] |
set thread name
Set the thread's name.
const Util::String & OSX::OSXThread::GetName | ( | ) | const [inline] |
get thread name
Get the thread's name. This is the vanilla method which returns the name member.
void OSX::OSXThread::Stop | ( | ) |
request threading code to stop, returns when thread has actually finished
This stops the thread by signalling the stopRequestEvent and waits for the thread to actually quit. If the thread code runs in a loop it should use the IsStopRequested() method to see if the thread object wants it to shutdown. If so DoWork() should simply return.
bool OSX::OSXThread::IsRunning | ( | ) | const |
return true if thread has been started
Returns true if the thread is currently running.
void OSX::OSXThread::YieldThread | ( | ) | [static] |
yield the thread (gives up current time slice)
Give up time slice.
Threading::ThreadId OSX::OSXThread::GetMyThreadId | ( | ) | [static] |
get the thread ID of this thread
Static method which returns the ThreadId of this thread.
void OSX::OSXThread::EmitWakeupSignal | ( | ) | [protected, virtual] |
override this method if your thread loop needs a wakeup call before stopping
This method is called by Thread::Stop() after setting the stopRequest event and before waiting for the thread to stop. If your thread runs a loop and waits for jobs it may need an extra wakeup signal to stop waiting and check for the ThreadStopRequested() event. In this case, override this method and signal your event object.
void OSX::OSXThread::DoWork | ( | ) | [protected, virtual] |
this method runs in the thread context
This method should be derived in a Thread subclass and contains the actual code which is run in the thread. The method must not call C-Lib functions under Win32. To terminate the thread, just return from this function. If DoWork() runs in an infinite loop, call ThreadStopRequested() to check whether the Thread object wants the thread code to quit.
bool OSX::OSXThread::ThreadStopRequested | ( | ) | const [inline, protected] |
check if stop is requested, call from DoWork() to see if the thread proc should quit
If the derived DoWork() method is running in a loop it must regularly check if the process wants the thread to terminate by calling ThreadStopRequested() and simply return if the result is true. This will cause the thread to shut down.
int Core::RefCounted::GetRefCount | ( | ) | const [inline, inherited] |
get the current refcount
Return the current refcount of the object.
void Core::RefCounted::AddRef | ( | ) | [inline, inherited] |
increment refcount by one
Increment the refcount of the object.
void Core::RefCounted::Release | ( | ) | [inline, inherited] |
decrement refcount and destroy object if refcount is zero
Decrement the refcount and destroy object if refcount is zero.
const Util::String & Core::RefCounted::GetClassName | ( | ) | const [inline, inherited] |
get the class name
Get the class name of the object.
Util::FourCC Core::RefCounted::GetClassFourCC | ( | ) | const [inline, inherited] |
get the class FourCC code
Get the class FourCC of the object.
void Core::RefCounted::DumpRefCountingLeaks | ( | ) | [static, inherited] |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!)
This method should be called as the very last before an application exits.