IO::BinaryWriter Class Reference
#include <binarywriter.h>
Inheritance diagram for IO::BinaryWriter:
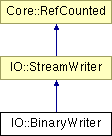
Detailed Description
A friendly interface for writing binary data to a stream. Optionally the writer can use memory mapping for optimal write performance.
- Todo:
- convert endianess!
Public Member Functions | |
BinaryWriter () | |
constructor | |
virtual | ~BinaryWriter () |
destructor | |
void | SetMemoryMappingEnabled (bool b) |
call before Open() to enable memory mapping (if stream supports mapping) | |
bool | IsMemoryMappingEnabled () const |
return true if memory mapping is enabled | |
void | SetStreamByteOrder (System::ByteOrder::Type byteOrder) |
set the stream byte order (default is host byte order) | |
System::ByteOrder::Type | GetStreamByteOrder () const |
get the stream byte order | |
virtual bool | Open () |
begin reading from the stream | |
virtual void | Close () |
end reading from the stream | |
void | WriteChar (char c) |
write an 8-bit char to the stream | |
void | WriteUChar (unsigned char c) |
write an 8-bit unsigned char to the stream | |
void | WriteShort (short s) |
write an 16-bit short to the stream | |
void | WriteUShort (unsigned short s) |
write an 16-bit unsigned short to the stream | |
void | WriteInt (int i) |
write an 32-bit int to the stream | |
void | WriteUInt (unsigned int i) |
write an 32-bit unsigned int to the stream | |
void | WriteFloat (float f) |
write a float value to the stream | |
void | WriteFloatAsNormalizedUByte2 (float f) |
write a compressed float value to the stream, lossy and needed to be in the range of -1.0 and +1.0 | |
void | WriteFloatAsUnsignedNormalizedUByte2 (float f) |
write a compressed float value to the stream, lossy and needed to be in the range of 0.0 and +1.0 | |
void | WriteDouble (double d) |
write a double value to the stream | |
void | WriteBool (bool b) |
write a boolean value to the stream | |
void | WriteString (const Util::String &s) |
write a string to the stream | |
void | WriteFloat2 (Math::float2 f) |
write a float value to the stream | |
void | WriteFloat4 (const Math::float4 &v) |
write a float4 to the stream | |
void | WritePoint (const Math::point &v) |
write a float4 to the stream | |
void | WriteVector (const Math::vector &v) |
write a float4 to the stream | |
void | WriteMatrix44 (const Math::matrix44 &m) |
write a matrix44 to the stream | |
void | WriteGuid (const Util::Guid &guid) |
write a guid | |
void | WriteBlob (const Util::Blob &blob) |
write a blob of data | |
void | WriteRawData (const void *ptr, SizeT numBytes) |
write raw data | |
void | SetStream (const Ptr< Stream > &s) |
set stream to write to | |
const Ptr< Stream > & | GetStream () const |
get currently set stream | |
bool | HasStream () const |
return true if a stream is set | |
bool | IsOpen () const |
return true if currently open | |
int | GetRefCount () const |
get the current refcount | |
void | AddRef () |
increment refcount by one | |
void | Release () |
decrement refcount and destroy object if refcount is zero | |
bool | IsInstanceOf (const Rtti &rtti) const |
return true if this object is instance of given class | |
bool | IsInstanceOf (const Util::String &className) const |
return true if this object is instance of given class by string | |
bool | IsInstanceOf (const Util::FourCC &classFourCC) const |
return true if this object is instance of given class by fourcc | |
bool | IsA (const Rtti &rtti) const |
return true if this object is instance of given class, or a derived class | |
bool | IsA (const Util::String &rttiName) const |
return true if this object is instance of given class, or a derived class, by string | |
bool | IsA (const Util::FourCC &rttiFourCC) const |
return true if this object is instance of given class, or a derived class, by fourcc | |
const Util::String & | GetClassName () const |
get the class name | |
Util::FourCC | GetClassFourCC () const |
get the class FourCC code | |
Static Public Member Functions | |
static void | DumpRefCountingLeaks () |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!) |
Member Function Documentation
void IO::BinaryWriter::WriteString | ( | const Util::String & | s | ) |
write a string to the stream
NOTE: for strings, first the length will be written into a 32-bit int, then the string contents without the 0-terminator.
set stream to write to
Attaches the writer to a stream. This will imcrement the refcount of the stream.
Reimplemented in Messaging::MessageWriter.
get currently set stream
Get pointer to the attached stream. If there is no stream attached, an assertion will be thrown. Use HasStream() to determine if a stream is attached.
bool IO::StreamWriter::HasStream | ( | ) | const [inherited] |
return true if a stream is set
Returns true if a stream is attached to the writer.
int Core::RefCounted::GetRefCount | ( | ) | const [inline, inherited] |
get the current refcount
Return the current refcount of the object.
void Core::RefCounted::AddRef | ( | ) | [inline, inherited] |
increment refcount by one
Increment the refcount of the object.
void Core::RefCounted::Release | ( | ) | [inline, inherited] |
decrement refcount and destroy object if refcount is zero
Decrement the refcount and destroy object if refcount is zero.
const Util::String & Core::RefCounted::GetClassName | ( | ) | const [inline, inherited] |
get the class name
Get the class name of the object.
Util::FourCC Core::RefCounted::GetClassFourCC | ( | ) | const [inline, inherited] |
get the class FourCC code
Get the class FourCC of the object.
void Core::RefCounted::DumpRefCountingLeaks | ( | ) | [static, inherited] |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!)
This method should be called as the very last before an application exits.