Base::SkinnedMeshRendererBase Class Reference
#include <skinnedmeshrendererbase.h>
Inheritance diagram for Base::SkinnedMeshRendererBase:
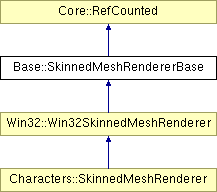
Detailed Description
Wraps platform-specific rendering of a skinned mesh. For a platform which supports GPU skinning (e.g. anything except the Wii), simply call the DrawGPUSkinnedMesh(). For a software-skinned platform, call the UpdateSoftwareSkinnedMesh() ideally once per frame (although the method makes sure, that a mesh isn't skinned twice even when the method is called multiple times), and then use the returned DrawHandle to call DrawSoftwareSkinnedMesh() several times per frame.See CharacterNodeInstance for details.
(C) 2008 Radon Labs GmbH
Public Types | |
typedef IndexT | DrawHandle |
an abstract draw handle | |
Public Member Functions | |
SkinnedMeshRendererBase () | |
constructor | |
virtual | ~SkinnedMeshRendererBase () |
destructor | |
void | Setup () |
setup the renderer | |
void | Discard () |
discard the renderer | |
bool | IsValid () const |
return true if renderer is valid | |
Characters::SkinningTechnique::Code | GetSkinningTechnique () const |
get the skinning technique used by the renderer | |
int | GetRefCount () const |
get the current refcount | |
void | AddRef () |
increment refcount by one | |
void | Release () |
decrement refcount and destroy object if refcount is zero | |
bool | IsInstanceOf (const Rtti &rtti) const |
return true if this object is instance of given class | |
bool | IsInstanceOf (const Util::String &className) const |
return true if this object is instance of given class by string | |
bool | IsInstanceOf (const Util::FourCC &classFourCC) const |
return true if this object is instance of given class by fourcc | |
bool | IsA (const Rtti &rtti) const |
return true if this object is instance of given class, or a derived class | |
bool | IsA (const Util::String &rttiName) const |
return true if this object is instance of given class, or a derived class, by string | |
bool | IsA (const Util::FourCC &rttiFourCC) const |
return true if this object is instance of given class, or a derived class, by fourcc | |
const Util::String & | GetClassName () const |
get the class name | |
Util::FourCC | GetClassFourCC () const |
get the class FourCC code | |
Static Public Member Functions | |
static void | DumpRefCountingLeaks () |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!) | |
Protected Member Functions | |
void | OnBeginFrame () |
call once at beginning of frame | |
void | OnEndFrame () |
call once at end of frame (after rendering) | |
void | BeginGatherSkins () |
begin gathering software-skinned meshes | |
DrawHandle | RegisterSoftwareSkinnedMesh (const Ptr< Characters::CharacterInstance > &charInst, const Ptr< CoreGraphics::Mesh > &mesh) |
update a software skinned mesh | |
void | EndGatherSkins () |
end gathering software-skinned meshes | |
void | UpdateSoftwareSkinnedMeshes () |
update software-skinned meshes | |
void | DrawSoftwareSkinnedMesh (DrawHandle h, IndexT primGroupIndex) |
draw a software skinned mesh | |
void | DrawGPUSkinnedMesh (const Ptr< Characters::CharacterInstance > &charInst, const Ptr< CoreGraphics::Mesh > &mesh, IndexT primGroupIndex, const Util::Array< IndexT > &jointPalette, const Ptr< CoreGraphics::ShaderVariable > &jointPaletteShdVar) |
draw a hardware skinned mesh | |
void | DrawGPUTextureSkinnedMesh (const Ptr< Characters::CharacterInstance > &charInst, const Ptr< CoreGraphics::Mesh > &mesh, IndexT primGroupIndex, const Ptr< CoreGraphics::ShaderVariable > &charInstShaderVar) |
draw a skinned mesh | |
IndexT | AllocJointTextureRow () |
allocate a row index in the joint texture | |
void | FreeJointTextureRow (IndexT rowIndex) |
free a row index in the joint texture | |
void * | AcquireJointTextureRowPointer (const Ptr< Characters::CharacterInstance > &charInst, SizeT &outRowPitch) |
get a pointer to the joint texture row for the given character instance |
Member Function Documentation
Characters::SkinningTechnique::Code Base::SkinnedMeshRendererBase::GetSkinningTechnique | ( | ) | const [inline] |
get the skinning technique used by the renderer
Override this method in a platform specific subclass!
Reimplemented in Win32::Win32SkinnedMeshRenderer.
SkinnedMeshRendererBase::DrawHandle Base::SkinnedMeshRendererBase::RegisterSoftwareSkinnedMesh | ( | const Ptr< Characters::CharacterInstance > & | charInst, | |
const Ptr< CoreGraphics::Mesh > & | mesh | |||
) | [protected] |
update a software skinned mesh
This method should only be called when RequiresSoftwareSkinning() returns true!
This registers a mesh for software-skinning in the UpdateSoftwareSkinnedMeshes() which must be called after EndGatherSkins().
This method may be called more then once per character-instance/mesh combination! The method will drop duplicates.
void Base::SkinnedMeshRendererBase::UpdateSoftwareSkinnedMeshes | ( | ) | [protected] |
update software-skinned meshes
On platforms with software-skinning, this method should perform the skinning for all meshes gathered during the GatherSkins pass.
void Base::SkinnedMeshRendererBase::DrawSoftwareSkinnedMesh | ( | DrawHandle | h, | |
IndexT | primGroupIndex | |||
) | [protected] |
draw a software skinned mesh
This method should only be called when RequiresSoftwareSkinning() returns true!
Software-skinning platforms call this method with the DrawHandle returned by UpdateSoftwareSkinnedMesh() to draw a portion of the skinned mesh/
void Base::SkinnedMeshRendererBase::DrawGPUSkinnedMesh | ( | const Ptr< Characters::CharacterInstance > & | charInst, | |
const Ptr< CoreGraphics::Mesh > & | mesh, | |||
IndexT | primGroupIndex, | |||
const Util::Array< IndexT > & | jointPalette, | |||
const Ptr< CoreGraphics::ShaderVariable > & | jointPaletteShdVar | |||
) | [protected] |
draw a hardware skinned mesh
This method should only be called when RequiresSoftwareSkinning() returns false!
This is the skinned-mesh rendering method for platforms which do skinning on the GPU.
int Core::RefCounted::GetRefCount | ( | ) | const [inline, inherited] |
get the current refcount
Return the current refcount of the object.
void Core::RefCounted::AddRef | ( | ) | [inline, inherited] |
increment refcount by one
Increment the refcount of the object.
void Core::RefCounted::Release | ( | ) | [inline, inherited] |
decrement refcount and destroy object if refcount is zero
Decrement the refcount and destroy object if refcount is zero.
const Util::String & Core::RefCounted::GetClassName | ( | ) | const [inline, inherited] |
get the class name
Get the class name of the object.
Util::FourCC Core::RefCounted::GetClassFourCC | ( | ) | const [inline, inherited] |
get the class FourCC code
Get the class FourCC of the object.
void Core::RefCounted::DumpRefCountingLeaks | ( | ) | [static, inherited] |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!)
This method should be called as the very last before an application exits.