Models::ModelNodeInstance Class Reference
#include <modelnodeinstance.h>
Inheritance diagram for Models::ModelNodeInstance:
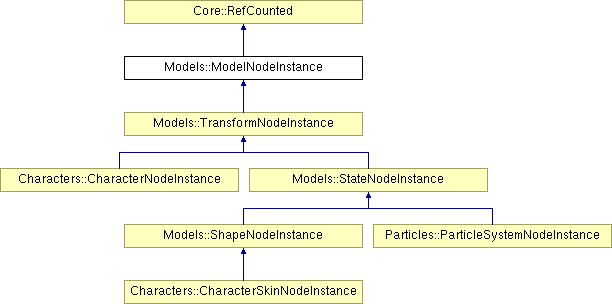
Detailed Description
A ModelNodeInstance holds the per-instance data of a ModelNode and does most of the actually interesting Model rendering stuff.(C) 2007 Radon Labs GmbH
Public Member Functions | |
ModelNodeInstance () | |
constructor | |
virtual | ~ModelNodeInstance () |
destructor | |
virtual void | Setup (const Ptr< ModelInstance > &inst, const Ptr< ModelNode > &node, const Ptr< ModelNodeInstance > &parentNodeInst) |
setup the model node instance | |
virtual void | Discard () |
discard the model node instance | |
virtual void | DiscardHierarchy () |
discard the model node instance and all of its children | |
bool | IsValid () const |
return true if the model node instance is valid | |
virtual void | OnNotifyCullingVisible (IndexT frameIndex, Timing::Time time) |
called from ModelEntity::OnNotifyCullingVisible | |
virtual void | OnRenderBefore (IndexT frameIndex, Timing::Time time) |
called from ModelEntity::OnRenderBefore | |
virtual void | OnVisibilityResolve (IndexT resolveIndex, float distanceToViewer) |
called during visibility resolve | |
virtual void | ApplyState () |
apply per-instance state prior to rendering | |
virtual void | Render () |
perform rendering | |
const Util::StringAtom & | GetName () const |
get model node name | |
bool | HasParent () const |
return true if node has a parent | |
const Ptr< ModelNodeInstance > & | GetParent () const |
get parent node | |
const Util::Array< Ptr< ModelNodeInstance > > & | GetChildren () const |
get child nodes | |
bool | HasChild (const Util::StringAtom &name) const |
return true if a direct child exists by name | |
const Ptr< ModelNodeInstance > & | LookupChild (const Util::StringAtom &name) const |
get pointer to direct child by name | |
Ptr< ModelNodeInstance > | LookupPath (const Util::String &path) |
get modelnodeinstance by hierarchy path | |
const Ptr< ModelInstance > & | GetModelInstance () const |
get the ModelInstance we are attached to | |
const Ptr< ModelNode > & | GetModelNode () const |
get the ModelNode we're associated with | |
void | SetVisible (bool b, Timing::Time time, bool recursive=true) |
set the node instance's visibility | |
bool | IsVisible () const |
return true if node instance is set to visible | |
IndexT | GetModelNodeInstanceIndex () const |
get model node instance index for current frame | |
int | GetRefCount () const |
get the current refcount | |
void | AddRef () |
increment refcount by one | |
void | Release () |
decrement refcount and destroy object if refcount is zero | |
bool | IsInstanceOf (const Rtti &rtti) const |
return true if this object is instance of given class | |
bool | IsInstanceOf (const Util::String &className) const |
return true if this object is instance of given class by string | |
bool | IsInstanceOf (const Util::FourCC &classFourCC) const |
return true if this object is instance of given class by fourcc | |
bool | IsA (const Rtti &rtti) const |
return true if this object is instance of given class, or a derived class | |
bool | IsA (const Util::String &rttiName) const |
return true if this object is instance of given class, or a derived class, by string | |
bool | IsA (const Util::FourCC &rttiFourCC) const |
return true if this object is instance of given class, or a derived class, by fourcc | |
const Util::String & | GetClassName () const |
get the class name | |
Util::FourCC | GetClassFourCC () const |
get the class FourCC code | |
Static Public Member Functions | |
static void | DumpRefCountingLeaks () |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!) | |
Protected Member Functions | |
void | SetParent (const Ptr< ModelNodeInstance > &p) |
set parent node | |
void | AddChild (const Ptr< ModelNodeInstance > &c) |
add a child node | |
virtual void | RenderDebug () |
render node specific debug shape | |
virtual void | OnShow (Timing::Time time) |
called when the node becomes visible with current time | |
virtual void | OnHide (Timing::Time time) |
called when the node becomes invisible |
Member Function Documentation
void Models::ModelNodeInstance::DiscardHierarchy | ( | ) | [virtual] |
discard the model node instance and all of its children
Discards this model node instance and all of its children recursively.
void Models::ModelNodeInstance::ApplyState | ( | ) | [virtual] |
apply per-instance state prior to rendering
The ApplyState() method is called when per-instance shader-state should be applied. This method may be called several times per frame. The calling order is always in "render order", first, the ApplyState() method on the ModelNode will be called, then each ApplyState() and Render() method of the ModelNodeInstance objects.
Reimplemented in Models::StateNodeInstance, Models::TransformNodeInstance, and Particles::ParticleSystemNodeInstance.
void Models::ModelNodeInstance::Render | ( | ) | [virtual] |
perform rendering
The Render() method is called when the ModelNodeInstance needs to render itself. There will always be a call to the Apply() method before Render() is called, however, Render() may be called several times per Apply() invokation.
Reimplemented in Characters::CharacterSkinNodeInstance, Models::ShapeNodeInstance, and Particles::ParticleSystemNodeInstance.
bool Models::ModelNodeInstance::IsVisible | ( | ) | const |
return true if node instance is set to visible
FIXME: The recursion in this is method makes it slow, especially in deep hierarchies. Also it can't be inlined.
int Core::RefCounted::GetRefCount | ( | ) | const [inline, inherited] |
get the current refcount
Return the current refcount of the object.
void Core::RefCounted::AddRef | ( | ) | [inline, inherited] |
increment refcount by one
Increment the refcount of the object.
void Core::RefCounted::Release | ( | ) | [inline, inherited] |
decrement refcount and destroy object if refcount is zero
Decrement the refcount and destroy object if refcount is zero.
const Util::String & Core::RefCounted::GetClassName | ( | ) | const [inline, inherited] |
get the class name
Get the class name of the object.
Util::FourCC Core::RefCounted::GetClassFourCC | ( | ) | const [inline, inherited] |
get the class FourCC code
Get the class FourCC of the object.
void Core::RefCounted::DumpRefCountingLeaks | ( | ) | [static, inherited] |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!)
This method should be called as the very last before an application exits.