Win32::Win32Socket Class Reference
#include <win32socket.h>
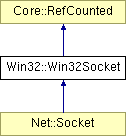
Detailed Description
A lowlevel socket wrapper class. This class is primarily a platform abstraction class, not an "end-user-class". Usually a Nebula3 application doesn't use this class directly but instead uses the higher level classes like TcpServer, TclClient, etc...(C) 2006 Radon Labs GmbH
Public Types | |
enum | Protocol |
protocol types | |
enum | ErrorCode |
error codes | |
Public Member Functions | |
Win32Socket () | |
constructor | |
virtual | ~Win32Socket () |
destructor | |
bool | Open (Protocol p) |
open the socket | |
void | Close () |
close the socket | |
bool | IsOpen () const |
return true if the socket is open | |
ErrorCode | GetErrorCode () const |
get the last error code | |
Util::String | GetErrorString () const |
get the last error string | |
void | SetAddress (const Net::IpAddress &a) |
set internet address of socket | |
const Net::IpAddress & | GetAddress () const |
get internet address of socket | |
void | SetBroadcast (bool b) |
set the broadcast flag (SO_BROADCAST) | |
bool | GetBroadcast () |
get the broadcast flag | |
void | SetDontLinger (bool b) |
set the don't linger flag (SO_DONTLINGER) | |
bool | GetDontLinger () |
get the don't linger flag | |
void | SetKeepAlive (bool b) |
set the keepalive flag (SO_KEEPALIVE) | |
bool | GetKeepAlive () |
get the keepalive flag | |
void | SetReUseAddr (bool b) |
set reuseaddr flag (SO_REUSEADDR) | |
bool | GetReUseAddr () |
get reuseaddr flag | |
void | SetNoDelay (bool b) |
set nodelay flag (TCP_NODELAY) | |
bool | GetNoDelay () |
get nodelay flag | |
void | SetRecvBufSize (SizeT s) |
set receive buffer size | |
SizeT | GetRecvBufSize () |
get receive buffer size | |
void | SetSendBufSize (SizeT s) |
set send buffer size | |
SizeT | GetSendBufSize () |
get send buffer size | |
void | SetBlocking (bool b) |
set blocking mode (FIONBIO) | |
bool | GetBlocking () const |
get blocking mode | |
SizeT | GetMaxMsgSize () |
get the maximum message size that can be sent atomically | |
bool | Bind () |
bind socket to ip address | |
bool | IsBound () const |
return true if the socket is bound to an address | |
bool | Listen () |
listen for incoming connections (for server sockets) | |
bool | Accept (Ptr< Net::Socket > &outSocket) |
accept incoming connection, return a new socket (for server sockets) | |
Result | Connect () |
connect to the sockets address (for client sockets) | |
bool | IsConnected () |
test if the socket is currently connected | |
Result | Send (const void *buf, SizeT numBytes, SizeT &bytesSent) |
send raw data into the socket | |
bool | HasRecvData () |
return true if recv data is available at the socket | |
Result | Recv (void *buf, SizeT bufSize, SizeT &bytesReceived) |
receive raw data from the socket | |
Result | SendTo (const void *buf, SizeT numBytes, uint addr, ushort port, SizeT &bytesSent) |
send raw data to address for connectionless sockets | |
Result | RecvFrom (void *buf, SizeT bufSize, uint addr, ushort port, SizeT &bytesReceived) |
receive raw data from address for connectionless sockets | |
int | GetRefCount () const |
get the current refcount | |
void | AddRef () |
increment refcount by one | |
void | Release () |
decrement refcount and destroy object if refcount is zero | |
bool | IsInstanceOf (const Rtti &rtti) const |
return true if this object is instance of given class | |
bool | IsInstanceOf (const Util::String &className) const |
return true if this object is instance of given class by string | |
bool | IsInstanceOf (const Util::FourCC &classFourCC) const |
return true if this object is instance of given class by fourcc | |
bool | IsA (const Rtti &rtti) const |
return true if this object is instance of given class, or a derived class | |
bool | IsA (const Util::String &rttiName) const |
return true if this object is instance of given class, or a derived class, by string | |
bool | IsA (const Util::FourCC &rttiFourCC) const |
return true if this object is instance of given class, or a derived class, by fourcc | |
const Util::String & | GetClassName () const |
get the class name | |
Util::FourCC | GetClassFourCC () const |
get the class FourCC code | |
Static Public Member Functions | |
static void | DumpRefCountingLeaks () |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!) |
Member Function Documentation
void Win32::Win32Socket::SetAddress | ( | const Net::IpAddress & | a | ) | [inline] |
set internet address of socket
Set internet address of socket.
const Net::IpAddress & Win32::Win32Socket::GetAddress | ( | ) | const [inline] |
get internet address of socket
Get internet address of socket.
void Win32::Win32Socket::SetBlocking | ( | bool | b | ) |
set blocking mode (FIONBIO)
Set the socket to blocking mode.
bool Win32::Win32Socket::Bind | ( | ) |
bind socket to ip address
Bind the socket to its ip address set with SetAddress() and SetPort(). After binding the socket to an address, call the Listen() method to wait for incoming connections. This method only makes sense for server sockets.
bool Win32::Win32Socket::Listen | ( | ) |
listen for incoming connections (for server sockets)
Wait for incoming connections to a server socket. Call this method on server side after binding the socket to its address.
bool Win32::Win32Socket::Accept | ( | Ptr< Net::Socket > & | outSocket | ) |
accept incoming connection, return a new socket (for server sockets)
Accept an incoming connection to a server socket. This will spawn a new socket for the connection which will be returned in the provided pointer reference. The address of the returned socket will be set to the address of the "connecting entity".
Win32Socket::Result Win32::Win32Socket::Connect | ( | ) |
connect to the sockets address (for client sockets)
Connect to a server socket. This method is called by a client socket to connect to a server socket identified by the socket object's address. A non-blocking socket will return immediately with WouldBlock, since the connection cannot be established immediately. In this case, just continue to call Connect() until the method returns Success, or alternative, check the IsConnected() method, which will also return true once the connection has been establish.
bool Win32::Win32Socket::IsConnected | ( | ) |
test if the socket is currently connected
This tests if the socket is actually connected by doing a select() on the socket to probe for writability. So the IsConnected() method basically checks whether data can be sent through the socket.
Win32Socket::Result Win32::Win32Socket::Send | ( | const void * | buf, | |
SizeT | numBytes, | |||
SizeT & | bytesSent | |||
) |
send raw data into the socket
Send raw data into the socket. Note that depending on the buffer size of the underlying socket implementation and other sockets, the method may not be able to send all provided data. In this case, the returned content of bytesSent will be less then numBytes, even though the return value will be Success. It is up to the caller to handle the extra data which hasn't been sent with the current call.
bool Win32::Win32Socket::HasRecvData | ( | ) |
return true if recv data is available at the socket
This method checks if the socket has received data available. Use this method in a loop with Recv() to get all data waiting at the socket. This method will never block.
Win32Socket::Result Win32::Win32Socket::Recv | ( | void * | buf, | |
SizeT | bufSize, | |||
SizeT & | bytesReceived | |||
) |
receive raw data from the socket
Receive raw data from a socket and write the received data into the provided buffer. On a blocking socket this method will block until data arrives at the socket. A non-blocking socket would immediately return in this case with a WouldBlock result. When valid data has been received the method will return with a Success result and the bytesReceived argument will contain the number of received bytes. It is not guaranteed that a single receive will return all data waiting on the socket. To make sure that the socket is really empty, call Recv() in a loop until HasRecvData() returns false. When the socket has been gracefully closed by the other side, the method will return with a Closed return value. Everything else will return with an Error return code. Call GetErrorCode() or GetErrorString() to find out more in this case.
Win32Socket::Result Win32::Win32Socket::SendTo | ( | const void * | buf, | |
SizeT | numBytes, | |||
uint | addr, | |||
ushort | port, | |||
SizeT & | bytesSent | |||
) |
send raw data to address for connectionless sockets
FIXME: this is the send method for connectionless sockets using the UDP protocol.
Win32Socket::Result Win32::Win32Socket::RecvFrom | ( | void * | buf, | |
SizeT | bufSize, | |||
uint | addr, | |||
ushort | port, | |||
SizeT & | bytesReceived | |||
) |
receive raw data from address for connectionless sockets
FIXME: this is the recv method for connectionless socket using the UDP protocol.
int Core::RefCounted::GetRefCount | ( | ) | const [inline, inherited] |
get the current refcount
Return the current refcount of the object.
void Core::RefCounted::AddRef | ( | ) | [inline, inherited] |
increment refcount by one
Increment the refcount of the object.
void Core::RefCounted::Release | ( | ) | [inline, inherited] |
decrement refcount and destroy object if refcount is zero
Decrement the refcount and destroy object if refcount is zero.
const Util::String & Core::RefCounted::GetClassName | ( | ) | const [inline, inherited] |
get the class name
Get the class name of the object.
Util::FourCC Core::RefCounted::GetClassFourCC | ( | ) | const [inline, inherited] |
get the class FourCC code
Get the class FourCC of the object.
void Core::RefCounted::DumpRefCountingLeaks | ( | ) | [static, inherited] |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!)
This method should be called as the very last before an application exits.