IO::XmlReader Class Reference
#include <xmlreader.h>
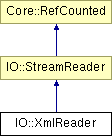
Detailed Description
Reads XML formatted data with random access from a stream using TinyXML as backend. The XML document is represented as a tree of nodes, which can be navigated and queried.(C) 2006 Radon Labs GmbH
Public Member Functions | |
XmlReader () | |
constructor | |
virtual | ~XmlReader () |
destructor | |
virtual bool | Open () |
begin reading from the stream | |
virtual void | Close () |
end reading from the stream | |
bool | HasNode (const Util::String &path) const |
return true if node exists | |
Util::String | GetCurrentNodeName () const |
get short name of current node | |
Util::String | GetCurrentNodePath () const |
get path to current node | |
int | GetCurrentNodeLineNumber () const |
returns the line number of the current node | |
void | SetToNode (const Util::String &path) |
set current node as path | |
bool | SetToFirstChild (const Util::String &name="") |
set current node to first child node, return false if no child exists | |
bool | SetToNextChild (const Util::String &name="") |
set current node to next sibling node, return false if no more sibling exists | |
bool | SetToParent () |
set current node to parent, return false if no parent exists | |
bool | HasAttr (const Util::String &attr) const |
return true if matching attribute exists on current node | |
Util::Array < Util::String > | GetAttrs () const |
return names of all attrs on current node | |
bool | HasContent () const |
return true if current node has embedded content | |
Util::String | GetContent () const |
return embedded content of current node | |
Util::String | GetString (const Util::String &attr) const |
get string attribute value from current node | |
bool | GetBool (const Util::String &attr) const |
get bool attribute value from current node | |
int | GetInt (const Util::String &attr) const |
get int attribute value from current node | |
float | GetFloat (const Util::String &attr) const |
get float attribute value from current node | |
Math::float4 | GetFloat4 (const Util::String &attr) const |
get float4 attribute value from current node | |
Math::matrix44 | GetMatrix44 (const Util::String &attr) const |
get matrix44 attribute value from current node | |
Util::String | GetOptString (const Util::String &attr, const Util::String &defaultValue) const |
get optional string attribute value from current node | |
bool | GetOptBool (const Util::String &attr, bool defaultValue) const |
get optional bool attribute value from current node | |
int | GetOptInt (const Util::String &attr, int defaultValue) const |
get optional int attribute value from current node | |
float | GetOptFloat (const Util::String &attr, float defaultValue) const |
get optional float attribute value from current node | |
Math::float4 | GetOptFloat4 (const Util::String &attr, const Math::float4 &defaultValue) const |
get optional float4 attribute value from current node | |
Math::matrix44 | GetOptMatrix44 (const Util::String &attr, const Math::matrix44 &defaultValue) const |
get optional matrix44 attribute value from current node | |
void | SetStream (const Ptr< Stream > &s) |
set stream to read from | |
const Ptr< Stream > & | GetStream () const |
get currently set stream | |
bool | HasStream () const |
return true if a stream is set | |
bool | Eof () const |
return true if the stream has reached EOF | |
bool | IsOpen () const |
return true if currently open | |
int | GetRefCount () const |
get the current refcount | |
void | AddRef () |
increment refcount by one | |
void | Release () |
decrement refcount and destroy object if refcount is zero | |
bool | IsInstanceOf (const Rtti &rtti) const |
return true if this object is instance of given class | |
bool | IsInstanceOf (const Util::String &className) const |
return true if this object is instance of given class by string | |
bool | IsInstanceOf (const Util::FourCC &classFourCC) const |
return true if this object is instance of given class by fourcc | |
bool | IsA (const Rtti &rtti) const |
return true if this object is instance of given class, or a derived class | |
bool | IsA (const Util::String &rttiName) const |
return true if this object is instance of given class, or a derived class, by string | |
bool | IsA (const Util::FourCC &rttiFourCC) const |
return true if this object is instance of given class, or a derived class, by fourcc | |
const Util::String & | GetClassName () const |
get the class name | |
Util::FourCC | GetClassFourCC () const |
get the class FourCC code | |
Static Public Member Functions | |
static void | DumpRefCountingLeaks () |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!) |
Member Function Documentation
bool IO::XmlReader::Open | ( | ) | [virtual] |
begin reading from the stream
Opens the stream and reads the content of the stream into TinyXML.
Reimplemented from IO::StreamReader.
bool IO::XmlReader::HasNode | ( | const Util::String & | path | ) | const |
return true if node exists
This method returns true if the node identified by path exists. Path follows the normal filesystem path conventions, "/" is the separator, ".." is the parent node, "." is the current node. An absolute path starts with a "/", a relative path doesn't.
String IO::XmlReader::GetCurrentNodeName | ( | ) | const |
get short name of current node
Get the short name (without path) of the current node.
String IO::XmlReader::GetCurrentNodePath | ( | ) | const |
get path to current node
This returns the full absolute path of the current node. Path components are separated by slashes.
int IO::XmlReader::GetCurrentNodeLineNumber | ( | ) | const |
returns the line number of the current node
This method returns the line number of the current node.
void IO::XmlReader::SetToNode | ( | const Util::String & | path | ) |
set current node as path
Set the node pointed to by the path string as current node. The path may be absolute or relative, following the usual filesystem path conventions. Separator is a slash.
bool IO::XmlReader::SetToFirstChild | ( | const Util::String & | name = "" |
) |
set current node to first child node, return false if no child exists
Sets the current node to the first child node. If no child node exists, the current node will remain unchanged and the method will return false. If name is a valid string, only child element matching the name will be returned. If name is empty, all child nodes will be considered.
bool IO::XmlReader::SetToNextChild | ( | const Util::String & | name = "" |
) |
set current node to next sibling node, return false if no more sibling exists
Sets the current node to the next sibling. If no more children exist, the current node will be reset to the parent node and the method will return false. If name is a valid string, only child element matching the name will be returned. If name is empty, all child nodes will be considered.
bool IO::XmlReader::SetToParent | ( | ) |
set current node to parent, return false if no parent exists
Sets the current node to its parent. If no parent exists, the current node will remain unchanged and the method will return false.
bool IO::XmlReader::HasAttr | ( | const Util::String & | attr | ) | const |
return true if matching attribute exists on current node
Return true if an attribute of the given name exists on the current node.
String IO::XmlReader::GetString | ( | const Util::String & | attr | ) | const |
get string attribute value from current node
Return the provided attribute as string. If the attribute does not exist the method will fail hard (use HasAttr() to check for its existance).
bool IO::XmlReader::GetBool | ( | const Util::String & | attr | ) | const |
get bool attribute value from current node
Return the provided attribute as a bool. If the attribute does not exist the method will fail hard (use HasAttr() to check for its existance).
int IO::XmlReader::GetInt | ( | const Util::String & | attr | ) | const |
get int attribute value from current node
Return the provided attribute as int. If the attribute does not exist the method will fail hard (use HasAttr() to check for its existance).
float IO::XmlReader::GetFloat | ( | const Util::String & | attr | ) | const |
get float attribute value from current node
Return the provided attribute as float. If the attribute does not exist the method will fail hard (use HasAttr() to check for its existance).
float4 IO::XmlReader::GetFloat4 | ( | const Util::String & | attr | ) | const |
get float4 attribute value from current node
Return the provided attribute as float4. If the attribute does not exist the method will fail hard (use HasAttr() to check for its existance).
matrix44 IO::XmlReader::GetMatrix44 | ( | const Util::String & | attr | ) | const |
get matrix44 attribute value from current node
Return the provided attribute as matrix44. If the attribute does not exist the method will fail hard (use HasAttr() to check for its existance).
String IO::XmlReader::GetOptString | ( | const Util::String & | attr, | |
const Util::String & | defaultValue | |||
) | const |
get optional string attribute value from current node
Return the provided optional attribute as string. If the attribute doesn't exist, the default value will be returned.
bool IO::XmlReader::GetOptBool | ( | const Util::String & | attr, | |
bool | defaultValue | |||
) | const |
get optional bool attribute value from current node
Return the provided optional attribute as bool. If the attribute doesn't exist, the default value will be returned.
int IO::XmlReader::GetOptInt | ( | const Util::String & | attr, | |
int | defaultValue | |||
) | const |
get optional int attribute value from current node
Return the provided optional attribute as int. If the attribute doesn't exist, the default value will be returned.
float IO::XmlReader::GetOptFloat | ( | const Util::String & | attr, | |
float | defaultValue | |||
) | const |
get optional float attribute value from current node
Return the provided optional attribute as float. If the attribute doesn't exist, the default value will be returned.
float4 IO::XmlReader::GetOptFloat4 | ( | const Util::String & | attr, | |
const Math::float4 & | defaultValue | |||
) | const |
get optional float4 attribute value from current node
Return the provided optional attribute as float4. If the attribute doesn't exist, the default value will be returned.
matrix44 IO::XmlReader::GetOptMatrix44 | ( | const Util::String & | attr, | |
const Math::matrix44 & | defaultValue | |||
) | const |
get optional matrix44 attribute value from current node
Return the provided optional attribute as matrix44. If the attribute doesn't exist, the default value will be returned.
set stream to read from
Attaches the reader to a stream. This will imcrement the refcount of the stream.
Reimplemented in Messaging::MessageReader.
get currently set stream
Get pointer to the attached stream. If there is no stream attached, an assertion will be thrown. Use HasStream() to determine if a stream is attached.
bool IO::StreamReader::HasStream | ( | ) | const [inherited] |
return true if a stream is set
Returns true if a stream is attached to the reader.
int Core::RefCounted::GetRefCount | ( | ) | const [inline, inherited] |
get the current refcount
Return the current refcount of the object.
void Core::RefCounted::AddRef | ( | ) | [inline, inherited] |
increment refcount by one
Increment the refcount of the object.
void Core::RefCounted::Release | ( | ) | [inline, inherited] |
decrement refcount and destroy object if refcount is zero
Decrement the refcount and destroy object if refcount is zero.
const Util::String & Core::RefCounted::GetClassName | ( | ) | const [inline, inherited] |
get the class name
Get the class name of the object.
Util::FourCC Core::RefCounted::GetClassFourCC | ( | ) | const [inline, inherited] |
get the class FourCC code
Get the class FourCC of the object.
void Core::RefCounted::DumpRefCountingLeaks | ( | ) | [static, inherited] |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!)
This method should be called as the very last before an application exits.