Models::StateNode Class Reference
#include <statenode.h>
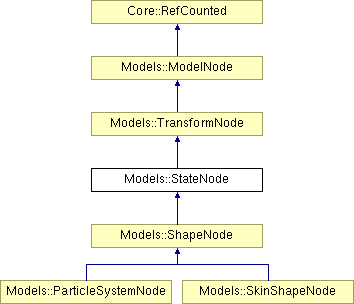
Detailed Description
A model node which holds shader state information and applies shared shader state for its set of StateNodeInstances.(C) 2007 Radon Labs GmbH
Public Member Functions | |
StateNode () | |
constructor | |
virtual | ~StateNode () |
destructor | |
virtual Resources::Resource::State | GetResourceState () const |
get overall state of contained resources (Initial, Loaded, Pending, Failed, Cancelled) | |
virtual void | ApplySharedState () |
apply state shared by all my ModelNodeInstances | |
const Ptr < CoreGraphics::ShaderInstance > & | GetShaderInstance () const |
get pointer to contained shader instance | |
void | SetPosition (const Math::point &p) |
set position | |
const Math::point & | GetPosition () const |
get position | |
void | SetRotate (const Math::quaternion &r) |
set rotate quaternion | |
const Math::quaternion & | GetRotate () const |
get rotate quaternion | |
void | SetScale (const Math::vector &s) |
set scale | |
const Math::vector & | GetScale () const |
get scale | |
void | SetRotatePivot (const Math::point &p) |
set rotate pivot | |
const Math::point & | GetRotatePivot () const |
get rotate pivot | |
void | SetScalePivot (const Math::point &p) |
set scale pivot | |
const Math::point & | GetScalePivot () const |
get scale pivot | |
const Util::Atom < Util::String > & | GetName () const |
get model node name | |
ModelNodeType::Code | GetType () const |
get the ModelNodeType | |
bool | HasParent () const |
return true if node has a parent | |
const Ptr< ModelNode > & | GetParent () const |
get parent node | |
const Util::Array < Ptr< ModelNode > > & | GetChildren () const |
get child nodes | |
bool | IsAttachedToModel () const |
return true if currently attached to a Model | |
const Ptr< Model > & | GetModel () const |
get model this node is attached to | |
const Math::bbox & | GetBoundingBox () const |
get bounding box of model node | |
const Attr::AttributeContainer & | GetAttrs () const |
read access to model node attributes | |
bool | HasAttr (const Attr::AttrId &attrId) const |
check if model node attribute exists | |
void | SetAttr (const Attr::Attribute &attr) |
set generic model node attribute | |
const Attr::Attribute & | GetAttr (const Attr::AttrId &attrId) const |
get generic model node attribute | |
void | SetBool (const Attr::BoolAttrId &attrId, bool val) |
set bool value | |
bool | GetBool (const Attr::BoolAttrId &attrId) const |
get bool value | |
void | SetFloat (const Attr::FloatAttrId &attrId, float val) |
set float value | |
float | GetFloat (const Attr::FloatAttrId &attrId) const |
get float value | |
void | SetInt (const Attr::IntAttrId &attrId, int val) |
set int value | |
int | GetInt (const Attr::IntAttrId &attrId) const |
get int value | |
void | SetString (const Attr::StringAttrId &attrId, const Util::String &val) |
set string value | |
const Util::String & | GetString (const Attr::StringAttrId &attrId) const |
get string value | |
void | SetFloat4 (const Attr::Float4AttrId &attrId, const Math::float4 &val) |
set float4 value | |
Math::float4 | GetFloat4 (const Attr::Float4AttrId &attrId) const |
get float4 value | |
void | SetMatrix44 (const Attr::Matrix44AttrId &attrId, const Math::matrix44 &val) |
set matrix44 value | |
const Math::matrix44 & | GetMatrix44 (const Attr::Matrix44AttrId &attrId) const |
get matrix44 value | |
void | SetGuid (const Attr::GuidAttrId &attrId, const Util::Guid &guid) |
set guid value | |
const Util::Guid & | GetGuid (const Attr::GuidAttrId &attrId) const |
get guid value | |
void | SetBlob (const Attr::BlobAttrId &attrId, const Util::Blob &blob) |
set blob value | |
const Util::Blob & | GetBlob (const Attr::BlobAttrId &attrId) const |
get blob value | |
void | SetName (const Util::Atom< Util::String > &n) |
set model node name | |
void | SetType (ModelNodeType::Code t) |
set ModelNodeType | |
void | SetParent (const Ptr< ModelNode > &p) |
set parent node | |
void | AddChild (const Ptr< ModelNode > &c) |
add a child node | |
void | AddVisibleNodeInstance (IndexT frameIndex, const Ptr< ModelNodeInstance > &nodeInst) |
called by model node instance on NotifyVisible() | |
int | GetRefCount () const |
get the current refcount | |
void | AddRef () |
increment refcount by one | |
void | Release () |
decrement refcount and destroy object if refcount is zero | |
bool | IsInstanceOf (const Rtti &rtti) const |
return true if this object is instance of given class | |
bool | IsInstanceOf (const Util::String &className) const |
return true if this object is instance of given class by string | |
bool | IsInstanceOf (const Util::FourCC &classFourCC) const |
return true if this object is instance of given class by fourcc | |
bool | IsA (const Rtti &rtti) const |
return true if this object is instance of given class, or a derived class | |
bool | IsA (const Util::String &rttiName) const |
return true if this object is instance of given class, or a derived class, by string | |
bool | IsA (const Util::FourCC &rttiFourCC) const |
return true if this object is instance of given class, or a derived class, by fourcc | |
const Util::String & | GetClassName () const |
get the class name | |
Util::FourCC | GetClassFourCC () const |
get the class FourCC code | |
Static Public Member Functions | |
static void | DumpRefCountingLeaks () |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!) | |
Protected Member Functions | |
virtual Ptr < ModelNodeInstance > | CreateNodeInstance () const |
create a model node instance | |
virtual void | LoadResources () |
called when resources should be loaded | |
virtual void | UnloadResources () |
called when resources should be unloaded | |
void | SetupManagedTextureVariable (const Attr::AttrId &resAttrId, const Ptr< CoreGraphics::ShaderVariable > &var) |
setup a new managed texture variable | |
void | UpdateManagedTextureVariables () |
update managed texture variables | |
virtual void | LoadFromAttrs (const Attr::AttributeContainer &attrs) |
called to initialize from attributes | |
virtual void | SaveToAttrs (Attr::AttributeContainer &attrs) |
called to save state back to attributes | |
void | SetBoundingBox (const Math::bbox &b) |
set bounding box | |
virtual void | OnAttachToModel (const Ptr< Model > &model) |
called when attached to model node | |
virtual void | OnRemoveFromModel () |
called when removed from model node | |
const Util::Array < Ptr < ModelNodeInstance > > & | GetVisibleModelNodeInstances (ModelNodeType::Code t) const |
get visible model node instances |
Member Function Documentation
void Models::StateNode::SetupManagedTextureVariable | ( | const Attr::AttrId & | resAttrId, | |
const Ptr< CoreGraphics::ShaderVariable > & | var | |||
) | [protected] |
setup a new managed texture variable
Create a new managed texture resource and bind it to the provided shader variable.
void Models::StateNode::UpdateManagedTextureVariables | ( | ) | [protected] |
update managed texture variables
This method transfers texture from our managed texture objects into their associated shader variable. This is necessary since the actual texture of a managed texture may change from frame to frame because of resource management.
int Core::RefCounted::GetRefCount | ( | ) | const [inline, inherited] |
get the current refcount
Return the current refcount of the object.
void Core::RefCounted::AddRef | ( | ) | [inline, inherited] |
increment refcount by one
Increment the refcount of the object.
void Core::RefCounted::Release | ( | ) | [inline, inherited] |
decrement refcount and destroy object if refcount is zero
Decrement the refcount and destroy object if refcount is zero.
const Util::String & Core::RefCounted::GetClassName | ( | ) | const [inline, inherited] |
get the class name
Get the class name of the object.
Util::FourCC Core::RefCounted::GetClassFourCC | ( | ) | const [inline, inherited] |
get the class FourCC code
Get the class FourCC of the object.
void Core::RefCounted::DumpRefCountingLeaks | ( | ) | [static, inherited] |
dump refcounting leaks, call at end of application (NEBULA3_DEBUG builds only!)
This method should be called as the very last before an application exits.